Detailed explanation of examples of set-map data structure in ES6
1. Set data structure (use new to create a set collection, add elements through the add method, and obtain the length of the set collection through size)
{ let list = new Set(); list.add(5); list.add(7); console.log('size',list.size); }
There is another way to initialize (by passing the array directly)
{ let arr = [1,2,3,4,5]; let list = new Set(arr); console.log('size',list.size); }
The elements in set cannot be repeated (Array deduplication can be carried out through this feature of set. Note: data type conversion will not be performed during the conversion process)
{ let list = new Set(); list.add(1); list.add(2); list.add(1);//不会报错 只是不会生效 console.log('list',list); let arr=[1,2,3,1,'2']; let list2=new Set(arr); console.log('unique',list2); }
Some methods of set (add, delete, clear, has)
{ let arr=['add','delete','clear','has']; let list=new Set(arr); console.log('has',list.has('add'));//是否包含 console.log('delete',list.delete('add'),list);//清空 list.clear(); console.log('list',list); }
Traversal of set (keys and values return the values in the set)
{ let arr=['add','delete','clear','has']; let list=new Set(arr); for(let key of list.keys()){ console.log('keys',key); } for(let value of list.values()){ console.log('value',value); } for(let [key,value] of list.entries()){ console.log('entries',key,value); } list.forEach(function(item){console.log(item);}) }
WeakSet (the elements inside can only be objects, and it will not detect whether the added object is referenced elsewhere, and traversal is not supported without size clear)
{ let weakList=new WeakSet(); let arg={}; weakList.add(arg); // weakList.add(2); console.log('weakList',weakList); }
2. Map data structure (Map is set through key/value, so the set method is used for setting and the get method is used for obtaining)
{ let map = new Map(); let arr=['123']; map.set(arr,456); console.log('map',map,map.get(arr)); }
Another way to define Map (at the same time the size delete clear method is the same)
{ let map = new Map([['a',123],['b',456]]); console.log('map args',map); console.log('size',map.size); console.log('delete',map.delete('a'),map); console.log('clear',map.clear(),map); }
weakMap data structure (the same characteristics as setMap)
{ let weakmap=new WeakMap(); let o={}; weakmap.set(o,123); console.log(weakmap.get(o)); }
The above is the detailed content of Detailed explanation of examples of set-map data structure in ES6. For more information, please follow other related articles on the PHP Chinese website!
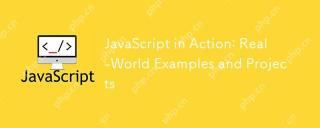
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
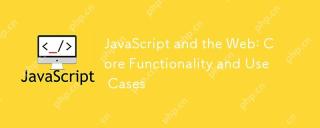
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
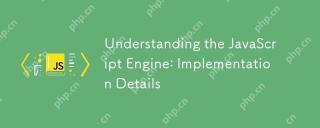
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
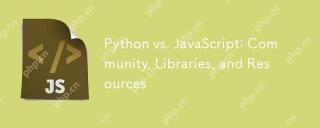
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
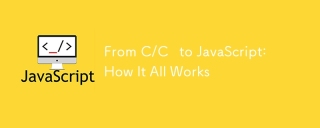
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
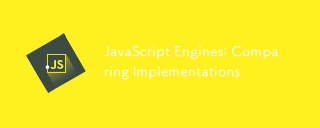
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
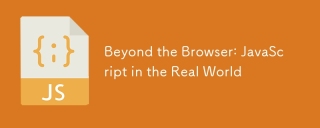
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
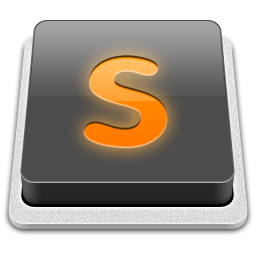
SublimeText3 Mac version
God-level code editing software (SublimeText3)
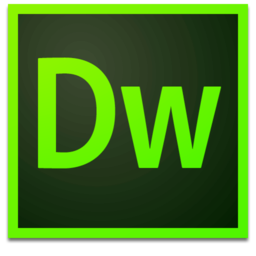
Dreamweaver Mac version
Visual web development tools
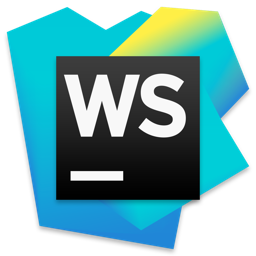
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment