Infinite loading strategy
Since it is infinite swiping, it is not possible to get all the pictures to be loaded at the same time; because there must be Scratching effect, so the left and right sides of the current image need to be preloaded. Therefore, you can use three pictures as a window and use the rotation strategy to achieve an infinite swipe list.
<p class="lightbox"> <p class="container"> <p class="lightbox-item prev"></p> <p class="lightbox-item current"></p> <p class="lightbox-item next"></p> </p> </p>
The .lightbox full-screen layout, the .lightbox-item contains the previous, current, and next pictures. Whenever the picture is swiped, we change the next picture to the previous picture, the current picture to the previous picture, the original previous picture as the next picture and preload the next picture resource.
Note that an extra layer of .container is added here and it wraps all images. In this way, when we need the picture to slide as a whole, we can animate it.
Layout style
We set the .lightbox to full screen, put the .prev to the left of the current screen, and .next to the right.
.lightbox, .container .lightbox-item{ position: fixed; left: 0; right: 0; top: 0; background-color: #000; } .container{ position: absolute; } .lightbox-item{ /* 我们用背景图来显示图片 */ position: absolute; background-repeat: no-repeat; background-position: center; background-size: contain; } .lightbox-item.prev{ left: -100%; right: 100%; } .lightbox-item.next{ left: 100%; right: -100%; }
Under some browsers (such as a certain Samsung's own browser), it will be found that the page content is actually three times as wide as the page. So the page was widened so that all three pictures were displayed. Setting overflow can fix this problem:
.lightbox{ overflow: hidden; }
Binding touch events
The key to the picture swiping effect lies in the user The touch event can be directly bound to the window because it is full-screen browsing. But when binding to window, we have to pay attention to conflicts and binding issues. You can .off the function you registered, or you can add a namespace, for example:
$(window) .on('mouseup.lightbox touchend.lightbox', onTouchEnd) .on('mousemove.lightbox touchmove.lightbox', onTouchMove) .on('mousedown.lightbox touchstart.lightbox', onTouchBegin) $(window) .off('mouseup.lightbox touchend.lightbox') .off('mousemove.lightbox touchmove.lightbox') .off('mousedown.lightbox touchstart.lightbox')
There are 6 The key events are:
## mousedown, mousemove, mouseup: mouse press, move and relax;
touchbegin, touchmove, touchend: touch press, move and leave.
Picture sliding animation
In fact, the picture is not animated as the finger moves, just Just update its position when touchmove.
// 起始位置,划动距离 var beginX, translateX; function onTouchBegin(e){ beginX = getCursorX(e); } function getCursorX(e) { // 如果是鼠标事件 if (['mousemove', 'mousedown'].indexOf(e.type) > -1) { return e.pageX; } // 如果是触摸事件 return e.changedTouches[0].pageX; } function onTouchMove(e){ translateX = getCursorX(e) - beginX; $('.container') .attr('transform:translate3d(' + translateX + ')'); .attr('-webkit-transform:translate3d(' + translateX + ')'); }
The -webkit-transform here is for compatibility with the Android UC browser, and everything else seems to be OK. Also note that translate3d enables hardware acceleration, while translateX does not. Therefore, the performance of translateX in ordinary Android browsers is very poor.
When encountering compatibility issues, I really want to talk about Tiansha’s UC. But then I thought about it, at least it doesn't have to be compatible with IE6, and I don't have to complain too much.
Determining the sliding target
The above code still lacks one onTouchEnd, that is, the user will let go after swiping a certain distance. what happens? If the swipe distance is large enough, then continue the animation and slide to the next picture, otherwise, return to the original position. At the same time, the swiping speed also needs to be detected. If the distance is short but the speed is very large, picture switching should also be performed.
Have we never considered the details here when we usually slide pictures?
Record the start time in onTouchBegin, and in onTouchEnd that is Calculable speed.
var beginTime, endTime; function onTouchBegin(e){ beginTime = Date.now(); } function onTouchEnd(e){ endTime = Date.now(); animateTo(getTarget()); }
Here getTarget() is used to calculate the picture to be swiped, while animateTo calls a swipe animation.
[Code]php code:function getTarget(){
// 首先检测划动距离,返回 -1, 0, 1 表示上一张,当前,下一张
var direction = getDirection(translateX, 0.3 * $(window).width());
// 如果划动距离检测为0,继续检测速度
if (direction === 0) {
var deltaT = Math.max(endTime - beginTime, 1);
var v = translateX / deltaT;
direction = getDirection(v, 0.3);
}
return ['.prev', '.current', '.next'][direction + 1];
}
function getDirection(offset, max) {
if (offset > max) return -1;
if (offset < -max) return 1;
return 0;
}
Animation after the stroke ends
After the swipe is completed, we need to slide the .container to the target image. In order to avoid abruptly replacing the current image with the target image, we set the transform animation to the target position and then replace it quietly. The following is the main logic of animateTo:
// 计算划动到的目标图片对应的translateX var translateX = $(window).width() * (1 - idx); $('.container').animate({ 'transform': 'translate3d(' + translateX + 'px, 0px, 0px)' '-webkit-transform': 'translate3d(' + translateX + 'px, 0px, 0px)' }, { duration: 1000, complete: function() { // 动画结束后进行图片轮换 var $wps = $('.container').find('.lightbox-item'); var $prev = $wps.filter('.prev'); var $curr = $wps.filter('.current'); var $next = $wps.filter('.next'); if (target === '.prev') { idx--; $prev.attr('class', 'lightbox-item current'); $curr.attr('class', 'lightbox-item next'); $next.attr('class', 'lightbox-item prev'); prefetch('.prev', idx - 1); } else if (target === '.next') { idx++; $next.attr('class', 'lightbox-item current'); $curr.attr('class', 'lightbox-item prev'); $prev.attr('class', 'lightbox-item next'); prefetch('.next', idx + 1); } $(.container).css('transform', 'none'); $(.container).css('-webkit-transform', 'none'); } });
Remember? We need to pre-fetch the next picture after sliding it. In this way, the picture can be scrolled continuously. The operation of prefetch is to prefetch the next image address from the server, and then replace the oldest image in the sliding window. Its specific implementation is also related to the server, so I won’t go into details here.
注意!当动画结束时对.prev,.current,.next进行轮换并重置transform。 如果重置为translate3d(0,0,0)则动画仍会继续,页面就会跳一下。 如果重置为none则会非常平滑,同时别忘了-webkit-transform来兼容更多浏览器。
TouchBegin 的兼容性
在Android ICS下如果touchbegin和第一个touchmove中都未调用 preventDefault, 后续的touchmove和touchend就不会被触发。 解决办法当然是在onTouchBegin中进行preventDefault(), 然而这样click事件(点击关闭全屏啊!)就不会被触发了:
function onTouchBegin(e) { e.preventDefault(); }
所以我们需要在onTouchMove中来判断这是否是一个Click,并手动触发它的行为。
function onTouchMove(e){ if(isClick()) onClick(); function isClick() { var deltaT = endTime - beginTime; var deltaX = Math.abs(translateX); // 时间很短,并且移动距离很小,那么应该是个点击! return deltaT < 700 && deltaX < 7; } }
图片渐进载入
当网速很慢时,连续划动就可能使得旧的图片显示出来(因为预取请求仍未返回)。 常见的一个实践是:立即使用一个已经载入的图片来作为Placeholder, 当目标图片载入后用它替换掉当前的Placeholder。
function loadImage($img, src){ // 先设置一个Placeholder $img.attr('src', 'data:image/gif;base64,R0lGODlhAQABAAAAACH5BAEKAAEALAAAAAABAAEAAAICTAEAOw=='); // 载入图片到临时变量 var tmp = new Image(); tmp.onload = function(){ // 资源载入后,将资源显示到目标的img $img.src = src; }; tmp.src = src; }
设置背景图与设置src属性一样,均可以使用该策略。浏览器会复用那个资源。
图片到底提示
在第一张图片右划和最后一张图片左划时,应当给出提示。 可以做一张带有提示信息的Placeholder:
$lightbox.attr('style', 'top:0;left:0;right:0;bottom:0;'); $lightbox.append($('<p class="alert-nomore">').html('没有更多了..'));
然后让文字居中:
.lightbox-item .alert-nomore{ position: absolute; text-align: center; bottom: 50%; left: 0; right: 0; color: #777; font-size: 20px; }
The above is the detailed content of JS realizes full-screen browsing of pictures with unlimited swiping. For more information, please follow other related articles on the PHP Chinese website!
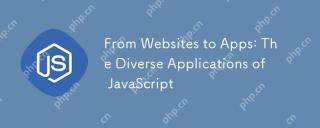
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
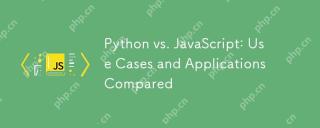
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
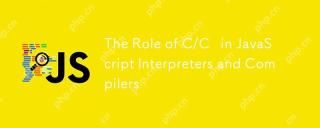
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
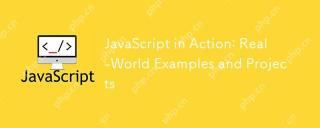
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
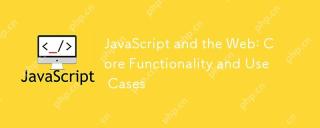
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
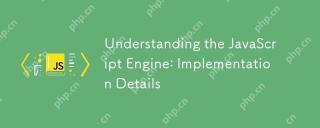
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
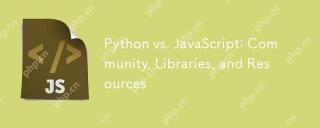
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
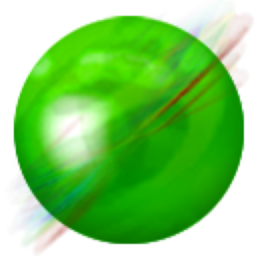
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
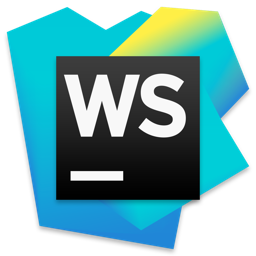
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor