If you want to determine what the rules are in this, the method is actually very simple. By checking its calling position, determine this when the function is called. Let’s follow the editor of Script House to learn through this article
Several rules determine what this is in a function.
It’s actually very simple to determine what this is. The general rule is to determine this when a function is called by checking where it was called. It follows these rules, described next in order of priority.
Rules
1. If you use the new keyword when calling a function, then this in the function is a brand new object.
function ConstructorExample() { console.log(this); this.value = 10; console.log(this); } new ConstructorExample(); // -> {} // -> { value: 10 }
2. If you use apply, call or bind to call a function, then this in the function is the object passed in as a parameter.
function fn() { console.log(this); } var obj = { value: 5 }; var boundFn = fn.bind(obj); boundFn(); // -> { value: 5 } fn.call(obj); // -> { value: 5 } fn.apply(obj); // -> { value: 5 }
3. If the function is called as a method, that is, if the function is called using dot notation, then this is the object that has this function as an attribute. In other words, when a point is to the left of a function call, this is the object to the left of the point.
var obj = { value: 5, printThis: function() { console.log(this); } }; obj.printThis(); // -> { value: 5, printThis: ƒ }
4. If the function is called as a pure function, that is, it is called without any of the above conditions, then this is the global object. In a browser, this is the window object.
function fn() { console.log(this); } // 如果在浏览器里调用: fn(); // -> Window {stop: ƒ, open: ƒ, alert: ƒ, ...}
Note that this rule is actually the same as the third rule. The difference is that functions not declared as methods will automatically become properties of the global object window. Therefore, this is actually an implicit method call. When we call fn(), it will actually be understood by the browser as window.fn(), so this is window.
console.log(fn === window.fn); // -> true
5. If multiple rules above apply, the one with higher priority will set this value.
6. If it is an arrow function in ES2015, it will ignore all the above rules and receive the scope containing it as the value of this when it is created. To determine what this is, just go up one line from where you created the arrow function and see what this is there. The value of this in an arrow function is the same.
const obj = { value: 'abc', createArrowFn: function() { return () => console.log(this); } }; const arrowFn = obj.createArrowFn(); arrowFn(); // -> { value: 'abc', createArrowFn: ƒ }
Looking back at the third rule, when we call obj.createArrowFn(), this in createArrowFn is obj, because this is a method call. Therefore, obj will be bound to this in arrowFn. If we create an arrow function in the global scope, then the this value will be window.
Applying the Rules
Let’s look at a code example and apply these rules. Try it and see if you can figure out what this is in different function calls.
Determine which rule is applied
var obj = { value: 'hi', printThis: function() { console.log(this); } }; var print = obj.printThis; obj.printThis(); // -> {value: "hi", printThis: ƒ} print(); // -> Window {stop: ƒ, open: ƒ, alert: ƒ, ...}
obj.printThis() belongs to the third rule, method call. On the other hand, print() falls under the fourth rule, a pure function call. For print(), we did not use new, bind/call/apply or dot notation when calling, so it corresponds to rule 4, this is the global object window.
When multiple rules are applied
When multiple rules are applied, the rule with higher priority in the list is used.
var obj1 = { value: 'hi', print: function() { console.log(this); }, }; var obj2 = { value: 17 };
If Rule 2 and Rule 3 apply at the same time, Rule 2 prevails.
obj1.print.call(obj2); // -> { value: 17 }
If Rule 1 and Rule 3 apply at the same time, Rule 1 prevails.
new obj1.print(); // -> {}
Library
Some libraries sometimes intentionally bind this value to certain functions inside. Usually the most useful value is bound to this in a function. For example, jQuery binds this to a DOM element and triggers an event in a callback. If a library has a this value that does not conform to the above rules, please read the documentation of the library carefully. It is likely to be bound using bind.
Summarize
The above is the detailed content of A brief introduction to this rule in JavaScript. For more information, please follow other related articles on the PHP Chinese website!
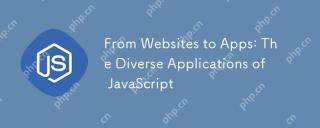
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
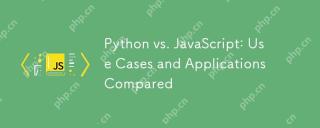
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
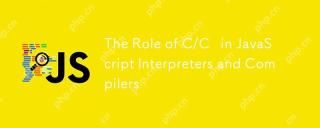
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
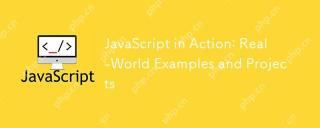
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
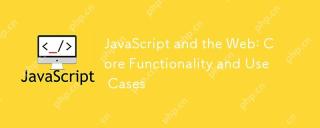
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
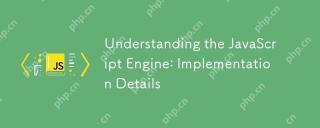
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
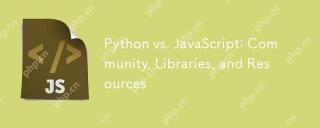
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
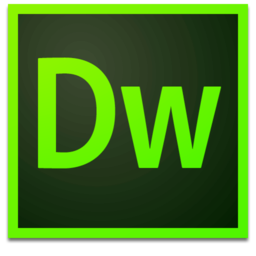
Dreamweaver Mac version
Visual web development tools
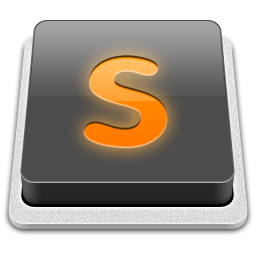
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
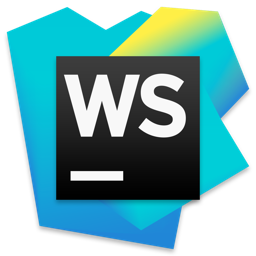
WebStorm Mac version
Useful JavaScript development tools