Understanding the prototype
The prototype is an object through which other objects can implement property inheritance. Any object can become an inheritance. All objects have a prototype by default. Because the prototype itself is also an object, each prototype itself has a prototype. Any object has a prototype attribute, recorded as: __proto__. Whenever we define an object, its __proto__ attribute points to its prototype. An example is as follows:
var foo = { x: 10, y: 20 };
This attribute will be reserved even if we do not specify prototype. If we have a clear pointer, then the linked list will be connected. It should be noted that prototype itself also has a pointer, which is the most advanced object.prototype. An example is as follows:
var a = { x: 10, calculate: function (z) { return this.x + this.y + z } }; var b = { y: 20, __proto__: a }; var c = { y: 30, __proto__: a }; // call the inherited method b.calculate(30); // 60
Using prototypes
After understanding the principles of prototypes, how to use prototypes? In other words, what is the role of prototypes?
General beginners, after just learning the basic javascript syntax, use function-oriented programming. The following code:
var decimalDigits = 2, tax = 5; function add(x, y) { return x + y; } function subtract(x, y) { return x - y; } //alert(add(1, 3));
Get the final result by executing each function. But using prototypes, we can optimize some of our code, using constructor:
First, only variables are stored in the function body:
var Calculator = function (decimalDigits, tax) { this.decimalDigits = decimalDigits; this.tax = tax; };
The specific method is through prototype Properties to set:
Calculator.prototype = { add: function (x, y) { return x + y; }, subtract: function (x, y) { return x - y; } }; //alert((new Calculator()).add(1, 3));
In this way, you can perform corresponding function operations by instantiating the object. This is also the method used by general js frameworks.
Another function of the prototype is to implement inheritance. First, define the parent object:
var BaseCalculator = function() { this.decimalDigits = 2; }; BaseCalculator.prototype = { add: function(x, y) { return x + y; }, subtract: function(x, y) { return x - y; } };
Then define the child object and point the prototype of the child object to the instantiation of the parent element:
var Calculator = function () { //为每个实例都声明一个税收数字 this.tax = 5; }; Calculator.prototype = new BaseCalculator();
We can see that the prototype of Calculator points to the one of BaseCalculator On the instance, the purpose is to let Calculator integrate its two functions of add(x,y) and subtract(x,y). Another point to mention is that since its prototype is an instance of BaseCalculator, no matter you create How many Calculator object instances, their prototypes point to the same instance.
After running the above code, we can see that because the prototype of Calculator points to the instance of BaseCalculator, its decimalDigits attribute value can be accessed. Then if I don’t want Calculator to access the constructor of BaseCalculator Declared attribute value, what should I do? Just point Calculator to the prototype of BaseCalculator instead of the instance. The code is as follows:
var Calculator = function () { this.tax= 5; }; Calculator.prototype = BaseCalculator.prototype;
When using third-party libraries, sometimes the prototype methods they define cannot meet our needs, so we can add some methods ourselves. The code is as follows:
//覆盖前面Calculator的add() function Calculator.prototype.add = function (x, y) { return x + y + this.tax; }; var calc = new Calculator(); alert(calc.add(1, 1));
Prototype Chain
The object's prototype points to the object's parent, and the parent's prototype points to the parent's parent. This prototype-level relationship is called Prototype Chain.
When looking for the properties of an object, JavaScript will traverse the prototype chain upward until it finds the property with the given name. When the search reaches the top of the prototype chain, that is, Object.prototype, the specified property is still not found. Property will return undefined.
The example is as follows:
function foo() { this.add = function (x, y) { return x + y; } } foo.prototype.add = function (x, y) { return x + y + 10; } Object.prototype.subtract = function (x, y) { return x - y; } var f = new foo(); alert(f.add(1, 2)); //结果是3,而不是13 alert(f.subtract(1, 2)); //结果是-1
We can find that subtrace follows the principle of looking upward, while add has an accident. The reason is that when searching for the attribute, it first searches for its own attributes, and if not, then searches for the prototype .
Speaking of Object.prototype, we have to mention one of its methods, hasOwnProperty. It can determine whether an object contains custom properties rather than properties on the prototype chain. It is the only function in JavaScript that handles properties but does not look up the prototype chain. The usage code is as follows:
// 修改Object.prototype Object.prototype.bar = 1; var foo = {goo: undefined}; foo.bar; // 1 'bar' in foo; // true foo.hasOwnProperty('bar'); // false foo.hasOwnProperty('goo'); // true
In order to determine the relationship between the prototype object and an instance, the isPrototyleOf method has to be introduced. The demonstration is as follows:
alert(Cat.prototype.isPrototypeOf(cat2)); //true
The above is the detailed content of In-depth understanding of JavaScript prototypes (picture). For more information, please follow other related articles on the PHP Chinese website!
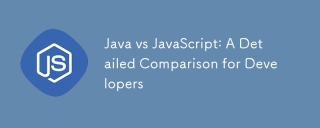
JavaandJavaScriptaredistinctlanguages:Javaisusedforenterpriseandmobileapps,whileJavaScriptisforinteractivewebpages.1)Javaiscompiled,staticallytyped,andrunsonJVM.2)JavaScriptisinterpreted,dynamicallytyped,andrunsinbrowsersorNode.js.3)JavausesOOPwithcl
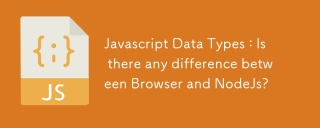
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
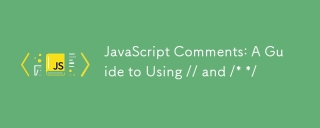
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
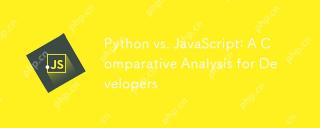
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
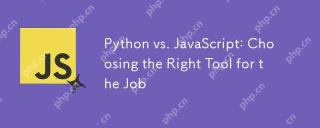
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
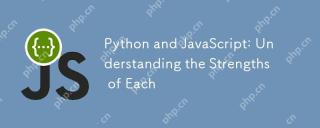
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
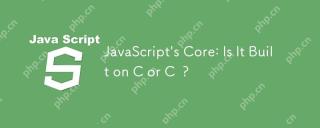
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
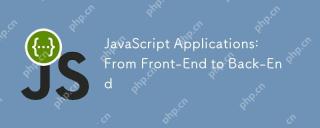
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
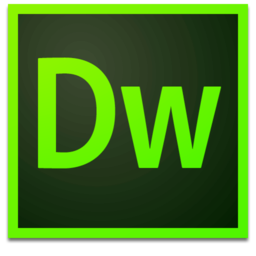
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!
