


Detailed explanation of ten design principles for Java programmers to be familiar with object-oriented
This article mainly introduces in detail the 10 Object-oriented design principles that Java programmers should know. It has certain reference value. Interested friends can refer to it
Object-oriented design principles are the core of OOPS programming, but most Java programmers I have seen are enthusiastic about design patterns such as Singleton (single case), Decorator (decorator), Observer (observer), etc., but do not put enough More attention is placed on learning object-oriented analysis and design. It's important to learn the basics of object-oriented programming like "abstraction", "encapsulation", "polymorphism", and "inheritance", but it's equally important to understand these design principles in order to create concise, modular designs. I often see Java programmers of varying experience levels who either don’t know these OOPS and SOLID design principles, or they just don’t know the benefits of a particular design principle, or even how to use them in coding. Design Principles.
(Design Principles) The bottom line is to always pursue coding or design with high cohesion and low coupling. Apache and Sun's open source code are good examples for learning Java and OOPS design principles. They show us how design principles are used in Java programming. Java JDK uses some design principles: factory pattern in the BorderFactory class, singleton pattern in the Runtime class, and decorator pattern in the java.io class. By the way, if you are really interested in Java coding principles, read Effective Java by Joshua Bloch, who wrote the Java API. My personal favorite about object-oriented design patterns is Kathy Sierra's Head First Design Pattern, as well as other books about object-oriented analysis and design in simple terms. These books are a great help in writing better code that takes full advantage of various object-oriented and SOLID design patterns.
Although the best way to learn design patterns (principles) is through real-life examples and understanding the inconveniences caused by violating design principles, the purpose of this article is to educate Java programmers who have not been exposed to or are in the learning stage. An introduction to object-oriented design principles. I personally think that OOPS and SOLID design principles need an article to clearly introduce them. I will try my best to do this here, but now please prepare to browse the following design patterns (principles) :)
DRY – Don't repeat yourself
Our first object-oriented design principle is: DRY. As can be seen from the name, DRY (don't repeat yourself) means not to write repeated code, but to abstract it into reusable code blocks. If you have two or more identical blocks of code, consider abstracting them into a separate method; or if you use hard-coded values multiple times, make them public constants. The advantage of this object-oriented design principle is ease of maintenance. It is important not to abuse this principle, duplication is not about code but functionality. What it means is that if you use a common code to verify the OrderID and SSN, it does not mean that they are the same or that they will remain the same going forward. By using common code to implement two different functions, or you tie these two different functions closely together; when your OrderID format changes, your SSN verification code will break. So be careful about this coupling, and don't combine similar code that has nothing to do with each other.
Encapsulate frequently modified code
Encapsulate What Changes
What never changes in the software field is "change", so put what you think or suspect Encapsulate code that will be modified in the future. The advantage of this object-oriented design pattern is that it is easy to test and maintain properly encapsulated code. If you are programming in Java, please abide by the following principles: The access permissions of variables and methods are set to private by default, and their access permissions are gradually released, such as from "private" to "protected", " not public". Some design patterns in Java use encapsulation. The factory design pattern is an example. It encapsulates the code for creating objects and provides the following flexibility: subsequent generation of new objects does not affect existing code.
Open/Close Design PrincipleOpenClosed Design Principle
Classes, methods/functions should be open to extensions (new functions) and closed to modifications . This is another elegant SOLID design principle to prevent someone from modifying the code that passes the test. Ideally if you add new functionality, your code will be tested, which is the goal of the on/off design principle. By the way, the letter "O" in SOLID refers to the open/closed design principle.
Single Responsibility PrincipleSingle Responsibility Principle(SRP)
The single responsibility principle is another SOLID design principle, and the letter "S" in SOLID refers to it. According to SRP, there should be one and only one reason for a class modification, or a class should always implement a single function. If a class in Java implements multiple functions, a coupling relationship is created between these functions; if you modify one of the functions, you may break the coupling relationship, and then another Round testing to avoid new problems.
Dependency Injection/Inversion principle
Dependency Injection or Inversion principle
Don’t ask what the framework’s dependency injection function will be What benefits does it bring to you? The dependency injection function has been well implemented in the spring framework. The elegance of this design principle is that any class injected by the DI framework can be easily tested with mock objects, and it is easier to Maintenance, because the code that creates objects is centralized within the framework and is isolated from client code. There are many ways to implement dependency injection, such as using bytecode tools, some AOP (aspect-oriented programming) frameworks such as pointcut expressions or proxies used in spring. To learn more about this SOLID design principle, see the examples in the IOC and DI design patterns. The letter "D" in SOLID refers to this design principle.
Prefer composition over inheritance
Favor Composition over Inheritance
If possible, give preference to composition over inheritance. Some of you may argue about this, but I find composition to be more flexible than inheritance. Composition allows you to modify the behavior of a class at runtime by setting properties. By using polymorphism, you can implement the composition relationship between classes in the form of an interface, and provide flexibility for modifying the composition relationship. Even Effective Java recommends using composition over inheritance.
Liskov Substitution Principle
Liskov Substitution Principle LSP
According to the Liskov Substitution Principle, the place where the parent class appears can be replaced with a subclass. For example, there should be no problem if a parent class method or function is replaced by a subclass object. LSP is closely related to the single responsibility principle and the interface isolation principle. If a parent class has more functionality than its subclasses, it may not support this functionality and violate LSP design principles. In order to follow the LSP SOLID design principles, derived classes or subclasses (relative to the parent class) must enhance functionality rather than reduce it. The letter "L" in SOLID refers to the LSP design principle.
Interface isolation principle
The interface isolation principle means that if the function of an interface is not needed, then do not implement this interface. This mostly happens when an interface contains multiple functions, but the implementing class only needs one of them. Interface design is a tricky business because once an interface is published, you cannot modify it without affecting the classes that implement it. Another benefit of this design principle in Java is that interfaces have a characteristic that all methods of the interface must be implemented before any class can use it, so using a single-function interface means implementing fewer methods.
Programming is centered on interfaces (rather than implementation objects)
Programming is always centered on interfaces (rather than implementation objects), which makes the structure of the code flexible , and any new interface implementation object is compatible with the existing code structure. Therefore, in Java, please use interfaces for the data types of variables, method return values, and method parameters. This is the advice of many Java programmers, as is the advice of books like Effective Java and head first design patterns.
Agency Principle
Don’t expect one class to complete all functions. You can appropriately delegate some functions to the proxy class for implementation. Examples of the proxy principle are: equals() and hashCode() methods in Java. To compare whether the contents of two objects are the same, we let the comparison classes themselves do the comparison work instead of their callers. The benefits of this design principle are: there is no duplication of coding and it is easy to modify the behavior of the class.
Summary
All the above object-oriented design principles can help you write flexible and elegant code: a code structure with high cohesion and low coupling. Theory is only the first step, it is more important for us to acquire the ability to discover when to use these design principles. To find out whether we have violated any design principles and affected the flexibility of the code, but nothing in the world is perfect. We cannot always use design patterns and design principles when solving problems. They are mostly used for projects with long maintenance cycles. Large enterprise projects.
The above is the detailed content of Detailed explanation of ten design principles for Java programmers to be familiar with object-oriented. For more information, please follow other related articles on the PHP Chinese website!
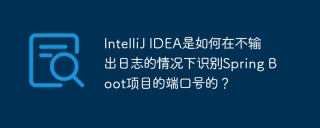
Start Spring using IntelliJIDEAUltimate version...
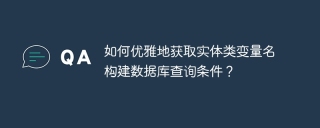
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
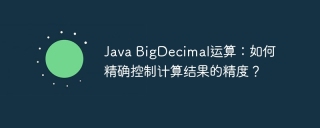
Java...
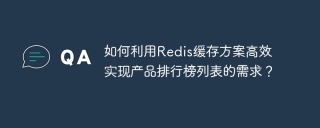
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
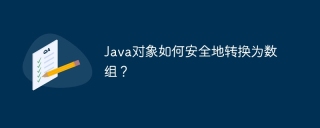
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
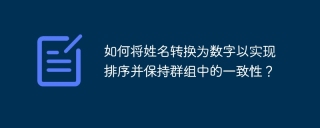
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
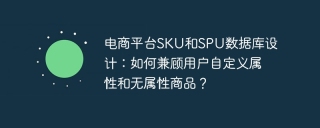
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
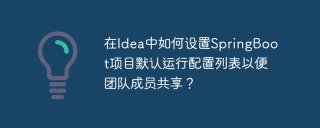
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use
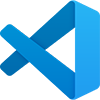
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software