


What is a collection? Basic introduction to Java collections (with framework diagram)
The content of this article is about what is a collection? A basic introduction to Java collections (with a frame diagram) has certain reference value. Friends in need can refer to it. I hope it will be helpful to you.
What is a collection?
The Java collection class is stored in the java.util package and is a container used to store objects.
Note: ① Collections can only store objects. For example, if you save an int type data 1 into a collection, it is actually converted into the Integer class automatically and then stored. Each basic type in Java has a corresponding reference type.
②. Collections store references to multiple objects, and the objects themselves are still placed in the heap memory.
③. Collections can store different types of data types, with no limit on the number.
Basic concepts of Java collection classes
In programming, it is often necessary to store multiple data in a centralized manner. Traditionally, arrays are a good choice for us, provided we know exactly in advance the number of objects we are going to save. Once the array length is specified during array initialization, the array length is immutable. If we need to save data that can grow dynamically (the specific number cannot be determined at compile time), Java's collection class is a good design. Planned.
Collection classes are mainly responsible for saving and holding other data, so collection classes are also called container classes. All collection classes are located under the java.util package. Later, in order to deal with concurrency security issues in multi-threaded environments, java5 also provided some collection classes with multi-thread support under the java.util.concurrent package.
When learning the API and programming principles of the collection class in Java, we must understand that "set" is a very old mathematical concept, which predates the emergence of Java. Understanding collections from the perspective of mathematical concepts can help us better understand when to use what type of collection class in programming.
The purpose of the Java container class library is to "save objects" and divide them into two different concepts:
1) Collection
A set of "opposing" elements, Usually these elements obey certain rules
1.1) List must maintain a specific order of elements
1.2) Set cannot have duplicate elements
1.3) Queue maintains the order of a queue (first in, first out)
2 ) Map
A set of pairs of "key-value pair" objects
1) Collection can only save one element (object) at each position
2) Map saves "Key-value pairs", like a small database. We can find the "value" corresponding to the key through the "key"
Java collection class architecture hierarchical relationship
1. Interface Iterable
Iterator interface, this is the parent interface of the Collection class. Objects that implement this Iterable interface allow traversal using foreach, that is, all Collection objects have "foreach traversability". This Iterable interface has only
one method: iterator(). It returns a generic
1.1 Collection
Collection is the most basic collection interface. A Collection represents a collection of Objects. These Objects Elements called Collection. Collection is an interface that provides standard definitions and cannot be instantiated for use
1) Set
The Set collection is similar to a jar. There is no obvious order among the multiple objects "thrown" into the Set collection. Set inherits from the Collection interface and cannot contain duplicate elements (remember, this is a common property of the entire Set class hierarchy).
Set determines that two objects are identical instead of using the "==" operator, but based on the equals method. That is to say, when we add a new element, if the equals comparison between the new element object and the existing object in the Set returns false, then Set will accept the new element object, otherwise it will be rejected.
Because of this restriction of Set, when using Set collection, you should pay attention to two points: 1) Implement an effective equals (Object) method for the implementation class of the elements in the Set collection, 2) For the constructor of Set, The passed-in Collection parameter cannot contain duplicate elements
1.1) HashSet
1. HashSet is a typical implementation of the Set interface. HashSet uses the HASH algorithm to store elements in the collection, so it has good access and search capabilities. performance. When an element is stored in the HashSet collection, the HashSet will call the hashCode() method of the object to get the hashCode value of the object, and then determine the storage location of the object in the HashSet based on the HashCode value.
# The LinkedHashSet collection also determines the storage location of the elements based on the hashCode value of the elements, but unlike HashSet, it also uses a linked list to maintain the order of the elements, so that the elements appear to be saved in the order of insertion.
When traversing the elements in the LinkedHashSet collection, LinkedHashSet will access the elements in the collection in the order in which the elements are added.
LinkedHashSet needs to maintain the insertion order of elements, so the performance is slightly lower than that of HashSet, but it will have good performance when iteratively accessing all elements in the Set (traversal) (linked lists are very suitable for traversal)
1.2 ) SortedSet
This interface is mainly used for sorting operations, that is, the subclasses that implement this interface belong to the sorted subclasses
1.2.1) TreeSet
Treeset is an implementation class of the SortedSet interface. In sorting state
1.3) EnumSet
EnumSet is a collection class specially designed for enumeration classes. All elements in EnumSet must be enumeration values of the specified enumeration type. This enumeration type is displayed when creating an EnumSet. formula, or implicitly specified. The collection elements of EnumSet are also ordered,
They determine the order of the collection elements based on the definition order of the enumeration values in the Enum class
2) List
The List collection represents an ordered and repeatable element Set, each element in the set has its corresponding sequential index. The List collection allows adding duplicate elements because it can access the collection element at the specified position through the index. By default, the List collection sets the index of elements in the order in which they are added
2.1) ArrayList
2.1) ArrayList is a List class implemented based on arrays. It encapsulates a dynamically growing Object[] array that allows redistribution .
2.2) Vector
Vector and ArrayList are almost identical in usage, but because Vector is an old collection, Vector provides some methods with long method names, but with JDK1.2 and later, java provides After understanding the collection framework of the system, we will change
to implement the List interface and integrate it into the collection framework system
2.2.1) Stack
Stack is a subclass provided by Vector to simulate the data structure of "stack" (lifo first out)
2.3) linkedlist
Implements list & lt; e & gt;, deque & lt; e & gt; Implement the List interface and perform queue operations on it, that is, you can randomly access elements in the collection based on the index. At the same time, it also implements the Deque interface, that is, LinkedList can be used as a double-ended queue
. Naturally, it can also be used as a "stack"
3) Queue
Queue is used to simulate the data structure of "queue" (first in first out FIFO). The head of the queue holds the element with the longest storage time in the queue, and the tail of the queue holds the element with the shortest storage time in the queue. A new element is inserted (offer) to the end of the queue.
The access element (poll) operation will return the element at the head of the queue. The queue does not allow random access to elements in the queue. This concept will be well understood by combining the common queuing in life
3.1) PriorityQueue
PriorityQueue is not a relatively standard queue implementation. The order in which the queue elements are saved by PriorityQueue is not in the order of joining the queue, but in the order of the queue. The size of the elements is reordered, which can also be seen from its class name
3.2) Deque
The Deque interface represents a "double-ended queue", and the double-ended queue can be added from both ends at the same time , delete elements, so the implementation class of Deque can be used as a queue or a stack. A dynamic, re-allocable Object[] array is used to store the collection elements. When the collection elements exceed the capacity of the array, the system will re-allocate a new Object[] array at the bottom layer to store the collection elements
3.2. 2) LinkedList
1.2 Map
Map is used to save data with "mapping relationships", so the Map collection saves two sets of values, one set of values is used to save the key in the Map, and the other set of values is used to Save the value in the Map. Both key and value can be any reference type of data. Map keys are not
allowed to be repeated, that is, any two keys of the same Map object will always return false when compared with the equals method.
Regarding Map, we need to understand it from the perspective of code reuse. Java first implements Map, and then implements the Set collection by packaging a Map with all values null.
These implementation classes and sub-classes of Map The storage form of the key set in the interface is exactly the same as the Set collection (that is, the key cannot be repeated)
The storage form of the value set in these implementation classes and sub-interfaces of Map is very similar to List (that is, the value can be repeated and searched according to the index)
1) HashMap
Just like the HashSet collection that cannot guarantee the order of elements, HashMap cannot guarantee the order of key-value pairs. And similar to HashSet, the standard for judging whether two keys are equal is: two keys are compared to return true through the equals() method,
At the same time, the hashCode values of the two keys must also be equal
1.1) LinkedHashMap
LinkedHashMap also Use a doubly linked list to maintain the order of key-value pairs. The linked list is responsible for maintaining the iteration order of the Map, which is consistent with the insertion order of key-value pairs (note that it is different from TreeMap in sorting all key-value pairs)
## 2) Hashtable
is an old Map implementation class
2.1) Properties
The Properties object is particularly convenient when processing property files (.ini files on Windows platforms). The Properties class can combine Map objects with The attribute files are associated, so that the key-value pairs in the Map object can be written to the attribute file
, or the "attribute name-attribute value" in the attribute file can be loaded into the Map object
3 ) SortedMap
Just as the Set interface derives a SortedSet sub-interface, and the SortedSet interface has a TreeSet implementation class, the Map interface also derives a SortedMap sub-interface, and the SortedMap interface also has a TreeMap implementation class
3.1) TreeMap
TreeMap It is a red-black tree data structure, and each key-value pair serves as a node of the red-black tree. When TreeMap stores key-value pairs (nodes), the nodes need to be sorted according to the key. TreeMap can ensure that all key-value pairs are in an ordered state. Similarly, TreeMap also has two sorting methods: natural sorting and customized sorting
4) WeakHashMap
The usage of WeakHashMap and HashMap are basically similar. The difference is that the HashMap key retains a "strong reference" to the actual object, which means that as long as the HashMap object is not destroyed, the object referenced by the HashMap will not be garbage collected.
But the key of WeakHashMap only retains a weak reference to the actual object, which means that if the objects referenced by the keys of the WeakHashMap object are not referenced by other strong reference variables, the objects referenced by these keys may be garbage collected. When the actual object corresponding to the key is garbage collected, WeakHashMap may also automatically delete the key-value pairs corresponding to these keys
5) IdentityHashMap
The implementation mechanism of IdentityHashMap is basically similar to HashMap. In IdentityHashMap, IdentityHashMap considers two keys equal if and only if they are strictly equal (key1 == key2)
6) EnumMap
EnumMap is a Map implementation used with enumeration classes. EnumMap All keys in must be enumeration values of a single enumeration class. When creating an EnumMap, you must explicitly or implicitly specify its corresponding enumeration class. EnumMap is based on the natural order of keys
(i.e. the order in which the enumeration values are defined in the enumeration class)
Java Collection Framework Diagram
Introduction to Java Collection Framework Architecture
Java Detailed introduction to the collection framework of core technical points
The above is the detailed content of What is a collection? Basic introduction to Java collections (with framework diagram). For more information, please follow other related articles on the PHP Chinese website!
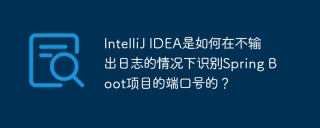
Start Spring using IntelliJIDEAUltimate version...
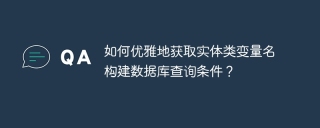
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
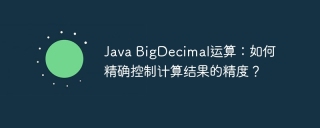
Java...
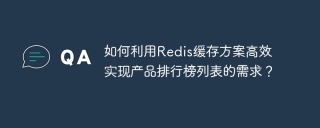
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
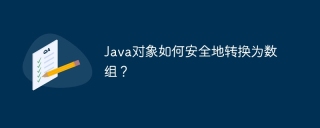
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
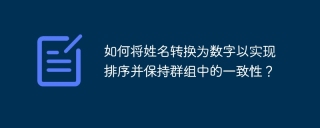
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
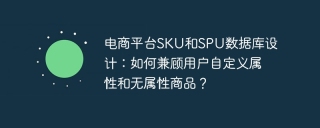
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
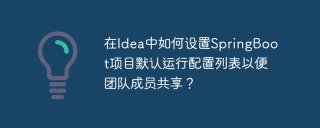
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
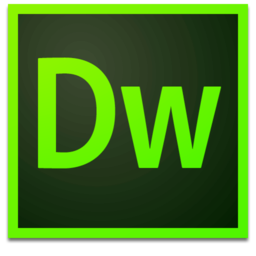
Dreamweaver Mac version
Visual web development tools
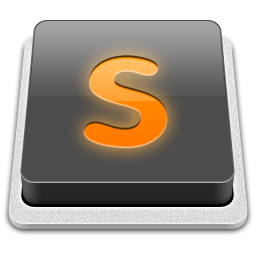
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
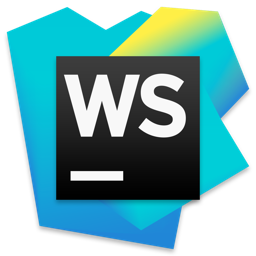
WebStorm Mac version
Useful JavaScript development tools