The Map type in ECMAScript 6 is an ordered list that stores many key-value pairs. Key-value pairs support all data types. Keys 0 and '0' will be treated as two different keys, and no forced type conversion will occur. This article mainly brings you an es6 series tutorial_ Map detailed explanation and common API introduction.
How to use Map?
let map = new Map();
Common methods:
set(key, value): Add a new key-value pair element
get(key) : Get the value corresponding to the key. If the value does not exist, return undefined
let map = new Map(); map.set( '0', 'ghostwu' ); map.set( 0, 'ghostwu' ); console.log( map.get( '0' ) ); //ghostwu console.log( map.get( 'name' ) ); //undefined;
let map = new Map(); var key1 = {}, key2 = {}; map.set( key1, 'ghostwu' ); map.set( key2, 22 ); console.log( map.get( key1 ) ); //ghostwu console.log( map.get( key2 ) ); //22
You can use objects as the keys of the Map. Although they are two empty objects, strong type conversion will not occur.
has( key ): Determine whether the key name exists
delete( key ): Delete the key name and the corresponding value
clear(): Remove all key-value pairs in the map collection
size: The number of elements in the map collection
let map = new Map(); map.set( 'name', 'ghostwu' ); map.set( 'age', 22 ); console.log( map.has( 'name' ) );//true console.log( map.size ); //2 map.delete( 'name' ); console.log( map.has( 'name' ) );//false console.log( map.size ); //1 console.log( map.has( 'age' ) ); //true map.clear(); console.log( map.size ); //0 console.log( map.has( 'age' ) ); //false
Map supports array initialization, using a two-dimensional array, and each array uses key-value pairs
let map = new Map( [ [ 'name', 'ghostwu' ], [ 'age', 22 ] ] ); console.log( map.has( 'name') ); //true console.log( map.has( 'age') ); //true console.log( map.size ); //2 map.set( 'sex', 'man' ); console.log( map.size ); console.log( map.get( 'name' ) ); //ghostwu map.clear(); console.log( map.size ); //0
Map also Supports the forEach method, supports 2 parameters, the first one: function, the function supports 3 parameters (value, key, current map), the second one: this
let map = new Map( [ [ 'name', 'ghostwu' ], [ 'age', 22 ] ] ); map.set( 'sex', 'man' ); map.forEach( function( val, key, cur ){ console.log( val, key, cur, this ); }, 100 );
Related recommendations:
Detailed introduction to the summary of commonly used APIs for JavaScript operations on DOM
Detailed explanation of html5 canvas common API summary (2)--Drawing API
The above is the detailed content of Detailed explanation of Map and common API examples. For more information, please follow other related articles on the PHP Chinese website!
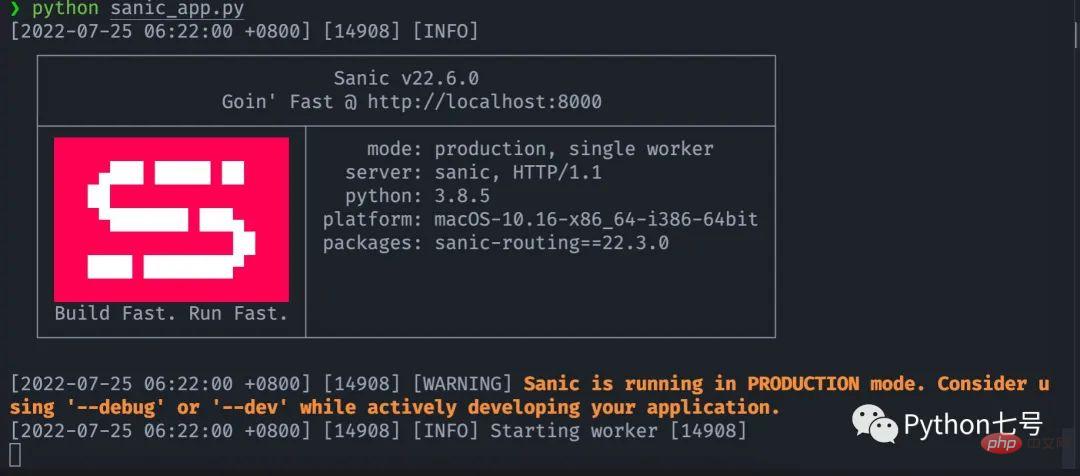
提到API开发,你可能会想到DjangoRESTFramework,Flask,FastAPI,没错,它们完全可以用来编写API,不过,今天分享的这个框架可以让你更快把现有的函数转化为API,它就是Sanic。Sanic简介Sanic[1],是Python3.7+Web服务器和Web框架,旨在提高性能。它允许使用Python3.5中添加的async/await语法,这可以有效避免阻塞从而达到提升响应速度的目的。Sanic致力于提供一种简单且快速,集创建和启动于一体的方法
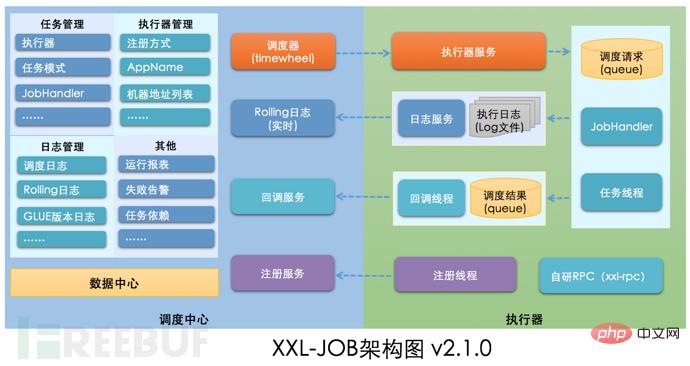
XXL-JOB描述XXL-JOB是一个轻量级分布式任务调度平台,其核心设计目标是开发迅速、学习简单、轻量级、易扩展。现已开放源代码并接入多家公司线上产品线,开箱即用。一、漏洞详情此次漏洞核心问题是GLUE模式。XXL-JOB通过“GLUE模式”支持多语言以及脚本任务,该模式任务特点如下:●多语言支持:支持Java、Shell、Python、NodeJS、PHP、PowerShell……等类型。●WebIDE:任务以源码方式维护在调度中心,支持通过WebIDE在线开发、维护。●动态生效:用户在线通
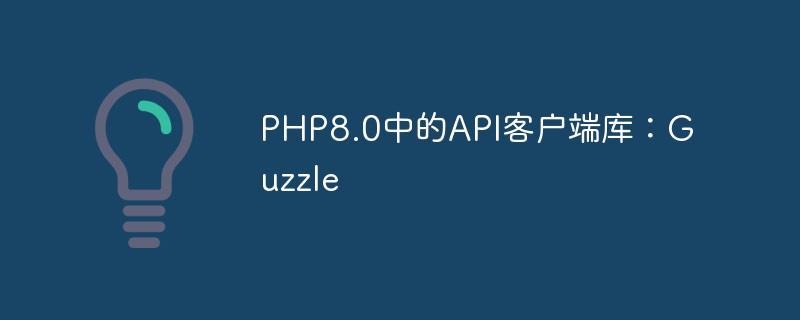
随着网络技术的发展,Web应用程序和API应用程序越来越普遍。为了访问这些应用程序,需要使用API客户端库。在PHP中,Guzzle是一个广受欢迎的API客户端库,它提供了许多功能,使得在PHP中访问Web服务和API变得更加容易。Guzzle库的主要目标是提供一个简单而又强大的HTTP客户端,它可以处理任何形式的HTTP请求和响应,并且支持并发请求处理。在
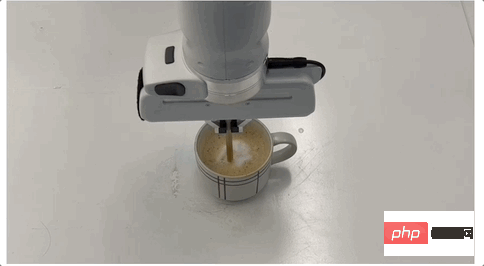
机器人也能干咖啡师的活了!比如让它把奶泡和咖啡搅拌均匀,效果是这样的:然后上点难度,做杯拿铁,再用搅拌棒做个图案,也是轻松拿下:这些是在已被ICLR 2023接收为Spotlight的一项研究基础上做到的,他们推出了提出流体操控新基准FluidLab以及多材料可微物理引擎FluidEngine。研究团队成员分别来自CMU、达特茅斯学院、哥伦比亚大学、MIT、MIT-IBM Watson AI Lab、马萨诸塞大学阿默斯特分校。在FluidLab的加持下,未来机器人处理更多复杂场景下的流体工作也都
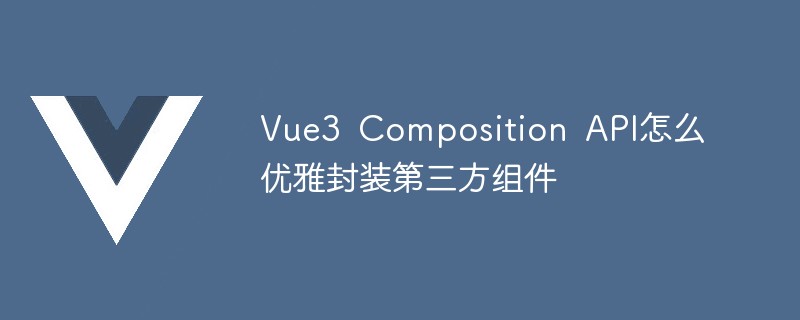
前言对于第三方组件,如何在保持第三方组件原有功能(属性props、事件events、插槽slots、方法methods)的基础上,优雅地进行功能的扩展了?以ElementPlus的el-input为例:很有可能你以前是这样玩的,封装一个MyInput组件,把要使用的属性props、事件events和插槽slots、方法methods根据自己的需要再写一遍://MyInput.vueimport{computed}from'vue'constprops=define
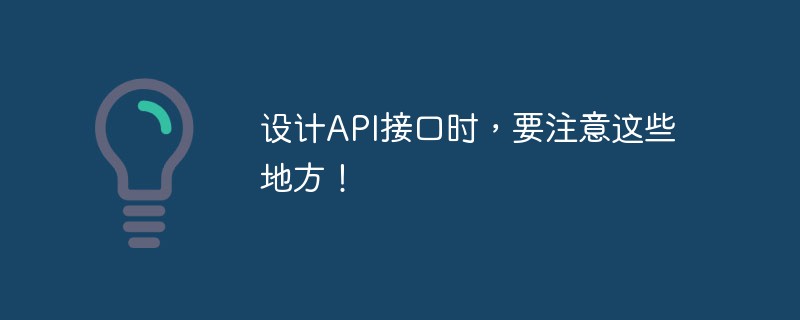
本篇文章给大家带来了关于API的相关知识,其中主要介绍了设计API需要注意哪些地方?怎么设计一个优雅的API接口,感兴趣的朋友,下面一起来看一下吧,希望对大家有帮助。
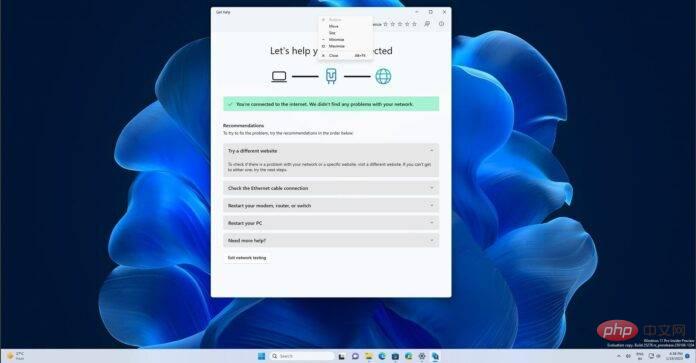
当您的WindowsPC出现网络问题时,问题出在哪里并不总是很明显。很容易想象您的ISP有问题。然而,Windows笔记本电脑上的网络并不总是顺畅的,Windows11中的许多东西可能会突然导致Wi-Fi网络中断。随机消失的Wi-Fi网络是Windows笔记本电脑上报告最多的问题之一。网络问题的原因各不相同,也可能因Microsoft的驱动程序或Windows而发生。Windows是大多数情况下的问题,建议使用内置的网络故障排除程序。在Windows11
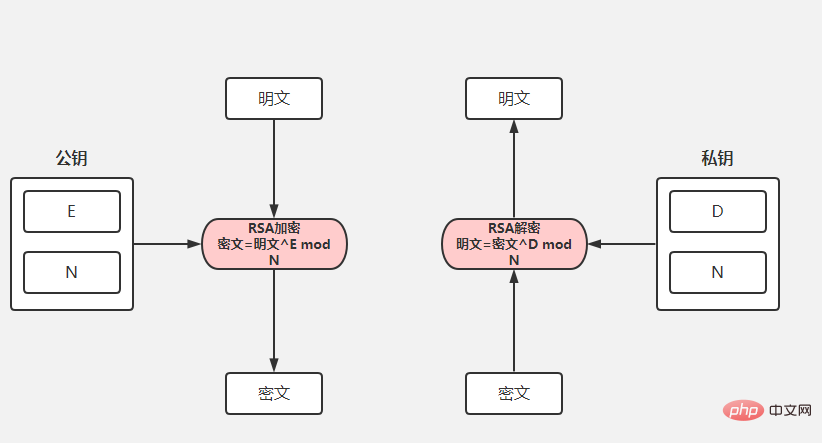
SpringBoot的API加密对接在项目中,为了保证数据的安全,我们常常会对传递的数据进行加密。常用的加密算法包括对称加密(AES)和非对称加密(RSA),博主选取码云上最简单的API加密项目进行下面的讲解。下面请出我们的最亮的项目rsa-encrypt-body-spring-boot项目介绍该项目使用RSA加密方式对API接口返回的数据加密,让API数据更加安全。别人无法对提供的数据进行破解。SpringBoot接口加密,可以对返回值、参数值通过注解的方式自动加解密。什么是RSA加密首先我


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
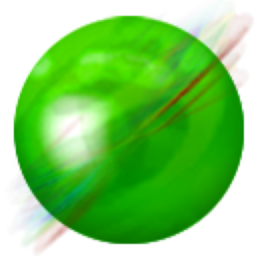
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor
