


Detailed explanation of how to implement overloading examples of functions and constructors in PHP
Since PHP is a weakly typed language, the input parameter type of the function cannot be determined (type hints can be used, but type hints cannot be used for integer types, strings and other scalar types), and for a function, for example, only 3 input parameters are defined, but PHP inputs 4 or more parameters when calling. Therefore, based on these two points, it is destined that functions cannot be overloaded in PHP (similar to Javascript languages), and there cannot be constructors of overloading.
Since implementing function overloading is very helpful to improve development efficiency, it would be great if it could be like C# or C++. In fact, PHP provides a magic method, mixed call (string name, array arguments). This method is also mentioned in the php manual. According to the official documentation, it is said that this method can achieve function overloading. When calling a method that does not exist in the object, if the call() method is defined, the method will be called. For example, the following code:
<?php class A { function call ( $name, $arguments ) { echo "call调用<br/>"; echo '$name为'.$name."<br/>"; print_r ($arguments); } } (new A)->test("test","argument"); ?>
The running result is:
call call
$name is test
Array ( [0] => test [ 1] => argument )
So you only need to use this magic method to achieve function overloading.
The code is as follows:
<?php class A { function call ($name, $args ) { if($name=='f') { $i=count($args); if (method_exists($this,$f='f'.$i)) { call_user_func_array(array($this,$f),$args); } } } function f1($a1) { echo "1个参数".$a1."<br/>"; } function f2($a1,$a2) { echo "2个参数".$a1.",".$a2."<br/>"; } function f3($a1,$a2,$a3) { echo "3个参数".$a1.",".$a2.",".$a3."<br/>"; } } (new A)->f('a'); (new A)->f('a','b'); (new A)->f('a','b','c'); 输出如下: 1个参数a 2个参数a,b 3个参数a,b,c
Similarly, in PHP, overloading of constructors can only be implemented in a flexible way. The key element of implementation is to obtain input parameters and determine which method to call based on the input parameters. The following is a sample code:
<?php class A { function construct() { echo "执行构造函数<br/>"; $a = func_get_args(); //获取构造函数中的参数 $i = count($a); if (method_exists($this,$f='construct'.$i)) { call_user_func_array(array($this,$f),$a); } } function construct1($a1) { echo "1个参数".$a1."<br/>"; } function construct2($a1,$a2) { echo "2个参数".$a1.",".$a2."<br/>"; } function construct3($a1,$a2,$a3) { echo "3个参数".$a1.",".$a2.",".$a3."<br/>"; } } $o = new A('a'); $o = new A('a','b'); $o = new A('a','b','c'); ?>
Execute the constructor
1 parameter a
Execute the constructor
2 parameters a,b
Execute the constructor
3 parameters a, b, c
By the way, just like C# and other object-oriented languages, in PHP, if the constructor is set to private or protected, the object cannot be instantiated.
The above is the detailed content of Detailed explanation of how to implement overloading examples of functions and constructors in PHP. For more information, please follow other related articles on the PHP Chinese website!

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
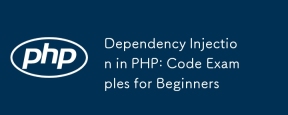
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
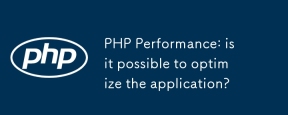
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
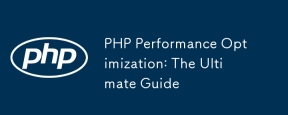
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
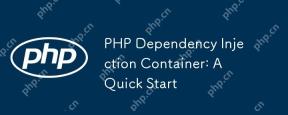
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
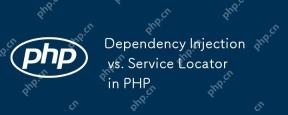
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
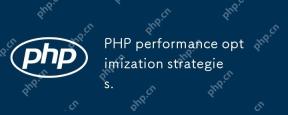
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
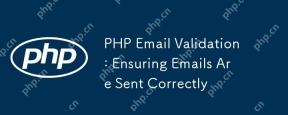
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
