


This article mainly introduces the example of AngularJS using ui-route to implement multi-layer nested routing. Now I share it with you and give it as a reference.
This article introduces an example of AngularJS using ui-route to implement multi-layer nested routing, and shares it with everyone. The details are as follows:
1. Expected implementation effect:
https://liyuan-meng.github.io/uiRouter-app/index.html
(Project address: https://github.com/liyuan-meng/uiRouter-app)
2. Analyze the question requirements, give dependencies, and build the project
1. service:
(1) Query people data checkPeople according to conditions. service, if no conditions are given, all will be queried.
(2) Get routing information getStateParams.service.
2. components:
(1) hello module: click the button button to change the content.
(2) peopleList module: displays the people list, click people to display people details. Depends on checkPeople.service module.
(3) peopleDetail module: displays people details, relying on the checkPeople.service module and getStateParams.service module.
3. Build the project:
As shown in the figure: the component directory is used to save all service modules and business modules, and the lib directory saves external references (I Angular.js1.5.8 and ui-route0.2.18 are used), the app.config.js file is used to configure routing, and index.html is used as the entry file.
3. Implement this example
1. Home page index.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <script src="./lib/angular.js"></script> <script src="./lib/angular-ui-route.js"></script> <script src="./app.config.js"></script> <script src="./components/core/people/checkPeople.service.js"></script> <script src="./components/core/people/getStateParams.service.js"></script> <script src="./components/hello/hello.component.js"></script> <script src="./components/people-list/people-list.component.js"></script> <script src="./components/people-detail/people-detail.component.js"></script> </head> <body ng-app="helloSolarSystem"> <p> <a ui-sref="helloState">Hello</a> <a ui-sref="aboutState">About</a> <a ui-sref="peopleState">People</a> </p> <ui-view></ui-view> </body> </html>
(1) Import the files in lib and all used services and component service files.
(2) ng-app="helloSolarSystem" specifies that parsing starts from the helloSolarSystem module.
(3) Define view
2. Configure routing app.config.js
'use strict'; angular.module("helloSolarSystem", ['peopleList', 'peopleDetail', 'hello','ui.router']). config(['$stateProvider', function ($stateProvider) { $stateProvider.state('helloState', { url: '/helloState', template:'<hello></hello>' }).state('aboutState', { url: '/about', template: '<h4 id="Its-nbsp-the-nbsp-UI-Router-nbsp-Hello-nbsp-Solar-nbsp-System-nbsp-app">Its the UI-Router Hello Solar System app!</h4>' }).state('peopleState', { url: '/peopleList', template:'<people-list></people-list>' }).state('peopleState.details', { url:'/detail/:id', template: '<people-detail></people-detail>' }) } ]);
(1) Module Name: helloSolarSystem;
(2) Inject 'peopleList', 'peopleDetail', 'hello', 'ui.router' modules.
(3) Configure the view control of the stateProvider service, for example, the first view controller named helloState: when ui-sref == "helloState", the route is updated to the value of url #/helloState, And the content displayed in
(4) Implementation of nested routing: The view controller named peopleState is the parent routing. The view controller named peopleState.details is a child route. This is a relative routing method. The parent route will match.../index.html#/peopleState/, and the child route will match.../index.html#/peopleState/detail/x (x is /detail/:id the value of id in ). If you change it to absolute routing, you only need to write url:'^/detail/:id', then the sub-route will match.../index.html#/detail/x (x is in /detail/:id) id value).
4. Implement checkPeople.service (find people based on conditions)
checkPeople.sercice.js
'use strict'; //根据条件(参数)查找信息。 angular.module('people.checkPeople', ['ui.router']). factory('CheckPeople', ['$http', function ($http) { return { getData: getData }; function getData(filed) { var people; var promise = $http({ method: 'GET', url: './data/people.json' }).then(function (response) { if (filed) { people = response.data.filter(function (value) { if (Number(value.id) === Number(filed)) { return value; } }) } else { people = response.data; } return people; }); return promise; } }]);
(1) In the getData function, we want to return a An array that saves people information. However, when using the $http().then() service, this is an asynchronous request. We don’t know when the request will end, so there is a problem with the world returning the people array. We noticed that $http().then() is a Promise object, so we can think of returning this object directly, so that we can use "result of function.then(function(data))" to get the asynchronous request The coming data data.
3. Implement getStateParams.service (get routing information)
getStatePatams.service.js
"use strict"; angular.module("getStateParams", ['ui.router']). factory("GetStateParams", ["$location", function ($location) { return { getParams: getParams }; function getParams() { var partUrlArr = $location.url().split("/"); return partUrlArr[partUrlArr.length-1]; } }]);
(1) The getParams function here returns the last one of the routing information Data, that is, people's IDs. This service is somewhat special and not universal enough. It may need to be optimized to make it more reasonable. But it does not affect our needs.
4. Implement the hello module
hello.template.html
<p> <p ng-hide="hideFirstContent">hello solar sytem!</p> <p ng-hide="!hideFirstContent">whats up solar sytem!</p> <button ng-click="ctlButton()">click</button> </p>
hello.component.js
'use strict'; angular.module("hello", []) .component('hello', { templateUrl: './components/hello/hello.template.html', controller: ["$scope", function HelloController($scope) { $scope.hideFirstContent = false; $scope.ctlButton = function () { this.hideFirstContent = !this.hideFirstContent; }; } ] });
5. Implement the peopleList module:
peopleList.template.html
<p> <ul> <a ng-repeat="item in people" ui-sref="peopleState.details({id:item.id})"> <li>{{item.name}}</li> </a> </ul> <ui-view></ui-view> </p>
(1) The
peopleList.component of peopleList .js
'use strict'; angular.module("peopleList", ['people.checkPeople']) .component('peopleList', { templateUrl: './components/people-list/people-list.template.html', controller: ['CheckPeople','$scope', function PeopleListController(CheckPeople, $scope) { $scope.people = []; CheckPeople.getData().then(function(data){ $scope.people = data; }); } ] });
6. Implement the peopleDetail module
peopleDetail.template.html
<ul ng-repeat="item in peopleDetails track by $index"> <li>名字: {{item.name}}</li> <li>介绍: {{item.intro}}</li> </ul>
peopleDetail.component.js
'use strict'; angular.module("peopleDetail", ['people.checkPeople', 'getStateParams']) .component('peopleDetail', { templateUrl: './components/people-detail/people-detail.template.html', controller: ['CheckPeople', 'GetStateParams', '$scope', function peopleDetailController(CheckPeople, GetStateParams, $scope) { $scope.peopleDetails = []; CheckPeople.getData(GetStateParams.getParams()).then(function(data){ $scope.peopleDetails = data; }); } ] });
The above is what I compiled for everyone Yes, I hope it will be helpful to everyone in the future.
Related articles:
How to implement the corresponding callback function after loading by using JS script
How to solve the packaging file 404 using vue webpack Blank page problem
How to implement it through the vue-cli webpack project Modify the project name
How to implement global registration and local registration in the vue component
The above is the detailed content of Using ui-route to implement multi-layer nested routing in AngularJS (detailed tutorial). For more information, please follow other related articles on the PHP Chinese website!
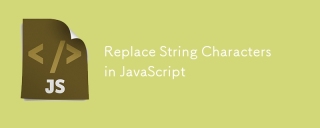
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
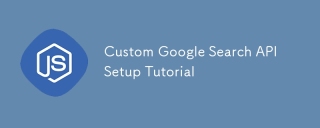
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
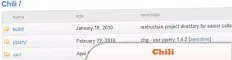
Enhance Your Code Presentation: 10 Syntax Highlighters for Developers Sharing code snippets on your website or blog is a common practice for developers. Choosing the right syntax highlighter can significantly improve readability and visual appeal. T
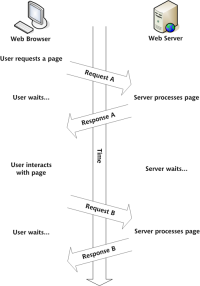
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
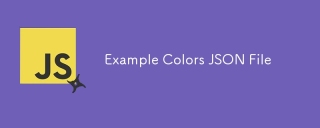
This article series was rewritten in mid 2017 with up-to-date information and fresh examples. In this JSON example, we will look at how we can store simple values in a file using JSON format. Using the key-value pair notation, we can store any kind
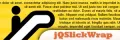
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
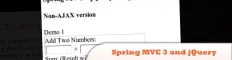
This article presents a curated selection of over 10 tutorials on JavaScript and jQuery Model-View-Controller (MVC) frameworks, perfect for boosting your web development skills in the new year. These tutorials cover a range of topics, from foundatio
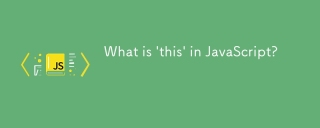
Core points This in JavaScript usually refers to an object that "owns" the method, but it depends on how the function is called. When there is no current object, this refers to the global object. In a web browser, it is represented by window. When calling a function, this maintains the global object; but when calling an object constructor or any of its methods, this refers to an instance of the object. You can change the context of this using methods such as call(), apply(), and bind(). These methods call the function using the given this value and parameters. JavaScript is an excellent programming language. A few years ago, this sentence was


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
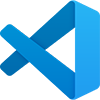
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
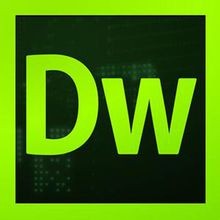
Dreamweaver CS6
Visual web development tools
