Detailed introduction to Java reflection to obtain class and object information
Reflection can solve the problem that it is impossible to predict at compile time which objectand class it belongs to, and the information of the object and class can only be known based on the information when the program is running.
When two people collaborate on development, you only need to know the other party's class name to carry out preliminary development.
Get the class object
Class.forName(String clazzName) static method
Call the class attribute of the class, Person. class returns the Person class object (recommended)
Call the getClass() method of an object
Specific use You still have to choose based on actual conditions. The first method is relatively free. You only need to know a class name. It will not verify whether the class exists. The second and third methods will verify whether the class exists.
Get class information
Get class constructor
##Connstructor
getConstructor(Class>.. .parameterTypes) : Returns the public constructor with specified formal parameters of the corresponding class of this Class object
Constructor>[] getConstructors()
:Return all public constructors of the class corresponding to this Class object
- ##Constructor
[] getDeclaredConstructor(Class>...parameterTypes) :Return This class object corresponds to the constructor of the class with specified parameters, regardless of the access permission of the constructor
##Constructor>[] getDeclaredConstructors() - : Return This class object corresponds to all constructors of the class, regardless of the access rights of the constructor
Get the class member method
- Method getMethod (String name,Class>...parameterTypes)
- : Returns the public method with specified formal parameters of the corresponding class of this class object
- : Returns all public methods of the class represented by this class object
##Method getDeclaredMethod(string name,Class>...parameterTypes) : Returns the method with specified formal parameters of the corresponding class of this class object, regardless of method access permissions -
Method[] getDeclaredMethods() : Returns this The class object corresponds to all methods of the class, regardless of the access rights of the method Get the class member variables
- : Returns the public member variable of the specified name corresponding to this class object
-
Field[] getFields() : Returns the public member variable of the specified class corresponding to this class object All public member variables -
Field getDeclaredField(String name) : Returns the member variable with the specified name corresponding to this class object, regardless of member variable access permissions -
Field[] getDeclaredFields() : Returns all member variables of the class corresponding to this class object, regardless of the access rights of the member variables
##A getAnnotation(ClassannotationClass)
: Try to get the corresponding class of the class object Annotation of the specified type. If the type annotation does not exist, null is returned##<a extends annotation>A getDeclaredAnnotation(Class</a><a>annotationClass)</a>
:This It is new in Java 8. This method obtains the Annotation of the specified type that directly modifies the corresponding class of the class object. If it does not exist, it returns null##Annotation[] getAnnotations ()
: Returns all the Annotations that exist on the corresponding class that modifies the class object
Annotation[] getDeclaredAnnotations()
: Returns the class corresponding to the modified Class object All Annotations that exist on
- ##A[] getAnnotationByType(ClassannotationClass)
: The function of this method is the same as the getAnnotation introduced earlier The () method is basically similar, but since Java8 has added the repeated annotation function, you need to use this method to obtain multiple Annotations of the specified type that modify the class
##A[] getDeclaredAnnotationByType(ClassannotationClass) - : The function of this method is similar to the getDeclaredAnnotations() method introduced earlier. It is also because of the repeated annotation function of Java8. You need to use this method to obtain the direct modification of the class. Multiple Annotations of the specified type
Get the internal class of the class ##Class>[] getDeclaredClasses()
:Return all internal classes contained in the corresponding class of the class formation
Get the external class where the object of this class is located
Class> getDeclaringClass()
: Returns the external class where the corresponding class of the Class object is located
Gets the interface implemented by the corresponding class of the class object
Class>[] getInterfaces()
: Returns all interfaces implemented by the corresponding class of the Class object
Get the parent class inherited by the corresponding class of this class object
##Class super T> getSuperclass()
: Return the The Class object of the super class corresponding to the Class object
int getModifiers()
: Returns all modifiers of this class or interface. The modifiers are composed of public, protected, private, final, static, abstract and other corresponding constants. The returned
integerYou should use the method of the Modifier tool class to decode to get the real modifierPackage getPackage()
: Get the package of this class
String getName()
: Returns the short name of the class represented by this CLass object in the form of
string
boolean isAnnotation()
: Returns whether this class object represents an annotation type
boolean isAnnotationPresent(Class extends Annotation>annotationClass)
: Determine whether this Class object is decorated with class Annotation
boolean isAnonymousClass()
: Returns whether this class object is an
anonymous class- ##boolean isArray()
: Returns this class object Whether it represents an
array
class - boolean isEnum()
: Returns whether this class object represents an enumeration
- boolean isInterface()
: Returns whether this class object represents an interface
##boolean isInstance( - Object
obj )
: Determine whether obj is an instance of this class object. This method can completely replace the instanceof<a href="http://www.php.cn/wiki/60.html" target="_blank"> operator</a>
public interface Colorable { public void value(); }
public class ClassInfo { public static void main(String[] args) throws NoSuchMethodException, SecurityException { Class<Colorable> cls=Colorable.class; System.out.println(cls.getMethod("value")); System.out.println(cls.isAnnotation()); System.out.println(cls.isInterface()); } }
result
public abstract void com.em.Colorable.value() false true
New method parameter reflection in Java8
- int getParameterCount()
- : Get the number of formal parameters of the constructor or method
- : Get all formal parameters of the constructor or method
- : Get the modifier that modifies the formal parameter
- : Get the formal parameter name
- : Get the formal parameter type with generics
- : Get the formal parameter type Parameter type
- : This method returns whether the class file of the class contains the formal parameter name information of the method
- : This method is used to determine whether the parameter is a variable number of formal parameters
public class Test { public void getInfo(String str,List<String>list){ System.out.println("成功"); } }
public class ClassInfo { public static void main(String[] args) throws NoSuchMethodException, SecurityException { Class<Test> cls=Test.class; Method med=cls.getMethod("getInfo", String.class,List.class); System.out.println(med.getParameterCount()); Parameter[] params=med.getParameters(); System.out.println(params.length); for(Parameter par:params){ System.out.println(par.getName()); System.out.println(par.getType()); System.out.println(par.getParameterizedType()); } } }
Result
2 2 arg0 class java.lang.String class java.lang.String arg1 interface java.util.List java.util.List<java.lang.String>
Reflection generated object
Use the newInstance() method of the Class object to create an instance of the Class object. This method requires a default constructor (more commonly used) - First use the Class object to obtain the specified Constructor object, and then call the newInstance() method of the Constructor object to create an instance of the corresponding class of the Class object
- Reflection calling method
- Object invoke(Object obj,Object...args)
- : obj in this method is the main call to execute the method, followed by The args are the actual parameters passed into the method when executing the method
public class Test { public Test(String str) { System.out.println(str); } public void getInfo(String str){ System.out.println(str); } }
public class ClassInfo { public static void main(String[] args) throws Exception { Class<Test> cls=Test.class; Constructor<Test>construct=cls.getConstructor(String.class); Test test=construct.newInstance("初始化"); Method med=cls.getMethod("getInfo", String.class); med.invoke(test, "调用方法成功"); } }
Result
初始化 调用方法成功
Next, the official will take a closer look at the chestnut below
public class Test { public Test(String str) { System.out.println(str); } //私有方法 private void getInfo(String str){ System.out.println(str); } }
public class ClassInfo { public static void main(String[] args) throws Exception { Class<Test> cls=Test.class; Constructor<Test>construct=cls.getConstructor(String.class); Test test=construct.newInstance("初始化"); //为啥使用这个方法呢? Method med=cls.getDeclaredMethod("getInfo", String.class); //为啥使用这个方法呢? med.setAccessible(true); med.invoke(test, "调用方法成功"); } }
Result
初始化 调用方法成功
setAccessible(boolean flag): Set the value to true, indicating that the Java language access permission check should be canceled when using this Method
Accessing member variable values
- getXxx(Object obj)
- : Get the value of the member variable of the obj object. Xxx here corresponds to 8 basic types. If the type of the member variable is setXxx(Object obj ,Xxx val)
- : Set the member variable of the obj object to the val value. The Xxx here corresponds to the 8 basic types. If the type of the member variable is a reference type, cancel the Xxx after set
public class Test { private int num; public Test(String str) { System.out.println(str); } private void getInfo(String str){ System.out.println(str); } public int getNum() { return num; } public void setNum(int num) { this.num = num; } }public class ClassInfo { public static void main(String[] args) throws Exception { Class<Test> cls=Test.class; Constructor<Test>construct=cls.getConstructor(String.class); Test test=construct.newInstance("初始化"); Method med=cls.getDeclaredMethod("getInfo", String.class); med.setAccessible(true); med.invoke(test, "调用方法成功"); Field fld=cls.getDeclaredField("num"); fld.setAccessible(true); fld.setInt(test, 12); System.out.println(fld.getInt(test)); } }Result
初始化 调用方法成功 12
Operation array
There is an Array under the java.lang.reflect package Class, which can dynamically create arrays
static Object newInstance(Class>componentType,int...length)
: Create a new array with the specified element type and specified dimensionsstatic xxx getXxx(Object array,int index)
:返回array数组中第index个元素。其中xxx是各种基本数据类型,如果数组元素是引用类型,则该方法变为get()
static void setXxx(Object array,int index,xxx val)
:将array数组中低index 个元素的值设为val,其中xxx是各种基本数据类型,如果数组元素是引用类型,则该方法变为set()
public class ArrayInfo { public static void main(String[] args) { Object arrays=Array.newInstance(String.class, 3); Array.set(arrays, 0, "第一个"); Array.set(arrays, 1, "第二个"); Array.set(arrays, 2, "第三个"); System.out.println(Array.get(arrays, 2)); } }
The above is the detailed content of Detailed introduction to Java reflection to obtain class and object information. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
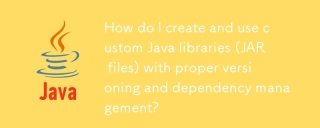
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
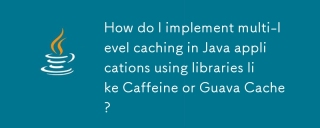
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
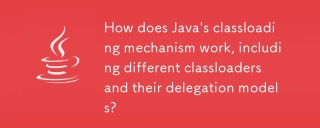
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
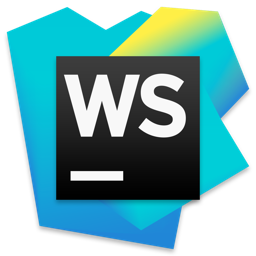
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
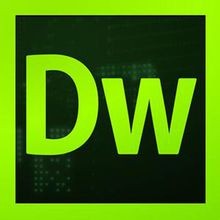
Dreamweaver CS6
Visual web development tools