Java uses three methods to implement high-concurrency lock sample code
This article mainly introduces the three implementation example codes of Java high concurrency lock. The editor thinks it is quite good, so I will share it with you now and give it as a reference. Let’s follow the editor and take a look.
Basic skills - optimistic lock
Optimistic lock is suitable for such a scenario: there will be no conflict in reading, but there will be conflict in writing. The frequency of simultaneous reads is much greater than that of writes.
Take the following code as an example, the implementation of pessimistic locking:
public Object get(Object key) { synchronized(map) { if(map.get(key) == null) { // set some values } return map.get(key); } }
The implementation of optimistic locking:
public Object get(Object key) { Object val = null; if((val = map.get(key) == null) { // 当map取值为null时再加锁判断 synchronized(map) { if(val = map.get(key) == null) { // set some value to map... } } } return map.get(key); }
Intermediate skill - String.intern()
Optimistic locking cannot solve a large number of write conflicts very well, but in many scenarios, the lock is actually only for a certain user Or an order. For example, a user must create a session before performing subsequent operations. However, due to network reasons, the request to create a user session and subsequent requests arrive almost at the same time, and parallel threads may process subsequent requests first. In general, the user sessionMap needs to be locked, such as the optimistic lock above. In this scenario, the lock can be limited to the user itself, that is, changed from the original
lock.lock(); int num=storage.get(key); storage.set(key,num+1); lock.unlock();
to:
lock.lock(key); int num=storage.get(key); storage.set(key,num+1); lock.unlock(key);
This is similar to the concepts of database table locks and row locks. Obviously, the concurrency capability of row locks is much higher than that of table locks.
Using String.inter() is a specific implementation of this idea. Class String maintains a pool of strings. When the intern method is called, if the pool already contains a string equal to this String object (as determined by the equals(Object) method), the string in the pool is returned. It can be seen that when the Strings are the same, String.intern() always returns the same object, so locking the same user is achieved. Since the granularity of the lock is limited to specific users, the system achieves maximum concurrency.
public void doSomeThing(String uid) { synchronized(uid.intern()) { // ... } }
CopyOnWriteMap?
Now that we have talked about "a concept similar to row locks in a database", we have to mention MVCC. The CopyOnWrite class in Java implements MVCC. Copy On Write is such a mechanism. When we read shared data, we read it directly without synchronization. When we modify the data, we copy a copy of the current data, and then modify it on this copy. After completion, we use the modified copy to replace the original data. This method is called Copy On Write.
But,,, JDK does not provide CopyOnWriteMap, why? There is a good answer below, that is, we already have ConcurrentHashMap, why do we need CopyOnWriteMap?
Fredrik Bromee wrote
I guess this depends on your use case, but why would you need a CopyOnWriteMap when you already have a ConcurrentHashMap?
For a plain lookup table with many readers and only one or few updates it is a good fit.
Compared to a copy on write collection:
Read concurrency:
Equal to a copy on write collection. Several readers can retrieve elements from the map concurrently in a lock-free fashion.
Write concurrency:
Better concurrency than the copy on write collections that basically serialize updates (one update at a time). Using a concurrent hash map you have a good chance of doing several updates concurrently. If your hash keys are evenly distributed.
If you do want to have the effect of a copy on write map, you can always initialize a ConcurrentHashMap with a concurrency level of 1.
Advanced Tips - Class ConcurrentHashMap
The flaw of String.inter() is that the String class maintains a string pool. Placed in the JVM perm area, if the number of users is particularly large, the String placed in the string pool will be uncontrollable, which may lead to OOM errors or excessive Full GC. How can we control the number of locks and reduce the granularity of locks at the same time? Use Java ConcurrentHashMap directly? Or do you want to add your own more granular control? Then you can learn from ConcurrentHashMap, divide the objects that need to be locked into multiple buckets, and add a lock to each bucket. The pseudo code is as follows:
Map locks = new Map(); List lockKeys = new List(); for(int number : 1 - 10000) { Object lockKey = new Object(); lockKeys.add(lockKey); locks.put(lockKey, new Object()); } public void doSomeThing(String uid) { Object lockKey = lockKeys.get(uid.hash() % lockKeys.size()); Object lock = locks.get(lockKey); synchronized(lock) { // do something } }
The above is the detailed content of Java uses three methods to implement high-concurrency lock sample code. For more information, please follow other related articles on the PHP Chinese website!
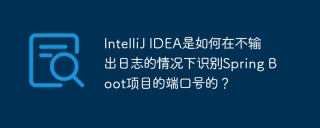
Start Spring using IntelliJIDEAUltimate version...
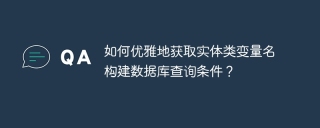
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
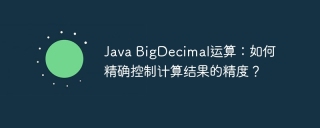
Java...
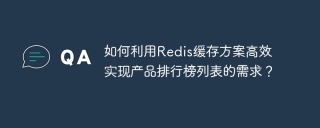
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
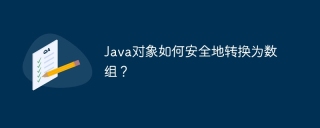
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
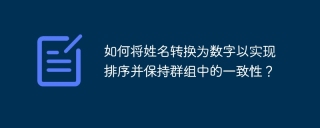
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
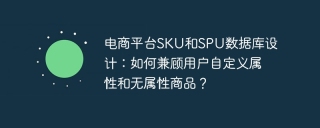
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
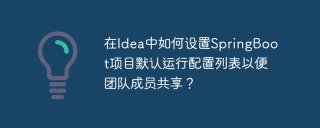
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
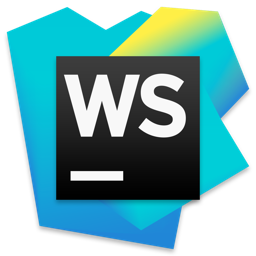
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
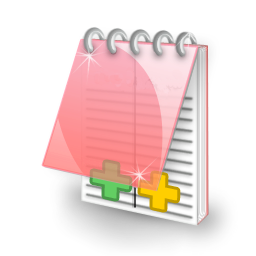
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software