This article mainly introduces the relevant information on how to use Canvas to operate pixels. The content is quite good. I will share it with you now and give it as a reference.
Modern browsers support video playback via the <video></video>
element. Most browsers can also access the camera through the MediaDevices.getUserMedia() API. But even if these two things are combined, we cannot directly access and manipulate these pixels.
Luckily, browsers have a Canvas API that allows us to draw graphics using JavaScript. We can actually draw images to the <canvas></canvas>
from the video itself, which allows us to manipulate and display those pixels.
What you learn here about how to manipulate pixels will provide you with the foundation for working with images and videos of any kind or source, not just canvas.
Add Image to Canvas
Before we start playing the video, let’s see how to add an image to canvas.
<img src alt="How to manipulate pixels using Canvas" > <p> <canvas id="Canvas" class="video"></canvas> </p>
We create an image element to represent the image to be drawn on the canvas. Alternatively, we can use the Image object in JavaScript.
var canvas; var context; function init() { var image = document.getElementById('SourceImage'); canvas = document.getElementById('Canvas'); context = canvas.getContext('2d'); drawImage(image); // Or // var image = new Image(); // image.onload = function () { // drawImage(image); // } // image.src = 'image.jpg'; } function drawImage(image) { // Set the canvas the same width and height of the image canvas.width = image.width; canvas.height = image.height; context.drawImage(image, 0, 0); } window.addEventListener('load', init);
The above code draws the entire image to the canvas.
View Paint image on canvas via Welling Guzman (@wellingguzman) on CodePen.
Now we can start playing with those pixels!
Update image data
Image data on the canvas allows us to manipulate and change pixels.
The data property is an ImageData object, which has three properties - width, height and data/ All of these represent something based on the original image. All of these properties are read-only. What we care about is the data, a one-dimensional array represented by a Uint8ClampedArray object, containing data for each pixel in RGBA format.
Although the data property is read-only, it does not mean that we cannot change its value. It means that we cannot assign another array to this property.
// Get the canvas image data var imageData = context.getImageData(0, 0, canvas.width, canvas.height); image.data = new Uint8ClampedArray(); // WRONG image.data[1] = 0; // CORRECT
You may ask, what value does the Uint8ClampedArray object represent. The following is a description from MDN:
The Uint8ClampedArray type array represents an array of 8-bit unsigned integers, which is clamped to 0-255; if If you specify a value outside the range [0,255], 0 or 255 will be set; if you specify a non-integer, the nearest integer will be set. The contents are initialized to 0. Once established, you can use the object's methods to reference the element, or use standard array indexing syntax (i.e. using bracket notation)
In short, this array stores a value ranging from 0 to 255 at each position, which makes it a perfect solution for the RGBA format scheme because each part is represented by a value from 0 to 255.
RGBA Color
Color can be represented in RGBA format which is red, green and blue A combination of. A represents the alpha value of the color opacity.
Each position in the array represents a color (pixel) channel value.
The first position is Red value
The second position is the green value
The third position is the blue value
-
The fourth position is the Alpha value
The fifth position is the next pixel red value
The sixth position is the next The green value of a pixel
The 7th position is the blue value of the next pixel
The 8th position is the next pixel Alpha Values
and so on...
If you have a 2x2 image, then we have a 16 bit array (2x2 pixels x 4 each value).
2x2 image scaled down
The array will look like this:
// RED GREEN BLUE WHITE [ 255, 0, 0, 255, 0, 255, 0, 255, 0, 0, 255, 255, 255, 255, 255, 255]
Change pixels Data
One of the quickest things we can do is set all pixels to white by changing all RGBA values to 255.
// Use a button to trigger the "effect" var button = document.getElementById('Button'); button.addEventListener('click', onClick); function changeToWhite(data) { for (var i = 0; i < data.length; i++) { data[i] = 255; } } function onClick() { var imageData = context.getImageData(0, 0, canvas.width, canvas.height); changeToWhite(imageData.data); // Update the canvas with the new data context.putImageData(imageData, 0, 0); }
The data will be passed as a reference, which means any modification we make to it, it will change the value of the passed parameter.
Invert Colors
A great effect that doesn’t require too much calculation is to invert the colors of an image.
Color values can be inverted using the XOR operator (^) or this formula 255 - value (value must be between 0-255).
function invertColors(data) { for (var i = 0; i < data.length; i+= 4) { data[i] = data[i] ^ 255; // Invert Red data[i+1] = data[i+1] ^ 255; // Invert Green data[i+2] = data[i+2] ^ 255; // Invert Blue } } function onClick() { var imageData = context.getImageData(0, 0, canvas.width, canvas.height); invertColors(imageData.data); // Update the canvas with the new data context.putImageData(imageData, 0, 0); }
We are incrementing the loop by 4 like before instead of 1, so we can go from pixel to pixel, filling the 4 elements in the array with each pixel .
The alpha value has no effect on inverting the color, so we skip it.
Brightness and Contrast
Use the following formula to adjust the brightness of an image: newValue = currentValue 255 * (brightness / 100).
Brightness must be between -100 and 100
currentValue is the current lighting value for red, green or blue.
newValue is the result of adding brightness to the current color light
Adjusting the contrast of the image can be done using this formula:
factor = (259 * (contrast + 255)) / (255 * (259 - contrast)) color = GetPixelColor(x, y) newRed = Truncate(factor * (Red(color) - 128) + 128) newGreen = Truncate(factor * (Green(color) - 128) + 128) newBlue = Truncate(factor * (Blue(color) - 128) + 128)
The main calculation is to get the contrast factor that will be applied to each color value. Truncation is a function that ensures the value remains between 0 and 255.
Let’s write these functions into JavaScript:
function applyBrightness(data, brightness) { for (var i = 0; i < data.length; i+= 4) { data[i] += 255 * (brightness / 100); data[i+1] += 255 * (brightness / 100); data[i+2] += 255 * (brightness / 100); } } function truncateColor(value) { if (value < 0) { value = 0; } else if (value > 255) { value = 255; } return value; } function applyContrast(data, contrast) { var factor = (259.0 * (contrast + 255.0)) / (255.0 * (259.0 - contrast)); for (var i = 0; i < data.length; i+= 4) { data[i] = truncateColor(factor * (data[i] - 128.0) + 128.0); data[i+1] = truncateColor(factor * (data[i+1] - 128.0) + 128.0); data[i+2] = truncateColor(factor * (data[i+2] - 128.0) + 128.0); } }
在这种情况下,您不需要truncateColor函数,因为Uint8ClampedArray会截断这些值,但为了翻译我们在其中添加的算法。
需要记住的一点是,如果应用亮度或对比度,则图像数据被覆盖后无法回到之前的状态。如果我们想要重置为原始状态,则原始图像数据必须单独存储以供参考。保持图像变量对其他函数可访问将会很有帮助,因为您可以使用该图像来重绘画布和原始图像。
var image = document.getElementById('SourceImage'); function redrawImage() { context.drawImage(image, 0, 0); }
使用视频
为了使它适用于视频,我们将采用我们的初始图像脚本和HTML代码并做一些小的修改。
HTML
通过替换以下行来更改视频元素的Image元素:
<img src alt="How to manipulate pixels using Canvas" >
...with this:
<video src></video>
JavaScript
替换这一行:
var image = document.getElementById('SourceImage');
...添加这行:
var video = document.getElementById('SourceVideo');
要开始处理视频,我们必须等到视频可以播放。
video.addEventListener('canplay', function () { // Set the canvas the same width and height of the video canvas.width = video.videoWidth; canvas.height = video.videoHeight; // Play the video video.play(); // start drawing the frames drawFrame(video); });
当有足够的数据可以播放媒体时,至少在几帧内播放事件播放。
我们无法看到画布上显示的任何视频,因为我们只显示第一帧。我们必须每n毫秒执行一次drawFrame以跟上视频帧速率。
在drawFrame内部,我们每10ms再次调用drawFrame。
function drawFrame(video) { context.drawImage(video, 0, 0); setTimeout(function () { drawFrame(video); }, 10); }
在执行drawFrame之后,我们创建一个循环,每10ms执行一次drawFrame - 足够的时间让视频在画布中保持同步。
将效果添加到视频
我们可以使用我们之前创建的相同函数来反转颜色:
function invertColors(data) { for (var i = 0; i < data.length; i+= 4) { data[i] = data[i] ^ 255; // Invert Red data[i+1] = data[i+1] ^ 255; // Invert Green data[i+2] = data[i+2] ^ 255; // Invert Blue } }
并将其添加到drawFrame函数中:
function drawFrame(video) { context.drawImage(video, 0, 0); var imageData = context.getImageData(0, 0, canvas.width, canvas.height); invertColors(imageData.data); context.putImageData(imageData, 0, 0); setTimeout(function () { drawFrame(video); }, 10); }
我们可以添加一个按钮并切换效果:
function drawFrame(video) { context.drawImage(video, 0, 0); if (applyEffect) { var imageData = context.getImageData(0, 0, canvas.width, canvas.height); invertColors(imageData.data); context.putImageData(imageData, 0, 0); } setTimeout(function () { drawFrame(video); }, 10); }
使用 camera
我们将保留我们用于视频的相同代码,唯一不同的是我们将使用MediaDevices.getUserMedia将视频流从文件更改为相机流。
MediaDevices.getUserMedia是弃用先前API MediaDevices.getUserMedia()的新API。浏览器仍旧支持旧版本,并且某些浏览器不支持新版本,我们必须求助于polyfill以确保浏览器支持其中一种。
首先,从视频元素中删除src属性:
<video><code>
<code>// Set the source of the video to the camera stream function initCamera(stream) { video.src = window.URL.createObjectURL(stream); } if (navigator.mediaDevices.getUserMedia) { navigator.mediaDevices.getUserMedia({video: true, audio: false}) .then(initCamera) .catch(console.error) ); }</code>
Live Demo
效果
到目前为止,我们所介绍的所有内容都是我们需要的基础,以便为视频或图像创建不同的效果。我们可以通过独立转换每种颜色来使用很多不同的效果。
灰阶
将颜色转换为灰度可以使用不同的公式/技巧以不同的方式完成,以避免陷入太深的问题我将向您展示基于GIMP desaturate tool去饱和工具和Luma的五个公式:
Gray = 0.21R + 0.72G + 0.07B // Luminosity Gray = (R + G + B) ÷ 3 // Average Brightness Gray = 0.299R + 0.587G + 0.114B // rec601 standard Gray = 0.2126R + 0.7152G + 0.0722B // ITU-R BT.709 standard Gray = 0.2627R + 0.6780G + 0.0593B // ITU-R BT.2100 standard
我们想要使用这些公式找到的是每个像素颜色的亮度等级。该值的范围从0(黑色)到255(白色)。这些值将创建灰度(黑白)效果。
这意味着最亮的颜色将最接近255,最暗的颜色最接近0。
Live Demo
双色调
双色调效果和灰度效果的区别在于使用了两种颜色。在灰度上,您有一个从黑色到白色的渐变色,而在双色调中,您可以从任何颜色到任何其他颜色(从蓝色到粉红色)都有一个渐变。
使用灰度的强度值,我们可以将其替换为梯度值。
我们需要创建一个从ColorA到ColorB的渐变。
function createGradient(colorA, colorB) { // Values of the gradient from colorA to colorB var gradient = []; // the maximum color value is 255 var maxValue = 255; // Convert the hex color values to RGB object var from = getRGBColor(colorA); var to = getRGBColor(colorB); // Creates 256 colors from Color A to Color B for (var i = 0; i <= maxValue; i++) { // IntensityB will go from 0 to 255 // IntensityA will go from 255 to 0 // IntensityA will decrease intensity while instensityB will increase // What this means is that ColorA will start solid and slowly transform into ColorB // If you look at it in other way the transparency of color A will increase and the transparency of color B will decrease var intensityB = i; var intensityA = maxValue - intensityB; // The formula below combines the two color based on their intensity // (IntensityA * ColorA + IntensityB * ColorB) / maxValue gradient[i] = { r: (intensityA*from.r + intensityB*to.r) / maxValue, g: (intensityA*from.g + intensityB*to.g) / maxValue, b: (intensityA*from.b + intensityB*to.b) / maxValue }; } return gradient; } // Helper function to convert 6digit hex values to a RGB color object function getRGBColor(hex) { var colorValue; if (hex[0] === '#') { hex = hex.substr(1); } colorValue = parseInt(hex, 16); return { r: colorValue >> 16, g: (colorValue >> 8) & 255, b: colorValue & 255 } }
简而言之,我们从颜色A创建一组颜色值,降低强度,同时转到颜色B并增加强度。
从 #0096ff 到 #ff00f0
var gradients = [ {r: 32, g: 144, b: 254}, {r: 41, g: 125, b: 253}, {r: 65, g: 112, b: 251}, {r: 91, g: 96, b: 250}, {r: 118, g: 81, b: 248}, {r: 145, g: 65, b: 246}, {r: 172, g: 49, b: 245}, {r: 197, g: 34, b: 244}, {r: 220, g: 21, b: 242}, {r: 241, g: 22, b: 242}, ];
缩放颜色过渡的表示
上面有一个从#0096ff到#ff00f0的10个颜色值的渐变示例。
颜色过渡的灰度表示
现在我们已经有了图像的灰度表示,我们可以使用它将其映射到双色调渐变值。
The duotone gradient has 256 colors while the grayscale has also 256 colors ranging from black (0) to white (255). That means a grayscale color value will map to a gradient element index.
var gradientColors = createGradient('#0096ff', '#ff00f0'); var imageData = context.getImageData(0, 0, canvas.width, canvas.height); applyGradient(imageData.data); for (var i = 0; i < data.length; i += 4) { // Get the each channel color value var redValue = data[i]; var greenValue = data[i+1]; var blueValue = data[i+2]; // Mapping the color values to the gradient index // Replacing the grayscale color value with a color for the duotone gradient data[i] = gradientColors[redValue].r; data[i+1] = gradientColors[greenValue].g; data[i+2] = gradientColors[blueValue].b; data[i+3] = 255; }
Live Demo
结论
这个主题可以更深入或解释更多的影响。为你做的功课是找到可以应用于这些骨架示例的不同算法。
了解像素在画布上的结构将允许您创建无限数量的效果,如棕褐色,混色,绿色屏幕效果,图像闪烁/毛刺等。
您甚至可以在不使用图像或视频的情况下即时创建效果
The above is the entire content of this article. I hope it will be helpful to everyone's study. For more related content, please pay attention to the PHP Chinese website!
Related recommendations:
About the properties of canvas lines
##How to use canvas to achieve image mosaic
The above is the detailed content of How to manipulate pixels using Canvas. For more information, please follow other related articles on the PHP Chinese website!
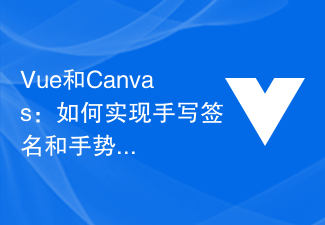
Vue和Canvas:如何实现手写签名和手势识别功能引言:手写签名和手势识别功能在现代应用程序中越来越常见,它们可以为用户提供更加直观和自然的交互方式。Vue.js作为一款流行的前端框架,搭配Canvas元素可以实现这两个功能。本文将介绍如何使用Vue.js和Canvas元素来实现手写签名和手势识别功能,并给出相应的代码示例。一、手写签名功能实现要实现手写签
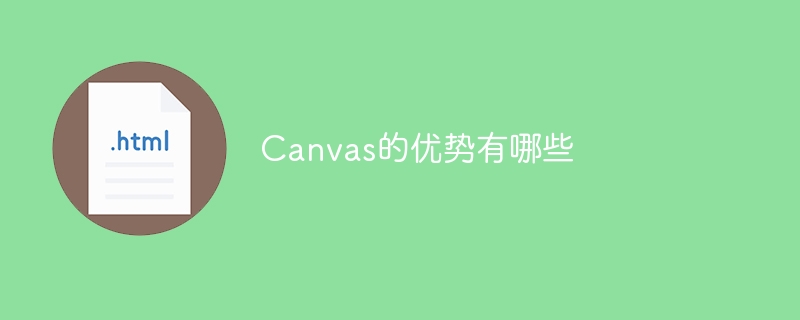
canvas的优势有强大的绘图功能、高性能、跨平台兼容性、支持多种图形格式、可以与其他Web技术集成、可以实现动态效果和可以实现复杂的图像处理。详细介绍:1、Canvas提供了丰富的绘图功能,可以绘制各种形状、线条、文本、图像等;2、Canvas在浏览器中直接操作像素,因此具有很高的性能;3、Canvas是基于HTML5标准的一部分,可以在各种现代浏览器上运行等等。
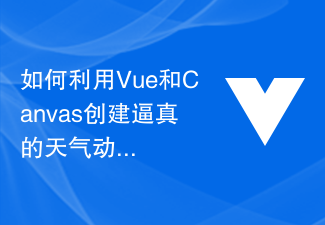
如何利用Vue和Canvas创建逼真的天气动态背景引言:在现代网页设计中,动态背景效果是吸引用户眼球的重要元素之一。本文将介绍如何利用Vue和Canvas技术来创建一个逼真的天气动态背景效果。通过代码示例,你将学习如何编写Vue组件和利用Canvas绘制不同天气场景,从而实现一个独特而吸引人的背景效果。步骤一:创建Vue项目首先,我们需要创建一个Vue项目。
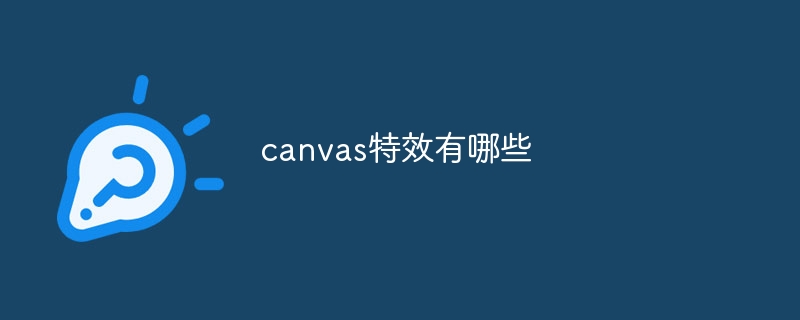
canvas特效有粒子效果、线条动画、图片处理、文字动画、音频可视化、3D效果、游戏开发等。详细介绍:1、粒子效果,通过控制粒子的位置、速度和颜色等属性来实现各种效果,如烟花、雨滴、星空等;2、线条动画,通过在画布上绘制连续的线条,创建出各种动态的线条效果;3、图片处理,通过对图片进行处理,可以实现各种炫酷的效果,如图片切换、图片特效等;4、文字动画等等特性。
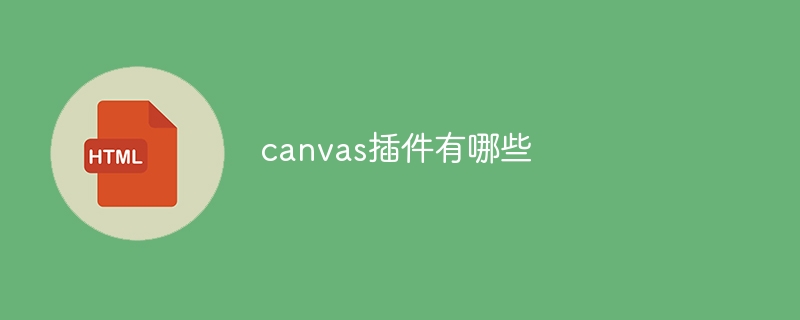
canvas插件有Fabric.js、EaselJS、Konva.js、Three.js、Paper.js、Chart.js和Phaser。详细介绍:1、Fabric.js 是一个基于Canvas的开源 JavaScript 库,它提供了一些强大的功能;2、EaselJS是CreateJS库中的一个模块,它提供了一套简化了Canvas编程的API;3、Konva.js等等。
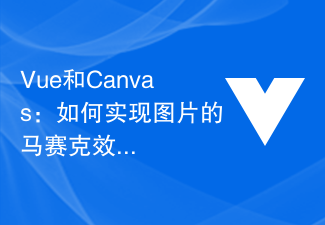
Vue和Canvas:如何实现图片的马赛克效果引言:随着Web技术的不断发展,越来越多的人开始使用Vue框架来构建交互式的前端应用。而在前端开发中,常常需要为用户提供图片处理的功能。本文将介绍如何利用Vue和Canvas实现图片的马赛克效果,为用户带来更好的视觉体验。一、马赛克效果概述马赛克效果是一种将图像的细节部分进行像素化处理,使得整个图像变得模糊和抽象
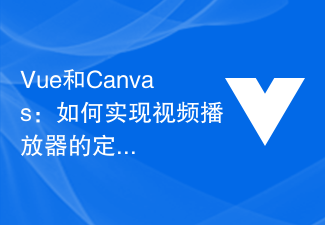
Vue和Canvas:如何实现视频播放器的定制化界面引言:在现代互联网时代,视频已经成为人们生活中必不可少的一部分。为了提供良好的用户体验,许多网站和应用程序都提供了自定义的视频播放器界面。本文将介绍如何使用Vue和Canvas技术实现一个定制化的视频播放器界面。一、前期准备在开始之前,您需要确保您已经安装了Vue和Canvas,并且熟悉这两种技术的基本用法
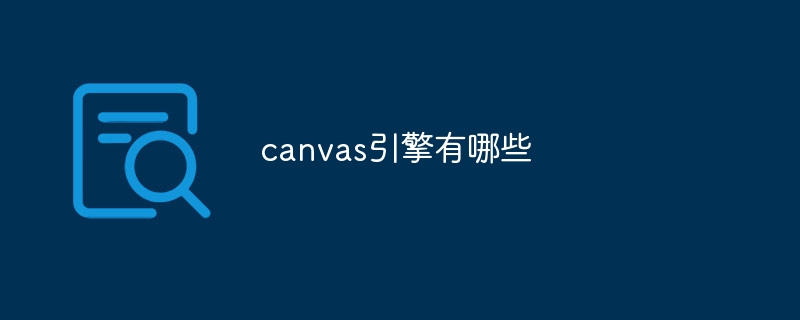
canvas引擎有Three.js、Pixi.js、EaselJS、Konva.js、Paper.js等。详细介绍:1、Pixi.js,提供了简单易用的API,支持精灵、纹理、滤镜等功能,同时还提供了丰富的工具和插件,方便开发者进行交互、动画和优化等操作;2、Pixi.js,提供了简单易用的API,支持精灵、纹理、滤镜等功能,还提供了丰富的工具和插件;3、EaselJS等等。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
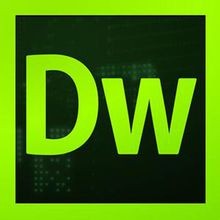
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
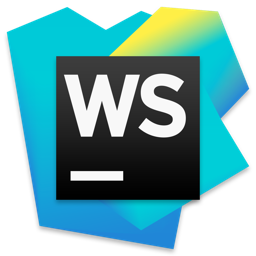
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
