Encoding
ASCII--0~127 65-A 97-a
Western European code table---ISO-8859-1---0-255---1 character Section
gb2312----0-65535---gbk---2 bytes
Unicode encoding system---utf-8---3 bytes
中 f
bit Byte 1Byte = 8bit 1KB=1024B MB GB TB PB---Storage unit in computer
Constant
Integer constant-- -All integers 3,99,107
Decimal constants---All decimals 3.5 100.9
Character constants---Use single quotes to identify a letter, number, or symbol 'a' ' =' ' '
String constant---Use double quotes to identify one or more characters "abc" "234" "q2" ""
Boolean constant---Use Indicates logical value---true/false
Empty constant---null
5-integer, 5.0-decimal '5'-character "5"-string '5.0'- Writing error "5.0"-String
Binary system: full binary to one, 0~1 1+1=10 0b10011 0b0011, starting from JDK1.7, 0b is allowed as Beginning to identify a number is a binary number
Octal: full eights into one, 0~7, 7+1=10 It is required to start with 0 06 015
Decimal: full tenths One, 0~9
Hexadecimal: full hexadecimal one, 0~9,, A~F, 9+1=A f+1=10 It is required to start with 0x 0x5 0xad
Conversion from decimal to binary
Convert from decimal to binary: keep dividing by 2 to take the remainder, and then put the remainder in reverse order
Convert from binary to decimal: from the low bit Starting from the bit order, multiply the bit order by the power of 2, and then sum up
Convert binary to octal: starting from the low order, every three digits are divided into one group, less than three Filling the bits with 0 produces one octal number. Arrange these numbers in order to convert octal to binary: one to three --- one octal number produces three binary digits.
Convert binary to hexadecimal: the process of converting four into one
Variable
System.out.println(i);
int i = 5;---No---The variable must be declared before use
int i;
System.out.println(i);---No--The variable is in use Must be initialized before
Data type
Basic data type
Numeric type
Integer type
byte---Byte type ---1 byte--- -2^7~2^7-1 --- -128~127
byte b = 5; byte b2 = -128;
short---short integer---2 bytes--- -2^15~2^15-1 --- -32768~32767
short s = 54; short s = -900 ;
int---integer---4 bytes--- -2^31~2^31-1
int i = 100000;
int j = 100_000_000;--It is allowed starting from JDK1.7. These will be automatically ignored during compilation_ -> int j = 100000000;
int i = 00001111;---Octal
The default type of integer in Java is int
long---long integer type---8 bytes--- -2^63~2^63-1---ending with L indicates that this number is a long type number
long l = 3L;
Floating point type
float---single precision---4 bytes---must end with f
float f = 3.2f;
double---double precision---8 bytes
The default decimal type in Java is double type
double d = 3.5;
double d = 4.6D;---Yes
double d = 3.9e4; //It is scientific notation in decimal system
double d = 0x3p2; //It is hexadecimal Scientific notation -> 12
Character type
char---2 bytes--- 0 ~65535
char c = 'a';
char c = '中';
Boolean
boolean---true/false
boolean b = false;
Reference data type
Class ---class Interface ---interface Array ---[]
Data type conversion
Implicit conversion/automatic type conversion
byte b = 100;
int i = b;
long l = 63;---Yes---when the integer value is within the range of int type , you don’t need to add the ending L
Rule 1: Small types can be converted into large types---byte->short->int->long float->double
int i = 5;
float f = i;
long l = 6;
float f = l;
Rule 2: Integer Can be converted to decimal, but precision loss may occur
char c = 'a';
int i = c;
Rule 3: Character type can be converted to integer type
short s = 'a';---Yes
char c = 100;---Yes
char c = 'a' ;
short s = c;---Not possible
defines a variable c of type char. The stored data is a character. There is no need to check the specific character encoding. When assigning a value to short type, short needs to check whether the encoding corresponding to the character is within the value range of the short type. At this time, the specific encoding corresponding to the character cannot be determined. Since the value range of the short type does not completely overlap with the char type, in order to prevent If it exceeds the range, assignment is not allowed.
short s = 97;
char c = s;--not possible
Explicit conversion/forced type Conversion
long l = 54;
int i = (int)l;
double d = 3.5;
int i = (int)d ;---When converting a decimal to an integer, the decimal part is discarded directly
double type cannot store decimals accurately
Hexadecimal--Hexadecimal
Decimal-- Decimal
Octal---Octal
Binary--Binary
Operator
Arithmetic operator
+addition-subtraction*multiplication/division% modulo++auto-increment--auto-decrement+string concatenation
int i = 5210 / 1000 * 1000;--->i = 5000;
Note:
1. After the integer operation is completed, the result must be an integer
2. Integer When dividing by 0, the compilation passes and the error is reported when running---ArimeticException---Arithmetic exception
3. The result of dividing a decimal by 0 is Infinity
4. The result of 0/0.0 is NaN---Not a Number---Not a number
5. The byte/short type will be automatically promoted to the int type during operation
%Remainder operation
-5%3=-2 -4%3=-1 -3%7=-3
5%-3=2 7%-2=1 2%-8= 2
-5%-3=-2 -9%-3=0
For the remainder of a negative number, first follow the remainder operation of a positive number and look at the number to the left of the remainder sign The sign of #++/--
For ++, increment by 1 on the original basis
int i = 5;
int j = ++i;--- > i increments by 1, and then assigns the value of i to j---increments first, and then operates
int j = i++;--->Get the value of i first, 5, and increments i becomes 6, and then assign the obtained value 5 to j---operate first, then increment
int i = 3;
int j = ++i * 2;-> ; j = 8;
int j = i++ * 2;->j = 6
int i = 6;
int j = i++ + ++i;->i = 8; j = 14;
int j = ++i + i++;->i = 8; j = 14
byte b = 5;
b++;---JVM will perform forced type conversion on the result at the bottom level, and then convert the result into byte type
char c = 'a';
System.out.println(c + 4);--can
char c2 = 'd';
System.out. println(c + c2);---Promote to int type and then perform operation
+ String concatenation operation
"a" + "b"-- -> "ab"
"a" + 3---> "a3"
"a" + true-> "atrue"
2 + 4 + “f”-> “6f”
“f” + 2 + 4-> “f24”
Assignment operator
= += -= *= /= %= &= |= ^= >= >>>= ~=
int i= 5;
i + = 3; -> i = i + 3; -> i = 8;
i -= 2;-> i = i - 2;-> i = 3;
int j;
j += 4;---No
int i = 5;
i += i -= i *= 5;--> i = -15;
i = 5 + ( 5 - (5 * 5)) ;
i += i -= i *= ++ i;--->i = -20;
i += i*= i-= (i++ + --i);---> i = -20;
i = 5 + ( 5 * (5 - (5 + 5)));
byte b = 5;
b += 3;--- Yes
byte b = 125;
b += 3;---Yes--- -128
Comparison/relational operator
= =Equal!=Not equal> = ##3 == 4;-> false
instanceof---Judges the relationship between objects and classes- -Can only be used for reference data typesString s = “abd”;System.out.println(s instanceof String);---trueSystem. out.println(“def” instanceof String);---trueLogical operator is used to operate logical values&AND|OR! NOT^XOR && short circuit with || short circuit or true&true=true true&false=false false&true=false false&false=falsetrue|true=true true|false=true false|true=true false|false= false!true=false !false=truetrue^true=false true^false=true false^true=true false^false=falseFor &&, If the value of the previous expression is false, then it can be determined that the value of the entire expression is false, and the operation after && will no longer be performed.ternary/ternary/conditional operatorLogical value? Expression 1: Expression 2If the logical value is true, execute expression 1; otherwise, execute expression 2int i = 5, j = 7;i > j ? System.out.println(i): System.out.println(j);---No! There must be a result after the ternary operator operation is completed! double d = i > j ? i * 2.5 : j;---The return value types of the two expressions are either consistent or compatibleGet data from the consoleimport java.util.Scanner; //Written under package, above classScanner s = new Scanner(System.in);int i = s. nextInt();double d = s.nextDouble();String str = s.nextLine();String str2 = s.next();The above is the detailed content of Java Basics Explained-Basic Data Types and Operations. For more information, please follow other related articles on the PHP Chinese website!
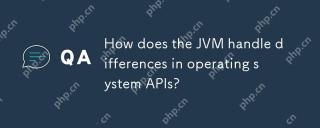
JVM handles operating system API differences through JavaNativeInterface (JNI) and Java standard library: 1. JNI allows Java code to call local code and directly interact with the operating system API. 2. The Java standard library provides a unified API, which is internally mapped to different operating system APIs to ensure that the code runs across platforms.
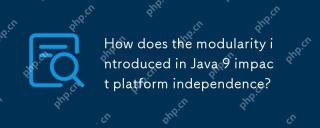
modularitydoesnotdirectlyaffectJava'splatformindependence.Java'splatformindependenceismaintainedbytheJVM,butmodularityinfluencesapplicationstructureandmanagement,indirectlyimpactingplatformindependence.1)Deploymentanddistributionbecomemoreefficientwi
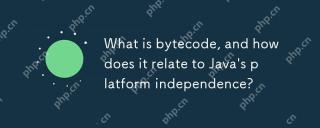
BytecodeinJavaistheintermediaterepresentationthatenablesplatformindependence.1)Javacodeiscompiledintobytecodestoredin.classfiles.2)TheJVMinterpretsorcompilesthisbytecodeintomachinecodeatruntime,allowingthesamebytecodetorunonanydevicewithaJVM,thusfulf
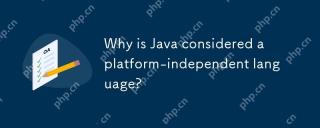
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),whichexecutesbytecodeonanydevicewithaJVM.1)Javacodeiscompiledintobytecode.2)TheJVMinterpretsandexecutesthisbytecodeintomachine-specificinstructions,allowingthesamecodetorunondifferentp
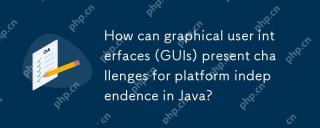
Platform independence in JavaGUI development faces challenges, but can be dealt with by using Swing, JavaFX, unifying appearance, performance optimization, third-party libraries and cross-platform testing. JavaGUI development relies on AWT and Swing, which aims to provide cross-platform consistency, but the actual effect varies from operating system to operating system. Solutions include: 1) using Swing and JavaFX as GUI toolkits; 2) Unify the appearance through UIManager.setLookAndFeel(); 3) Optimize performance to suit different platforms; 4) using third-party libraries such as ApachePivot or SWT; 5) conduct cross-platform testing to ensure consistency.
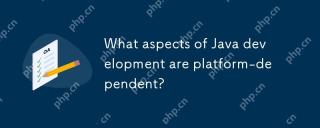
Javadevelopmentisnotentirelyplatform-independentduetoseveralfactors.1)JVMvariationsaffectperformanceandbehavioracrossdifferentOS.2)NativelibrariesviaJNIintroduceplatform-specificissues.3)Filepathsandsystempropertiesdifferbetweenplatforms.4)GUIapplica
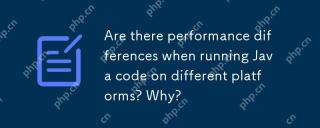
Java code will have performance differences when running on different platforms. 1) The implementation and optimization strategies of JVM are different, such as OracleJDK and OpenJDK. 2) The characteristics of the operating system, such as memory management and thread scheduling, will also affect performance. 3) Performance can be improved by selecting the appropriate JVM, adjusting JVM parameters and code optimization.
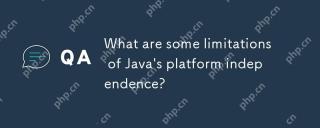
Java'splatformindependencehaslimitationsincludingperformanceoverhead,versioncompatibilityissues,challengeswithnativelibraryintegration,platform-specificfeatures,andJVMinstallation/maintenance.Thesefactorscomplicatethe"writeonce,runanywhere"


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
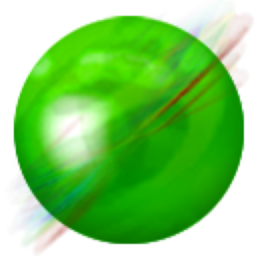
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
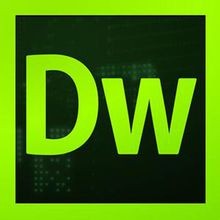
Dreamweaver CS6
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
