How to use Jest to test JavaScript (Mock function)
The content of this article is about how to use Jest to test JavaScript (Mock function). It has certain reference value. Friends in need can refer to it. I hope it will be helpful to you.
In this tutorial, we will introduce three APIs related to Mock functions in Jest, namely jest.fn(), jest.spyOn(), and jest.mock(). Using them to create Mock functions can help us better test some of the more logically complex codes in the project, such as nested calls of test functions, calls of callback functions, etc.
If you don’t know the basic usage of Jest, please read first: http://www.php.cn/js-tutorial-411835.html
Why use Mock function?
In projects, methods of one module often call methods of another module. In unit testing, we may not need to care about the execution process and results of the internally called method, we just want to know whether it is called correctly, and even specify the return value of the function. At this time, it is very necessary to use the Mock function.
The following three features provided by the Mock function are very useful when we write test code:
Capture the function call situation
Set the function return value
Change the internal implementation of the function
We then use the directory structure in the previous article, in test/functions Write the test code in the .test.js file, and write the tested code in the src/ directory.
1. jest.fn()
jest.fn() is the simplest way to create a Mock function. If the internal implementation of the function is not defined, jest.fn () will return undefined as the return value.
// functions.test.js test('测试jest.fn()调用', () => { let mockFn = jest.fn(); let result = mockFn(1, 2, 3); // 断言mockFn的执行后返回undefined expect(result).toBeUndefined(); // 断言mockFn被调用 expect(mockFn).toBeCalled(); // 断言mockFn被调用了一次 expect(mockFn).toBeCalledTimes(1); // 断言mockFn传入的参数为1, 2, 3 expect(mockFn).toHaveBeenCalledWith(1, 2, 3); })
The Mock function created by jest.fn() can also set a return value, define an internal implementation or return a Promise object.
// functions.test.js test('测试jest.fn()返回固定值', () => { let mockFn = jest.fn().mockReturnValue('default'); // 断言mockFn执行后返回值为default expect(mockFn()).toBe('default'); }) test('测试jest.fn()内部实现', () => { let mockFn = jest.fn((num1, num2) => { return num1 * num2; }) // 断言mockFn执行后返回100 expect(mockFn(10, 10)).toBe(100); }) test('测试jest.fn()返回Promise', async () => { let mockFn = jest.fn().mockResolvedValue('default'); let result = await mockFn(); // 断言mockFn通过await关键字执行后返回值为default expect(result).toBe('default'); // 断言mockFn调用后返回的是Promise对象 expect(Object.prototype.toString.call(mockFn())).toBe("[object Promise]"); })
The above code is several commonly used APIs and assertion statements provided by jest.fn(). Next, we write some tested code in the src/fetch.js file to be closer to the business. Understand the practical application of Mock function.
The tested code relies on axios, a commonly used request library, and JSONPlaceholder, a free request interface mentioned in the previous article. Please first execute npm install axios --save in the shell to install the dependencies.
// fetch.js import axios from 'axios'; export default { async fetchPostsList(callback) { return axios.get('https://jsonplaceholder.typicode.com/posts').then(res => { return callback(res.data); }) } }
We encapsulate a fetchPostsList method in fetch.js, which requests the interface provided by JSONPlaceholder and returns the processed return value through the incoming callback function. If we want to test that the interface can be requested normally, we only need to capture that the incoming callback function can be called normally. Below is the code for the test in functions.test.js.
import fetch from '../src/fetch.js' test('fetchPostsList中的回调函数应该能够被调用', async () => { expect.assertions(1); let mockFn = jest.fn(); await fetch.fetchPostsList(mockFn); // 断言mockFn被调用 expect(mockFn).toBeCalled(); })
2. jest.mock()
The request method encapsulated in the fetch.js folder may not need to be performed when other modules are called. The actual request (the request method has passed one side or requires the method to return non-real data). At this time, it is very necessary to use jest.mock() to mock the entire module.
Next we create a src/events.js in the same directory as src/fetch.js.
// events.js import fetch from './fetch'; export default { async getPostList() { return fetch.fetchPostsList(data => { console.log('fetchPostsList be called!'); // do something }); } }
The test code in functions.test.js is as follows:
// functions.test.js import events from '../src/events'; import fetch from '../src/fetch'; jest.mock('../src/fetch.js'); test('mock 整个 fetch.js模块', async () => { expect.assertions(2); await events.getPostList(); expect(fetch.fetchPostsList).toHaveBeenCalled(); expect(fetch.fetchPostsList).toHaveBeenCalledTimes(1); });
In the test code we use jest.mock('../src/fetch.js') to mock the entire fetch.js module. If you comment out this line of code, the following error message will appear when executing the test script
From this error report, we can draw an important conclusion :
If you want to capture the calling status of a function in jest, the function must be mocked or spied!
3. jest.spyOn()
jest.spyOn() method also creates a mock function, but the mock function can not only capture the calling status of the function, but also The spied function can be executed normally. In fact, jest.spyOn() is syntactic sugar for jest.fn(), which creates a mock function with the same internal code as the spied function.
The above picture is a screenshot of the correct execution result in the previous jest.mock() sample code. You can see the console from the shell script. log('fetchPostsList be called!'); This line of code is not printed in the shell. This is because after passing jest.mock(), the methods in the module will not be actually executed by jest. At this time we need to use jest.spyOn().
// functions.test.js import events from '../src/events'; import fetch from '../src/fetch'; test('使用jest.spyOn()监控fetch.fetchPostsList被正常调用', async() => { expect.assertions(2); const spyFn = jest.spyOn(fetch, 'fetchPostsList'); await events.getPostList(); expect(spyFn).toHaveBeenCalled(); expect(spyFn).toHaveBeenCalledTimes(1); })
After executing npm run test, you can see the print information in the shell, indicating that fetchPostsList was executed normally through jest.spyOn().
4. Summary
In this article we introduced jest.fn(), jest .mock() and jest.spyOn() to create mock functions. Through the mock function, we can better write our test code through the following three features:
Capture the function call situation
Set the function return value
Change the internal implementation of the function
In unit testing of actual projects, jest.fn() is often used to perform certain tests with callback functions; jest.mock() can mock methods in the entire module. When a certain When a module has been 100% covered by unit tests, it is necessary to use jest.mock() to mock the module to save test time and test redundancy; when you need to test certain methods that must be fully executed, you often need to use jest.spyOn(). These require developers to make flexible choices based on actual business code.
The above is the detailed content of How to use Jest to test JavaScript (Mock function). For more information, please follow other related articles on the PHP Chinese website!
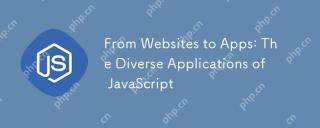
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
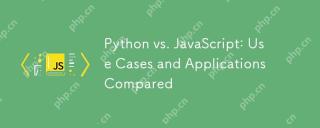
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
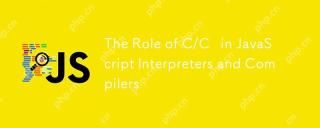
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
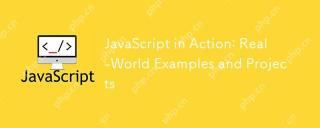
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
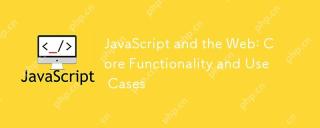
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
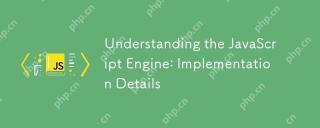
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
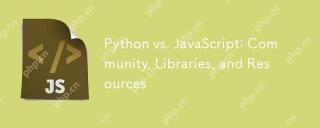
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
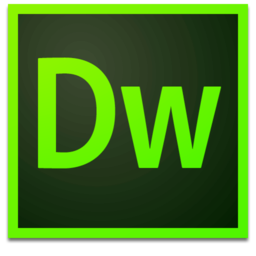
Dreamweaver Mac version
Visual web development tools
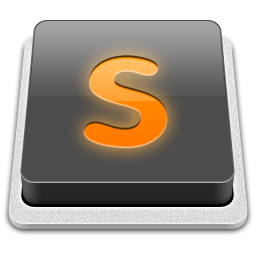
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
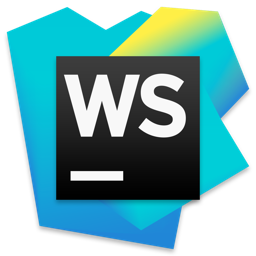
WebStorm Mac version
Useful JavaScript development tools