Example of how Java generates and decodes QR codes based on google zxing
This article mainly introduces Java QR code generation and decoding based on google zxing in detail. It has certain reference value. Interested friends can refer to it.
The examples in this article are shared with everyone. The specific code for Java QR code generation and decoding is provided for your reference. The specific content is as follows
1. Add Maven dependency (you need to upload the QR code image when decoding, so you need to rely on the file upload package)
<!-- google二维码工具 --> <dependency> <groupId>com.google.zxing</groupId> <artifactId>javase</artifactId> <version>3.1.0</version> </dependency> <!-- 文件上传 --> <dependency> <groupId>commons-fileupload</groupId> <artifactId>commons-fileupload</artifactId> <version>1.3.1</version> </dependency> <dependency> <groupId>commons-io</groupId> <artifactId>commons-io</artifactId> <version>2.4</version> </dependency>
2. Create a QR code generation interface (the interface parameter is the QR code content, usually a URL)
/** * 生成二维码 * google zxing 实现 * @param text * @return */ @RequestMapping(value = "/qrcode/encode", method = RequestMethod.POST) public void encodeQrCode(String text, HttpServletResponse response) { try { // 设置二维码参数 Map<EncodeHintType, Object> hints = new HashMap<EncodeHintType, Object>(); hints.put(EncodeHintType.CHARACTER_SET, "UTF-8"); BitMatrix bitMatrix = new MultiFormatWriter().encode(text, BarcodeFormat.QR_CODE, 150, 150, hints); //返回二维码 MatrixToImageWriter.writeToStream(bitMatrix, "jpg", response.getOutputStream()); } catch (Exception e) { e.printStackTrace(); } }
3. Create a QR code decoding interface (directly returns the decoded QR code content)
/** * 二维码图片解码 * google zxing 实现 * @param qrImg * @return */ @RequestMapping(value = "/qrcode/decode", method = RequestMethod.POST) public String decodeQrCode(MultipartFile qrImg) { if (!qrImg.isEmpty()) { try { BufferedImage image = ImageIO.read(qrImg.getInputStream()); BinaryBitmap binaryBitmap = new BinaryBitmap(new HybridBinarizer(new BufferedImageLuminanceSource(image))); // 定义二维码的参数: HashMap<DecodeHintType, Object> hints = new HashMap<>(); // 定义字符集 hints.put(DecodeHintType.CHARACTER_SET, "utf-8"); Result result = new MultiFormatReader().decode(binaryBitmap, hints); return result.getText(); } catch (Exception e) { e.printStackTrace(); } } return null; }
The above is the detailed content of Example of how Java generates and decodes QR codes based on google zxing. For more information, please follow other related articles on the PHP Chinese website!
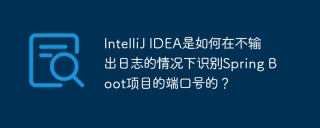
Start Spring using IntelliJIDEAUltimate version...
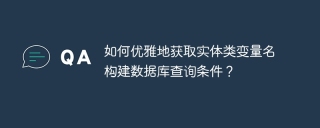
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
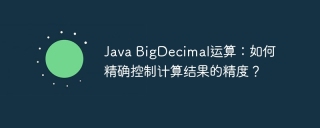
Java...
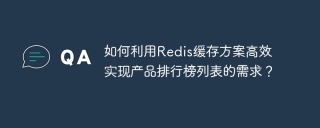
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
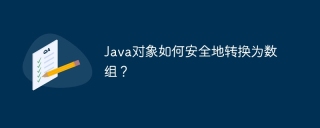
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
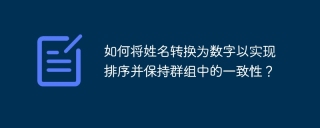
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
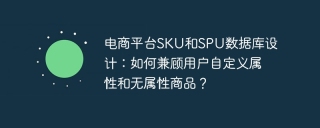
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
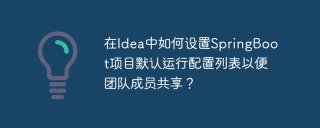
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Atom editor mac version download
The most popular open source editor