Some time ago, we organized and optimized our native module API (iOS and Android modules are encapsulated into JavaScript interfaces), so I studied several articles on JavaScript API design. Although they are old articles, I benefited a lot. Record it here.
Good API design: achieve abstract goals while being self-describing.
With a well-designed API, developers can get started quickly. There is no need to hold manuals and documents frequently, and there is no need to frequently visit the technical support community.
Smooth interface
Method chain: smooth and easy to read, easier to understand
//常见的 API 调用方式:改变一些颜色,添加事件监听 var elem = document.getElementById("foobar"); elem.style.background = "red"; elem.style.color = "green"; elem.addEventListener('click', function(event) { alert("hello world!"); }, true); //(设想的)方法链 API DOMHelper.getElementById('foobar') .setStyle("background", "red") .setStyle("color", "green") .addEvent("click", function(event) { alert("hello world"); });
Set and get operations can be combined into one ;The more methods, the more difficult it may be to write the document
var $elem = jQuery("#foobar"); //setter $elem.setCss("background", "green"); //getter $elem.getCss("color") === "red"; //getter, setter 合二为一 $elem.css("background", "green"); $elem.css("color") === "red";
Consistency
Relevant interfaces maintain a consistent style. If a whole set of APIs conveys a sense of familiarity and comfort, it will Greatly ease developers' adaptability to new tools.
Name this thing: it should be short, self-descriptive, and most importantly consistent
“There are only two hard problems in computer science: cache-invalidation and naming things.”
“There are only two headaches in computer science: cache invalidations and naming problems”
—Phil Karlton
Choose a phrasing you like and continue use. Choose a style and keep it that way.
Processing parameters
You need to consider how people use the method you provide. Will it be called repeatedly? Why is it called repeatedly? How does your API help developers reduce duplicate calls?
Receive map mapping parameters, callbacks or serialized attribute names, which not only makes your API cleaner, but also makes it more comfortable and efficient to use.
jQuery’s css()
method can set styles for DOM elements:
jQuery("#some-selector") .css("background", "red") .css("color", "white") .css("font-weight", "bold") .css("padding", 10);
This method can accept a JSON object:
jQuery("#some-selector").css({ "background" : "red", "color" : "white", "font-weight" : "bold", "padding" : 10 }); //通过传一个 map 映射绑定事件 jQuery("#some-selector").on({ "click" : myClickHandler, "keyup" : myKeyupHandler, "change" : myChangeHandler }); //为多个事件绑定同一个处理函数 jQuery("#some-selector").on("click keyup change", myEventHandler);
Processing type
When defining a method, you need to decide what parameters it can receive. We don't know how people use our code, but we can be more forward-looking and consider what parameter types we support.
//原来的代码 DateInterval.prototype.days = function(start, end) { return Math.floor((end - start) / 86400000); }; //修改后的代码 DateInterval.prototype.days = function(start, end) { if (!(start instanceof Date)) { start = new Date(start); } if (!(end instanceof Date)) { end = new Date(end); } return Math.floor((end.getTime() - start.getTime()) / 86400000); };
With the addition of just 6 lines of code, our method is powerful enough to accept Date
objects, numeric timestamps, and even things like Sat Sep 08 2012 15:34: 35 GMT+0200 (CEST)
Such a string
If you need to ensure the type of the incoming parameter (string, number, Boolean), you can convert it like this:
function castaway(some_string, some_integer, some_boolean) { some_string += ""; some_integer += 0; // parseInt(some_integer, 10) 更安全些 some_boolean = !!some_boolean; }
Processing undefined
To make your API more robust, you need to identify whether the real undefined
value is passed in. You can check the arguments
object:
function testUndefined(expecting, someArgument) { if (someArgument === undefined) { console.log("someArgument 是 undefined"); } if (arguments.length > 1) { console.log("然而它实际是传进来的"); } } testUndefined("foo"); // 结果: someArgument 是 undefined testUndefined("foo", undefined); // 结果: someArgument 是 undefined , 然而它实际是传进来的
Name the parameters
event.initMouseEvent( "click", true, true, window, 123, 101, 202, 101, 202, true, false, false, false, 1, null);
Event.initMouseEvent This method is simply crazy. Without reading the documentation, who can tell what each parameter means?
Give each parameter a name and assign a default value. For example,
event.initMouseEvent( type="click", canBubble=true, cancelable=true, view=window, detail=123, screenX=101, screenY=202, clientX=101, clientY=202, ctrlKey=true, altKey=false, shiftKey=false, metaKey=false, button=1, relatedTarget=null);
ES6 or Harmony has default parameter values and rest parameters.
Parameters receive JSON objects
Instead of receiving a bunch of parameters, it is better to receive a JSON object:
function nightmare(accepts, async, beforeSend, cache, complete, /* 等28个参数 */) { if (accepts === "text") { // 准备接收纯文本 } } function dream(options) { options = options || {}; if (options.accepts === "text") { // 准备接收纯文本 } }
It is also simpler to call:
nightmare("text", true, undefined, false, undefined, /* 等28个参数 */); dream({ accepts: "text", async: true, cache: false });
Parameters Default value
It is best to have a default value for the parameters. The preset default value can be overridden through jQuery.extend() http://www.php.cn/) and Protoype's Object.extend.
var default_options = { accepts: "text", async: true, beforeSend: null, cache: false, complete: null, // … }; function dream(options) { var o = jQuery.extend({}, default_options, options || {}); console.log(o.accepts); } dream({ async: false }); // prints: "text"
Extensibility
Callbacks
Through callbacks, API users can overwrite a certain part of your code. Open some functions that require customization into configurable callback functions, allowing API users to easily override your default code.
Once the API interface receives callbacks, make sure to document them and provide code examples.
Events
It is best to know the name of the event interface. You can freely choose the event name to avoid duplication of names with native events.
Handling Errors
Not all errors are useful for developers to debug code:
// jQuery 允许这么写 $(document.body).on('click', {}); // 点击时报错 // TypeError: ((p.event.special[l.origType] || {}).handle || l.handler).apply is not a function // in jQuery.min.js on Line 3
Such errors are painful to debug. Don’t waste developers’ time. Tell them directly. What mistake did they make:
if (Object.prototype.toString.call(callback) !== '[object Function]') { // 看备注 throw new TypeError("callback is not a function!"); }
Note:
typeof callback === "function"
will have problems on old browsers,object
will Think of it as afunction
.
Predictability
A good API is predictable and developers can infer its usage based on examples.
Modernizr's feature detection is an example:
a) It uses attribute names that completely match HTML5, CSS concepts and APIs
b) Each individual detection is consistent Returning true or false values
// 所有这些属性都返回 'true' 或 'false' Modernizr.geolocation Modernizr.localstorage Modernizr.webworkers Modernizr.canvas Modernizr.borderradius Modernizr.boxshadow Modernizr.flexbox
relies on concepts that developers are already familiar with and can be predictable.
jQuery’s selector syntax is an obvious example. CSS1-CSS3 selectors can be used directly in its DOM selector engine.
$("#grid") // Selects by ID $("ul.nav > li") // All LIs for the UL with class "nav" $("ul li:nth-child(2)") // Second item in each list
Proportional coordination
A good API is not necessarily a small API. The size of the API must be commensurate with its function.
For example, Moment.js, a well-known date parsing and formatting library, can be called balanced. Its API is both concise and functional.
With a function-specific library like Moment.js, it’s important to keep the API focused and small.
Writing API Documentation
One of the most difficult tasks in software development is writing documentation. In fact, everyone hates writing documentation. The complaint is that there is no easy-to-use documentation tool.
The following are some automatic document generation tools:
YUIDoc (requires Node.js, npm)
JsDoc Toolkit ( requires Node.js, npm)
- ##Markdox (requires Node.js, npm)
- Dox (requires Node.js, npm)
- Docco (requires Node.js, Python, CoffeeScript)
- JSDuck (reqires Ruby, gem)
- JSDoc 3 (requires Java)
#The most important thing is: ensure that the documentation and code are updated simultaneously.
References:
- Good API Design
- Designing Better JavaScript APIs
- Secrets of Awesome JavaScript API Design
##
The above is the detailed content of A detailed introduction to JavaScript API design principles. For more information, please follow other related articles on the PHP Chinese website!
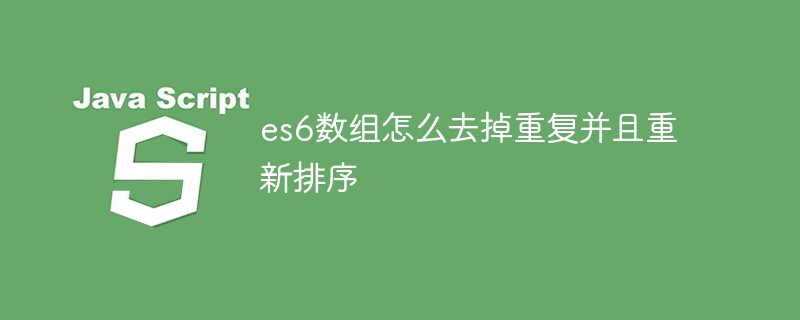
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
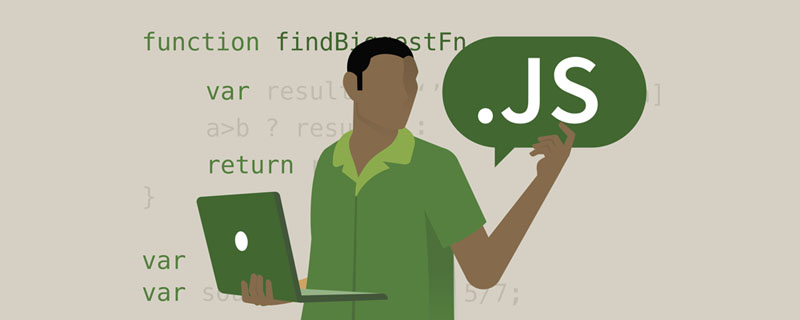
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
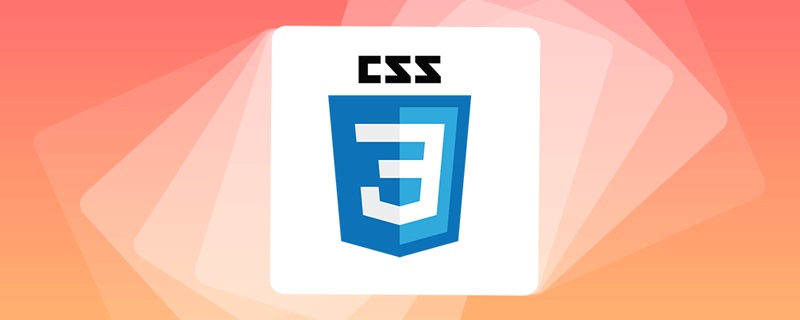
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
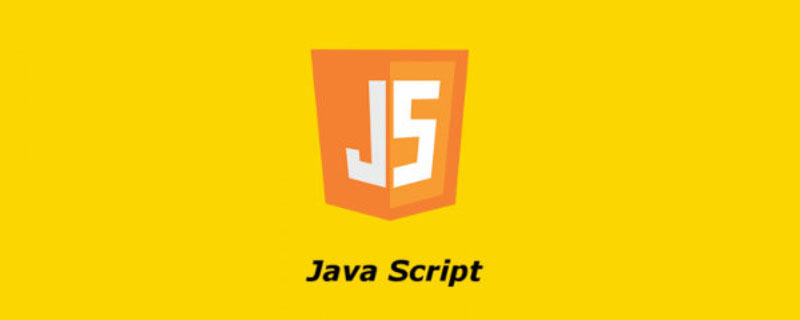
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
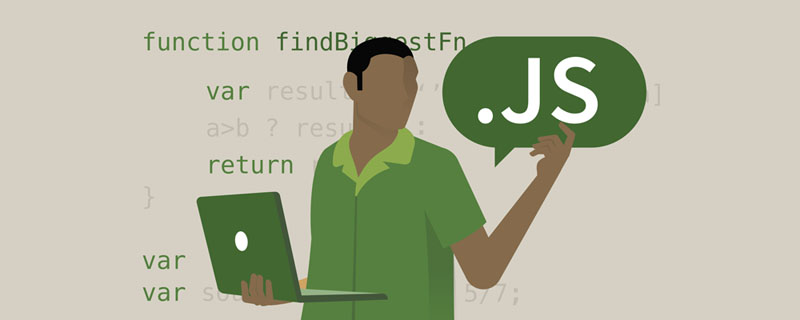
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
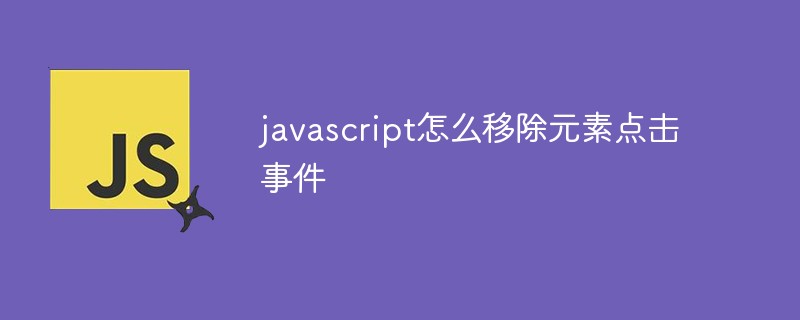
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
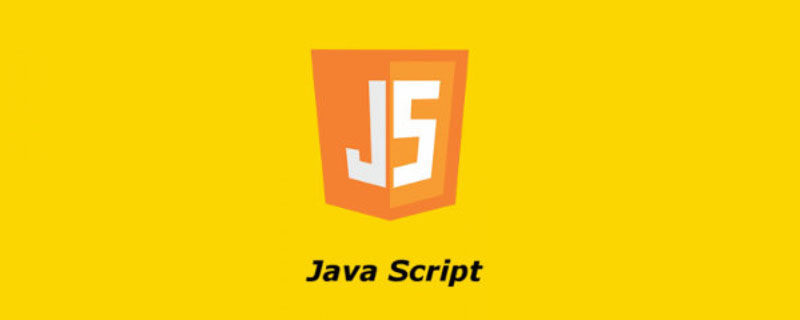
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
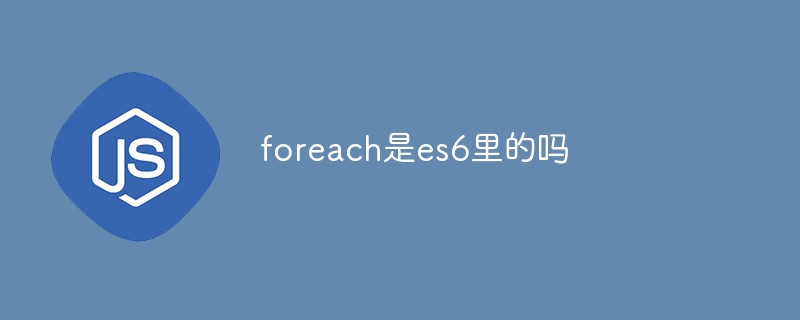
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
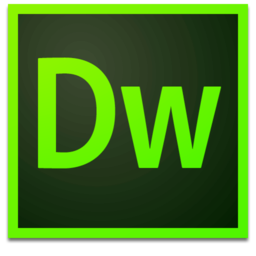
Dreamweaver Mac version
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
