Preface:
It took me roughly a week to read half of this book carefully. These are the reading notes I made. I hope it can give people who read this book a rough reference. It may be a bit messy and not comprehensive at the moment, I will sort it out and add it later. (2017-7-17)
Chapter 1 JavaScriptIntroduction
Chapter 2 Used in HTMLJavaScript
## Chapter 3 Basic concepts
3.1.Grammar
Case sensitive
Identifier(recommended to use camel casemyCar)
Comments // /**/Strict mode(use strict)
Statement Recommendationvar diff-a-b; use semicolon, and if(test) { alert(test); }Also use {}
3.2 Keywords and reserved words 3.3 VariableUninitializedundefined var message = “hi”; message = 100; The type can be changed at will
Function test(){ Var a = “hi”; 局部 b = “hi”; 全局 }
Test(); alert(a); Error alert(b); hi
3.4 Data type
typeof
Undefined (defined but not assigned)
Null(null object pointer)
Boolean(Boolean valuetrue false The flow control statement will automatically execute the corresponding boolean Conversion)
Number
(octal0 decimal ten Hexadecimal 0x Floating point value rangeNaN Number conversionNumber parseInt )
String(String, no difference between single and double quotes, escape sequence, string characteristics, toString String)
Object(Object The basis of all objectsvar o = new Object();)
Function in ES is an object, not a data type.
3.5Operator
Unary operator ++ -- , + -(can be used to convert data types)
Bit operators Bitwise not~num1 Bitwise AND& Bitwise OR| Bitwise XOR^ Shift leftShift right>> Unsigned right Shift>>
Boolean operator ! && || Multiplicative operator* / % Additive operator+ - Relational operator =
Equality operator == != === !== Conditional operator? :
Assignment operator = *= /= %= += -= >= >> >=
Comma operator
3.6 Statement
3.7 Function
##arguments No overloading
Chapter 4 Variables, scope and memory issues
4.1Values of basic types and reference types
##Dynamic Attributes(Reference types can dynamically add attributes)
Copy variable values(The basic types are different Space, the reference type refers to the same space)
Pass parameters (Same) Detection type (typeof instanceof) 4.2Execution environment and scope Scope Chain Extended scope chain(try-catch with) No block-level scope(if for Declare variable query identifier) 4.3Garbage collection Strategy(Mark clear reference count ) Performance Issues Managing Memory 5.1 ObjectType Var person = {};(Suitable for passing a large number of parameters to functions) var parson = new Object(); Person["name"](Use # when using variables to represent attributes ##) person.name(Recommended) Type Detect array: instanceof Array.isArray() Conversion method: toString() valueOf() toLocaleString() join() Stack method: push() pop() Queue method: shift() unshift() Reordering method: reverse() sort(You can add this parameter of comparison function) Operation method:concat()The original has not changed and added slice()The original has not changed to intercept the segment splice()The original has changed Can be deleted or added Position method: indexOf() lastIndexOf() Return the position of the item Iteration method: every() filter() forEach() map() some() Each item is processed, but the original array will not change Merge method: reduce()Order reduceRight()Reverse order The two items are always the same Traverse to the end Type Inside parse("May 25,2004") UTC(2005,0) Date.now() Inherited method: toLocaleString( ) toString() valueOf() Date formatting method: toDateString() toTimeString() toLocaleDateString() toLocaleTimeString() toUTCString() Date/Time component method: too many specific readings Type Literal var pattern1 = / [bc]at / i; Constructor var pattern2 = new RegExp(“ [bc]at ”, ” i ”); Instance attributeglobal ignoreCase lastIndex multiline source It’s useless RegExpInstance method: exec() Capturing group(Capturing multiple groups of matches) text() Check if it matches once RegExpConstructor attributes: There are a lot of them, used to see the relevant information of the latest match Limitations of the pattern: Lack of advanced regular expression features supported by some languages (Perl), but in most cases Enough 5.5 Function type Function is an object(is alsoFunction Instances of ), the function name is a pointer. function sum(num1,num2) { } Var sum = function(num1,num2){ }; No overloading: the function is an object and the function name is a pointer. Function declaration and function expression: function ******* Function declaration promotion var sum = ** ***** Will not be promoted Function internal attributes: argumentsObject (Attributecalleepoints to function) thisObject(Points to the environment object where the function executes ) Attribute caller (Points to the function that calls the current function, if it is a global scope function, the value is null) Function attributes and methods: Attributes(lengthDeclaration parameters Number prototypeInheritance) Non-inherited method: call( ) apply() bind() a.apply( b, c); a.call( b, d ); Run the function a in the environment object b, and pass in the parameters to ac( argumentor array) / d( Write each item out ) Var a = b.bind(c); a is a function that runs in the c environment object 5.6Basic packaging type: BooleanType: var booleanObject = new Boolean(true); It is recommended never to use Boolean objects. NumberType: var numberObject = new Number(10); toFixed( ) toExponential() toPrecision() It is also recommended not to instantiate the Number type directly. String Type: var stringObject = new String(“hello world”); lengthAttributes 1.Character method: charAt()or string[] Return the corresponding character charCodeAt() Return the corresponding character encoding 2. String operation method: concat() or# The ##+ operator looks the same as the array's concat() Intercept string, three are very flexible String position method: indexOf( ) lastIndexOf() Method Remove all prefix and suffix spaces trimLeft() trimRight() Classic method Specific regions This is more secure The same essence as RegExpexec() returns the string that matches Array Returns the index of the first matching item otherwise returns -1 Match and replace the string Match the delimiter to generate an array Compare two strings Static method Encode multiple characters Become a string method For example big() string Single built-in object Object Encoding method: Entire encodeURIComponent() some A paragraph of encoding The whole decodeURIComponent() A certain paragraph of decoding Method Very powerful and very dangerousECMAScriptParser Object properties undefined、Array......are all properties The browser implements the global object as a part of the window object Object Properties of the object Some special values such as π Method Rounding method: convert decimal value into integer Math.ceil(25) Add one Math.floor(25) Back one Math.round(25) Rounding random()Method [0,1) Random number between Other methods Too much reading 6.1Understanding objects 6.1.1Attribute type: Data attribute: 4 attributes Accessor properties: does not contain data values 4 properties are defined via Object.defineProperty() 6.1.2Define multiple properties: Object.defineProperties() Can be a data property or an accessor property. 6.1.3Read property characteristics: Object.getOwnPropertyDescriptor() 6.2Create Object 6.2.1Factory Mode(看书) 6.2.2Constructor pattern(看书 new constructor ) 6.2.3Prototype pattern: 1. Understand the prototype object 2.Prototype and inoperator(in for-in) 3.Simpler prototype syntax(Object literal to access the object) 4.The dynamics of the prototype(The prototype can be replaced by another object, but it will be invalid for the instance that has been created) 5.Prototype of native object( is to use prototype mode, you can define new methods in it, but it is not recommended) 6. Problem with prototype objects: There are no properties belonging to the instance itself 6.2.4Use the constructor pattern and prototype pattern in combination The most common mode, instances have their own properties and methods, and some are shared. 6.2.5Dynamic prototype mode Add a judgment statement to the above mode to dynamically add a judgment statement to the prototype Add method. 6.2.6Parasite Constructor Pattern The only difference between the Parasitic Constructor Pattern and the Factory Pattern is one new, and it has multiple return. The returned object has nothing to do with the constructor, so the instanceof operator cannot be used to determine the object type. Not recommended. 6.2.7Safe constructor pattern is similar to the parasitic constructor pattern, but does not use this and new. instanceofcannot be detected either 6.3Inheritance 6.3.1Prototype chain Prototype search mechanism 1.Don’t forget the default prototype: the default for all functions Prototypes are all instances of Object. 2.Determine the relationship between prototype and instance: instanceof isPrototypeOf() 3. Carefully Define methods: New methods must be defined after the instance replaces the prototype. 4. Prototype chain problem: Attributes outside the prototype are also inherited When creating an instance of a subtype, parameters cannot be passed to the constructor of the supertype. Rarely used alone. 6.3.2 Borrow the constructor using call or apply Inherit and pass parameters but only use the constructor pattern. is also rarely used alone. 6.3.3Combined inheritance Prototype chain implementation Prototype attributes and Inheritance of methods Use constructor to implement Inheritance of instance attributes The most commonly used inheritance method 6.3.4Prototypal inheritance(看书) The constructor is not used The retained type can be used, but the properties of the reference type will be shared like the prototype mode. 6.3.5Parasitic inheritance(看书) Constructor is not considered Can be used But function reuse cannot be achieved 6.3.6Parasitic combined inheritance(看书) Problem with compositional inheritance: the supertype's constructor is called twice, resulting in duplicate properties in the instance and prototype. Parasitic combined inheritance solves this problem and becomes the most ideal inheritance paradigm. How to define a function: Function declaration(Function declaration is promoted with name) Function expression( name is empty anonymous function/Named general function expressions are anonymous functions) 7.1Recursion Strict mode cannot be used arguments.callee Named function expressions can be used. 7.2Closure: A function that has access to a variable in the scope of another function Self-summary: Closures are functions, generally anonymous functions are used 1. Closures can access variables in returned external functions. 2.The active object of another function will be saved until the closure's scope chain is destroyed. 7.2.1 Closures and variables Side effects: closures are saved in the scope chain is the entire variable object, so the variable returned is the last value saved. For example, in the for loop, unsatisfactory situations will occur. 7.2.2AboutthisObject The this object in the closure, the closure may be placed on the global object in some special circumstances, so this changes. Know. 7.2.3Memory leak Use closures to ensure normal memory recovery 7.3Imitate block-level scope Use anonymous self-executing functions to imitate. Self-summary effect: 1.It will be destroyed after internal execution. 2.Anonymous self-executing functions can reduce pollution of global methods and properties. Closures have a memory problem, and it is very comfortable to use this combination. 7.4Private variables Everything on the object is public Everything in the function is private Create the public method of the function through closure(Privileged method) ---------Used to obtain private variables Created in the constructor ##this.****** = function(){ **** }; But each instantiation will rebuild a set of methods, which is wasteful. 7.4.1Static private variables An instance does not have its own private properties Improves code reusability, but each instance does not have its own private variables. (Because this is called a static private variable) Use instance variables or static private variables, depending on the specific needs. 7.4.2Module Mode Literal An instance object ( ) Define private variables and methods inside the anonymous function, and then use an object literal as the return value of the function. 7.4.3 Must be an instance of a certain type , some properties or methods must also be added. Chapter 5 Reference Type
Chapter Six Object-oriented programming
Chapter 7 Function expression
The above is the detailed content of Introduction to JavaScript Advanced Programming. For more information, please follow other related articles on the PHP Chinese website!
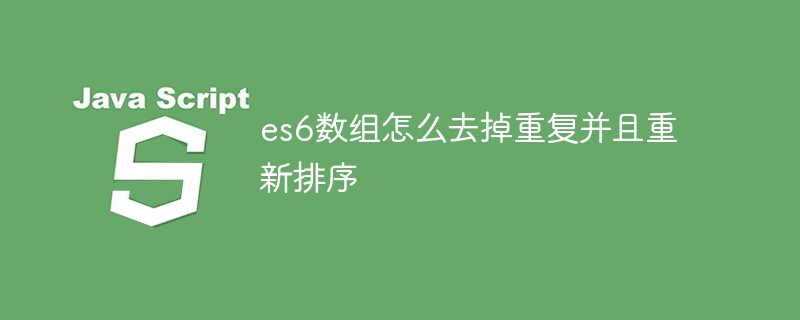
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
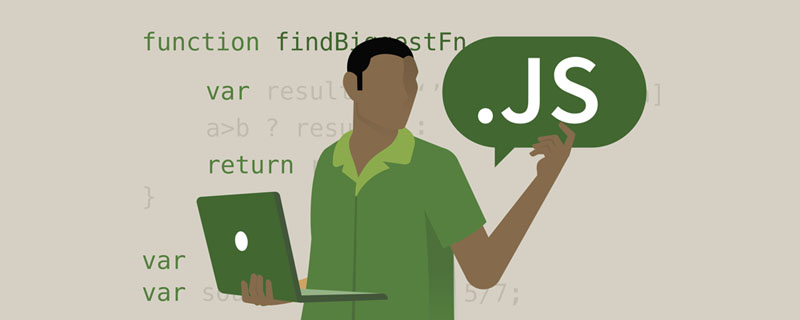
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
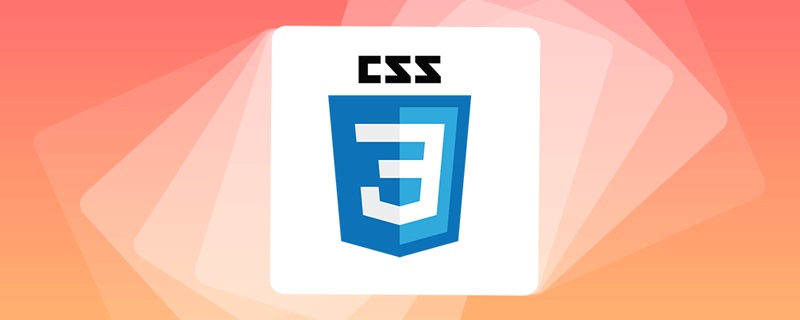
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
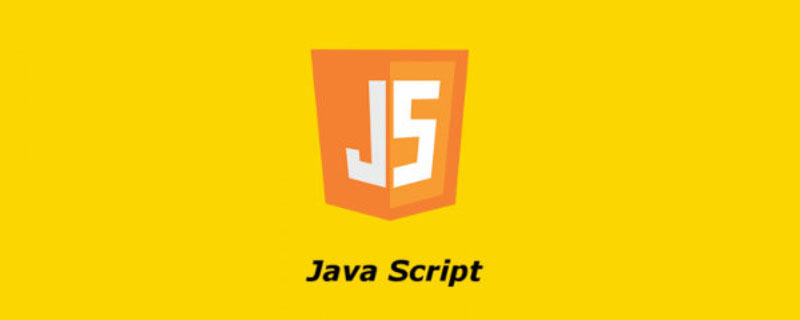
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
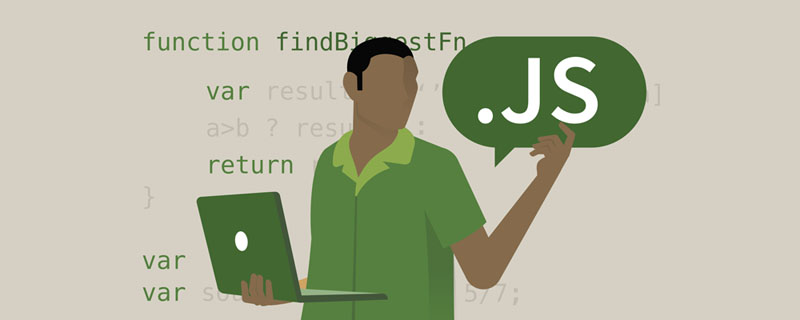
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
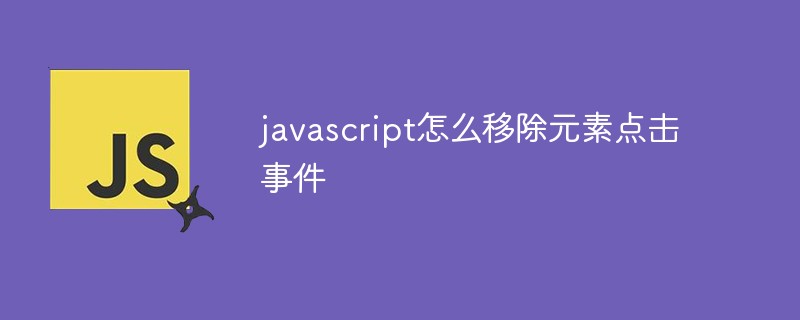
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
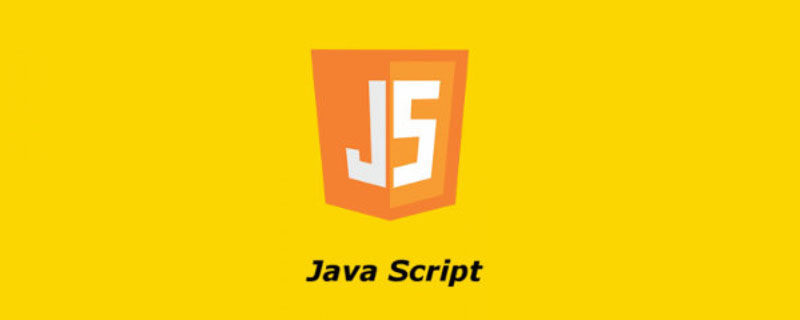
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
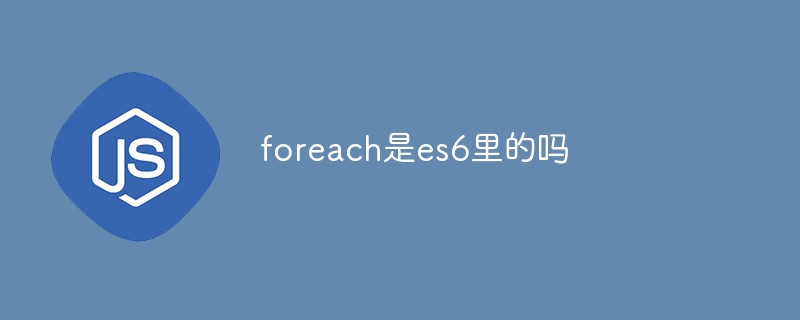
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
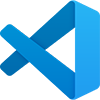
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
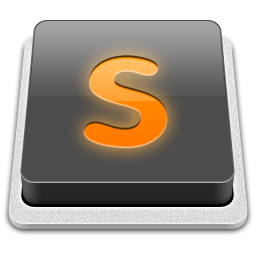
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Zend Studio 13.0.1
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
