


This article introduces the solution for php json_encode that does not support the private properties of objects. json_encode can convert the object into json format, and use json_decode to restore it to the object.
But if the object contains private attributes, the private attributes will be lost after json_encode is executed.
Example: json_encode loses object private attributes
<?php// 用户类class user{ public $id = 1; public $name = 'fdipzone'; public $profession = 'programmer'; private $age = 18; }// 对象$oUser = new User;// json_encode$json = json_encode($oUser);echo $json;// json_decode$oUser = json_decode($json); var_dump($oUser);?>
Output:
{"id":1,"name":"fdipzone","profession":"programmer"}object(stdClass)[2] public 'id' => int 1 public 'name' => string 'fdipzone' (length=8) public 'profession' => string 'programmer' (length=10)
After executing json_encode, the private attribute age is lost.
Solution to the loss of private attributes of the object after json_encode
The modified code is as follows:
<?php// 用户类class user implements JsonSerializable{ public $id = 1; public $name = 'fdipzone'; public $profession = 'programmer'; private $age = 18; // 实现的抽象类方法,指定需要被序列化JSON的数据 public function jsonSerialize() { $data = []; foreach ($this as $key=>$val){ if ($val !== null) $data[$key] = $val; } return $data; } }// 对象$oUser = new User;// json_encode$json = json_encode($oUser);echo $json;// json_decode$oUser = json_decode($json); var_dump($oUser);?>
Output:
{"id":1,"name":"fdipzone","profession":"programmer","age":18}object(stdClass)[2] public 'id' => int 1 public 'name' => string 'fdipzone' (length=8) public 'profession' => string 'programmer' (length=10) public 'age' => int 18
After specifying the data that needs to be serialized into JSON, json_encode can read the private attribute age.
This article explains the relevant knowledge that php json_encode does not support object private attributes. For more related content, please pay attention to the php Chinese website.
Related recommendations:
Explanation on PHP generating unique RequestID class related content
MySQL View database table capacity size
Detailed explanation of a singleton mode Mysql operation class encapsulated by PHP
The above is the detailed content of Explanation on php json_encode not supporting object private attributes. For more information, please follow other related articles on the PHP Chinese website!
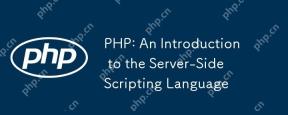
PHP is a server-side scripting language used for dynamic web development and server-side applications. 1.PHP is an interpreted language that does not require compilation and is suitable for rapid development. 2. PHP code is embedded in HTML, making it easy to develop web pages. 3. PHP processes server-side logic, generates HTML output, and supports user interaction and data processing. 4. PHP can interact with the database, process form submission, and execute server-side tasks.
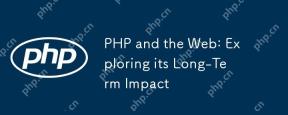
PHP has shaped the network over the past few decades and will continue to play an important role in web development. 1) PHP originated in 1994 and has become the first choice for developers due to its ease of use and seamless integration with MySQL. 2) Its core functions include generating dynamic content and integrating with the database, allowing the website to be updated in real time and displayed in personalized manner. 3) The wide application and ecosystem of PHP have driven its long-term impact, but it also faces version updates and security challenges. 4) Performance improvements in recent years, such as the release of PHP7, enable it to compete with modern languages. 5) In the future, PHP needs to deal with new challenges such as containerization and microservices, but its flexibility and active community make it adaptable.
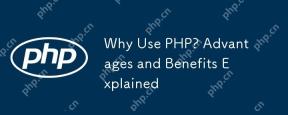
The core benefits of PHP include ease of learning, strong web development support, rich libraries and frameworks, high performance and scalability, cross-platform compatibility, and cost-effectiveness. 1) Easy to learn and use, suitable for beginners; 2) Good integration with web servers and supports multiple databases; 3) Have powerful frameworks such as Laravel; 4) High performance can be achieved through optimization; 5) Support multiple operating systems; 6) Open source to reduce development costs.
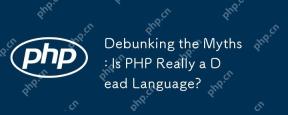
PHP is not dead. 1) The PHP community actively solves performance and security issues, and PHP7.x improves performance. 2) PHP is suitable for modern web development and is widely used in large websites. 3) PHP is easy to learn and the server performs well, but the type system is not as strict as static languages. 4) PHP is still important in the fields of content management and e-commerce, and the ecosystem continues to evolve. 5) Optimize performance through OPcache and APC, and use OOP and design patterns to improve code quality.
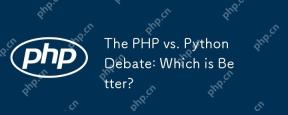
PHP and Python have their own advantages and disadvantages, and the choice depends on the project requirements. 1) PHP is suitable for web development, easy to learn, rich community resources, but the syntax is not modern enough, and performance and security need to be paid attention to. 2) Python is suitable for data science and machine learning, with concise syntax and easy to learn, but there are bottlenecks in execution speed and memory management.
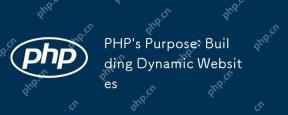
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.

PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
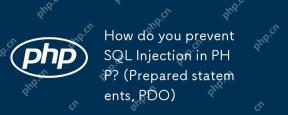
Using preprocessing statements and PDO in PHP can effectively prevent SQL injection attacks. 1) Use PDO to connect to the database and set the error mode. 2) Create preprocessing statements through the prepare method and pass data using placeholders and execute methods. 3) Process query results and ensure the security and performance of the code.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
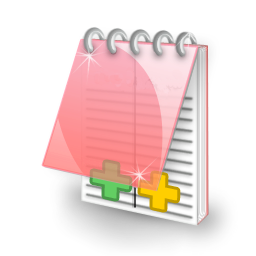
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
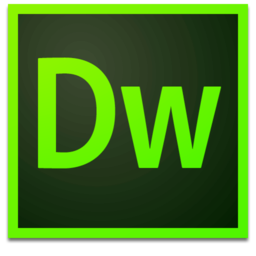
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor