


Example tutorial on mock.js random data and using express to output json interface
Front-end projects will all use the back-end interface, but when the back-end interface is not written well, you can use mock.js to randomly generate some fake data to debug the page. This article mainly introduces the implementation method of using mock.js random data and using express to output json interface. Friends in need can refer to it. I hope it can help everyone.
Install mock.js
First use express to create a nodejs web project. If the name is demo, I won’t go into it here
yarn add mockjs
Use
const Mock = require('mockjs') var data = Mock.mock({ 'list|2': [{ 'id|+1': 1, 'color': '@color()', 'date': '@datetime()', 'img': '@image()', 'url': '@url(http)', 'email': '@email()', 'name': "@name(1,2)", 'text': '@paragraph()' }] }) console.log(JSON.stringify(data))
The above random method can be found in the link to the mockjs document given at the bottom. The method called by Mock.Random can be used directly by adding @ in front. It is very convenient.
Output
{ "list": [ { "id": 1, "color": "#8179f2", "date": "2015-06-22 12:10:08", "img": "http://dummyimage.com/250x250", "url": "http://hwujcvh.fk/vfrjfmi", "email": "y.ahbatuekk@mbkhfybrh.pl", "name": "James Ronald Rodriguez", "text": "Zsogshtw qjscoe qwggnfk ppbdpqd avftd pvczrvnu gsyfyefm rbnbjyy tgemy buple ieghyjp klcxauofu lhjmnb kjpyodk. Njync ysrvx jevei stawy mcosrlpo iacryqob wkkcfuh nkrrdutr zduikjvtf dcv pppbhi ygjnkmg xvpusp ayu lvu. Wgqmtwvo ibqzp cct kodyh ovz slo cpc uqaseuwv ubjgbf hihh oudly mooztiojpi tubmwhsmb kktbkyqp hsvwgoluu jrkosqudm. Wpumdvtw riytwoa sbittrr xtjy beorkb osnjpigntu ndrgxhozeq iufhu hpuirgmh lstoijpqx hopk lkxceqhvr uymj pgdms njjmu ivxijmokn." }, { "id": 2, "color": "#94f279", "date": "1980-02-20 19:46:44", "img": "http://dummyimage.com/336x280", "url": "http://voyqj.cx/jjyksqzur", "email": "k.ydgui@gixl.cr", "name": "Ronald Nancy Harris", "text": "Lbdpwqwpgd sodipqu oncnnyis ebtwho dnbt fqxnjyzr qtrriop gfbjt prto dgmtgff gwaqnhon sdlvpxpj pqomfflsc skj. Cvteunoj oqmjnfm vowvmw ypywtr klcazkvg cvsyzayytl bgvywe kfqqzhfg iahm jwury xsgf xnr pvfxwhaed nauookwi xuxtgnwq flcbmnrm qglgziy vegml. Cexit vdotefuj nptmei hekmljnt bukxrd ndhj lkfyjcv oldpgo rrj kprfklt nfks yvrvc. Aynbyd hxguza ftjrn kmlirqo wxald jqjkvahim jnhezpgm usev qbynwhm yotehgkwdg eyxj vfnm icchnds dgmd odxajing vqrdl yhpp eba. Tykxnhe njod bslwbsjcj rwlv rkvxk rypew fpfqrqi psislxuwgs jcwrbtfn qeszy leovhc jwupwzo kitct nhbdhjq xyiajxms. Gfgkw nnlg drcqnpwn bowqknwy oiw yysaohk fqqsbgvp mulik vusikwv nbp kpbo nhti dhf hrgql." } ] }
Integrated into express to output json
const Mock = require('mockjs') exports.index = function(req, res) { var data = Mock.mock({ 'list|2': [{ 'id|+1': 1, 'color': '@color()', 'date': '@datetime()', 'img': '@image()', 'url': '@url(http)', 'email': '@email()', 'name': "@name(1,2)", 'text': '@paragraph()' }] }) // 延时1秒,模拟网络请求时间 setTimeout(function() { res.send(JSON.stringify(data)) }, 1000); }
express cross-domain
The interface address will definitely be different from the front-end project address. At this time, requesting the interface will involve cross-domain issues. , the solution in express is as follows
app.all('*', function(req, res, next) { res.header("Access-Control-Allow-Origin", "http://localhost:8080"); res.header("Access-Control-Allow-Headers", "X-Requested-With"); res.header("Access-Control-Allow-Methods","PUT,POST,GET,DELETE,OPTIONS"); res.header("X-Powered-By",' 3.2.1') res.header("Content-Type", "application/json;charset=utf-8"); res.header('Access-Control-Allow-Credentials', true); next(); });
Explanation: The above code was found online, but the sentence res.header('Access-Control-Allow-Credentials', true);## was not found online.
#The address of my front-end project is http://localhost:8080, so the value of Access-Control-Allow-Origin is http://localhost:8080It can be modified according to your own server. Related recommendations:Express and mockjs realize the simulation background data sending function example sharing
Detailed explanation of using nodejs+express to implement simple files Upload function
Detailed explanation of node.js using websocket based on express
The above is the detailed content of Example tutorial on mock.js random data and using express to output json interface. For more information, please follow other related articles on the PHP Chinese website!
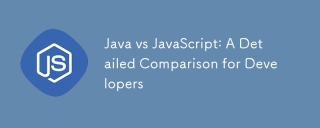
JavaandJavaScriptaredistinctlanguages:Javaisusedforenterpriseandmobileapps,whileJavaScriptisforinteractivewebpages.1)Javaiscompiled,staticallytyped,andrunsonJVM.2)JavaScriptisinterpreted,dynamicallytyped,andrunsinbrowsersorNode.js.3)JavausesOOPwithcl
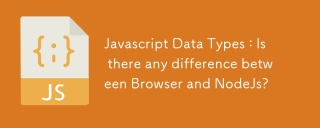
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
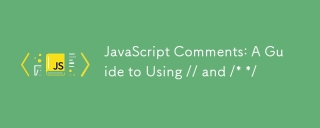
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
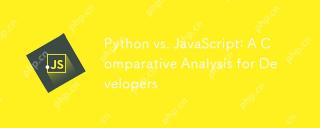
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
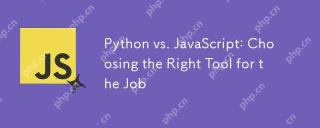
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
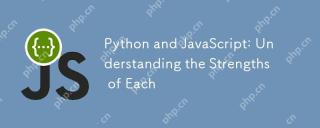
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
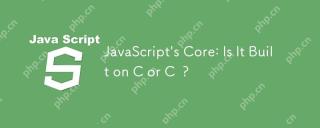
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
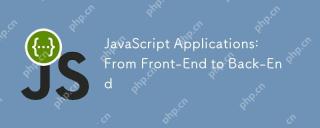
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
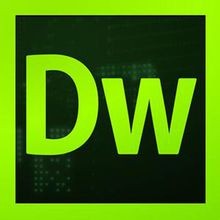
Dreamweaver CS6
Visual web development tools
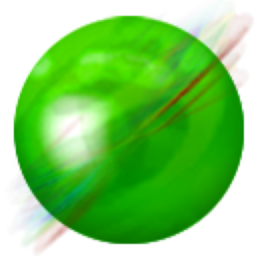
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
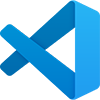
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
