


Python cookbook (String and Text) splits string operations for any number of delimiters
This article mainly introduces the Python cookbook (string and text) to split the string operation for any number of delimiters, and analyzes the use of split() and regular expressions in Python to split the string using examples. For implementation tips, friends in need can refer to
. The example in this article describes Python's string splitting operation for any number of delimiters. Share it with everyone for your reference, the details are as follows:
Question: Split strings with inconsistent delimiters (and spaces between delimiters) into different fields;
Solution: Use the more flexible re.split() method, which can specify multiple patterns for the delimiter.
Note: The split() of the string object can only handle simple situations, and does not support multiple delimiters, and it cannot do anything about the spaces that may exist around the delimiters.
# example.py # # Example of splitting a string on multiple delimiters using a regex import re #导入正则表达式模块 line = 'asdf fjdk; afed, fjek,asdf, foo' # (a) Splitting on space, comma, and semicolon parts = re.split(r'[;,\s]\s*', line) print(parts) # (b) 正则表达式模式中使用“捕获组”,需注意捕获组是否包含在括号中,使用捕获组导致匹配的文本也包含在最终结果中 fields = re.split(r'(;|,|\s)\s*', line) print(fields) # (c) 根据上文的分隔字符改进字符串的输出 values = fields[::2] delimiters = fields[1::2] delimiters.append('') print('value =', values) print('delimiters =', delimiters) newline = ''.join(v+d for v,d in zip(values, delimiters)) print('newline =', newline) # (d) 使用非捕获组(?:...)的形式实现用括号对正则表达式模式分组,且不输出分隔符 parts = re.split(r'(?:,|;|\s)\s*', line) print(parts)
>>> ================================ RESTART ================================ >>> ['asdf', 'fjdk', 'afed', 'fjek', 'asdf', 'foo'] ['asdf', ' ', 'fjdk', ';', 'afed', ',', 'fjek', ',', 'asdf', ',', 'foo'] value = ['asdf', 'fjdk', 'afed', 'fjek', 'asdf', 'foo'] delimiters = [' ', ';', ',', ',', ',', ''] newline = asdf fjdk;afed,fjek,asdf,foo ['asdf', 'fjdk', 'afed', 'fjek', 'asdf', 'foo'] >>>
(Code is excerpted from "Python Cookbook")
Related recommendations:
PythonCookbook - Data Structures and Algorithms
The above is the detailed content of Python cookbook (String and Text) splits string operations for any number of delimiters. For more information, please follow other related articles on the PHP Chinese website!
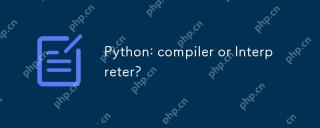
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
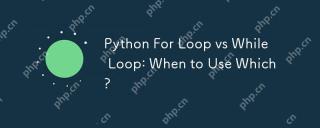
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
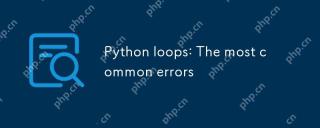
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i
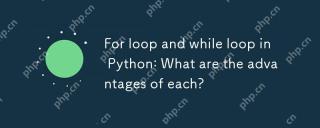
Forloopsareadvantageousforknowniterationsandsequences,offeringsimplicityandreadability;whileloopsareidealfordynamicconditionsandunknowniterations,providingcontrolovertermination.1)Forloopsareperfectforiteratingoverlists,tuples,orstrings,directlyacces
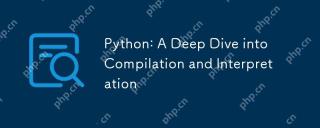
Pythonusesahybridmodelofcompilationandinterpretation:1)ThePythoninterpretercompilessourcecodeintoplatform-independentbytecode.2)ThePythonVirtualMachine(PVM)thenexecutesthisbytecode,balancingeaseofusewithperformance.
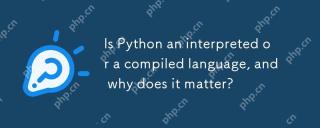
Pythonisbothinterpretedandcompiled.1)It'scompiledtobytecodeforportabilityacrossplatforms.2)Thebytecodeistheninterpreted,allowingfordynamictypingandrapiddevelopment,thoughitmaybeslowerthanfullycompiledlanguages.
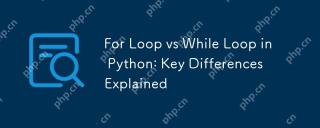
Forloopsareidealwhenyouknowthenumberofiterationsinadvance,whilewhileloopsarebetterforsituationswhereyouneedtoloopuntilaconditionismet.Forloopsaremoreefficientandreadable,suitableforiteratingoversequences,whereaswhileloopsoffermorecontrolandareusefulf
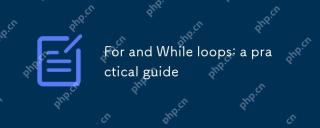
Forloopsareusedwhenthenumberofiterationsisknowninadvance,whilewhileloopsareusedwhentheiterationsdependonacondition.1)Forloopsareidealforiteratingoversequenceslikelistsorarrays.2)Whileloopsaresuitableforscenarioswheretheloopcontinuesuntilaspecificcond


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
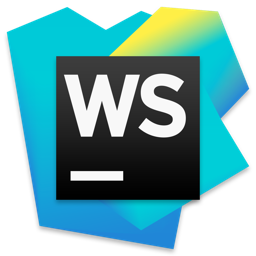
WebStorm Mac version
Useful JavaScript development tools
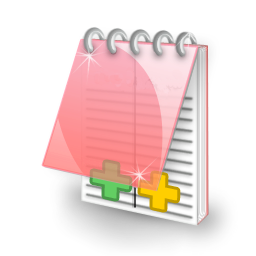
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
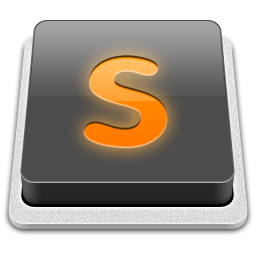
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor
