This article mainly introduces you to the detailed implementation of react server rendering from scratch. The editor thinks it is quite good, so I will share it with you now and give it as a reference. Let’s follow the editor to take a look, I hope it can help everyone.
Preface
When I was writing koa recently, I thought, if part of my code provides API and part of the code supports SSR, then how should I write it? Woolen cloth? (If you don’t want to split it into two services)
And I have also used some server-side rendering in the projects I have written recently, such as nuxt, and I have also worked on next projects. It is true that the development experience is very friendly, but friendly is still friendly. , how is it implemented specifically? Have you ever considered it?
Based on a pragmatic attitude, I chose react as the research object (mainly because Vue has been written too much, which is disgusting). Then I will simply write a react server-side rendering demo at the minimum cost.
Technology stack used
react 16 + webpack3 + koa2
Look at how it implements server-side rendering Yes, here we go!
Why use server-side rendering
Advantages
It’s nothing more than two points
SEO friendly
Speed up first screen rendering and reduce white screen time
Then the question arises What is SEO
In one sentence, most of the websites we make now are SPA websites. All pages and data come from ajax. When the search engine spider comes to collect the web pages, , found completely empty? So do you think the weight and effect of your website's inclusion are good or bad?
The core of our SEO optimization is also described in the following content:
The following is the key point!
Let the server return the HTML with content to us. If the event occurs, the browser will render it again to mount it
Build the koa environment
Create a new ssr project and initialize npm in the project
mkdir ssr && cd ssr npm init
In the following code we use syntax such as import jsx, which is not supported by the node environment. So you need to configure babel
Create new files app.js and index.js in the current project, and then
babel’s entrance, the index.js code is as follows
require('babel-core/register')() require('babel-polyfill') require('./app')
The entrance to our project, the app.js code is as follows
import Koa from 'koa' const app = new Koa() // response app.use((ctx) => { ctx.body = 'Hello Koa' }) app.listen(3000) console.log("系统启动,端口:3000")
Create a new .babelrc file in the root directory
Content Yes:
{ "presets": ["react", "env"] }
Install the dependencies required above
npm install babel-core babel-polyfill babel-preset-env babel-preset-react nodemon --save-dev npm i koa --save
Configure startup script
package .json
"scripts": { "dev": "nodemon index.js", }
Here you run npm run dev and open localhost:3000
You will see hello Koa
Yes It is not very simple to start a service
Install React
##
cnpm install react react-dom --saveCreate a new app folder in the root directory , and create a new main.js in the folderThe main.js code is as follows
import React from 'react' export default class Home extends React.Component { render () { return <p>hello world</p> } }Before modifying server.js
import Koa from 'koa' import React from 'react' import { renderToString } from 'react-dom/server' import App from './app/main' const app = new Koa() // response app.use(ctx => { let str = renderToString(<App />) ctx.body = str }) app.listen(3000) console.log('系统启动,端口:8080')At this time, npm run dev
import React from 'react' export default class Home extends React.Component { render () { return <p onClick={() => window.alert(123)}>hello world</p> } }Refresh our page again, hey, is it useless?That’s because the backend can only talk about components Rendering into a string of html, event binding and other things need to be performed on the browser side
So how do we bind the event?
Configure webpack
var path = require('path') var webpack = require('webpack') module.exports = { entry: { main: './app/index.js' }, output: { filename: '[name].js', path: path.join(__dirname, 'public'), publicPath: '/' }, resolve: { extensions: ['.js', '.jsx'] }, module: { loaders: [ {test: /\.jsx?$/, loaders: ['babel-loader'], } ] } }The above configuration sets the entry to the app/index.js fileThen we will create oneThe following is the code of app/index.js:
import Demo from './main' import ReactDOM from 'react-dom' import React from 'react' ReactDOM.render(<Demo />, document.getElementById('root'))Because browser rendering requires mounting the root component to a dom node, the react code given to us Setting up an entranceThere is a problem at this time, that is, the document object does not exist in the node environment, so how to solve it? does not exist? If it doesn’t exist, then I don’t need it. The core of SSR is to return specific HTML content in the requested URL. I don’t care about events, so I just return the root component directly to renderToString
cnpm i --save koa-static koa-views ejs
koa-static: 处理静态文件的中间件
koa-views: 配置模板的中间件
ejs:一个模板引擎
修改server.js的代码
import Koa from 'koa' import React from 'react' import { renderToString } from 'react-dom/server' import views from 'koa-views' import path from 'path' import Demo from './app/main' const app = new Koa() // 将/public文件夹设置为静态路径 app.use(require('koa-static')(__dirname + '/public')) // 将ejs设置为我们的模板引擎 app.use(views(path.resolve(__dirname, './views'), { map: { html: 'ejs' } })) // response app.use(async ctx => { let str = renderToString(<Demo />) await ctx.render('index', { root: str }) }) app.listen(3000) console.log('系统启动,端口:8080')
下面新建我们的渲染模板
新建一个views文件夹
里面新建一个index.html:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title>Document</title> <base href="/client" rel="external nofollow" > </head> <body> <p id="root"><%- root %></p> <script src="/main.js"></script> </body> </html>
这个 html 里面可以放一些变量,比如这个,就是等下要放renderToString结果的地方
/main.js则是react构建出来的代码
下面直接来测试一下我们的代码
1. 在 package.json里面
新增:
"scripts": { "dev": "nodemon index.js", "build": "webpack" },
2. 运行 npm run build, 构建出我们的react代码
3. npm run dev
点击一下代码,是不是会 alert(123)
tada 撒花,恭喜你,一个最简单服务器渲染就已经完成
到这里核心的思想就都已经讲完了,总结来说就下面三点:
起一个node服务
把react 根组件 renderToString渲染成字符串一起返回前端
前端再重新render一次
原理就是这么简单
但是具体开发的时候还会有各种各样的需求,比如:
不可能我每次改完代码都重新构建看效果吧 => 需要 实时构建
create-react-app 都是热更新,你还要刷新是不是太蠢了 => 需要支持热更新
其他一些配套的周边,如: react-router, redux 或者mobx怎么支持呢 => 需要完善的生态
.etc
这些问题都是用完 官方脚手架之后就回不去了的,所以更多的配置可以参考下面的repo(是一个工具链完善的最小实现),欢迎提PR
GitHub - ws456999/koa-react-ssr-starter: to understand && to explain how react ssr works
目前你可以在里面找到 react + react-router + mobx + postcss + 热更新的配置,除了react-router的配置有些差别,其他都跟client端差别不大
相关推荐:
The above is the detailed content of Implement basic methods of react server rendering. For more information, please follow other related articles on the PHP Chinese website!
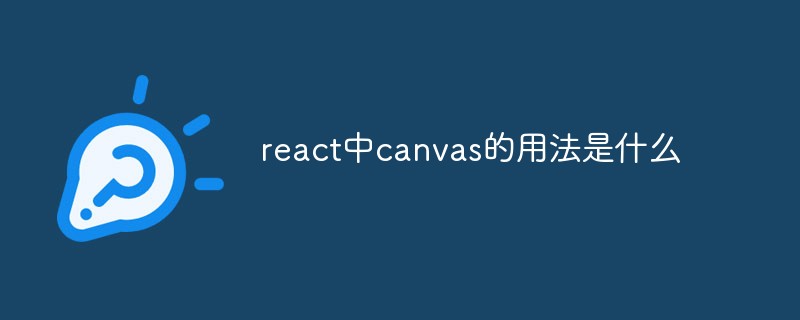
在react中,canvas用于绘制各种图表、动画等;可以利用“react-konva”插件使用canvas,该插件是一个canvas第三方库,用于使用React操作canvas绘制复杂的画布图形,并提供了元素的事件机制和拖放操作的支持。
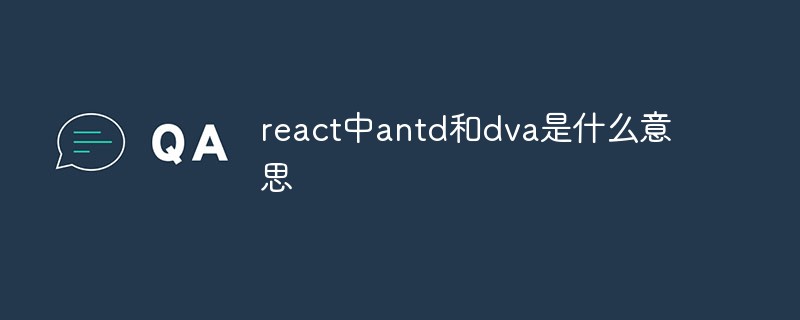
在react中,antd是基于Ant Design的React UI组件库,主要用于研发企业级中后台产品;dva是一个基于redux和“redux-saga”的数据流方案,内置了“react-router”和fetch,可理解为应用框架。
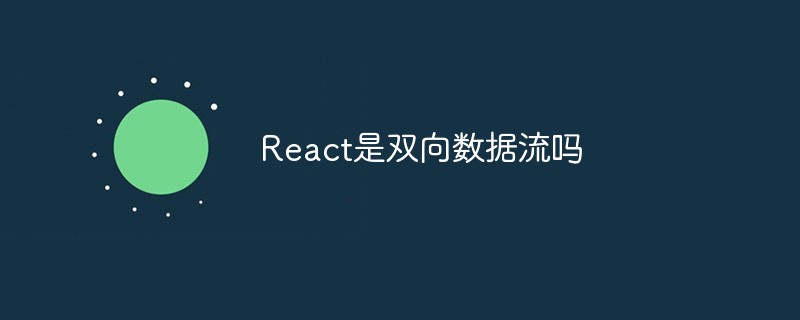
React不是双向数据流,而是单向数据流。单向数据流是指数据在某个节点被改动后,只会影响一个方向上的其他节点;React中的表现就是数据主要通过props从父节点传递到子节点,若父级的某个props改变了,React会重渲染所有子节点。
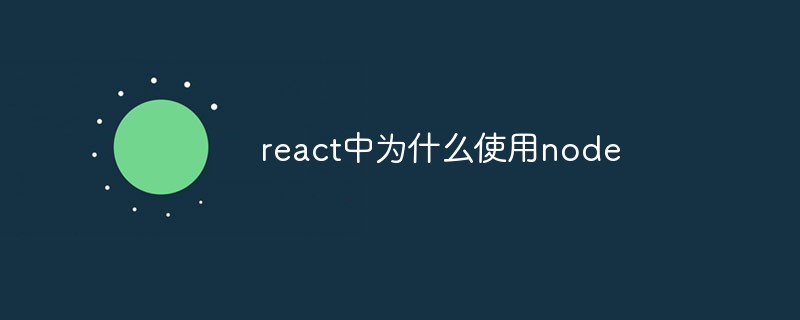
因为在react中需要利用到webpack,而webpack依赖nodejs;webpack是一个模块打包机,在执行打包压缩的时候是依赖nodejs的,没有nodejs就不能使用webpack,所以react需要使用nodejs。
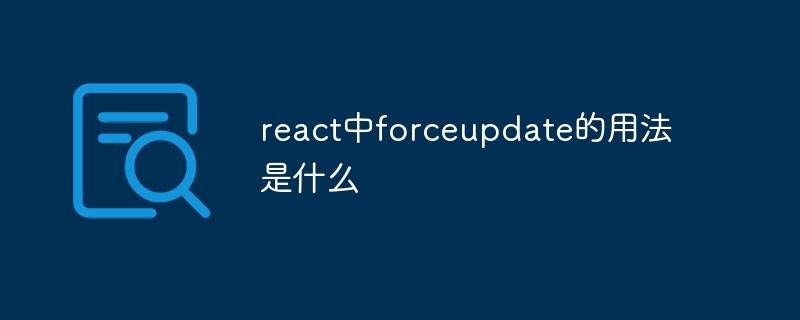
在react中,forceupdate()用于强制使组件跳过shouldComponentUpdate(),直接调用render(),可以触发组件的正常生命周期方法,语法为“component.forceUpdate(callback)”。
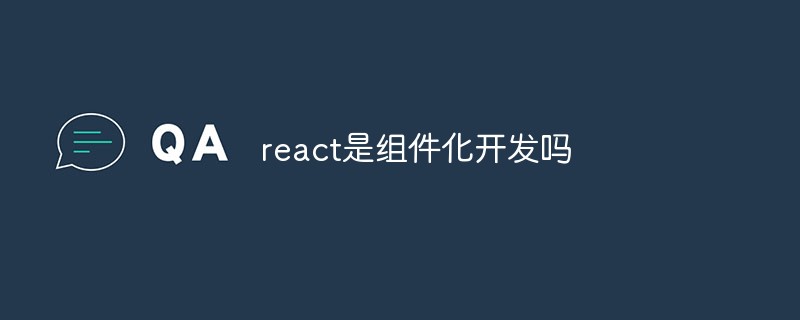
react是组件化开发;组件化是React的核心思想,可以开发出一个个独立可复用的小组件来构造应用,任何的应用都会被抽象成一颗组件树,组件化开发也就是将一个页面拆分成一个个小的功能模块,每个功能完成自己这部分独立功能。
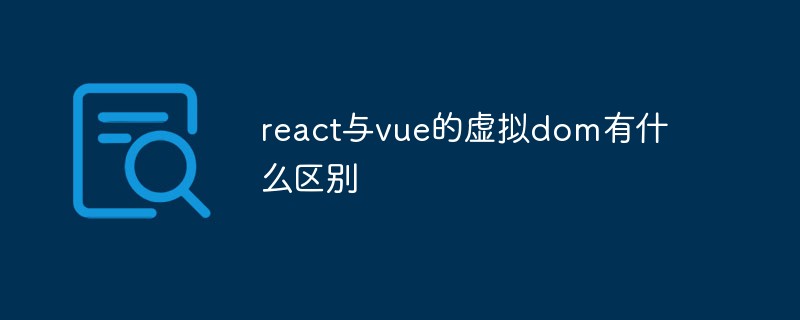
react与vue的虚拟dom没有区别;react和vue的虚拟dom都是用js对象来模拟真实DOM,用虚拟DOM的diff来最小化更新真实DOM,可以减小不必要的性能损耗,按颗粒度分为不同的类型比较同层级dom节点,进行增、删、移的操作。
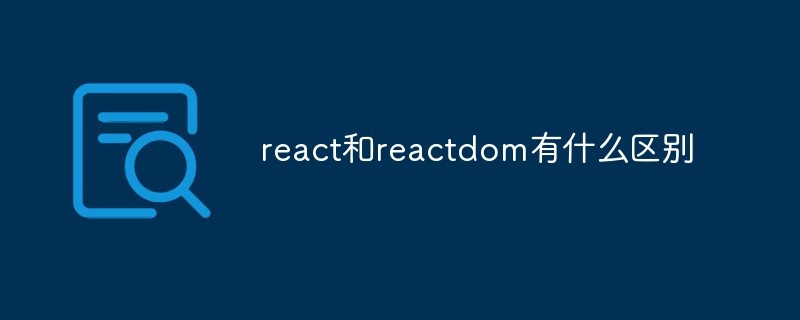
react和reactdom的区别是:ReactDom只做和浏览器或DOM相关的操作,例如“ReactDOM.findDOMNode()”操作;而react负责除浏览器和DOM以外的相关操作,ReactDom是React的一部分。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
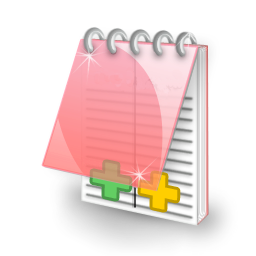
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
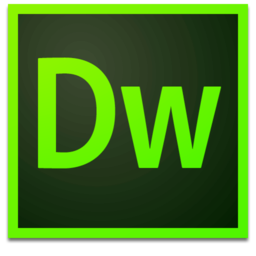
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
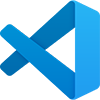
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
