


Share an encapsulated javascript event queue function code to solve the problem of binding events
There are several minor problems when using addEventListener() or attachEvent() to bind events in JavaScript:
1. Anonymous functions added using addEventListener() or attachEvent() Cannot be removed.
var oBtn = document.getElementById('btn'); oBtn.addEventListener('click',function(){ alert('button is clicked') },false) oBtn.reomveEventListener('click',function(){ alert('button is clicked') },false) //oBtn上的事件无法移除,因为传入的是一个匿名函数
2. In ie6-ie8, the reverse order execution problem of using attachEvent() to bind multiple events.
var oBtn = document.getElementById('btn'); oBtn.attachEvent('onclick',function(){ alert(1) }) oBtn.attachEvent('onclick',function(){ alert(2) }) oBtn.attachEvent('onclick',function(){ alert(3) }) //ie9+ 下执行顺序1、2、3 //ie6-ie8下执行顺序3、2、1
Solve the problem
I want to write a cross-browser event binding module so that it can be reused in the future. At the same time, I want to solve the appeal problem. JQuery internally uses event queues and data caching mechanisms to solve this problem. I looked at the relevant source code and found that it is really complicated. I tried some methods myself and managed to achieve it. The code snippet posted was originally written in an object-oriented way. I didn't want it to be complicated, so I changed it to functions.
/*绑定事件的接口 * *@param {dom-DOM}和{type-string}和{fn-function} 可选参数{fnName-string} *@execute 创建事件队列,添加到DOM对象属性上, 将事件处理程序(函数)加入事件队列 可为事件处理程序添加一个标识符,用于删除指定事件处理程序 */ function bind(dom,type,fn,fnName){ dom.eventQueue = dom.eventQueue || {}; dom.eventQueue[type] = dom.eventQueue[type] || {}; dom.handler = dom.handler || {}; if (!fnName) { var index = queueLength(dom,type); dom.eventQueue[type]['fnQueue'+index] = fn; } else { dom.eventQueue[type][fnName] = fn; }; if (!dom.handler[type]) bindEvent(dom,type); }; /*绑定事件 * *@param {dom-DOM}和{type-string} *@execute 只绑定一次事件,handler用于遍历执行事件队列中的事件处理程序(函数) *@caller bind() */ function bindEvent(dom,type){ dom.handler[type] = function(){ for(var guid in dom.eventQueue[type]){ dom.eventQueue[type][guid].call(dom); } }; if (window.addEventListener) { dom.addEventListener(type,dom.handler[type],false); } else { dom.attachEvent('on'+type,dom.handler[type]); }; }; /*移除事件的接口 * *@param {dom-DOM}和{type-string} 可选参数{fnName-function} *@execute 如果没有标识符,则执行unBindEvent() 如果有标识符,则删除指定事件处理程序,如果事件队列长度为0,执行unBindEvent() */ function unBind(dom,type,fnName){ var hasQueue = dom.eventQueue && dom.eventQueue[type]; if (!hasQueue) return; if (!fnName) { unBindEvent(dom,type) } else { delete dom.eventQueue[type][fnName]; if (queueLength(dom,type) == 0) unBindEvent(dom,type); }; }; /*移除事件 * *@param {dom-DOM}和{type-string} *@execute 移除绑定的事件处理程序handler,并清空事件队列 *@caller unBind() */ function unBindEvent(dom,type){ if (window.removeEventListener) { dom.removeEventListener(type,dom.handler[type]) } else { dom.detachEvent(type,dom.handler[type]) } delete dom.eventQueue[type]; }; /*判断事件队列长度 * *@param {dom-DOM}和{type-string} *@caller bind() unBind() */ function queueLength(dom,type){ var index = 0; for (var length in dom.eventQueue[type]){ index++ ; } return index; };
How to use
var oBtn = document.getElementById('btn'); //绑定事件 //为button同时绑定三个click事件函数 //ie6-ie8下执行顺序不变 bind(oBtn,'click',function(){ alert(1); }) bind(oBtn,'click',function(){ alert(2); },'myFn') bind(oBtn,'click',function(){ alert(3); }) //移除事件 //移除所有绑定的click事件函数,支持移除匿名函数 unBind(oBtn,'click') //只移除标识符为myfn的事件函数 unBind(oBtn,'click','myFn')
The above is the detailed content of Share an encapsulated javascript event queue function code to solve the problem of binding events. For more information, please follow other related articles on the PHP Chinese website!
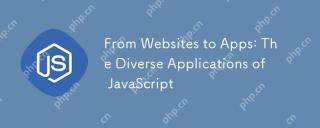
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
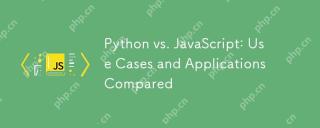
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
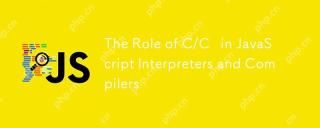
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
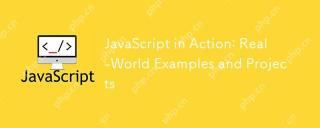
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
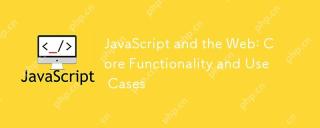
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
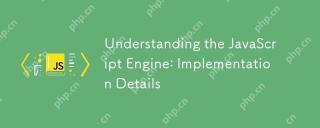
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
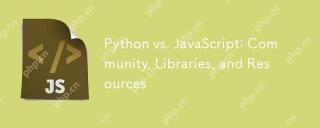
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
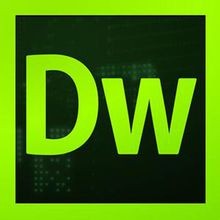
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
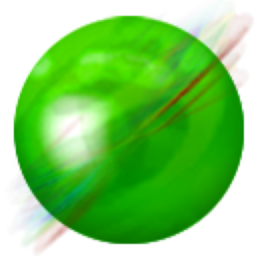
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment