With the rise of HTML5 and JavaScript trying to dominate the world, a technology called [cross-platform] is becoming more and more popular. Why is it so popular? Because software developers only need to write the program once, it can be run on Windows, Linux, Mac, IOS, Android and other platforms, which greatly reduces the workload of programmers and allows the company's products to be iterated quickly. This article mainly introduces how Electron packages web pages into desktop applications (how web front-end pages generate exe executable files). I hope it will be useful to everyone.
Cross-platform technology was once not considered promising, but now it is developing rapidly with the development of mobile phones and computer hardware. All of this is almost driven by HTML5 technology. Of course, JavaScript is the biggest contributor. The well-known cross-platform technologies based on HTML5 include PhoneGap and Cordova, which are often used to develop webapps; there are also Egret, Cocos-creator, Unity, etc., which are often used to develop games; and nw.js based on Node.js, which is used for development Desktop applications, and Electron, an artifact that uses web technology to develop desktop applications that is more powerful than nw.js.
Actually, the above is all nonsense, now let’s get to the topic: How to use Electron to package web pages into exe executable files!
Assumptions:
1. You have installed and configured node.js (global installation)
2. You have installed electron using npm (global installation)
3 , You have already written the front-end web page (html, css, javascript, etc., or the web page written based on the front-end framework)
4. If you can’t understand the above three points, go to Baidu quickly. . .
If you have the above assumptions, please continue reading:
1. Find your front-end web page project folder, create new package.json, main.js, index.html 3 file (note: index.html is the home page of your web page)
Your project directory/
├── package.json ├── main.js └── index.html
2. In package.json Add the following content
{ "name" : "app-name", "version" : "0.1.0", "main" : "main.js" }
3. Add the following content in main.js. This main.js file is the value of the "main" key in the package.json above. So you can modify it as needed
const {app, BrowserWindow} = require('electron') const path = require('path') const url = require('url') // Keep a global reference of the window object, if you don't, the window will // be closed automatically when the JavaScript object is garbage collected. let win function createWindow () { // Create the browser window. win = new BrowserWindow({width: 800, height: 600}) // and load the index.html of the app. win.loadURL(url.format({ pathname: path.join(__dirname, 'index.html'), protocol: 'file:', slashes: true })) // Open the DevTools. // win.webContents.openDevTools() // Emitted when the window is closed. win.on('closed', () => { // Dereference the window object, usually you would store windows // in an array if your app supports multi windows, this is the time // when you should delete the corresponding element. win = null }) } // This method will be called when Electron has finished // initialization and is ready to create browser windows. // Some APIs can only be used after this event occurs. app.on('ready', createWindow) // Quit when all windows are closed. app.on('window-all-closed', () => { // On macOS it is common for applications and their menu bar // to stay active until the user quits explicitly with Cmd + Q if (process.platform !== 'darwin') { app.quit() } }) app.on('activate', () => { // On macOS it's common to re-create a window in the app when the // dock icon is clicked and there are no other windows open. if (win === null) { createWindow() } }) // In this file you can include the rest of your app's specific main process // code. You can also put them in separate files and require them here.
4. If the file name of your web page homepage is not "index.html", then please change the 'index' in main.js .html' is changed to the name of your webpage home page
5. Open DOS, cd to your project directory (or directly shift+right-click in a blank space in your project directory, and then click Open command here Window, I can’t understand it here, alas, Baidu Bar Boy)
6. Under DOS in the previous step, enter npm install electron-packager -g
Globally install our packaging artifact
npm install electron-packager -g
7. After installing the packaging artifact, still under DOS in the previous step, enter electron-packager . app --win --out presenterTool --arch= x64 --version 1.4.14 --overwrite --ignore=node_modules
to start packaging
electron-packager . app --win --out presenterTool --arch=x64 --version 1.4.14 --overwrite --ignore=node_modules
What does this command mean? The blue part can be modified by yourself:
electron-packager. The file name of the executable file--win --out the packaged folder name--arch=x64 bit or 32 bit--version version number- -overwrite --ignore=node_modules
8. After the packaging is successful, a new folder will be generated. Click in it and find the exe file. Double-click it to see the web page change. It has become a desktop application!
The above is the simplest packaging method. As for how to modify the window size, how to add a menu bar, how to call the system API, etc., I will slowly study Electron for you.
If your packaging always fails and you find it annoying, and you have no requirements for extended functions,
Click to enter my Coding code repository: https://coding.net/u/ linhongbijkm/p/Electron-packager-build-project/git
contains the index.html webpage with the content hello,world that I have packaged into a desktop application under the Windows environment through the Electron framework.
Now just copy your web front-end project to the /resources/app/project directory, double-click the exe file to run your web page as a desktop application.
Related recommendations:
php and js open the local exe program and pass the relevant parameters method
How to do it on the web page Call the desktop exe program
Installable exe program implementation method in Java
The above is the detailed content of How to generate exe executable file from web front-end page. For more information, please follow other related articles on the PHP Chinese website!
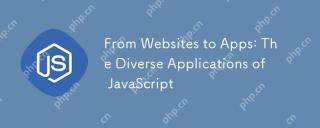
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
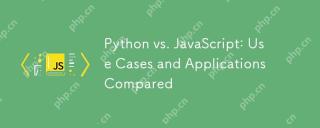
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
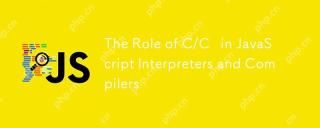
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
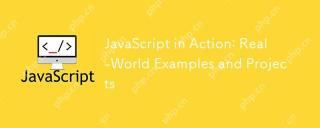
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
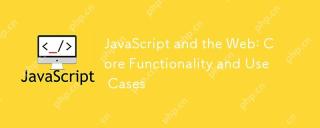
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
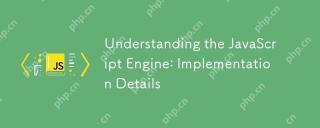
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
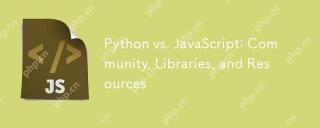
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
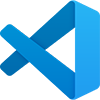
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
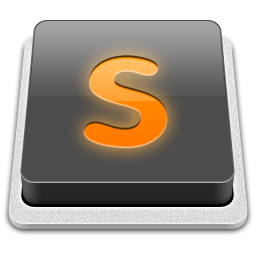
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
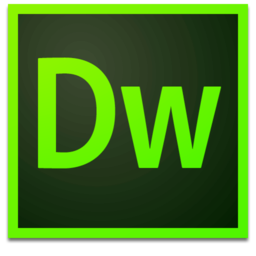
Dreamweaver Mac version
Visual web development tools