This time I will show you how to use this in React components. What are the precautions when using this in React components. Here are practical cases, let’s take a look.
What is this of the React component?
By writing a simple component and rendering it, print out the custom function and the this:
import React from 'react'; const STR = '被调用,this指向:'; class App extends React.Component{ constructor(){ super() } //测试函数 handler() { console.log(`handler ${STR}`,this); } render(){ console.log(`render ${STR}`,this); return( <p> </p><h1 id="hello-World">hello World</h1> <label>单击打印函数handler中this的指向</label> <input> ) } } export default App
The result is as shown in the figure:
As you can see, this in the render function points to the component instance, and this in the handler() function is undefined, why is this?
This in JavaScript function
We all know that this in JavaScript function is not defined when the function is declared, but when the function is called (that is, run)
var student = { func: function() { console.log(this); }; }; student.func(); var studentFunc = student.func; studentFunc();
defined when running this code, you can see that student.func() prints the student object, because this points to the student object at this time; and studentFunc() prints window, because it is called by window at this time , this points to window.
This code vividly verifies that this in the JavaScript function is not defined when the function is declared, but when the function is running;
Similarly, React components also follow JavaScript This feature means that different 'callers' of component methods will lead to differences in this (the "caller" here refers to the current object when the function is executed)
Different "callers" will cause this is different
Test: print this in the lifecycle function and custom function that come with the component, and use this.handler() in the render() method respectively , window.handler(), onCilck={this.handler} These three methods call handler():
/App.jsx
//测试函数 handler() { console.log(`handler ${STR}`,this); } render(){ console.log(`render ${STR}`,this); this.handler(); window.handler = this.handler; window.handler(); return( <p> </p><h1 id="hello-World">hello World</h1> <label>单击打印函数handler中this的指向</label> <input> ) } } export default App
Yes See: this -> component instance App object in
render; this.handler() -> component instance in
render App object;
render in window.handler() -> window object;
- ##onClick ={this .handler} -> undefined
Use Three buttons trigger the loading, updating and uninstalling process of components: /index.htmlimport React from 'react' import {render,unmountComponentAtNode} from 'react-dom' import App from './App.jsx' const root=document.getElementById('root') console.log("首次挂载"); let instance = render(<app></app>,root); window.renderComponent = () => { console.log("挂载"); instance = render(<app></app>,root); } window.setState = () => { console.log("更新"); instance.setState({foo: 'bar'}); } window.unmountComponentAtNode = () => { console.log('卸载'); unmountComponentAtNode(root); }
Run the program, click "Mount" in turn, and bind onClick={this.handler} The results of "Click" button, "Update" and "Uninstall" buttons are as follows:nbsp;html> <title>react-this</title> <button>挂载</button> <button>更新</button> <button>卸载</button> <p> <!-- app --> </p>
Automatic binding and manual binding
- React.createClass has a built-in magic that can automatically bind the method used so that its this points to The instantiation object of the component, but other JavaScript classes do not have this feature;
- So the React team decided not to implement automatic binding in the React component class and give the freedom of context conversion to For developers;
- So we usually bind the this point of the method in the
constructor :
import React from 'react'; const STR = '被调用,this指向:'; class App extends React.Component{ constructor(){ super(); this.handler = this.handler.bind(this); } //测试函数 handler() { console.log(`handler ${STR}`,this); } render(){ console.log(`render ${STR}`,this); this.handler(); window.handler = this.handler; window.handler(); return( <p> </p><h1 id="hello-World">hello World</h1> <label>单击打印函数handler中this的指向</label> <input> ) } } export default App
将this.handler()绑定为组件实例后,this.handler()中的this就指向组将实例,即onClick={this.handler}打印出来的为组件实例;
总结:
React组件生命周期函数中的this指向组件实例;
自定义组件方法的this会因调用者不同而不同;
为了在组件的自定义方法中获取组件实例,需要手动绑定this到组将实例。
相信看了本文案例你已经掌握了方法,更多精彩请关注php中文网其它相关文章!
推荐阅读:
The above is the detailed content of How to use this in React components. For more information, please follow other related articles on the PHP Chinese website!
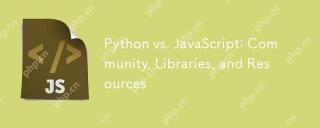
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
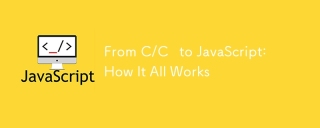
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
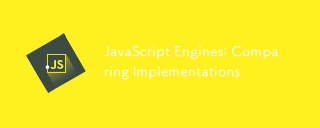
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
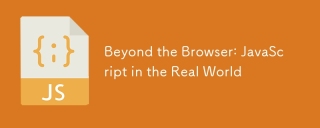
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
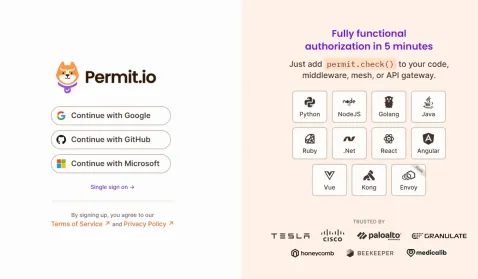
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
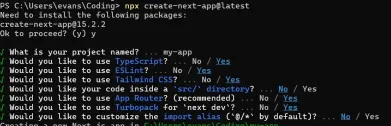
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
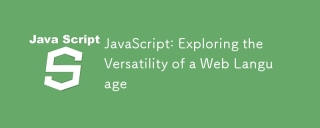
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
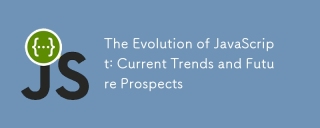
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
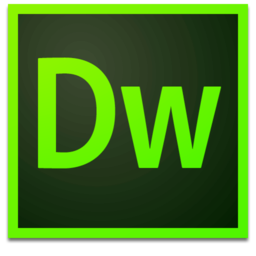
Dreamweaver Mac version
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.