


Detailed explanation of Spring, Spring MVC, MyBatis integration file configuration
I have done several small projects using the SSM framework, and it feels good that it is time to summarize. Let’s first summarize the file configuration of SSM integration. In fact, it is best to read the official documentation for specific usage.
Spring: http://spring.io/docs
MyBatis: http://mybatis.github.io/mybatis-3/
I won’t go into the basic organizational structure and usage. The previous blogs and official documents are very comprehensive. Jar packages can be organized and managed using Maven. Let’s look at the configuration file.
Web.xml configuration
web.xml should be the most important configuration file of the entire project, but servlet3.0 already supports annotation configuration. Before servlet3.0, each servlet must configure the servlet and its mapping relationship in web.xml. But it is not necessary in the spring framework, because Spring is Dependency Injection, also called Inversion of Control. But you also need to configure an important servlet, which is the front-end controller (DispatcherServlet). The configuration method is basically similar to an ordinary servlet.
The configuration content is as follows:
<!-- 配置前端控制器 --> <servlet> <servlet-name>spring</servlet-name> <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> <init-param> <!-- ContextconfigLocation配置springmvc加载的配置文件 适配器、处理映射器等 --> <param-name>contextConfigLocation</param-name> <param-value>WEB-INF/classes/spring/springmvc.xml</param-value> </init-param> </servlet> <servlet-mapping> <servlet-name>spring</servlet-name> <!-- 1、.action访问以.action结尾的 由DispatcherServlet进行解析 2、/,所有访问都由DispatcherServlet进行解析 --> <url-pattern>/</url-pattern> </servlet-mapping>
It should be noted here that springmvc.xml is the spring configuration file, which will be discussed later. If the URL in
Configure spring container:
<context-param> <param-name>contextConfigLocation</param-name> <param-value>WEB-INF/classes/spring/applicationContext-*.xml</param-value> </context-param>
Among them, applicationContext-*.xml contains 3 configuration files, which are the specific configurations of the springIoC container. Will be mentioned later.
Configure a listener:
<listener> <listener-class>org.springframework.web.context.ContextLoaderListener</listener-class> </listener>
The complete configuration of web.xml is as follows:
404 /error404.jsp 500 /error500.jsp contextConfigLocation WEB-INF/classes/spring/applicationContext-*.xml <!-- 配置前端控制器 --> <servlet> <servlet-name>spring</servlet-name> <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> <init-param> <!-- ContextconfigLocation配置springmvc加载的配置文件 适配器、处理映射器等 --> <param-name>contextConfigLocation</param-name> <param-value>WEB-INF/classes/spring/springmvc.xml</param-value> </init-param> </servlet> <servlet-mapping> <servlet-name>spring</servlet-name> <!-- 1、.action访问以.action结尾的 由DispatcherServlet进行解析 2、/,所有访问都由DispatcherServlet进行解析 --> <url-pattern>/</url-pattern> </servlet-mapping> org.springframework.web.context.ContextLoaderListener CharacterEncodingFilter org.springframework.web.filter.CharacterEncodingFilter encoding utf-8 CharacterEncodingFilter /* welcome.jsp
See that there are two more pieces of content in the configuration file. One is the error page, which is used to handle errors in a friendly manner. You can use the error code to distinguish and jump to the corresponding processing page. This configuration code is best placed at the front and processed before the front-end controller intercepts it.
Another piece of content is a filter to solve the post garbled problem. It intercepts post requests and encodes them into utf8.
Configuration of springmvc.xml View resolver configuration:
<!-- 配置视图解析器 --> <bean> <!-- 使用前缀和后缀 --> <property></property> <property></property> </bean>When setting the view name in the Controller, the prefix and suffix will be automatically added. Controller configuration Automatic scanning mode scans all Controllers under the package, and you can use annotations to specify the access path.
<!-- 使用组件扫描的方式可以一次扫描多个Controller --> <component-scan></component-scan>You can also use a single configuration method, and you need to specify the fully qualified name of the Controller.
<bean></bean>Configure the annotated processor adapter and processor mapper:
<bean></bean> <bean></bean>You can also use the following simplified configuration:
<!-- 配置注解的处理器映射器和处理器适配器 --> <annotation-driven></annotation-driven>Configure the interceptor, you can directly define to intercept all requests, or you can customize the interception path.
Configure global exception handler<bean></bean>
<bean></bean>Configure the file upload data parser, which needs to be configured when uploading files.
<!--配置上传文件数据解析器 --> <bean> <property> <value>9242880</value> </property> </bean>You can also configure some custom parameter types, taking date type binding as an example.
As mentioned above, if all requests are intercepted when configuring the front-end controller, some static resources will become unusable without special processing. If this is the case, you can use the following configuration to access static resource files.<bean></bean>
<resources></resources> <resources></resources> <resources></resources> <resources></resources>You can also use the default, but it needs to be configured in web.xml.
<!-- 访问静态资源文件 --> <!-- <mvc:default-servlet-handler/> 需要在web.xml中配置-->You can avoid this problem by not intercepting all paths. The complete configuration is probably like this. You need to pay attention to the namespace of the xml file, which sometimes has an impact.
<!-- 访问静态资源文件 --> <!-- <mvc:default-servlet-handler/> 需要在web.xml中配置--> <bean></bean>
<bean></bean> <!--配置上传文件数据解析器 --> <bean> <property> <value>9242880</value> </property> </bean> <bean></bean>
ApplicationContext-*.xml configuration
applicationContext-*.xml包括三个配置文件,分别对应数据层控制、业务逻辑service控制和事务的控制。
数据访问层的控制,applicationContext-dao.xml的配置:
配置加载数据连接资源文件的配置,把数据库连接数据抽取到一个properties资源文件中方便管理。
配置为:
<!-- 加载数据库连接的资源文件 --> <property-placeholder></property-placeholder>
其中jdbc.properties文件的内容如下:
jdbc.driver=com.mysql.jdbc.Driver jdbc.url=jdbc:mysql://localhost:3306/database jdbc.username=root jdbc.password=1234
配置数据库连接池,这里使用的是dbcp,别忘了添加jar包!
<!-- 配置数据源 dbcp数据库连接池 --> <bean> <property></property> <property></property> <property></property> <property></property> </bean>
Spring和MyBatis整合配置,jar包由MyBatis提供。
配置sqlSessionFactory
<!-- 配置sqlSessionFactory --> <bean> <!-- 数据库连接池 --> <property></property> <!-- 加载Mybatis全局配置文件 --> <property></property> </bean>
SqlMapConfig.xml文件是MyBatis的配置文件,后面会提到。
配置Mapper扫描器,扫描mapper包下的所有mapper文件和类,要求mapper配置文件和类名需要一致。
<!-- 配置mapper扫描器 --> <bean> <!-- 扫描包路径,如果需要扫描多个包中间用半角逗号隔开 --> <property></property> <property></property> </bean>
整个applicationContext-dao.xml配置文件应该是这样的:
<!-- 加载数据库连接的资源文件 --> <property-placeholder></property-placeholder> <!-- 配置数据源 dbcp数据库连接池 --> <bean> <property></property> <property></property> <property></property> <property></property> </bean> <!-- 配置mapper扫描器 --> <bean> <!-- 扫描包路径,如果需要扫描多个包中间用半角逗号隔开 --> <property></property> <property></property> </bean>
业务逻辑控制,applicationContext-service.xml的配置:
这个文件里暂时只需要定义service的实现类即可。
<bean></bean>
事务控制,applicationContext-transaction.xml的配置
配置数据源,使用JDBC控制类。
<bean> <!-- 配置数据源 --> <property></property> </bean>
配置通知,事务控制。
<!-- 通知 --> <advice> <attributes> <!-- 传播行为 --> <method></method> <method></method> <method></method> <method></method> <method></method> <method></method> </attributes> </advice>
配置AOP切面
<!-- 配置aop --> <config> <advisor></advisor> </config>
整个事务控制的配置是这样的:
<bean> <!-- 配置数据源 --> <property></property> </bean> <!-- 配置aop --> <config> <advisor></advisor> </config>
MyBatis的配置
SqlMapConfig.xml的配置 全局setting配置这里省略,数据库连接池在spring整合文件中已经配置,具体setting配置参考官方文档。
别名的定义:
<typealiases> <!-- 批量定义别名 ,指定包名,自动扫描包中的类,别名即为类名,首字母大小写无所谓--> <package></package> </typealiases>
mapper映射文件的配置:
<mappers> <!-- 加载映射文件 --> <!-- 这里也可以使用class来加载映射文件,前提是:使用mapper代理的方法,遵循规范, 并且两个文件必须同名且在同一目录 <mapper class="com.wxisme.mybatis0100.mapper.UserMapper"/> 基于class加载,可以进行批量加载 --> <!-- 通过扫描包的方式来进行批量加载映射文件 --> <package></package> </mappers>
整个文件的配置应该是这样的:
nbsp;configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"><typealiases> <!-- 批量定义别名 ,指定包名,自动扫描包中的类,别名即为类名,首字母大小写无所谓--> <package></package> </typealiases>
具体mapper文件的配置,在使用mapper代理的方法时,命名空间需要是对应的Mapper类。
<?xml version="1.0" encoding="UTF-8" ?> nbsp;mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd" > <mapper> </mapper>
以上只是对SSM框架简单使用时的配置文件,如果需要深入使用或者需要理解其内部机理需要参考官方文档和其源代码。
The above is the detailed content of Detailed explanation of Spring, Spring MVC, MyBatis integration file configuration. For more information, please follow other related articles on the PHP Chinese website!
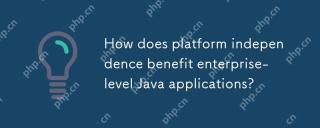
Java is widely used in enterprise-level applications because of its platform independence. 1) Platform independence is implemented through Java virtual machine (JVM), so that the code can run on any platform that supports Java. 2) It simplifies cross-platform deployment and development processes, providing greater flexibility and scalability. 3) However, it is necessary to pay attention to performance differences and third-party library compatibility and adopt best practices such as using pure Java code and cross-platform testing.
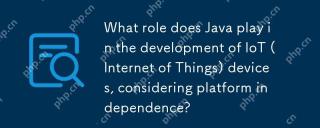
JavaplaysasignificantroleinIoTduetoitsplatformindependence.1)Itallowscodetobewrittenonceandrunonvariousdevices.2)Java'secosystemprovidesusefullibrariesforIoT.3)ItssecurityfeaturesenhanceIoTsystemsafety.However,developersmustaddressmemoryandstartuptim
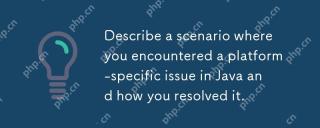
ThesolutiontohandlefilepathsacrossWindowsandLinuxinJavaistousePaths.get()fromthejava.nio.filepackage.1)UsePaths.get()withSystem.getProperty("user.dir")andtherelativepathtoconstructthefilepath.2)ConverttheresultingPathobjecttoaFileobjectifne
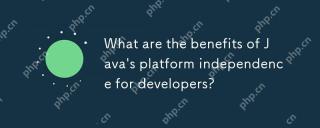
Java'splatformindependenceissignificantbecauseitallowsdeveloperstowritecodeonceandrunitonanyplatformwithaJVM.This"writeonce,runanywhere"(WORA)approachoffers:1)Cross-platformcompatibility,enablingdeploymentacrossdifferentOSwithoutissues;2)Re
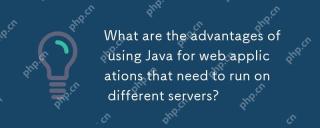
Java is suitable for developing cross-server web applications. 1) Java's "write once, run everywhere" philosophy makes its code run on any platform that supports JVM. 2) Java has a rich ecosystem, including tools such as Spring and Hibernate, to simplify the development process. 3) Java performs excellently in performance and security, providing efficient memory management and strong security guarantees.
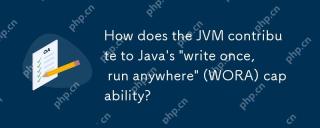
JVM implements the WORA features of Java through bytecode interpretation, platform-independent APIs and dynamic class loading: 1. Bytecode is interpreted as machine code to ensure cross-platform operation; 2. Standard API abstract operating system differences; 3. Classes are loaded dynamically at runtime to ensure consistency.
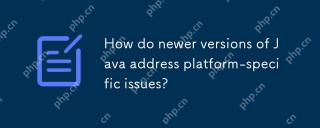
The latest version of Java effectively solves platform-specific problems through JVM optimization, standard library improvements and third-party library support. 1) JVM optimization, such as Java11's ZGC improves garbage collection performance. 2) Standard library improvements, such as Java9's module system reducing platform-related problems. 3) Third-party libraries provide platform-optimized versions, such as OpenCV.
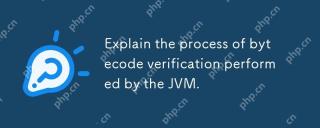
The JVM's bytecode verification process includes four key steps: 1) Check whether the class file format complies with the specifications, 2) Verify the validity and correctness of the bytecode instructions, 3) Perform data flow analysis to ensure type safety, and 4) Balancing the thoroughness and performance of verification. Through these steps, the JVM ensures that only secure, correct bytecode is executed, thereby protecting the integrity and security of the program.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
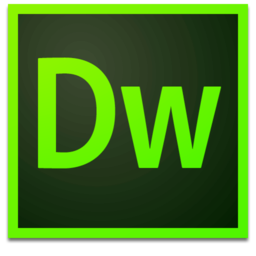
Dreamweaver Mac version
Visual web development tools
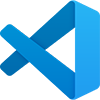
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
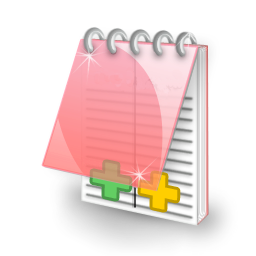
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Atom editor mac version download
The most popular open source editor
