


This article mainly introduces the method of using plotly to draw data charts in Python. It analyzes the techniques of plotly drawing with examples and has certain reference value. Interested friends can refer to it
Introduction: Use The python-plotly module is used to draw stress test data and generate static html page results for display.
Many friends have the experience of stress testing modules during the development process. After the stress test, everyone often likes to use Excel to process the stress test data and draw data visualization views, but this is not very convenient to use. Web page for data display. This article will introduce the use of the python-plotly module to draw stress test data and generate a static HTML page to facilitate the display of results.
Introduction to Plotly
Plotly is a charting tool developed using JavaScript, providing an API to interact with mainstream data analysis languages (such as: Python, R, MATLAB). You can go to the official website https://plot.ly/ for more detailed information. Plotly is capable of drawing beautiful charts with user interaction.
Python-Plotly installation
This document mainly introduces the use of plotly’s Python API to perform several A simple chart drawing. For more usage of Plotly, please refer to https://plot.ly/python/
Python-Plotly can be installed using pip, and it is best to install and use it in Python version 2.7 and above. If you use Python2.6 version, please install Python2.7 and the corresponding pip yourself.
Plotly drawing example
line-plots
Drawing effect:
The generated html page provides rich interactive tools in the upper right corner.
Code:
def line_plots(name): ''' 绘制普通线图 ''' #数据,x为横坐标,y,z为纵坐标的两项指标,三个array长度相同 dataset = {'x':[0,1,2,3,4,5,6,7,8,9], 'y':[5,4,1,3,11,2,6,7,19,20], 'z':[12,9,0,0,3,25,8,17,22,5]} data_g = [] #分别插入 y, z tr_x = Scatter( x = dataset['x'], y = dataset['y'], name = 'y' ) data_g.append(tr_x) tr_z = Scatter( x = dataset['x'], y = dataset['z'], name = 'z' ) data_g.append(tr_z) #设置layout,指定图表title,x轴和y轴名称 layout = Layout(title="line plots", xaxis={'title':'x'}, yaxis={'title':'value'}) #将layout设置到图表 fig = Figure(data=data_g, layout=layout) #绘图,输出路径为name参数指定 pltoff.plot(fig, filename=name)
scatter-plots
Plotting effect :
Code:
def scatter_plots(name): ''' 绘制散点图 ''' dataset = {'x':[0,1,2,3,4,5,6,7,8,9], 'y':[5,4,1,3,11,2,6,7,19,20], 'text':['5_txt','4_txt','1_txt','3_txt','11_txt','2_txt','6_txt','7_txt','19_txt','20_txt']} data_g = [] tr_x = Scatter( x = dataset['x'], y = dataset['y'], text = dataset['text'], textposition='top center', mode='markers+text', name = 'y' ) data_g.append(tr_x) layout = Layout(title="scatter plots", xaxis={'title':'x'}, yaxis={'title':'value'}) fig = Figure(data=data_g, layout=layout) pltoff.plot(fig, filename=name)
bar-charts
Drawing Effect:
Code:
def bar_charts(name): ''' 绘制柱状图 ''' dataset = {'x':['Windows', 'Linux', 'Unix', 'MacOS'], 'y1':[45, 26, 37, 13], 'y2':[19, 27, 33, 21]} data_g = [] tr_y1 = Bar( x = dataset['x'], y = dataset['y1'], name = 'v1' ) data_g.append(tr_y1) tr_y2 = Bar( x = dataset['x'], y = dataset['y2'], name = 'v2' ) data_g.append(tr_y2) layout = Layout(title="bar charts", xaxis={'title':'x'}, yaxis={'title':'value'}) fig = Figure(data=data_g, layout=layout) pltoff.plot(fig, filename=name)
pie-charts
Plotting effect:
Code:
def pie_charts(name): ''' 绘制饼图 ''' dataset = {'labels':['Windows', 'Linux', 'Unix', 'MacOS', 'Android', 'iOS'], 'values':[280, 25, 10, 100, 250, 270]} data_g = [] tr_p = Pie( labels = dataset['labels'], values = dataset['values'] ) data_g.append(tr_p) layout = Layout(title="pie charts") fig = Figure(data=data_g, layout=layout) pltoff.plot(fig, filename=name)
filled-area-plots
This example is to draw a stacked line chart with a fill effect, which is suitable for analyzing data with stacked percentage attributes
Drawing effect:
Code:
def filled_area_plots(name): ''' 绘制堆叠填充的线图 ''' dataset = {'x':[0,1,2,3,4,5,6,7,8,9], 'y1':[5,4,1,3,11,2,6,7,19,20], 'y2':[12,9,0,0,3,25,8,17,22,5], 'y3':[13,22,46,1,15,4,18,11,17,20]} #计算y1,y2,y3的堆叠占比 dataset['y1_stack'] = dataset['y1'] dataset['y2_stack'] = [y1+y2 for y1, y2 in zip(dataset['y1'], dataset['y2'])] dataset['y3_stack'] = [y1+y2+y3 for y1, y2, y3 in zip(dataset['y1'], dataset['y2'], dataset['y3'])] dataset['y1_text'] = ['%s(%s%%)'%(y1, y1*100/y3_s) for y1, y3_s in zip(dataset['y1'], dataset['y3_stack'])] dataset['y2_text'] = ['%s(%s%%)'%(y2, y2*100/y3_s) for y2, y3_s in zip(dataset['y2'], dataset['y3_stack'])] dataset['y3_text'] = ['%s(%s%%)'%(y3, y3*100/y3_s) for y3, y3_s in zip(dataset['y3'], dataset['y3_stack'])] data_g = [] tr_1 = Scatter( x = dataset['x'], y = dataset['y1_stack'], text = dataset['y1_text'], hoverinfo = 'x+text', mode = 'lines', name = 'y1', fill = 'tozeroy' #填充方式: 到x轴 ) data_g.append(tr_1) tr_2 = Scatter( x = dataset['x'], y = dataset['y2_stack'], text = dataset['y2_text'], hoverinfo = 'x+text', mode = 'lines', name = 'y2', fill = 'tonexty' #填充方式:到下方的另一条线 ) data_g.append(tr_2) tr_3 = Scatter( x = dataset['x'], y = dataset['y3_stack'], text = dataset['y3_text'], hoverinfo = 'x+text', mode = 'lines', name = 'y3', fill = 'tonexty' ) data_g.append(tr_3) layout = Layout(title="field area plots", xaxis={'title':'x'}, yaxis={'title':'value'}) fig = Figure(data=data_g, layout=layout) pltoff.plot(fig, filename=name)
Summary
This article introduces the method of using python-plotly to draw data graphs, including line plots and scatter plots in examples. These five typical charts, scatter plots, bar charts, pie charts, and filled area plots, basically cover most types of test data. Dear friends, It can be deformed to draw more beautiful icons.
The above is the detailed content of Share a case of how Python uses plotly to draw data charts (pictures and texts). For more information, please follow other related articles on the PHP Chinese website!
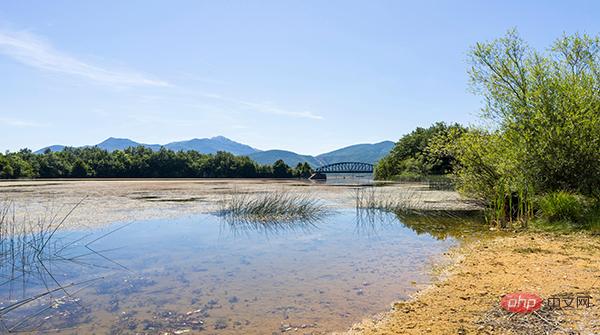
一、简介Plotly是一个非常著名且强大的开源数据可视化框架,它通过构建基于浏览器显示的web形式的可交互图表来展示信息,可创建多达数十种精美的图表和地图。二、绘图语法规则2.1离线绘图方式Plotly中绘制图像有在线和离线两种方式,因为在线绘图需要注册账号获取APIkey,较为麻烦,所以本文仅介绍离线绘图的方式。离线绘图又有plotly.offline.plot()和plotly.offline.iplot()两种方法,前者是以离线的方式在当前工作目录下生成html格式的图像文件,并自动打开;
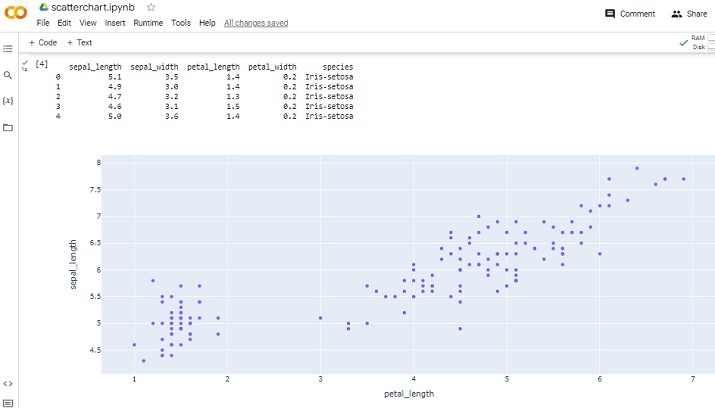
有时,任务是分析数据集并使用图表或绘图进行数据可视化。Plotly是一个很好的开源图形库,可以与Python一起使用,用于快速轻松地制作各种绘图和图表。在本文中,使用两个不同的示例,将名为Plotly的Python库与Python代码结合使用来绘制散点图。在第一个示例中,计算机系统中安装的Python用于运行为制作散点图而编写的Python程序。另一个例子,使用GoogleColab展示了在计算机中没有安装Python的情况下,仍然可以使用Python和Plotly并可以制作散点图的方法。在这两
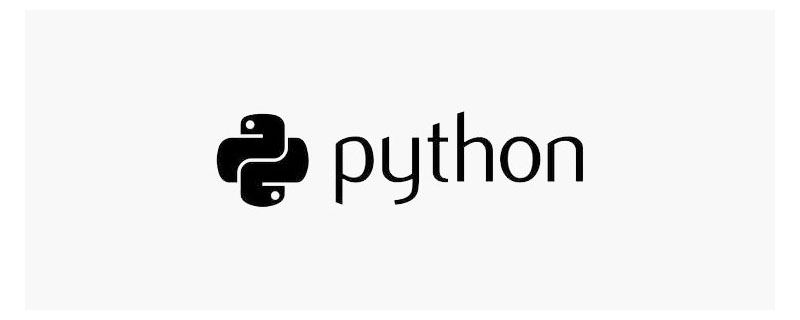
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于Seaborn的相关问题,包括了数据可视化处理的散点图、折线图、条形图等等内容,下面一起来看一下,希望对大家有帮助。
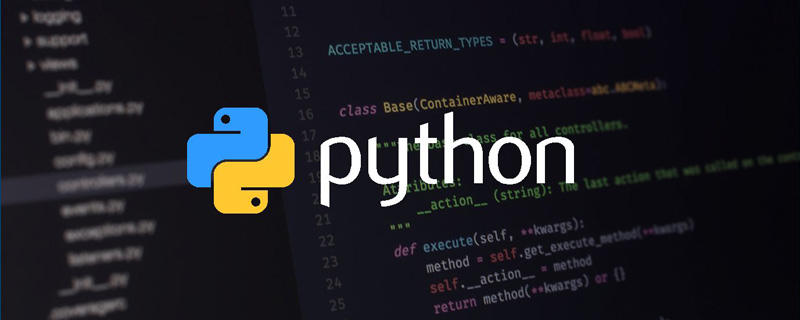
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于进程池与进程锁的相关问题,包括进程池的创建模块,进程池函数等等内容,下面一起来看一下,希望对大家有帮助。
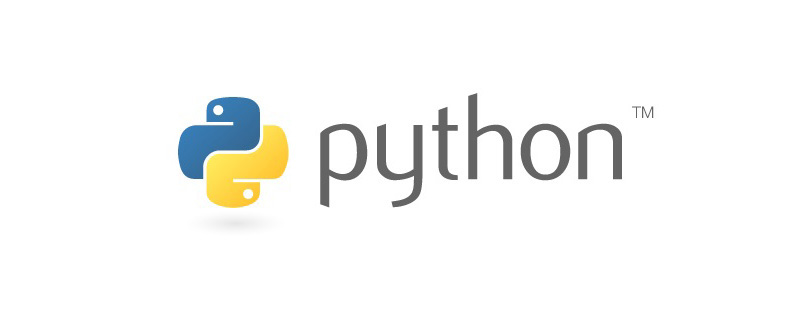
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于简历筛选的相关问题,包括了定义 ReadDoc 类用以读取 word 文件以及定义 search_word 函数用以筛选的相关内容,下面一起来看一下,希望对大家有帮助。
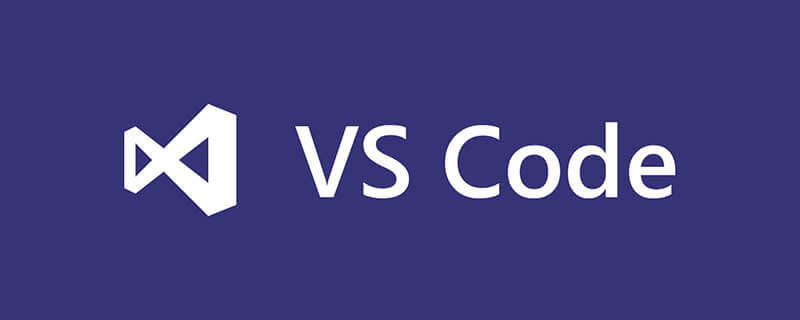
VS Code的确是一款非常热门、有强大用户基础的一款开发工具。本文给大家介绍一下10款高效、好用的插件,能够让原本单薄的VS Code如虎添翼,开发效率顿时提升到一个新的阶段。
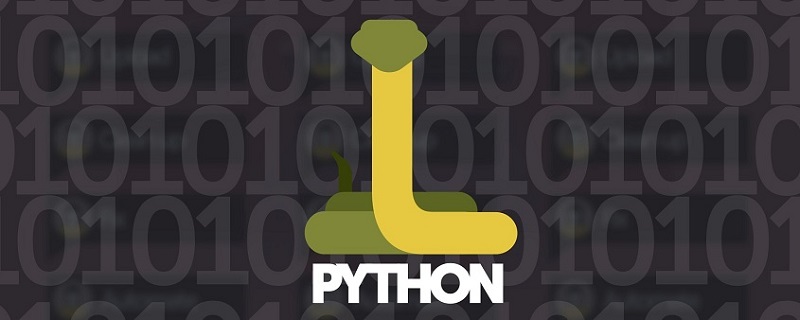
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于数据类型之字符串、数字的相关问题,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
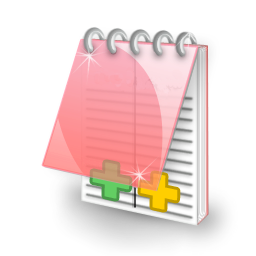
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
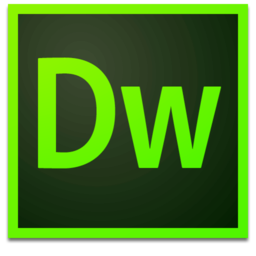
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
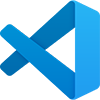
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
