


Detailed introduction to 10 practical code snippets frequently used by C# programmers
10 practical code snippets often used by C# programmers are introduced in detail:
1 Read the version of the operating system and CLR
OperatingSystem os = System.Environment.OSVersion; Console.WriteLine(“Platform: {0}”, os.Platform); Console.WriteLine(“Service Pack: {0}”, os.ServicePack); Console.WriteLine(“Version: {0}”, os.Version); Console.WriteLine(“VersionString: {0}”, os.VersionString); Console.WriteLine(“CLR Version: {0}”, System.Environment.Version);
In my Windows 7 system, the following information is output
Platform: Win32NT
Service Pack:
Version: 6.1.7600.0
VersionString: Microsoft Windows NT 6.1.7600.0
CLR Version: 4.0.21006.1
2. Read the number of CPUs and memory capacity
The required information can be read through the interface provided by Windows Management Instrumentation (WMI).
private static UInt32 CountPhysicalProcessors() { ManagementObjectSearcher objects = new ManagementObjectSearcher( “SELECT * FROM Win32_ComputerSystem”); ManagementObjectCollection coll = objects.Get(); foreach(ManagementObject obj in coll) { return (UInt32)obj[“NumberOfProcessors”]; } return 0; } private static UInt64 CountPhysicalMemory() { ManagementObjectSearcher objects =new ManagementObjectSearcher( “SELECT * FROM Win32_PhysicalMemory”); ManagementObjectCollection coll = objects.Get(); UInt64 total = 0; foreach (ManagementObject obj in coll) { total += (UInt64)obj[“Capacity”]; } return total; }
Please add a reference to the assembly System.Management to ensure that the code can be compiled correctly.
Console.WriteLine(“Machine: {0}”, Environment.MachineName); Console.WriteLine(“# of processors (logical): {0}”, Environment.ProcessorCount); Console.WriteLine(“# of processors (physical): {0}” CountPhysicalProcessors()); Console.WriteLine(“RAM installed: {0:N0} bytes”, CountPhysicalMemory()); Console.WriteLine(“Is OS 64-bit? {0}”, Environment.Is64BitOperatingSystem); Console.WriteLine(“Is process 64-bit? {0}”, Environment.Is64BitProcess); Console.WriteLine(“Little-endian: {0}”, BitConverter.IsLittleEndian); foreach (Screen screen in System.Windows.Forms.Screen.AllScreens) { Console.WriteLine(“Screen {0}”, screen.DeviceName); Console.WriteLine(“\tPrimary {0}”, screen.Primary); Console.WriteLine(“\tBounds: {0}”, screen.Bounds); Console.WriteLine(“\tWorking Area: {0}”,screen.WorkingArea); Console.WriteLine(“\tBitsPerPixel: {0}”,screen.BitsPerPixel); }
3. Read the registry key-value pair
using (RegistryKey keyRun = Registry.LocalMachine.OpenSubKey(@”Software\Microsoft\Windows\CurrentVersion\Run”)) { foreach (string valueName in keyRun.GetValueNames()) { Console.WriteLine(“Name: {0}\tValue: {1}”, valueName, keyRun.GetValue(valueName)); } }
Please add the namespace Microsoft.Win32 to ensure that the above code can be compiled.
4 Start and stop Windows services
The practical functions provided by this API are often used to manage services in applications without having to go to the management services of the control panel.
<p style="margin-bottom: 7px;">ServiceController controller = new ServiceController(“e-M-POWER”); <br>controller.Start(); <br>if (controller.CanPauseAndContinue) <br>{ <br> controller.Pause(); <br> controller.Continue(); <br>} <br>controller.Stop();<br></p>
In the API provided by .net, you can install and uninstall services in one sentence
if (args[0] == "/i") { ManagedInstallerClass.InstallHelper(new string[] { Assembly.GetExecutingAssembly().Location }); } else if (args[0] == "/u") { ManagedInstallerClass.InstallHelper(new string[] { "/u", Assembly.GetExecutingAssembly().Location }); }
As shown in the code, pass the i or u parameter to the application to indicate whether to uninstall or install the program.
5. Verify whether the program has a strong name (P/Invoke)
For example, in a program, in order to verify whether the assembly is signed, the following method can be called
[DllImport("mscoree.dll", CharSet=CharSet.Unicode)] static extern bool StrongNameSignatureVerificationEx(string wszFilePath, bool fForceVerification, ref bool pfWasVerified); bool notForced = false; bool verified = StrongNameSignatureVerificationEx(assembly, false, ref notForced); Console.WriteLine("Verified: {0}\nForced: {1}", verified, !notForced);
This function is commonly used in software protection methods and can be used to verify signed components. Even if your signature is removed, or the signatures of all assemblies are removed, as long as there is this calling code in the program, the program can be stopped from running.
6. Respond to changes in system configuration items
For example, after we lock the system, if QQ does not exit, it will show a busy status.
Please add the namespace Microsoft.Win32, and then register the following events.
. DisplaySettingsChanged (including Changing) Display Settings
. InstalledFontsChanged Font changes
.PaletteChanged
. PowerModeChanged power status
.SessionEnded (The user is logging out or the session has ended)
.SessionSwitch (Change current user)
. TimeChanged Time change
. UserPreferenceChanged (user preference number includes Changing)
Our ERP system will monitor whether the system time has changed. If the time is adjusted outside the range of the ERP license file, the ERP software will become unavailable.
7. Use the new features of Windows 7
The Windows 7 system introduces some new features, such as the Open File dialog box, and the status bar can display the progress of the current task.
Microsoft.WindowsAPICodePack.Dialogs.CommonOpenFileDialog ofd = new Microsoft.WindowsAPICodePack.Dialogs.CommonOpenFileDialog(); ofd.AddToMostRecentlyUsedList = true; ofd.IsFolderPicker = true; ofd.AllowNonFileSystemItems = true; ofd.ShowDialog();
Using this method to open a dialog box has more functions than the OpenFileDialog in BCL's own class library. However, it is limited to Windows 7 systems, so to call this code, you must also check that the version of the operating system is greater than 6, and add a reference to the assembly Windows API Code Pack for Microsoft®.NET Framework. Please go to this address to download http ://www.php.cn/
8 Check the memory consumption of the program
Use the following method to check the amount of memory allocated by .NET to the program
long available = GC.GetTotalMemory(false); Console.WriteLine(“Before allocations: {0:N0}”, available); int allocSize = 40000000; byte[] bigArray = new byte[allocSize]; available = GC.GetTotalMemory(false); Console.WriteLine(“After allocations: {0:N0}”, available);
In my system, the result of its operation is as follows
Before allocations: 651,064 After allocations: 40,690,080
Use the following method to check the memory occupied by the current application
Process proc = Process.GetCurrentProcess(); Console.WriteLine(“Process Info: “+Environment.NewLine+
“Private Memory Size: {0:N0}”+Environment.NewLine + “Virtual Memory Size: {1:N0}” + Environment.NewLine +
“Working Set Size: {2:N0}” + Environment.NewLine + “Paged Memory Size: {3:N0}” + Environment.NewLine + “Paged System Memory Size: {4:N0}” + Environment.NewLine +
“Non-paged System Memory Size: {5:N0}” + Environment.NewLine, proc.PrivateMemorySize64, proc.VirtualMemorySize64, proc.WorkingSet64, proc.PagedMemorySize64, proc.PagedSystemMemorySize64, proc.NonpagedSystemMemorySize64 );
9 Use a stopwatch to check the program running time
If you are worried that some code is very time-consuming, you can use StopWatch to check the time consumed by this code, as shown in the following code
System.Diagnostics.Stopwatch timer = new System.Diagnostics.Stopwatch(); timer.Start(); Decimal total = 0; int limit = 1000000; for (int i = 0; i < limit; ++i) { total = total + (Decimal)Math.Sqrt(i); } timer.Stop(); Console.WriteLine(“Sum of sqrts: {0}”,total); Console.WriteLine(“Elapsed milliseconds: {0}”, timer.ElapsedMilliseconds); Console.WriteLine(“Elapsed time: {0}”, timer.Elapsed);
Now there are special tools to detect the running time of the program, which can be refined to each method, such as dotNetPerformance software.
Taking the above code as an example, you need to modify the source code directly, which is somewhat inconvenient if it is used to test the program. Please refer to the example below.
class AutoStopwatch : System.Diagnostics.Stopwatch, IDisposable { public AutoStopwatch() { Start(); } public void Dispose() { Stop(); Console.WriteLine(“Elapsed: {0}”, this.Elapsed); } }
With the help of using syntax, as shown in the following code, you can check the running time of a piece of code and print it on the console.
using (new AutoStopwatch()) { Decimal total2 = 0; int limit2 = 1000000; for (int i = 0; i < limit2; ++i) { total2 = total2 + (Decimal)Math.Sqrt(i); } }
10 Use the cursor
When the program is running a save or delete operation in the background, the cursor status should be changed to busy. Use the following tips.
class AutoWaitCursor : IDisposable { private Control _target; private Cursor _prevCursor = Cursors.Default; public AutoWaitCursor(Control control) { if (control == null) { throw new ArgumentNullException(“control”); } _target = control; _prevCursor = _target.Cursor; _target.Cursor = Cursors.WaitCursor; } public void Dispose() { _target.Cursor = _prevCursor; } }
The usage is as follows. This way of writing is to anticipate that the program may throw an exception
using (new AutoWaitCursor(this)) { ... throw new Exception(); }
As shown in the code, even if an exception is thrown, the cursor can be restored to the previous state.
The above is the detailed content of Detailed introduction to 10 practical code snippets frequently used by C# programmers. For more information, please follow other related articles on the PHP Chinese website!
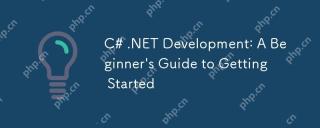
To start C#.NET development, you need to: 1. Understand the basic knowledge of C# and the core concepts of the .NET framework; 2. Master the basic concepts of variables, data types, control structures, functions and classes; 3. Learn advanced features of C#, such as LINQ and asynchronous programming; 4. Be familiar with debugging techniques and performance optimization methods for common errors. With these steps, you can gradually penetrate the world of C#.NET and write efficient applications.
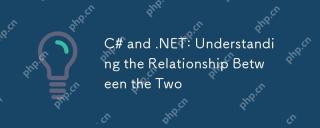
The relationship between C# and .NET is inseparable, but they are not the same thing. C# is a programming language, while .NET is a development platform. C# is used to write code, compile into .NET's intermediate language (IL), and executed by the .NET runtime (CLR).
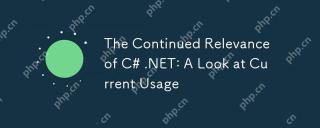
C#.NET is still important because it provides powerful tools and libraries that support multiple application development. 1) C# combines .NET framework to make development efficient and convenient. 2) C#'s type safety and garbage collection mechanism enhance its advantages. 3) .NET provides a cross-platform running environment and rich APIs, improving development flexibility.
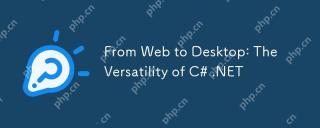
C#.NETisversatileforbothwebanddesktopdevelopment.1)Forweb,useASP.NETfordynamicapplications.2)Fordesktop,employWindowsFormsorWPFforrichinterfaces.3)UseXamarinforcross-platformdevelopment,enablingcodesharingacrossWindows,macOS,Linux,andmobiledevices.
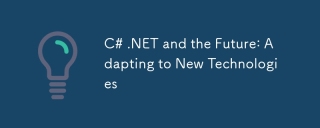
C# and .NET adapt to the needs of emerging technologies through continuous updates and optimizations. 1) C# 9.0 and .NET5 introduce record type and performance optimization. 2) .NETCore enhances cloud native and containerized support. 3) ASP.NETCore integrates with modern web technologies. 4) ML.NET supports machine learning and artificial intelligence. 5) Asynchronous programming and best practices improve performance.
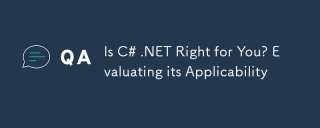
C#.NETissuitableforenterprise-levelapplicationswithintheMicrosoftecosystemduetoitsstrongtyping,richlibraries,androbustperformance.However,itmaynotbeidealforcross-platformdevelopmentorwhenrawspeediscritical,wherelanguageslikeRustorGomightbepreferable.
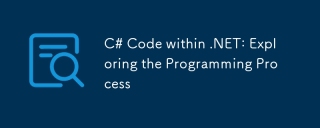
The programming process of C# in .NET includes the following steps: 1) writing C# code, 2) compiling into an intermediate language (IL), and 3) executing by the .NET runtime (CLR). The advantages of C# in .NET are its modern syntax, powerful type system and tight integration with the .NET framework, suitable for various development scenarios from desktop applications to web services.
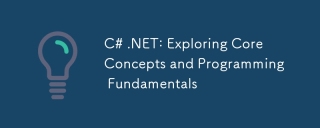
C# is a modern, object-oriented programming language developed by Microsoft and as part of the .NET framework. 1.C# supports object-oriented programming (OOP), including encapsulation, inheritance and polymorphism. 2. Asynchronous programming in C# is implemented through async and await keywords to improve application responsiveness. 3. Use LINQ to process data collections concisely. 4. Common errors include null reference exceptions and index out-of-range exceptions. Debugging skills include using a debugger and exception handling. 5. Performance optimization includes using StringBuilder and avoiding unnecessary packing and unboxing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool