


Detailed explanation of why traversal method is preferred over loop code in JavaScript
Prefer traversal methods rather than loops
When using loops, it is easy to violate the DRY (Don't Repeat Yourself) principle. This is because we usually choose the copy-paste method to avoid hand-writing paragraphs of circular statements. But doing so will result in a lot of duplicate code in the code, and developers will "reinvent the wheel" meaninglessly. More importantly, it is easy to overlook the details in the loop when copying and pasting, such as the starting index value, termination condition, etc.
For example, the following for loop has this problem. Assume n is the length of the collection object:
for (var i = 0; i <= n; i++) { ... } // 终止条件错误,应该是i < n for (var i = 1; i < n; i++) { ... } // 起始变量错误,应该是i = 0 for (var i = n; i >= 0; i--) { ... } // 起始变量错误,应该是i = n - 1 for (var i = n - 1; i > 0; i--) { ... } // 终止条件错误,应该是i >= 0
It can be seen that it is easy to deal with some details of the loop. Something went wrong. Using the closure provided by JavaScript (see Item 11), the details of the loop can be encapsulated for reuse. In fact, ES5 provides some methods to deal with this problem. Among them, Array.prototype.forEach is the simplest one. Using it, we can write the loop like this:
// 使用for循环 for (var i = 0, n = players.length; i < n; i++) { players[i].score++; } // 使用forEach players.forEach(function(p) { p.score++; });
In addition to traversing the collection object, another common pattern is to traverse each element in the original collection To perform some operation and then get a new collection, we can also use the forEach method to implement the following:
// 使用for循环 var trimmed = []; for (var i = 0, n = input.length; i < n; i++) { trimmed.push(input[i].trim()); } // 使用forEach var trimmed = []; input.forEach(function(s) { trimmed.push(s.trim()); });
But due to this method, one collection is converted into another collection The pattern is very common. ES5 also provides the Array.prototype.map method to make the code simpler and more elegant:
var trimmed = input.map(function(s) { return s.trim(); });
In addition, there is another common pattern that is to set Filter according to certain conditions, and then obtain a subset of the original collection. Array.prototype.filter is provided in ES5 to implement this mode. This method accepts a Predicate as a parameter, which is a function that returns true or false: returning true means that the element will be retained in the new collection; returning false means that the element will not appear in the new collection. For example, we use the following code to filter product prices and only retain products with prices in the [min, max] range:
##
listings.filter(function(listing) { return listing.price >= min && listing.price <= max; });Of course, the above method is Available in environments that support ES5. In other environments, we have two options: 1. Use third-party libraries, such as underscore or lodash, which provide quite a few common methods to operate objects and collections. 2. Define it as needed. For example, define the following method to obtain the previous elements in the set based on a certain condition:
function takeWhile(a, pred) { var result = []; for (var i = 0, n = a.length; i < n; i++) { if (!pred(a[i], i)) { break; } result[i] = a[i]; } return result; } var prefix = takeWhile([1, 2, 4, 8, 16, 32], function(n) { return n < 10; }); // [1, 2, 4, 8]In order to better reuse this method, we It can be defined on the Array.prototype object. For specific effects, please refer to Item 42.
Array.prototype.takeWhile = function(pred) { var result = []; for (var i = 0, n = this.length; i < n; i++) { if (!pred(this[i], i)) { break; } result[i] = this[i]; } return result; }; var prefix = [1, 2, 4, 8, 16, 32].takeWhile(function(n) { return n < 10; }); // [1, 2, 4, 8]There is only one situation where using a loop is better than using a traversal function: when you need to use break and continue. For example, there will be problems when using forEach to implement the takeWhile method above. How should it be implemented when the predicate is not satisfied?
function takeWhile(a, pred) { var result = []; a.forEach(function(x, i) { if (!pred(x)) { // ? } result[i] = x; }); return result; }We can use an internal exception to make the judgment, but it is also a bit clumsy and inefficient:
function takeWhile(a, pred) { var result = []; var earlyExit = {}; // unique value signaling loop break try { a.forEach(function(x, i) { if (!pred(x)) { throw earlyExit; } result[i] = x; }); } catch (e) { if (e !== earlyExit) { // only catch earlyExit throw e; } } return result; }But after using forEach, the code is even more verbose than before using it. This is obviously problematic. For this problem, ES5 provides some and every methods to handle loops that terminate prematurely. Their usage is as follows:
[1, 10, 100].some(function(x) { return x > 5; }); // true [1, 10, 100].some(function(x) { return x < 0; }); // false [1, 2, 3, 4, 5].every(function(x) { return x > 0; }); // true [1, 2, 3, 4, 5].every(function(x) { return x < 3; }); // falseThese two methods are short-circuit Method (Short-circuiting): As long as any element returns true in the predicate of the some method, then some will return; as long as any element returns false in the predicate of the every method, then the every method will also return false. Therefore, takeWhile can be implemented as follows:
function takeWhile(a, pred) { var result = []; a.every(function(x, i) { if (!pred(x)) { return false; // break } result[i] = x; return true; // continue }); return result; }In fact, this is the idea of functional programming. In functional programming, you rarely see explicit for loops or while loops. The details of the loops are nicely encapsulated.
The above is the detailed content of Detailed explanation of why traversal method is preferred over loop code in JavaScript. For more information, please follow other related articles on the PHP Chinese website!
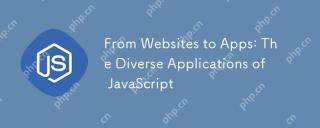
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
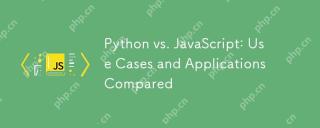
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
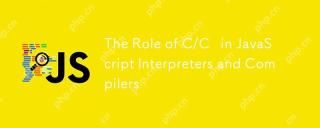
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
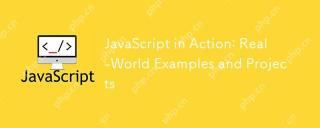
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
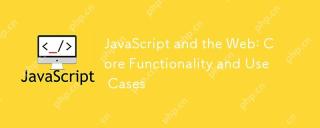
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
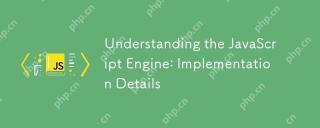
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
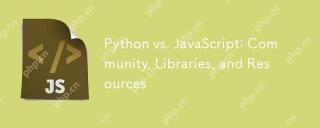
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
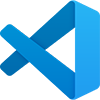
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
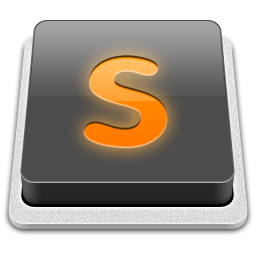
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
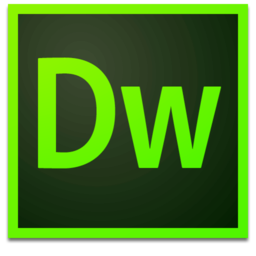
Dreamweaver Mac version
Visual web development tools