


This article mainly shares with you a detailed explanation of the implementation of Ajax asynchronous request using JavaScript. Ajax is now a very popular technology. Although you can now use JQuery or some third-party plug-ins or even some controls provided by Microsoft to implement the ajax function, but It is also very important to understand its principle. The following is to use pure javascript to obtain server-side functions to show how to use pure javascript to implement ajax function to clarify its principle.
In the process of front-end page development, it is often Use Ajax requests to submit form data asynchronously or refresh the page asynchronously.
Generally speaking, it is very convenient to use $.ajax, $.post, $.getJSON in Jquery, but sometimes, we only need the ajax function, so it is not cost-effective to introduce Jquery.
So next, we will use native JavaScrpit to implement a simple Ajax request, and explain the cross-domain access issues in ajax requests, as well as the data synchronization issues of multiple ajax requests.
JavaScript implements Ajax asynchronous request
Simple ajax request implementation
The principle of Ajax request is to create an XMLHttpRequest object and use this object to send requests asynchronously. Please refer to the following code for specific implementation:
function ajax(option) { // 创建一个 XMLHttpRequest 对象 var xhr = window.XMLHttpRequest ? new XMLHttpRequest() : new ActiveXObject("Microsoft.XMLHTTP"), requestData = option.data, requestUrl = option.url, requestMethod = option.method; // 如果是GET请求,需要将option中的参数拼接到URL后面 if ('POST' != requestMethod && requestData) { var query_string = ''; // 遍历option.data对象,构建GET查询参数 for(var item in requestData) { query_string += item + '=' + requestData[item] + '&'; } // 注意这儿拼接的时候,需要判断是否已经有 ? requestUrl.indexOf('?') > -1 ? requestUrl = requestUrl + '&' + query_string : requestUrl = requestUrl + '?' + query_string; // GET 请求参数放在URL中,将requestData置为空 requestData = null; } // ajax 请求成功之后的回调函数 xhr.onreadystatechange = function () { // readyState=4表示接受响应完毕 if (xhr.readyState == ("number" == typeof XMLHttpRequest.DONE ? XMLHttpRequest.DONE : 4)) { if (200 == xhr.status) { // 判断状态码 var response = xhr.response || xhr.responseText || {}; // 获取返回值 // if define success callback, call it, if response is string, convert it to json objcet console.log(response); option.success && option.success(response); // 调用回调函数处理返回数据 // 可以判断返回数据类型,对数据进行JSON解析或者XML解析 // option.success && option.success('string' == typeof response ? JSON.parse(response) : response); } else { // if define error callback, call it option.error && option.error(xhr, xhr.statusText); } } }; // 发送ajax请求 xhr.open(requestMethod, requestUrl, true); // 请求超时的回调 xhr.ontimeout = function () { option.timeout && option.timeout(xhr, xhr.statusText); }; // 定义超时时间 xhr.timeout = option.timeout || 0; // 设置响应头部,这儿默认设置为json格式,可以定义为其他格式,修改头部即可 xhr.setRequestHeader && xhr.setRequestHeader('Content-Type', 'application/json;charset=utf-8'); xhr.withCredentials = (option.xhrFields || {}).withCredentials; // 这儿主要用于发送POST请求的数据 xhr.send(requestData); }
There are detailed comments in the above code. The principle of ajax is very simple. In general, it uses the XMLHttpRequest object to send data. Here is a supplementary explanation of this object.
Basic properties of the XMLHttpRequest object
The readyState property has five status values:
0: is uninitialized: not initialized. The XMLHttpRequest object has been created but not initialized.
1: It’s loading: it’s ready to be sent.
2: It is loaded,: It has been sent, but no response has been received yet.
3: It is interactive: the response is being received, but it has not been received yet.
4: Yes completed: Accepting the response is completed.
responseText: The response text returned by the server. It only has a value when readyState>=3. When readyState=3, the response text returned is incomplete. Only readyState=4, the complete response text is received.
responseXML: The response information is xml and can be parsed into a Dom object.
status: The HTTP status code of the server. If it is 200, it means OK, and 404 means not found.
statusText: The text of the server http status code. For example, OK, Not Found.
Basic methods of XMLHttpRequest object
open(method, url, asyn): Open the XMLHttpRequest object. The methods include get, post, delete, and put. url is the address of the requested resource. The third parameter indicates whether to use asynchronous. The default is true, because the characteristic of Ajax is asynchronous transmission. False if synchronization is used.
send(body): Send request Ajax. The content sent can be the required parameters. If there are no parameters, send directly (null)
Using method
Directly call the ajax function defined above and transfer the corresponding options and parameters That’s it.
ajax({ url: '/post.php', data: { name: 'uusama', desc: 'smart' }, method: 'GET', success: function(ret) { console.log(ret); } });
Cross-domain request issue
When using ajax requests, you must pay attention to one issue: cross-domain requests.
Without using special means, cross-domain requests: When requesting URL resources under other domain names and ports, Access-Control-Allow-Origin related errors will be reported. The main reason is the browser's same-origin policy restriction, which stipulates that cross-domain resource requests cannot be made.
Solution
Here are some simple solutions.
Add a header that allows cross-domain requests in the ajax header. This method also requires the server to cooperate with adding a header that allows cross-domain requests. Here is a PHP example of adding a cross-domain header that allows POST requests:
// 指定允许其他域名访问 header('Access-Control-Allow-Origin:*'); // 响应类型 header('Access-Control-Allow-Methods:POST'); // 响应头设置 header('Access-Control-Allow-Headers:x-requested-with,content-type');
Using dynamic scrpit tags, a method of dynamically creating a scrpit tag and pointing it to the requested address, also It is the JSONP method. You need to splice a callback function after the URL. The callback function will be called after the tag is loaded successfully.
var url = "http://uusama.com", callbaclName = 'jsonpCallback'; script = document.createElement('script'); script.type = 'text/javascript'; script.src = url + (url.indexOf('?') > -1 ? '&' : '?') + 'callback=' + callbaclName; document.body.appendChild(script);
The callback function needs to be set as a global function:
window['jsonpCallback'] = function jsonpCallback(ret) {}
Multiple ajax request data synchronization issues
Asynchronous processing of single ajax return data
Multiple ajax requests are not related to each other. They send their own requests after being called, and the request is successful. Calling your own callback methods in the future will not affect each other.
Because of the asynchronous nature of ajax requests, all operations that depend on the completion of the request need to be placed inside the callback function. Otherwise, the value you read outside the callback function will be empty. Look at the following example:
var result = null; ajax({ url: '/get.php?id=1', method: 'GET', success: function(ret) { result = ret; } }); console.log(result); // 输出 null
Although we set the result value in the callback function, the output of console.log(result); in the last line is empty.
Because the ajax request is asynchronous, when the program executes to the last line, the request is not completed and the value has not had time to be modified.
Here we should put console.log(result) related processing in the success callback function.
The problem of multiple ajax returning data
If there are multiple ajax requests, the situation will become a little complicated.
如果多个ajax请求是按照顺序执行的,其中一个完成之后,才能进行下一个,则可以把后面一个请求放在前一后请求的回调中。
比如有两个ajax请求,其中一个请求的数据依赖于另外一个,则可以在第一个请求的回调里面再进行ajax请求:
// 首先请求第一个ajax ajax({ url: '/get1.php?id=1', success: function(ret1) { // 第一个请求成功回调以后,再请求第二个 if (ret1) { ajax({ url: '/get2.php?id=4', success:function(ret2) { console.log(ret1); console.log(ret2) } }) } } }); // 也可以写成下面的形式 // 将第二个ajax请求定义为一个函数,然后调用 var ajax2 = function(ret1) { ajax({ url: '/get2.php?id=4', success:function(ret2) { console.log(ret1); console.log(ret2) } }); }; ajax({ url: '/get1.php?id=1', success: function(ret1) { if(ret1){ ajax2(ret1); // 调用第二个ajax请求 } } });
如果不关心不同的ajax请求的顺序,而只是关心所有请求都完成,才能进行下一步。
一种方法是可以在每个请求完成以后都调用同一个回调函数,只有次数减少到0才执行下一步。
var count = 3, all_ret = []; // 调用3次 ajax({ url: '/get1.php?id=1', success:function(ret) { callback(ret); // 请求成功后调用统一回调,次数减1 } }); ajax({ url: '/get2.php?id=1', success:function(ret) { callback(ret); } }); ajax({ url: '/get3.php?id=1', success:function(ret) { callback(ret); } }); function callback(ret) { // 当调用3次以上以后,说明3个ajax军完成 if (count > 0) { count--; // 每调用一次,次数减1 // 可以在这儿保存 ret 到全局变量 all_ret.push(ret); return; } else { // 调用三次以后 // todo console.log(ret); } }
另一种方法是设置一个定时任务去轮训是否所有ajax请求都完成,需要在每个ajax的成功回调中去设置一个标志。
这儿可以用是否获得值来判断,也可以设置标签来判断,用值来判断时,要注意设置的值和初始相同的情况。
var all_ret = { ret1: null, // 第一个ajax标识 ret2: null, // 第二个ajax标识 ret3: null, // 第三个ajax标识 }; ajax({ url: '/get1.php?id=1', success:function(ret) { all_ret['ret1'] = ret; // 修改第一个ajax请求标识 } }); ajax({ url: '/get2.php?id=1', success:function(ret) { all_ret['ret2'] = ret; // 修改第二个ajax请求标识 } }); ajax({ url: '/get3.php?id=1', success:function(ret) { all_ret['ret3'] = ret; // 修改第三个ajax请求标识 } }); var repeat = setInterval(function(){ // 遍历是否所有ajax请求标识都已被修改,以此判断是否所有ajax请求都已完成 for(var item in all_ret) { if (all_ret[item] === null){ return; } } // todo, 到这儿所有ajax请求均已完成 clearInterval(repeat); }, 50); // 调用次数可以适当调整,不应设的过小或者过大
相关推荐:
The above is the detailed content of Detailed explanation of Ajax asynchronous request examples using JavaScript. For more information, please follow other related articles on the PHP Chinese website!
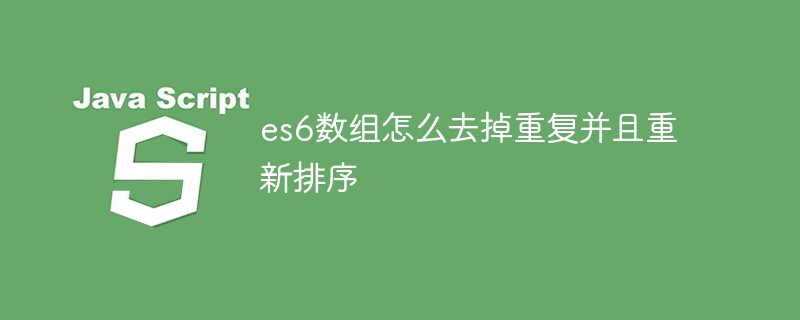
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
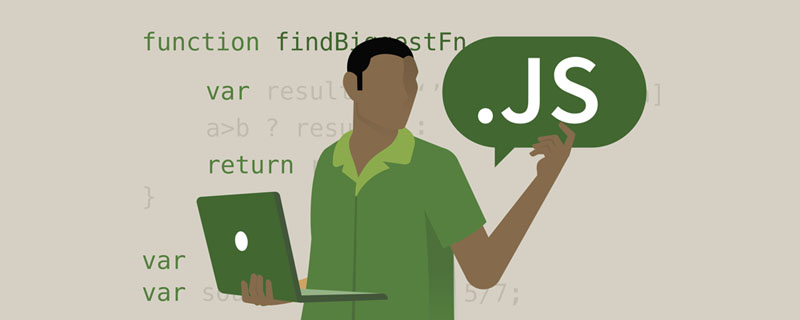
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
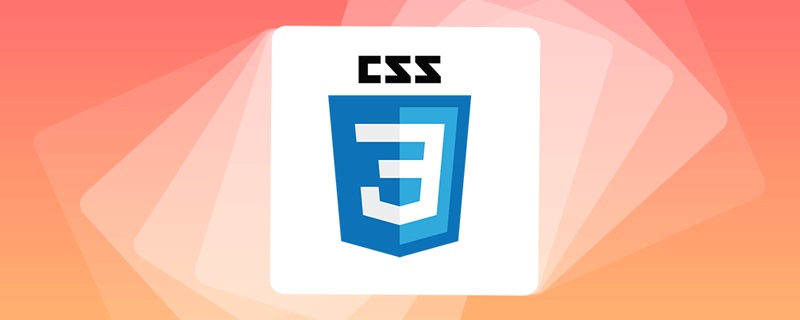
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
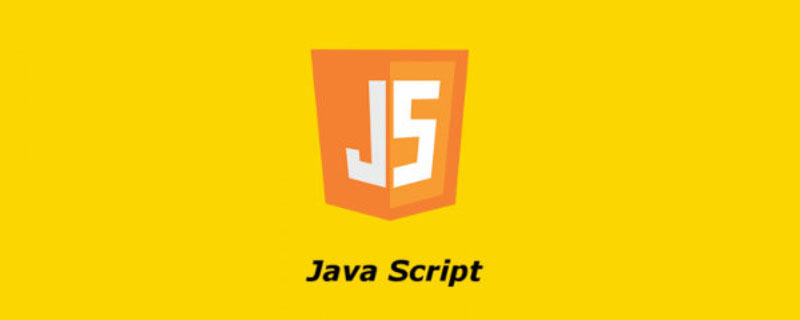
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
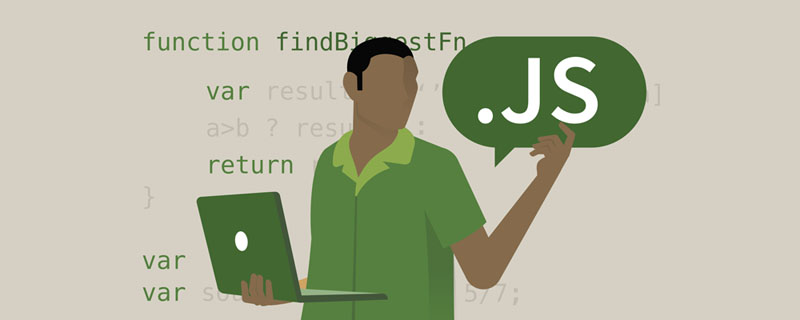
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
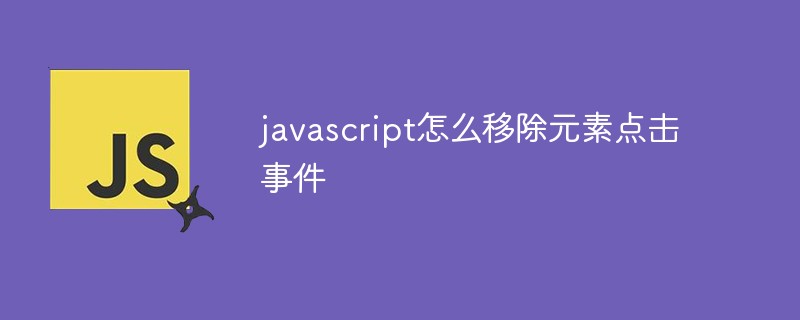
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
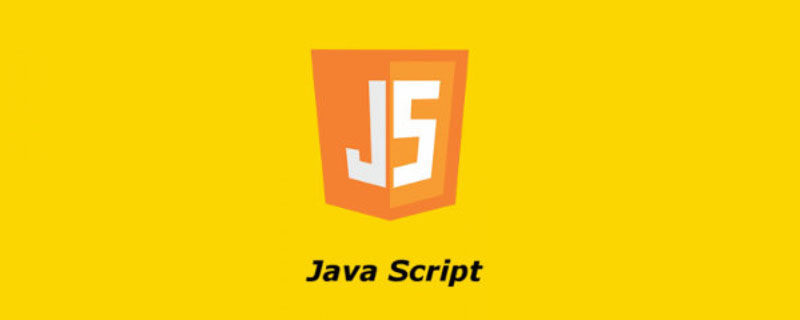
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
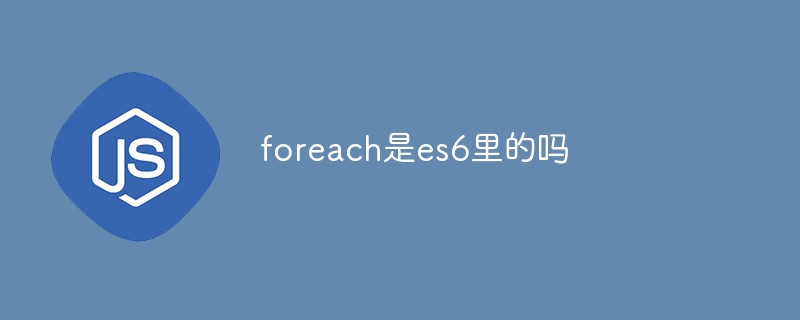
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
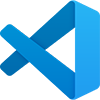
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment
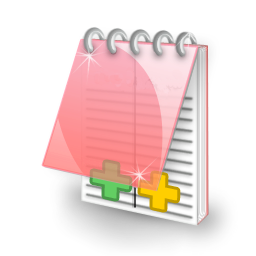
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
