This time I will show you how to use react router4 redux to implement routing authority control, and what are the precautions for using react router4 redux to implement routing authority control. The following is a practical case, let's take a look.
Overview
A complete routing system should look like this. When the component linked to is required to be logged in before it can be viewed, it must be able to jump to the login page. , and then after successful login, it will jump back to the page you wanted to visit before. Here we mainly use a permission control class to define routingrouting information, and at the same time use redux to save the routing address to be accessed after successful login. When the login is successful, check whether there is any in redux Save the address. If no address is saved, jump to the default routing address.
Routing permission control class
In this method, use sessionStorage to determine whether you are logged in. If you are not logged in, save the current route you want to jump to redux. in. Then jump to our login page.
import React from 'react' import { Route, Redirect } from 'react-router-dom' import { setLoginRedirectUrl } from '../actions/loginAction' class AuthorizedRoute extends React.Component { render() { const { component: Component, ...rest } = this.props const isLogged = sessionStorage.getItem("userName") != null ? true : false; if(!isLogged) { setLoginRedirectUrl(this.props.location.pathname); } return ( <route> { return isLogged ? <component></component> : <redirect></redirect> }} /> ) } } export default AuthorizedRoute</route>
Routing definitionInformation
Routing information is also very simple. Only use AuthorizedRoute to define routes that need to be logged in to view.
import React from 'react' import { BrowserRouter, Switch, Route, Redirect } from 'react-router-dom' import Layout from '../pages/layout/Layout' import Login from '../pages/login/Login' import AuthorizedRoute from './AuthorizedRoute' import NoFound from '../pages/noFound/NoFound' import Home from '../pages/home/Home' import Order from '../pages/Order/Order' import WorkOrder from '../pages/Order/WorkOrder' export const Router = () => ( <browserrouter> <p> <switch> <route></route> <redirect></redirect>{/*注意redirect转向的地址要先定义好路由*/} <authorizedroute></authorizedroute> <route></route> </switch> </p> </browserrouter> )
Login page
is to take out the address stored in redux. After successful login, jump to it. If not, jump to the default page. I This is the default to jump to the home page. Because the antd form is used, the code is a bit long. You only need to look at the two sentences connecting redux and the content in handleSubmit.
import React from 'react' import './Login.css' import { login } from '../../mock/mock' import { Form, Icon, Input, Button, Checkbox } from 'antd'; import { withRouter } from 'react-router-dom'; import { connect } from 'react-redux' const FormItem = Form.Item; class NormalLoginForm extends React.Component { constructor(props) { super(props); this.isLogging = false; } handleSubmit = (e) => { e.preventDefault(); this.props.form.validateFields((err, values) => { if (!err) { this.isLogging = true; login(values).then(() => { this.isLogging = false; let toPath = this.props.toPath === '' ? '/layout/home' : this.props.toPath this.props.history.push(toPath); }) } }); } render() { const { getFieldDecorator } = this.props.form; return (); } } const WrappedNormalLoginForm = Form.create()(NormalLoginForm); const loginState = ({ loginState }) => ({ toPath: loginState.toPath }) export default withRouter(connect( loginState )(WrappedNormalLoginForm))
By the way, let’s talk about the use of redux here. For the time being, I will only basically use the method: define reducer, define actions, create store, and then connect redux when I need to use redux variables. When I need to dispatch to change the variables, I will directly introduce the methods in actions. , just call it directly. In order to match the event names in actions and reducer, I created an actionsEvent.js to store the event names for fear of typos and easy modification later.
reducer:
import * as ActionEvent from '../constants/actionsEvent' const initialState = { toPath: '' } const loginRedirectPath = (state = initialState, action) => { if(action.type === ActionEvent.Login_Redirect_Event) { return Object.assign({}, state, { toPath: action.toPath }) } return state; } export default loginRedirectPath
actions:
import store from '../store' import * as ActionEvent from '../constants/actionsEvent' export const setLoginRedirectUrl = (toPath) => { return store.dispatch({ type: ActionEvent.Login_Redirect_Event, toPath: toPath }) }
Create store
import { createStore, combineReducers } from 'redux' import loginReducer from './reducer/loginReducer' const reducers = combineReducers({ loginState: loginReducer //这里的属性名loginState对应于connect取出来的属性名 }) const store = createStore(reducers) export default store
I believe you have mastered the method after reading the case in this article. For more exciting information, please pay attention to other php Chinese websites related articles!
Recommended reading:
Using JS to determine the content contained in a string Summary of methods
Angular RouterLink makes different flowers Type jump
The above is the detailed content of How to use react router4+redux to implement routing permission control. For more information, please follow other related articles on the PHP Chinese website!
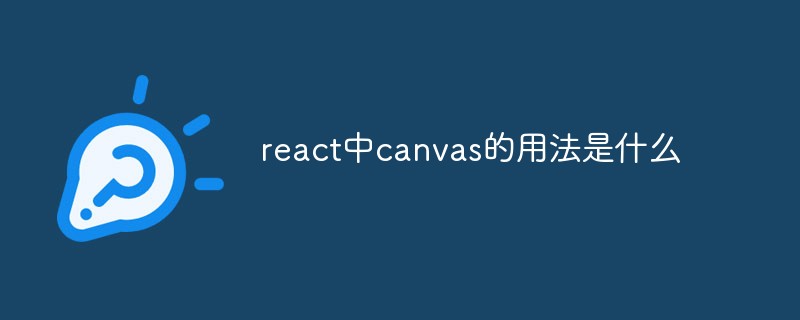
在react中,canvas用于绘制各种图表、动画等;可以利用“react-konva”插件使用canvas,该插件是一个canvas第三方库,用于使用React操作canvas绘制复杂的画布图形,并提供了元素的事件机制和拖放操作的支持。
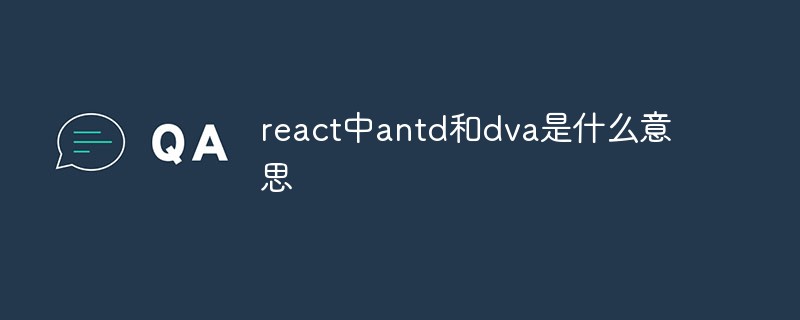
在react中,antd是基于Ant Design的React UI组件库,主要用于研发企业级中后台产品;dva是一个基于redux和“redux-saga”的数据流方案,内置了“react-router”和fetch,可理解为应用框架。
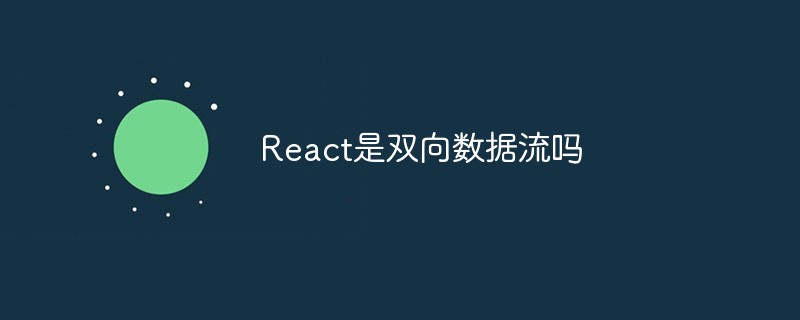
React不是双向数据流,而是单向数据流。单向数据流是指数据在某个节点被改动后,只会影响一个方向上的其他节点;React中的表现就是数据主要通过props从父节点传递到子节点,若父级的某个props改变了,React会重渲染所有子节点。
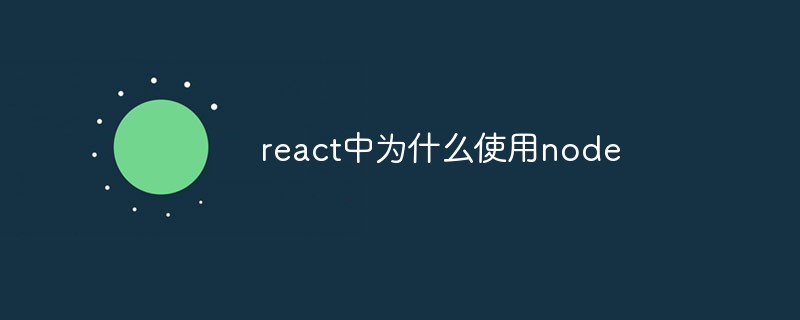
因为在react中需要利用到webpack,而webpack依赖nodejs;webpack是一个模块打包机,在执行打包压缩的时候是依赖nodejs的,没有nodejs就不能使用webpack,所以react需要使用nodejs。
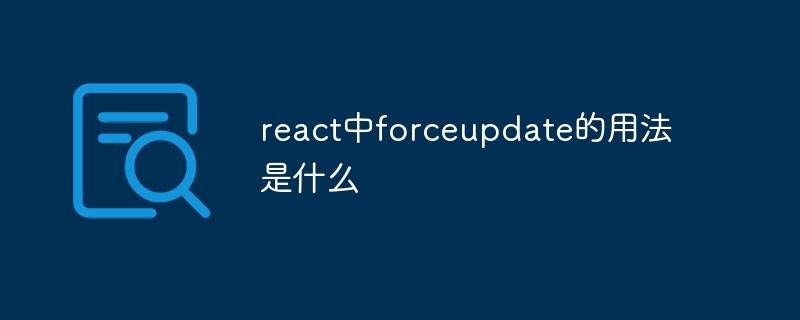
在react中,forceupdate()用于强制使组件跳过shouldComponentUpdate(),直接调用render(),可以触发组件的正常生命周期方法,语法为“component.forceUpdate(callback)”。
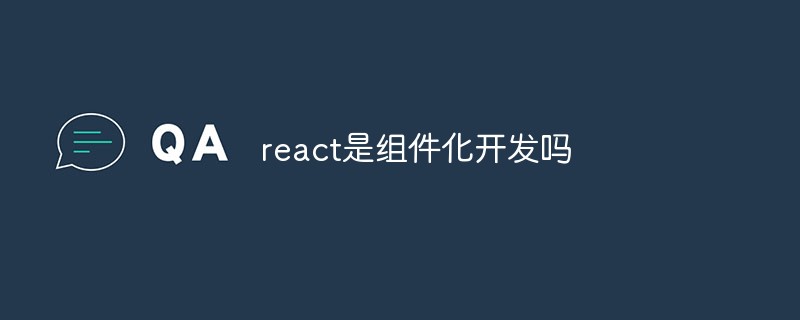
react是组件化开发;组件化是React的核心思想,可以开发出一个个独立可复用的小组件来构造应用,任何的应用都会被抽象成一颗组件树,组件化开发也就是将一个页面拆分成一个个小的功能模块,每个功能完成自己这部分独立功能。
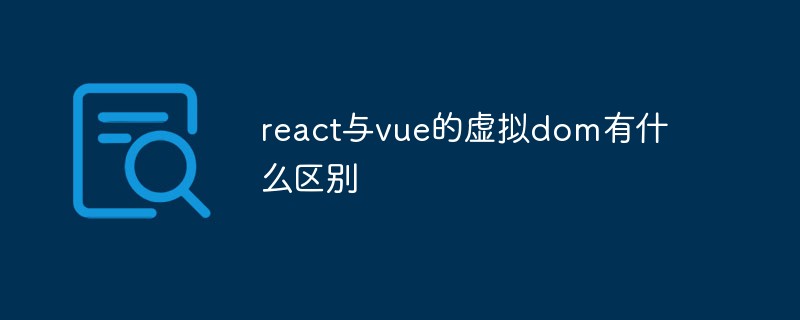
react与vue的虚拟dom没有区别;react和vue的虚拟dom都是用js对象来模拟真实DOM,用虚拟DOM的diff来最小化更新真实DOM,可以减小不必要的性能损耗,按颗粒度分为不同的类型比较同层级dom节点,进行增、删、移的操作。
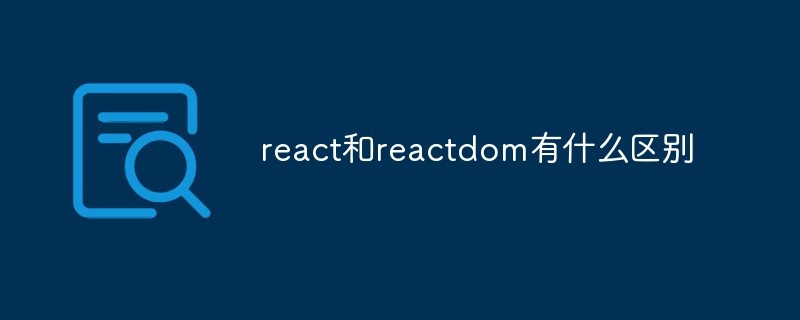
react和reactdom的区别是:ReactDom只做和浏览器或DOM相关的操作,例如“ReactDOM.findDOMNode()”操作;而react负责除浏览器和DOM以外的相关操作,ReactDom是React的一部分。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
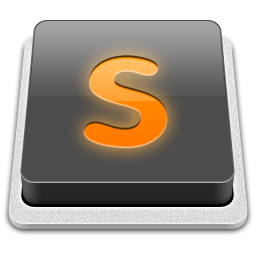
SublimeText3 Mac version
God-level code editing software (SublimeText3)
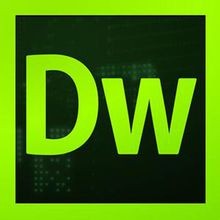
Dreamweaver CS6
Visual web development tools
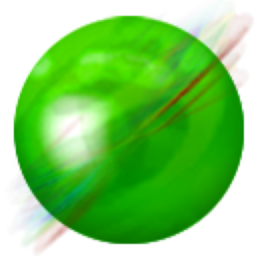
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
