This article brings you a brief discussion of decorators in ES6. It has certain reference value. Friends in need can refer to it. I hope it will be helpful to you.
Decorator
Decorator is mainly used for:
Decoration class
Decoration method or attribute
Decoration class
@annotation class MyClass { } function annotation(target) { target.annotated = true; }
Decoration method or attribute
class MyClass { @readonly method() { } } function readonly(target, name, descriptor) { descriptor.writable = false; return descriptor; }
Babel
Installation and compilation
We can install it in Babel Try it out on the official website to view the Babel compiled code.
But we can also choose local compilation:
npm init npm install --save-dev @babel/core @babel/cli npm install --save-dev @babel/plugin-proposal-decorators @babel/plugin-proposal-class-properties
Create a new .babelrc file
{ "plugins": [ ["@babel/plugin-proposal-decorators", { "legacy": true }], ["@babel/plugin-proposal-class-properties", {"loose": true}] ] }
Compile the specified file
babel decorator.js --out-file decorator-compiled.js
Compilation of decoration classes
Before compilation:
@annotation class MyClass { } function annotation(target) { target.annotated = true; }
After compilation:
var _class; let MyClass = annotation(_class = class MyClass {}) || _class; function annotation(target) { target.annotated = true; }
We can see the decoration of the class, the principle is:
@decorator class A {} // 等同于 class A {} A = decorator(A) || A;
Compilation of decoration methods
Before compilation:
class MyClass { @unenumerable @readonly method() { } } function readonly(target, name, descriptor) { descriptor.writable = false; return descriptor; } function unenumerable(target, name, descriptor) { descriptor.enumerable = false; return descriptor; }
After compilation:
var _class; function _applyDecoratedDescriptor(target, property, decorators, descriptor, context ) { /** * 第一部分 * 拷贝属性 */ var desc = {}; Object["ke" + "ys"](descriptor).forEach(function(key) { desc[key] = descriptor[key]; }); desc.enumerable = !!desc.enumerable; desc.configurable = !!desc.configurable; if ("value" in desc || desc.initializer) { desc.writable = true; } /** * 第二部分 * 应用多个 decorators */ desc = decorators .slice() .reverse() .reduce(function(desc, decorator) { return decorator(target, property, desc) || desc; }, desc); /** * 第三部分 * 设置要 decorators 的属性 */ if (context && desc.initializer !== void 0) { desc.value = desc.initializer ? desc.initializer.call(context) : void 0; desc.initializer = undefined; } if (desc.initializer === void 0) { Object["define" + "Property"](target, property, desc); desc = null; } return desc; } let MyClass = ((_class = class MyClass { method() {} }), _applyDecoratedDescriptor( _class.prototype, "method", [readonly], Object.getOwnPropertyDescriptor(_class.prototype, "method"), _class.prototype ), _class); function readonly(target, name, descriptor) { descriptor.writable = false; return descriptor; }
Compiled source code analysis of decoration method
We can see that Babel has built an _applyDecoratedDescriptor function for Decorate the method.
Object.getOwnPropertyDescriptor()
When passing in parameters, we use an Object.getOwnPropertyDescriptor() method. Let’s take a look at this method:
Object. The getOwnPropertyDescriptor() method returns the property descriptor corresponding to an own property on the specified object. (Own properties refer to properties that are directly assigned to the object and do not need to be looked up from the prototype chain)
By the way, please note that this is an ES5 method.
For example:
const foo = { value: 1 }; const bar = Object.getOwnPropertyDescriptor(foo, "value"); // bar { // value: 1, // writable: true // enumerable: true, // configurable: true, // } const foo = { get value() { return 1; } }; const bar = Object.getOwnPropertyDescriptor(foo, "value"); // bar { // get: /*the getter function*/, // set: undefined // enumerable: true, // configurable: true, // }
The first part of the source code analysis
Inside the _applyDecoratedDescriptor function, we first make a property descriptor object returned by Object.getOwnPropertyDescriptor() Copy:
// 拷贝一份 descriptor var desc = {}; Object["ke" + "ys"](descriptor).forEach(function(key) { desc[key] = descriptor[key]; }); desc.enumerable = !!desc.enumerable; desc.configurable = !!desc.configurable; // 如果没有 value 属性或者没有 initializer 属性,表明是 getter 和 setter if ("value" in desc || desc.initializer) { desc.writable = true; }
So what is the initializer attribute? The object returned by Object.getOwnPropertyDescriptor() does not have this property. Indeed, this is a property generated by Babel's Class in order to cooperate with the decorator. For example, for the following code:
class MyClass { @readonly born = Date.now(); } function readonly(target, name, descriptor) { descriptor.writable = false; return descriptor; } var foo = new MyClass(); console.log(foo.born);
Babel will Compiled as:
// ... (_descriptor = _applyDecoratedDescriptor(_class.prototype, "born", [readonly], { configurable: true, enumerable: true, writable: true, initializer: function() { return Date.now(); } })) // ...
At this time, the descriptor passed into the _applyDecoratedDescriptor function has the initializer attribute.
The second part of source code analysis
The next step is to apply multiple decorators:
/** * 第二部分 * @type {[type]} */ desc = decorators .slice() .reverse() .reduce(function(desc, decorator) { return decorator(target, property, desc) || desc; }, desc);
For one method, multiple decorators are applied, such as:
class MyClass { @unenumerable @readonly method() { } }
Babel will compile to:
_applyDecoratedDescriptor( _class.prototype, "method", [unenumerable, readonly], Object.getOwnPropertyDescriptor(_class.prototype, "method"), _class.prototype )
In the second part of the source code, reverse() and reduce() operations are performed. From this, we can also find that if the same method has multiple decorators, it will be Execute from the inside out.
Part 3 Source Code Analysis
/** * 第三部分 * 设置要 decorators 的属性 */ if (context && desc.initializer !== void 0) { desc.value = desc.initializer ? desc.initializer.call(context) : void 0; desc.initializer = undefined; } if (desc.initializer === void 0) { Object["define" + "Property"](target, property, desc); desc = null; } return desc;
If desc has an initializer attribute, it means that when the class attribute is decorated, the value of value will be set to:
desc.initializer.call(context)
The value of context is _class.prototype
. The reason why we need to call(context)
is also easy to understand, because it is possible
class MyClass { @readonly value = this.getNum() + 1; getNum() { return 1; } }
In the end, whether it is a decoration method Or attributes, will be executed:
Object["define" + "Property"](target, property, desc);
It can be seen that the decoration method is essentially implemented using Object.defineProperty()
.
Application
1.log
Add the log function to a method and check the input parameters:
class Math { @log add(a, b) { return a + b; } } function log(target, name, descriptor) { var oldValue = descriptor.value; descriptor.value = function(...args) { console.log(`Calling ${name} with`, args); return oldValue.apply(this, args); }; return descriptor; } const math = new Math(); // Calling add with [2, 4] math.add(2, 4);
More perfect:
let log = (type) => { return (target, name, descriptor) => { const method = descriptor.value; descriptor.value = (...args) => { console.info(`(${type}) 正在执行: ${name}(${args}) = ?`); let ret; try { ret = method.apply(target, args); console.info(`(${type}) 成功 : ${name}(${args}) => ${ret}`); } catch (error) { console.error(`(${type}) 失败: ${name}(${args}) => ${error}`); } return ret; } } };
2.autobind
class Person { @autobind getPerson() { return this; } } let person = new Person(); let { getPerson } = person; getPerson() === person; // true
A scenario we can easily think of is when React binds events:
class Toggle extends React.Component { @autobind handleClick() { console.log(this) } render() { return ( <button> button </button> ); } }
Let’s write such an autobind function:
const { defineProperty, getPrototypeOf} = Object; function bind(fn, context) { if (fn.bind) { return fn.bind(context); } else { return function __autobind__() { return fn.apply(context, arguments); }; } } function createDefaultSetter(key) { return function set(newValue) { Object.defineProperty(this, key, { configurable: true, writable: true, enumerable: true, value: newValue }); return newValue; }; } function autobind(target, key, { value: fn, configurable, enumerable }) { if (typeof fn !== 'function') { throw new SyntaxError(`@autobind can only be used on functions, not: ${fn}`); } const { constructor } = target; return { configurable, enumerable, get() { /** * 使用这种方式相当于替换了这个函数,所以当比如 * Class.prototype.hasOwnProperty(key) 的时候,为了正确返回 * 所以这里做了 this 的判断 */ if (this === target) { return fn; } const boundFn = bind(fn, this); defineProperty(this, key, { configurable: true, writable: true, enumerable: false, value: boundFn }); return boundFn; }, set: createDefaultSetter(key) }; }
3 .debounce
Sometimes, we need to perform anti-shake processing on the executed method:
class Toggle extends React.Component { @debounce(500, true) handleClick() { console.log('toggle') } render() { return ( <button> button </button> ); } }
Let’s implement it:
function _debounce(func, wait, immediate) { var timeout; return function () { var context = this; var args = arguments; if (timeout) clearTimeout(timeout); if (immediate) { var callNow = !timeout; timeout = setTimeout(function(){ timeout = null; }, wait) if (callNow) func.apply(context, args) } else { timeout = setTimeout(function(){ func.apply(context, args) }, wait); } } } function debounce(wait, immediate) { return function handleDescriptor(target, key, descriptor) { const callback = descriptor.value; if (typeof callback !== 'function') { throw new SyntaxError('Only functions can be debounced'); } var fn = _debounce(callback, wait, immediate) return { ...descriptor, value() { fn() } }; } }
4.time
Used to count method execution time:
function time(prefix) { let count = 0; return function handleDescriptor(target, key, descriptor) { const fn = descriptor.value; if (prefix == null) { prefix = `${target.constructor.name}.${key}`; } if (typeof fn !== 'function') { throw new SyntaxError(`@time can only be used on functions, not: ${fn}`); } return { ...descriptor, value() { const label = `${prefix}-${count}`; count++; console.time(label); try { return fn.apply(this, arguments); } finally { console.timeEnd(label); } } } } }
5.mixin
Used to mix object methods into Class:
const SingerMixin = { sing(sound) { alert(sound); } }; const FlyMixin = { // All types of property descriptors are supported get speed() {}, fly() {}, land() {} }; @mixin(SingerMixin, FlyMixin) class Bird { singMatingCall() { this.sing('tweet tweet'); } } var bird = new Bird(); bird.singMatingCall(); // alerts "tweet tweet"
A simple implementation of mixin is as follows:
function mixin(...mixins) { return target => { if (!mixins.length) { throw new SyntaxError(`@mixin() class ${target.name} requires at least one mixin as an argument`); } for (let i = 0, l = mixins.length; i <h3 id="redux">6.redux</h3><p>In actual development, when React is used in combination with the Redux library, it is often necessary to write it as follows. </p><pre class="brush:php;toolbar:false">class MyReactComponent extends React.Component {} export default connect(mapStateToProps, mapDispatchToProps)(MyReactComponent);
With the decorator, you can rewrite the above code.
@connect(mapStateToProps, mapDispatchToProps) export default class MyReactComponent extends React.Component {};
Relatively speaking, the latter way of writing seems easier to understand.
7. Note
The above are all used to modify class methods. The way we get the value is:
const method = descriptor.value;
But if we modify the instance attribute of the class, Because of Babel, the value cannot be obtained through the value attribute. We can write it as:
const value = descriptor.initializer && descriptor.initializer();
The above is the detailed content of A brief discussion on decorators in ES6. For more information, please follow other related articles on the PHP Chinese website!
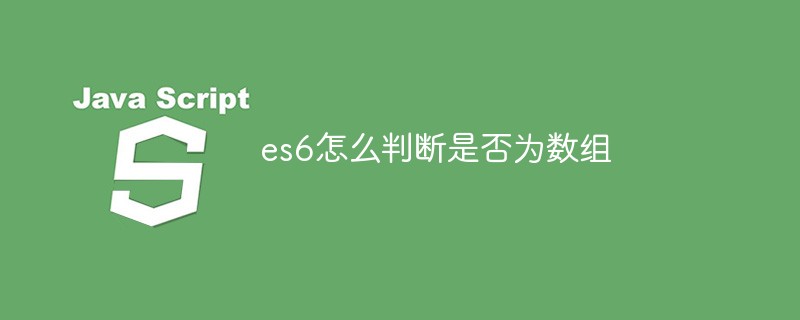
在es6中,可以利用“Array.isArray()”方法判断对象是否为数组,若判断的对象是数组,返回的结果是true,若判断对象不是数组,返回的结果是false,语法为“Array.isArray(需要检测的js对象)”。
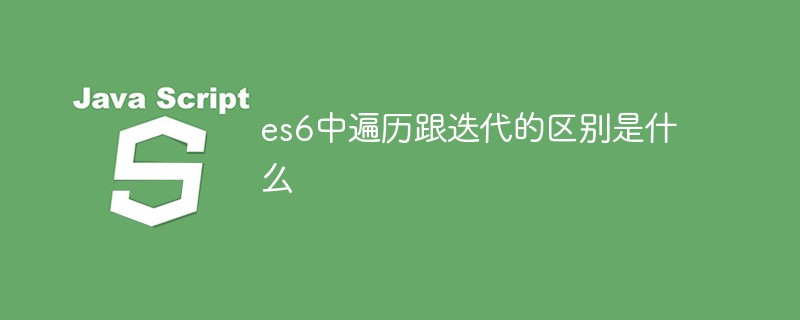
es6中遍历跟迭代的区别是:遍历强调的是要把整个数据依次全部取出来,是访问数据结构的所有元素;而迭代虽然也是依次取出数据,但是并不保证取多少,也不保证把所有的数据取完,是遍历的一种形式。
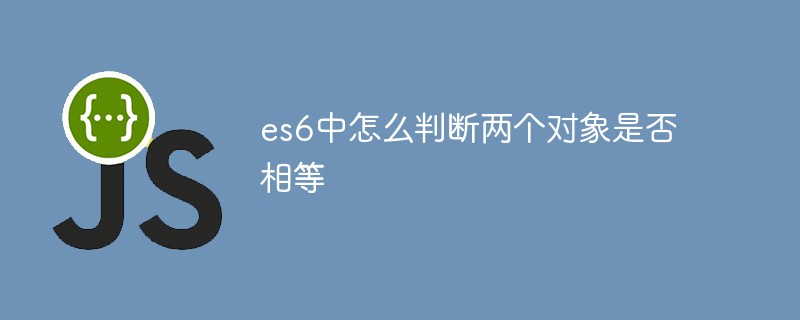
在es6中,可用Object对象的is()方法来判断两个对象是否相等,该方法检测两个变量的值是否为同一个值,判断两个对象的引用地址是否一致,语法“Object.is(对象1,对象2)”;该方法会返回布尔值,若返回true则表示两个对象相等。
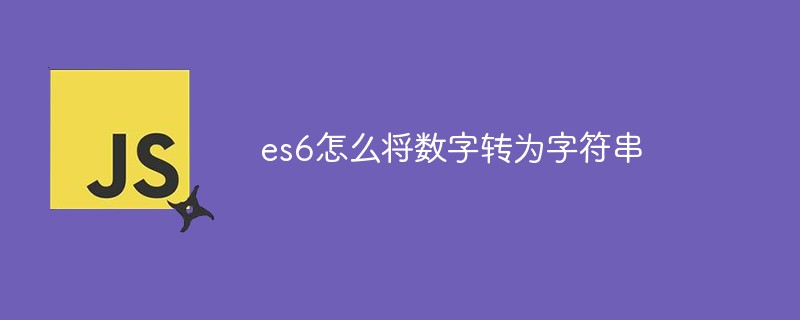
转换方法:1、利用“+”给数字拼接一个空字符,语法“数字+""”;2、使用String(),可把对象的值转换为字符串,语法“String(数字对象)”;3、用toString(),可返回数字的字符串表示,语法“数字.toString()”。
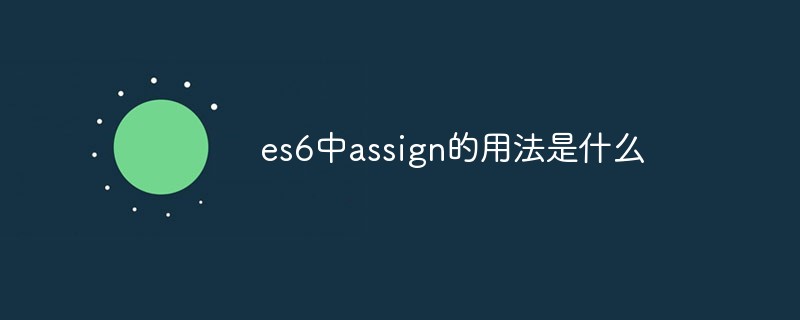
在es6中,assign用于对象的合并,可以将源对象的所有可枚举属性复制到目标对象;若目标对象与源对象有同名属性,或多个源对象有同名属性,则后面的属性会覆盖前面的属性,语法为“Object.assign(...)”
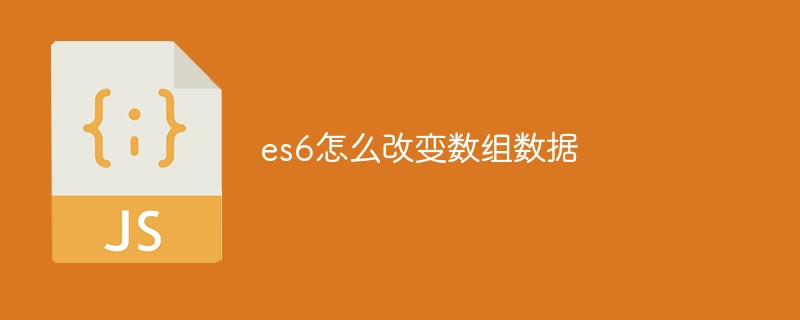
改变方法:1、利用splice()方法修改,该方法可以直接修改原数组的内容,语法为“数组.splice(开始位置,修改个数,修改后的值)”;2、利用下标访问数组元素,并重新赋值来修改数组数据,语法为“数组[下标值]=修改后的值;”。
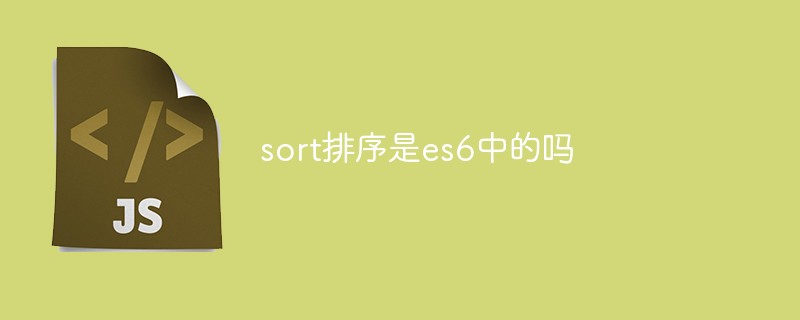
sort排序是es6中的;sort排序是es6中用于对数组的元素进行排序的方法,该方法默认不传参,按照字符编码顺序进行排序,排序顺序可以是字母或数字,并按升序或降序,语法为“array.sort(callback(a,b))”。
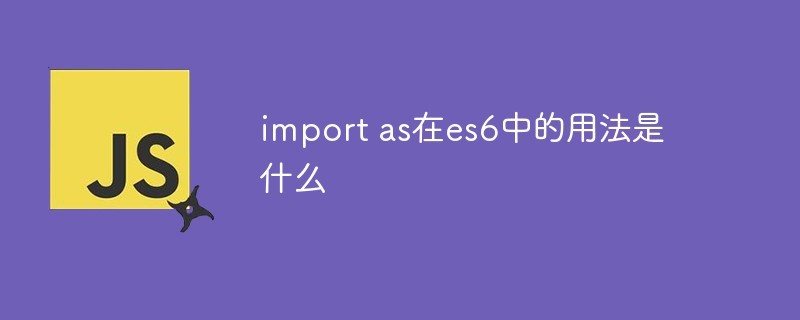
在es6中,import as用于将若干export导出的内容组合成一个对象返回;ES6的模块化分为导出与导入两个模块,该方法能够将所有的导出内容包裹到指定对象中,语法为“import * as 对象 from ...”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
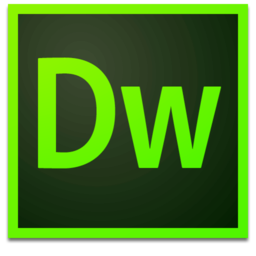
Dreamweaver Mac version
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor
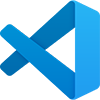
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Chinese version
Chinese version, very easy to use
