How to use readline module and util module in Node.js
This article mainly introduces the use of Node.js readline module and util module. Now I share it with you and give you a reference.
1. Use the readline module to read stream data line by line
1.1. Create an Interface object
In the readline module, through the use of the Interface object To realize the processing of reading stream data line by line. Therefore, you must first create an Interface object. In the readline module, you can create an Interface object through the createInterface method. readline.createInterface(options), options is an object, and the attributes are as follows
input: Attributes The value is an object that can be used to read stream data, used to specify the source of the read data.
output: The attribute value is an object that can be used to write stream data and is used to specify the output destination of the data.
computer: The attribute value is a function used to specify Tab completion processing. The parameter value of the function is automatically set to the data before the Tab character read from the line. The function should return an array consisting of all matching strings used for Tab completion and the data read from the line. The data before the Tab character.
terminal: This attribute is a Boolean type attribute. When it is necessary to output the input data stream in real time like a terminal, and ANSI/VT100 control needs to be written in the output data When it is a string, the attribute value needs to be set to true. The default attribute value is equal to the isTTY attribute value of the output attribute value object.
// 输入 exit, quit,q这三个任意之一的时候,会退出 const readline = require('readline'); let rl = readline.createInterface({ input: process.stdin, output: process.stdout, completer: completer }); rl.on('line', (line) => { if (line === 'exit' || line === 'quit' || line === 'q') { rl.close(); } else { console.log('您输入了:', line); } }); rl.on('close', () => { console.log('行数据读取操作被终止'); }); function completer(line) { const completions = '.help .error .exit .quit .q'.split(' '); let hits = completions.filter((c) => { return c.indexOf(line) === 0; }); return [hits.length ? hits : completions, line] }
1.2. Use the Interface object to read the file line by line
The content of the original fs.js file
console.log('this is line 1'); console.log('this is line 2'); console.log('this is line 3'); console.log('this is line 4'); console.log('this is line 5');
Code content
const readline = require('readline'); const fs = require('fs'); let file = fs.createReadStream('./fs.js'); let out = fs.createWriteStream('./anotherFs.js'); let index = 1; out.write('/*line' + index.toString() + ": */"); let rl = readline.createInterface({ input: file, output: out, terminal: true }); rl.on('line', (line) => { if (line === '') { rl.close(); } else { index++; out.write('/*line' + index.toString() + ': */'); } });
Contents of the generated anotherFs.js file
/*line1: */console.log('this is line 1'); /*line2: */console.log('this is line 2'); /*line3: */console.log('this is line 3'); /*line4: */console.log('this is line 4'); /*line5: */console.log('this is line 5');/*line6: */
2. Use some methods provided in the util module
format method
is similar to the printf method in C language, Use the first parameter value as a format string, use the other parameter values as each parameter used in the format string, and return a formatted string.util.format('You entered %d parameters, the parameter values are %s, %s, %s', 3, 'nice', 'excelent', 'holy');
Parameter specification symbols that can be used in the format string
*`%s`: used to specify string parameters
*`%d`: used to specify numerical parameters, including integers and Floating point number
*`%j`: used to specify a `JSON` object
*`%%`: used to specify a Percent sign
*If the number of parameters used in the format string is more than the other parameters used in the format method except the `format` parameter, the format string Parameters that are more than will not be replaced.`console.log(util.format('%s:%s','one'));`
*If formatted If the number of parameters used in the string is less than the other parameters used in the `format` method except the `format` parameter, it will be automatically converted into a string according to the type of the parameter value in the `format` method. Use a space to separate.
inspect(object,[options]) returns a string that contains information about the object, which is very useful in debugging applications.
*`showHidden
`If `true`, the non-enumerable symbols and properties of `object` will also be included in the formatted result. Default Specifies the number of times to recurse when formatting `object` for `false.` *`depth
`. This is useful for viewing large complex objects. Defaults to `2`. To recurse infinitely, pass in `null`. *`colors
`If `true`, the output style uses `ANSI` color code. The default is` false`. The color can be customized. *`customInspect
`If it is `false`, then the custom `inspect(depth,opts)` function on `object` Will not be called. Defaults to `true`. *`showProxy
`If `true`, the objects and functions of the `Proxy` object will display their` target` and `handler` objects. The default is `false`. *`maxArrayLength
` specifies the maximum number of elements that arrays and `TypedArray` can contain when formatting. Default is `100`. Set to `null` to display all array elements. Set to `0*` or a negative number to not display array elements. *`breakLength
`The keys of an object are split into multiple line lengths. Set to `Infinity` to format an object into a single line. The default is `60`.
Custom util.inspect Color
You can globally customize the color output of util.inspect through the util.inspect.styles and util.inspect.colors properties (if enabled)
const util = require('util'); console.log(util.format('您输入了%d个参数,参数值分别为%s,%s,%s', 3, 'nice', 'excelent', 'holy')); //您输入了3个参数,参数值分别为nice,excelent,holy console.log(util.format('一个JSON对象%j', {'name': 'jack', 'age': 25})); // 一个JSON对象{"name":"jack","age":25} console.log(util.format('一个百分号%'));// 一个百分号% console.log(util.format('%s:%s', 'one'));// one:%s console.log(util.format('%s', 'one', 'two', 'three', {'name': 'jack'})); function test(one, two) { return one + two; } let parent = new Object(); parent.name = 'parent'; parent.func = test; let child1 = new Object(); child1.name = 'child1'; parent.child1 = child1; let child2 = new Object(); child2.name = 'child2'; child1.child = child2; let child3 = new Object(); child3.name = 'child3'; child2.child = child3; child2.inspect = function (depth) { return util.inspect(this, {depth: depth - 2, customInspect: false}) }; console.log(util.inspect(parent, {customInspect: true, depth: 4})); /** * { name: 'parent', * func: [Function: test], * child1: * { name: 'child1', * child: { name: 'child2', child: [Object], inspect: [Function] } } } * **/
The above is what I compiled for everyone , I hope it will be helpful to everyone in the future.
Related articles:
Usage of vue carousel plug-in vue-concise-slider
Instances of executing functions after leaving the vue page
Solve the problem of vue page refresh or loss of back parameters
The above is the detailed content of How to use readline module and util module in Node.js. For more information, please follow other related articles on the PHP Chinese website!
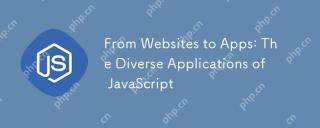
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
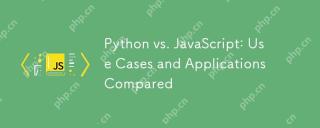
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
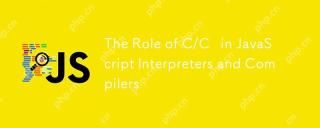
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
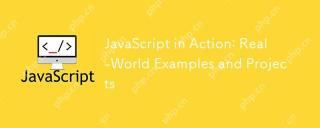
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
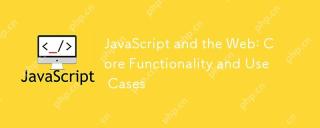
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
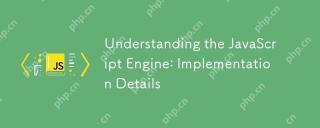
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
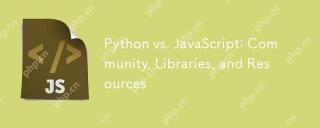
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
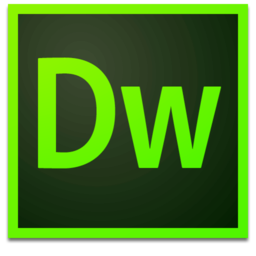
Dreamweaver Mac version
Visual web development tools
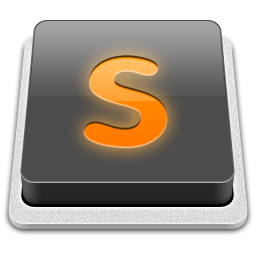
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
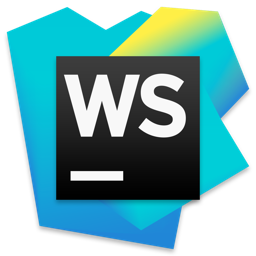
WebStorm Mac version
Useful JavaScript development tools