


Detailed example of how Angular implements recursive display of blog comments and obtains data for reply comments
This article mainly introduces to you the relevant information on how Angular implements recursive display similar to blog comments and obtains data for replying to comments. The article introduces it in detail through sample code, which has certain reference learning value for everyone's study or work. , friends who need it, please follow the editor to learn together.
Preface
We often see a lot of recursive comments in some technology blogs, that is, we can reply to bloggers’ comments, and the interface is very beautiful and has a gradient display format. Recently, I write similar demos in my spare time, so recording them can provide some reference for those who need them.
Okay, stop talking nonsense and get to the point. . .
Thinking
When we write background programs, we often encounter data structures that generate tree-like data. Our intuition is to use recursive methods to achieve it. At first, I thought so too. , that is, write a recursive method in Angular4 to form a string, and then display it on the interface, similar to the code below
@Component({ selector: "comment", template: '{{ comments }}' }) export class CommentComponent { public comments: string = ""; generateComment(Comment comment) { this.comments = this.comments + "<p>" + comment.content + "</p>"; if (comment.pComment != null) { generateComment(comment.pComment); } } }
I naively thought it was ok, but when I tried it, the tag would not be parsed, and then I remembered that it had been passed Understand the process of parsing tags. . .
Later I thought about it, the current front-end frameworks all claim to be componentized, and Angular4 is no exception. So if a Component can be embedded in any Component, it can definitely embed itself, and there is a concept similar to recursion. Try it immediately as soon as you think of it. . .
Specific implementation
The idea is like this. I defined the format of the data. There is an array of child comments under each comment, instead of each comment having a parent comment. The data format is as follows :
"comments": [ { "id": 1, "username": "James1", "time": "2017-07-09 21:02:21", "content": "哈哈哈1<h1 id="哈哈哈">哈哈哈</h1>", "status": 1, "email": "1xxxx@xx.com", "cComments": [ { "id": 2, "username": "James2", "time": "2017-07-09 21:02:22", "content": "哈哈哈2", "status": 1, "email": "2xxxx@xx.com", "cComments": null } ] } ]
CommentComponent component implements the comment module, but recursive comments are not implemented in this component, but in the sub-component CommentViewComponent, because CommentComponent also includes a text box for entering comments one by one.
Comment total module ComponentComponent code:
comment.component.ts
@Component({ selector: 'comment', templateUrl: './comment.component.html', styleUrls: ['./comment.component.css'] }) export class CommentComponent implements OnInit { @Input() public comments: Comment[]; ngOnInit(): void { } }
comment.component.html
<p> </p><p> </p><p> <comment-view></comment-view> </p><p> </p><h4 id="comment-leaveComment-translate">{{ 'comment.leaveComment' | translate }}</h4>
comment.component.css
.media { font-size: 14px; } .media-object { padding-left: 10px; }
Submodule ComponentViewComponent code:
component-view.component.ts
@Component({ selector: 'comment-view', templateUrl: './comment-view.component.html', styleUrls: ['./comment-view.component.css'] }) export class CommentViewComponent implements OnInit { @Input() public comments: Comment[]; constructor(private router: Router, private activateRoute: ActivatedRoute ) { } ngOnInit(): void { } }
component-view.component.html
<p> </p><p> </p><p> <span></span> </p> <p> </p><h4>{{ comment.username }} <small>{{ comment.time }} | <a>{{ 'comment.reply' | translate }}</a></small> </h4> {{ comment.content }} <hr> <comment-view></comment-view>
comonent-view.component.css
.media { font-size: 14px; } .media-object { padding-left: 10px; }
Result
The display result at this time is as shown below:
The above only explains how to realize the comment ladder display. In blog comments, we often see that we can reply to a certain comment. This article describes how to obtain the content of the comment and display it in the input box after clicking the reply button of a certain comment. Similar to CSDN blog comments, after clicking reply, the input box is automatically added with [reply]u011642663[/reply]
IDEA
According to the ladder display of comments in the previous article, we still need to implement After clicking to reply, the screen automatically reaches the input box position and the information of the comment clicked to reply is obtained. First, let’s break down this function point. In the project, we will often break down the function point. This function point has two small points: First, add a [reply] button to each comment, and click the reply to jump to the input box; Second, after clicking to reply, the information of the comment that was clicked to reply is obtained. Let’s solve them one by one below.
Jump to the input box
The first language we came into contact with in the previous section is HTML. We know that there is a # positioning in HTML. The following code is briefly explained.
Assume that this HTML code file is index.html
<a>Click me to pointer</a> <p> </p><h1 id="哈哈哈哈">哈哈哈哈</h1>
As long as you click the link Click me to pointer in the above code, the page will jump to the position of p with id="pointer" . So we can use this method when implementing this click reply to jump to the input box.
We add id="comment" to the comment input box in comment-component.html. The next step is the problem of path splicing. We can get the URL of this page through the url of Angular's Router. path, and then add #comment after the path to achieve the jump. The following is the code to implement this jump function
Add id=”comment”
comment-component.html
<p> </p><p> </p><p> <comment-view></comment-view> </p><p> </p><h4 id="comment-leaveComment-translate">{{ 'comment.leaveComment' | translate }}</h4>
Add to get the current page URL through routing
comment-view.component.ts
@Component({ selector: 'comment-view', templateUrl: './comment-view.component.html', styleUrls: ['./comment-view.component.css'] }) export class CommentViewComponent implements OnInit { @Input() public comments: Comment[]; // 用于跳转到回复输入框的url拼接 public url: string = ""; constructor(private router: Router, private activateRoute: ActivatedRoute ) { } ngOnInit(): void { this.url = this.router.url; this.url = this.url.split("#")[0]; this.url = this.url + "#comment"; } }
Add link href=""
comment-view.component .html
<p> </p><p> </p><p> <span></span> </p> <p> </p><h4>{{ comment.username }} <small>{{ comment.time }} | <a>{{ 'comment.reply' | translate }}</a></small> </h4> {{ comment.content }} <hr> <comment-view></comment-view>
This realizes the function point of page jump, and then realizes obtaining the information of the reply comment.
Get reply comment information
有人会说获取回复的评论信息,这不简单么?加个 click 事件不就行了。还记得上一篇文章咱们是如何实现梯形展示评论的么?咱们是通过递归来实现的,怎么添加 click 事件让一个不知道嵌了多少层的组件能够把评论信息传给父组件?首先不具体想怎么实现,我们这个思路是不是对的:把子组件的信息传给父组件?答案是肯定的,我们就是要把不管嵌了多少层的子组件的信息传给 comment.component.ts 这个评论模块的主组件。
Angular 提供了 @Output 来实现子组件向父组件传递信息,我们在 comment-view.component.ts 模块中添加 @Output 向每个调用它的父组件传信息,我们是嵌套的,这样一层一层传出来,直到传给 comment-component.ts 组件。我们看代码怎么实现。
实现代码
comment-view.component.ts
@Component({ selector: 'comment-view', templateUrl: './comment-view.component.html', styleUrls: ['./comment-view.component.css'] }) export class CommentViewComponent implements OnInit { @Input() public comments: Comment[]; // 点击回复时返回数据 @Output() public contentEvent: EventEmitter<comment> = new EventEmitter<comment>(); // 用于跳转到回复输入框的url拼接 public url: string = ""; constructor(private router: Router, private activateRoute: ActivatedRoute ) { } ngOnInit(): void { this.url = this.router.url; this.url = this.url.split("#")[0]; this.url = this.url + "#comment"; } reply(comment: Comment) { this.contentEvent.emit(comment); } transferToParent(event) { this.contentEvent.emit(event); } }</comment></comment>
comment-view.component.html
<p> </p><p> </p><p> <span></span> </p> <p> </p><h4>{{ comment.username }} <small>{{ comment.time }} | <a>{{ 'comment.reply' | translate }}</a></small> </h4> {{ comment.content }} <hr> <comment-view></comment-view>
comment.component.ts
@Component({ selector: 'comment', templateUrl: './comment.component.html', styleUrls: ['./comment.component.css'] }) export class CommentComponent implements OnInit { @Input() public comments: Comment[]; // 要回复的评论 public replyComment: Comment = new Comment(); public id: number = 0; public content: string = ""; ngOnInit(): void { } getReplyComment(event) { this.replyComment = event; this.id = this.replyComment.id; this.content = "[reply]" + this.replyComment.username + "[reply]\n"; } }
comment.component.html
<p> </p><p> </p><p> <comment-view></comment-view> </p><p> </p><h4 id="comment-leaveComment-translate">{{ 'comment.leaveComment' | translate }}</h4>
解释一下代码逻辑:
我们在 comment-view.component.ts 添加以下几点:
定义了@Output() contentEvent
添加了reply(comment: Comment) 事件在点击回复的时候触发的,触发的时候 contentEvent 将 comment 传到父模块
添加 transferToParent(event) 是接受子组件传来的 event, 并且继续将 event 传到父组件
在 comment.component.ts 中定义了 getReplyComment(event) 方法,该方法接收子组件传递来的评论信息,并将信息显示在页面上。大功告成。。。
效果图
相关推荐:
如何PHP制作简易博客
The above is the detailed content of Detailed example of how Angular implements recursive display of blog comments and obtains data for reply comments. For more information, please follow other related articles on the PHP Chinese website!
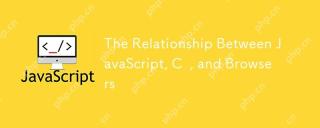
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
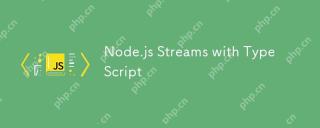
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
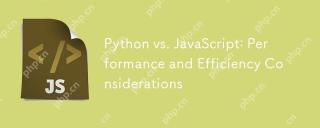
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
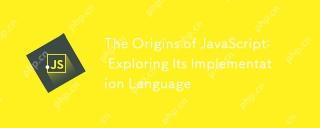
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
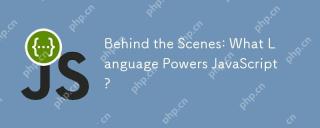
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
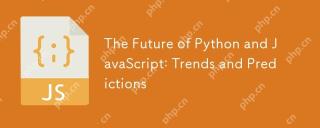
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
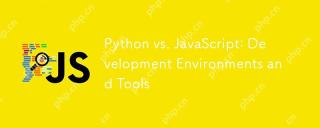
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
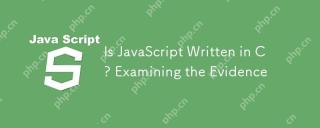
Yes, the engine core of JavaScript is written in C. 1) The C language provides efficient performance and underlying control, which is suitable for the development of JavaScript engine. 2) Taking the V8 engine as an example, its core is written in C, combining the efficiency and object-oriented characteristics of C. 3) The working principle of the JavaScript engine includes parsing, compiling and execution, and the C language plays a key role in these processes.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
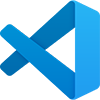
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
