How to stop bubbling and prevent browser default behavior in js
<br>
Event compatibility
function myfn(e){ var evt = e ? e:window.event; }
js stop bubbling
function myfn(e){ window.event? window.event.cancelBubble = true : e.stopPropagation(); }
js prevent default behavior
function myfn(e){ window.event? window.event.returnValue = false : e.preventDefault(); }
Prevent bubbling
w3c’s method is e.stopPropagation(), IE uses e.cancelBubble = true
stopPropagation is also a method of the event object (Event), which is used to prevent the bubbling of the target element. event, but will not prevent the default behavior. What is a bubbling event? If a "click" event is bound to a button, the "click" event will be triggered in its parent element in turn. stopPropagation prevents events from the target element from bubbling up to the parent element. For example: ·
<div> <ul> <li>test</li> </ul> </div>
above, the Demo is as follows. When we click test, alert("li"), alert("ul"), alert("div") will be triggered in sequence. This It's just events bubbling up.
Prevent bubbling
window.event? window.event.cancelBubble = true : e.stopPropagation();
Prevent default behavior
The w3c method is e.preventDefault() , IE uses e.returnValue = false;
preventDefault, which is a method of the event object (Event), and its function is to cancel the default behavior of a target element. Since we are talking about default behavior, of course the element must have a default behavior before it can be canceled. If the element itself does not have a default behavior, the call will of course be invalid. What elements have default behaviors? Such as link , submit button , etc. When the event object's cancelable is false, it means that there is no default behavior. Even if there is a default behavior, calling preventDefault will not work.
We all know that the default action of the link is to jump to the specified page. Let’s take it as an example to prevent it from jumping:
//假定有链接<a>caibaojian.com</a> var a = document.getElementById("testA"); a.onclick =function(e){ if(e.preventDefault){ e.preventDefault(); }else{ window.event.returnValue == false; } }
return false
javascript's return false will only prevent the default behavior, but using jQuery will both prevent the default behavior and prevent the object from bubbling.
The following one uses native js, which will only prevent the default behavior and will not stop bubbling.
<div> <ul> <li><a>caibaojian.com</a></li> </ul> </div> var a = document.getElementById("testB"); a.onclick = function(){ return false; };
The following one Use jQuery to both prevent the default behavior and stop bubbling
//code from <div> <ul> <li><a>caibaojian.com</a></li> </ul> </div> $("#testC").on('click',function(){ return false; });
Demonstration: Stop bubbling and prevent the default behavior
Summary of usage
When you need to stop the bubbling behavior, you can use
function stopBubble(e) { //如果提供了事件对象,则这是一个非IE浏览器 if ( e && e.stopPropagation ) //因此它支持W3C的stopPropagation()方法 e.stopPropagation(); else //否则,我们需要使用IE的方式来取消事件冒泡 window.event.cancelBubble = true; }
When you need to prevent the default behavior, you can use
//阻止浏览器的默认行为 function stopDefault( e ) { //阻止默认浏览器动作(W3C) if ( e && e.preventDefault ) e.preventDefault(); //IE中阻止函数器默认动作的方式 else window.event.returnValue = false; return false; }
Event attention points
event represents the status of the event, such as the element that triggered the event object, the position and status of the mouse, Keys pressed, etc.;
#event object is only valid during the event.
The events in firefox are different from those in IE. The events in IE are global variables and can be used at any time; the events in firefox can only be used when guided by parameters, and are temporary variables at runtime.
In IE/Opera it is window.event, in Firefox it is event; and the object of the event is window.event.srcElement in IE, in Firefox it is event.target, both are available in Opera.
The following two sentences have the same effect:
function a(e){ var e = (e) ? e : ((window.event) ? window.event : null); var e = e || window.event; // firefox下window.event为null, IE下event为null }
<br>
The above is the detailed content of How to stop bubbling and prevent browser default behavior in js. For more information, please follow other related articles on the PHP Chinese website!
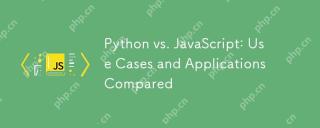
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
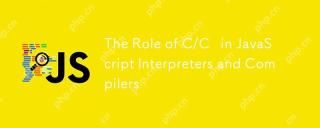
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
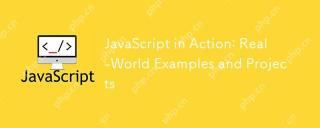
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
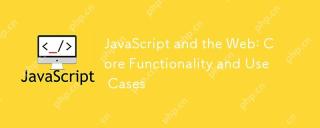
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
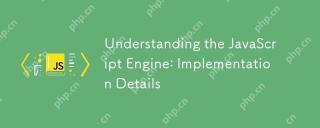
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
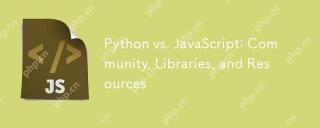
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
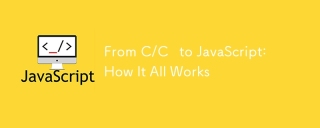
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
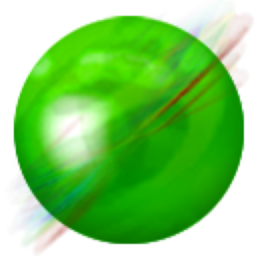
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Atom editor mac version download
The most popular open source editor
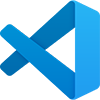
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment