This article mainly introduces the Vue 2.0 study notes on using $refs to access the DOM in Vue. Now I share it with you and give it as a reference.
Through the previous study of Vue, it is necessary for us to further understand some special properties and methods in Vue instances. The first thing to understand is the $refs
attribute. But before we dive into the JavaScript part, let's take a look at templates.
<p id="app"> <h1 id="nbsp-message-nbsp">{{ message }}</h1> <button @click="clickedButton">点击偶</button> </p> let app = new Vue({ el: '#app', data () { return { message: 'Hi,大漠!' } }, methods: { clickedButton: function () { console.log('Hi,大漠!') } } })
In Vue's template, we can add the ref
attribute to any element in the template, so that these elements can be referenced in the Vue instance. More specifically, DOM elements can be accessed. Try adding the ref
attribute to <button></button>
in the example above. This button has been bound to a click
event. This event allows us to print Hi, Desert! in the browser's control panel.
information.
<button ref="myButton" @click="clickedButton">点击偶</button>
Note that the ref
attribute is not a standard HTML attribute, but an attribute in Vue. In fact, it won't even be part of the DOM, so when you look at the rendered HTML in a browser, you won't see anything about ref
. Because there is no :
added in front of it, and it is not a directive.
Use the $refs
property on the Vue instance to reference this button through myButton
. Let's see what it looks like when printed out in the browser's console.
let app = new Vue({ el: '#app', data () { return { message: 'Hi!大漠' } }, methods: { clickedButton: function () { console.log(this.$refs); } } })
If you open the browser console, we can see that this attribute is a JavaScript object, which contains references to all elements of the ref
attribute.
Note that the key name in this object (key
) is the same as the name we specified in the ref
attribute (name
) matches, and its value (value
) is a DOM element. In this case, we can see that the key name is myButton
, and its value is the button
element. And this has nothing to do with Vue.
So in Vue, you can access the DOM element by accessing the name of the ref
on the $refs
object. Consider the following example. After we click the button, the text of the button will change the value in the message
data.
let app = new Vue({ el: '#app', data () { return { message: 'Hi!大漠' } }, methods: { clickedButton: function () { console.log(this.$refs) this.$refs.myButton.innerText = this.message } } })
After clicking the button, the text of the button will change to "Hi,! Desert
":
Of course, we can also This effect is achieved by using query selectors to access DOM elements, but using the ref
attribute is more concise, and this is also the method in Vue. It will also be more secure since you won't be relying on class
and id
. Therefore, there is almost no impact from changing HTML tags or CSS styles.
One of the main purposes of JavaScript frameworks like Vue is to relieve developers from having to deal with the DOM. So you should avoid doing things like this unless you really need to. There is also a potential problem that should be noted.
First let’s look at a simple example, adding a ref
attribute to the h1
element.
{{ message }}
<button ref="myButton" @click="clickedButton">点击偶</button>
When we click the button, the value output by the browser console will change:
Because we assigned the Vue instance to the variable app
, so we can continue to use it. What we need to do now is change the text of the element. Initially, the content of the <h1></h1>
element is the value of message
. In the following example, look at the element <h1 id="setTimeout-code-code-Changes-that-occurred">setTimeout<code> ;
Changes that occurred:
let app = new Vue({ el: '#app', data () { return { message: 'Hi!大漠' } }, methods: { clickedButton: function () { console.log(this.$refs); this.$refs.myButton.innerText = this.message } } }) setTimeout(function() { app.$refs.message.innerText = '延迟2000ms修改h1元素的文本'; }, 2000);
As you can see, we are overwriting the changes we made to the DOM when updating the data attribute. The reason for this is that when accessing DOM elements and manipulating them directly, you actually skip the virtual DOM discussed in the previous article. Therefore, Vue still controls the h1
element, and even when Vue makes an update to the data, it updates the virtual DOM and then updates the DOM itself. Therefore, you should be careful with direct changes to the DOM, as any changes you make may be overwritten even if you accidentally make changes. Although you should be careful when changing the DOM when using refs
, it is relatively safe to do read-only operations, such as reading values from the DOM.
Also, let’s take a look at the effect of using the refs
attribute in the v-for
directive. For example, in the following example, given an unordered list ul
, the numbers from 1
to 10
are output through the v-for
instruction.
<ul> <li v-for="n in 10" ref="numbers">{{ n }}</li> </ul>
When you click the button, the $refs
attribute will be output in the browser console:
正如上图所看到的一样,把numbers
属性添加到了对象中,但需要注意该值的类型。与之前看到的DOM元素不同,它实际上是一个数组,一个DOM元素的数组。当使用ref
属性和v-for
指令时,Vue会迭代所有DOM元素,并将它们放置在数组中。在这种情况下,这就输出了10
个li
的DOM元素的数组,因为我们迭代了10
次。每个元素都可以像我们之前看到的那样使用。
上面通过简单的示例了解了Vue中的$refs
在Vue中是怎么访问到DOM元素的。接下来看一个简单的示例。
在Web中Modal组件是经常可见的一个组件。来看看$refs
怎么来来控制Modal的打开和关闭。
<!-- HTML --> <p id="app"> <p class="actions"> <button @click="toggleModal('new-item')">添加列表</button> <button @click="toggleModal('confirm')">删除列表</button> </p> <modal ref="new-item"> <p>添加新的列表</p> <p slot="actions"> <button>保存</button> <button>取消</button> </p> </modal> <modal ref="confirm"> <p>删除列表?</p> <p slot="actions"> <button>删除</button> <button>取消</button> </p> </modal> <script type="x-template" id="modal-template"> <transition name="modal-toggle"> <p class="modal" v-show="toggle"> <button class="modal__close" @click="close">X</button> <p class="modal__body"> <h1 id="Modal">Modal</h1> <slot>这是一个Modal,是否需要添加新的内容?</slot> </p> <p class="modal__actions"> <slot name="actions"> <button @click="close">关闭</button> </slot> </p> </p> </transition> </script> </p> // JavaScript let Modal = Vue.component('modal', { template: "#modal-template", data () { return { toggle: false } }, methods: { close: function() { this.toggle = false; } } }); let app = new Vue({ el: "#app", methods: { toggleModal(modal) { this.$refs[modal].toggle = !this.$refs[modal].toggle; } } });
效果如下:
上面是我整理给大家的,希望今后会对大家有帮助。
相关文章:
The above is the detailed content of $refs access DOM in Vue (detailed tutorial). For more information, please follow other related articles on the PHP Chinese website!
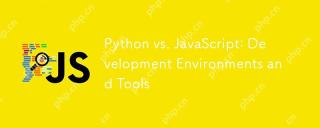
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
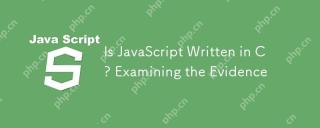
Yes, the engine core of JavaScript is written in C. 1) The C language provides efficient performance and underlying control, which is suitable for the development of JavaScript engine. 2) Taking the V8 engine as an example, its core is written in C, combining the efficiency and object-oriented characteristics of C. 3) The working principle of the JavaScript engine includes parsing, compiling and execution, and the C language plays a key role in these processes.
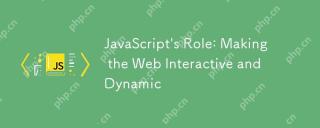
JavaScript is at the heart of modern websites because it enhances the interactivity and dynamicity of web pages. 1) It allows to change content without refreshing the page, 2) manipulate web pages through DOMAPI, 3) support complex interactive effects such as animation and drag-and-drop, 4) optimize performance and best practices to improve user experience.
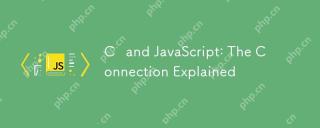
C and JavaScript achieve interoperability through WebAssembly. 1) C code is compiled into WebAssembly module and introduced into JavaScript environment to enhance computing power. 2) In game development, C handles physics engines and graphics rendering, and JavaScript is responsible for game logic and user interface.
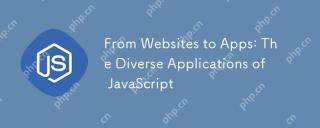
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
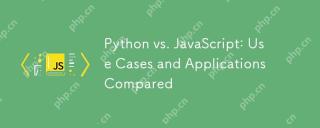
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
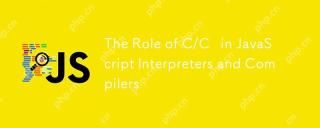
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
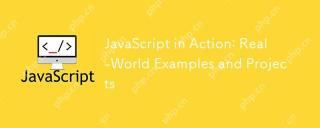
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
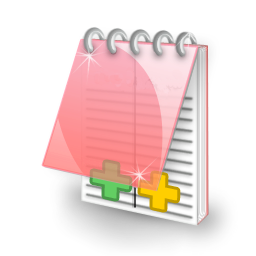
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
