


Detailed explanation of the underlying operating mechanism code of JavaScript closures
I have been studying JavaScriptclosure (closure) for some time. I had just learned how to use them without having a thorough understanding of how they actually worked. So, what exactly is a closure?
The explanation given by Wikipedia is not very helpful. When is the closure created and when is it destroyed? What is the specific implementation?
"use strict"; var myClosure = (function outerFunction() { var hidden = 1; return { inc: function innerFunction() { return hidden++; } }; }()); myClosure.inc(); // 返回 1 myClosure.inc(); // 返回 2 myClosure.inc(); // 返回 3 // 相信对JS熟悉的朋友都能很快理解这段代码 // 那么在这段代码运行的背后究竟发生了怎样的事情呢?
Now that I finally know the answer, I’m excited and decided to explain it to everyone. At least, I will definitely not forget this answer.
Tell me and I forget. Teach me and I remember. Involve me and I learn.
© Benjamin Franklin
And, when I read with closure When looking at relevant existing information, I tried very hard to think about the connection between everything in my mind: how objects are referenced, what are the inheritance relationships between objects, etc. I couldn't find a good diagram of these responsible relationships, so I decided to draw some myself.
I will assume that the reader is already familiar with JavaScript, knows what a global object is, knows that functions are "first-class objects" in JavaScript, etc.
Scope Chain
When JavaScript is running, it needs some space to store local variables. We call these spaces scope objects (Scope objects), sometimes also called LexicalEnvironment
. For example, when you call a function, the function defines some local variables, and these variables are stored in a scope object. You can think of a scope function as an ordinary JavaScript object, but one big difference is that you can't get this object directly in JavaScript. You can only modify the properties of this object, but you cannot obtain a reference to this object.
The concept of scope objects makes JavaScript very different from C and C++. In C and C++, local variables are stored on the stack. In JavaScript, scope objects are created on the heap (at least that's how it behaves), so they can still be accessed without being destroyed after the function returns.
As you can imagine, scope objects can have parent scope objects. When code attempts to access a variable, the interpreter will look for this property in the current scope object. If the property does not exist, the interpreter looks for the property in the parent scope object. In this way, the search continues to the parent scope object until the property is found or there is no more parent scope object. We ride the scope chain (Scope chain) on the scope objects passed through in the process of finding variables.
The process of finding variables in the scope chain is very similar to prototypal inheritance. However, the very different thing is that when you can't find a property in the prototype chain (prototype chain), it will not raise an error, but will get undefined
. But if you try to access a property that doesn't exist in the scope chain, you will get a ReferenceError
.
The top-level element in the scope chain is the Global Object. In JavaScript code running in the global environment, the scope chain always contains only one element, which is the global object. So, when you define variables in the global environment, they are defined in the global object. When a function is called, the scope chain will contain multiple scope objects.
Code running in the global environment
Okay, that’s it for the theory. Next let's start with the actual code.
// my_script.js "use strict"; var foo = 1; var bar = 2;
We created two variables in the global environment. As I just said, the scope object at this time is the global object.
In the above code, we have an execution context (myscript.js its own code), and the scope object it refers to . The global object also contains many different properties, which we ignore here.
Non-nested functions
Next, let’s look at this code
"use strict"; var foo = 1; var bar = 2; function myFunc() { //-- define local-to-function variables var a = 1; var b = 2; var foo = 3; console.log("inside myFunc"); } console.log("outside"); //-- and then, call it: myFunc();
When myFunc
is defined At that time, the identifier of myFunc
is added to the current scope object (in this case, the global object), and this identifier refers to a function object. The function object contains the source code of the function and other attributes. One of the properties we are concerned about is the internal property [[scope]]
. [[scope]]
points to the current scope object. That is to say, it refers to the scope object (in this case, the global object) that we can directly access when the function identifier is created.
“直接访问”的意思就是,在当前作用域链中,该作用域对象处于最底层,没有子作用域对象。
所以,在console.log("outside")
被运行之前,Detailed explanation of the underlying operating mechanism code of JavaScript closures是如下图所示。
温习一下。myFunc
所引用的函数对象其本身不仅仅含有函数的代码,并且还含有指向其被创建的时候的作用域对象。这一点非常重要!
当myFunc
函数被调用的时候,一个新的作用域对象被创建了。新的作用域对象中包含myFunc
函数所定义的本地变量,以及其参数(arguments)。这个新的作用域对象的父作用域对象就是在运行myFunc
时我们所能直接访问的那个作用域对象。
所以,当myFunc
被执行的时候,Detailed explanation of the underlying operating mechanism code of JavaScript closures如下图所示。
现在我们就拥有了一个作用域链。当我们试图在myFunc
当中访问某些变量的时候,JavaScript会先在其能直接访问的作用域对象(这里就是myFunc() scope
)当中查找这个属性。如果找不到,那么就在它的父作用域对象当中查找(在这里就是Global Object
)。如果一直往上找,找到没有父作用域对象为止还没有找到的话,那么就会抛出一个ReferenceError
。
例如,如果我们在myFunc
中要访问a
这个变量,那么在myFunc scope
当中就可以找到它,得到值为1
。
如果我们尝试访问foo
,我们就会在myFunc() scope
中得到3
。只有在myFunc() scope
里面找不到foo
的时候,JavaScript才会往Global Object
去查找。所以,这里我们不会访问到Global Object
里面的foo
。
如果我们尝试访问bar
,我们在myFunc() scope
当中找不到它,于是就会在Global Object
当中查找,因此查找到2。
很重要的是,只要这些作用域对象依然被引用,它们就不会被垃圾回收器(garbage collector)销毁,我们就一直能访问它们。当然,当引用一个作用域对象的最后一个引用被解除的时候,并不代表垃圾回收器会立刻回收它,只是它现在可以被回收了。
所以,当myFunc()
返回的时候,再也没有人引用myFunc() scope
了。当垃圾回收结束后,Detailed explanation of the underlying operating mechanism code of JavaScript closures变成回了调用前的关系。
接下来,为了图表直观起见,我将不再将函数对象画出来。但是,请永远记着,函数对象里面的[[scope]]
属性,保存着该函数被定义的时候所能够直接访问的作用域对象。
嵌套的函数(Nested functions)
正如前面所说,当一个函数返回后,没有其他对象会保存对其的引用。所以,它就可能被垃圾回收器回收。但是如果我们在函数当中定义嵌套的函数并且返回,被调用函数的一方所存储呢?(如下面的代码)
function myFunc() { return innerFunc() { // ... } } var innerFunc = myFunc();
你已经知道的是,函数对象中总是有一个[[scope]]
属性,保存着该函数被定义的时候所能够直接访问的作用域对象。所以,当我们在定义嵌套的函数的时候,这个嵌套的函数的[[scope]]
就会引用外围函数(Outer function)的当前作用域对象。
如果我们将这个嵌套函数返回,并被另外一个地方的标识符所引用的话,那么这个嵌套函数及其[[scope]]
所引用的作用域对象就不会被垃圾回收所销毁。
"use strict"; function createCounter(initial) { var counter = initial; function increment(value) { counter += value; } function get() { return counter; } return { increment: increment, get: get }; } var myCounter = createCounter(100); console.log(myCounter.get()); // 返回 100 myCounter.increment(5); console.log(myCounter.get()); // 返回 105
当我们调用createCounter(100)
的那一瞬间,Detailed explanation of the underlying operating mechanism code of JavaScript closures如下图
注意increment
和get
函数都存有指向createCounter(100) scope
的引用。如果createCounter(100)
没有任何返回值,那么createCounter(100) scope
不再被引用,于是就可以被垃圾回收。但是因为createCounter(100)
实际上是有返回值的,并且返回值被存储在了myCounter
中,所以对象之间的引用关系变成了如下图所示
所以,createCounter(100)
虽然已经返回了,但是它的作用域对象依然存在,可以且仅只能被嵌套的函数(increment
和get
)所访问。
让我们试着运行myCounter.get()
。刚才说过,函数被调用的时候会创建一个新的作用域对象,并且该作用域对象的父作用域对象会是当前可以直接访问的作用域对象。所以,当myCounter.get()
被调用时的一瞬间,Detailed explanation of the underlying operating mechanism code of JavaScript closures如下。
在myCounter.get()
运行的过程中,作用域链最底层的对象就是get() scope
,这是一个空对象。所以,当myCounter.get()
访问counter
变量时,JavaScript在get() scope
中找不到这个属性,于是就向上到createCounter(100) scope
当中查找。然后,myCounter.get()
将这个值返回。
调用myCounter.increment(5)
的时候,事情变得更有趣了,因为这个时候函数调用的时候传入了参数。
正如你所见,increment(5)
的调用创建了一个新的作用域对象,并且其中含有传入的参数value
。当这个函数尝试访问value
的时候,JavaScript立刻就能在当前的作用域对象找到它。然而,这个函数试图访问counter
的时候,JavaScript无法在当前的作用域对象找到它,于是就会在其父作用域createCounter(100) scope
中查找。
我们可以注意到,在createCounter
函数之外,除了被返回的get
和increment
两个方法,没有其他的地方可以访问到value
这个变量了。这就是用闭包实现“私有变量”的方法。
我们注意到initial
变量也被存储在createCounter()
所创建的作用域对象中,尽管它没有被用到。所以,我们实际上可以去掉var counter = initial;
,将initial
改名为counter
。但是为了代码的可读性起见,我们保留原有的代码不做变化。
需要注意的是作用域链是不会被复制的。每次函数调用只会往作用域链下面新增一个作用域对象。所以,如果在函数调用的过程当中对作用域链中的任何一个作用域对象的变量进行修改的话,那么同时作用域链中也拥有该作用域对象的函数对象也是能够访问到这个变化后的变量的。
这也就是为什么下面这个大家都很熟悉的例子会不能产出我们想要的结果。
"use strict"; var elems = document.getElementsByClassName("myClass"), i; for (i = 0; i < elems.length; i++) { elems[i].addEventListener("click", function () { this.innerHTML = i; }); }
在上面的循环中创建了多个函数对象,所有的函数对象的[[scope]]
都保存着对当前作用域对象的引用。而变量i
正好就在当前作用域链中,所以循环每次对i
的修改,对于每个函数对象都是能够看到的。
“看起来一样的”函数,不一样的作用域对象
现在我们来看一个更有趣的例子。
"use strict"; function createCounter(initial) { // ... } var myCounter1 = createCounter(100); var myCounter2 = createCounter(200);
当myCounter1
和myCounter2
被创建后,Detailed explanation of the underlying operating mechanism code of JavaScript closures为
在上面的例子中,myCounter1.increment
和myCounter2.increment
的函数对象拥有着一样的代码以及一样的属性值(name
,length
等等),但是它们的[[scope]]
指向的是不一样的作用域对象。
这才有了下面的结果
var a, b; a = myCounter1.get(); // a 等于 100 b = myCounter2.get(); // b 等于 200 myCounter1.increment(1); myCounter1.increment(2); myCounter2.increment(5); a = myCounter1.get(); // a 等于 103 b = myCounter2.get(); // b 等于 205
作用域链和this
this
的值不会被保存在作用域链中,this
的值取决于函数被调用的时候的情景。
译者注:对这部分,译者自己曾经写过一篇更加详尽的文章,请参考《用自然语言的角度理解JavaScript中的this关键字》。原文的这一部分以及“
this
在嵌套的函数中的使用”译者便不再翻译。
总结
让我们来回想我们在本文开头提到的一些问题。
什么是闭包?闭包就是同时含有对函数对象以及作用域对象引用的最想。实际上,所有JavaScript对象都是闭包。
闭包是什么时候被创建的?因为所有JavaScript对象都是闭包,因此,当你定义一个函数的时候,你就定义了一个闭包。
闭包是什么时候被销毁的?当它不被任何其他的对象引用的时候。
专有名词翻译表
本文采用下面的专有名词翻译表,如有更好的翻译请告知,尤其是加*
的翻译
*全局环境中运行的代码:top-level code
参数:arguments
作用域对象:Scope object
作用域链:Scope Chain
栈:stack
原型继承:prototypal inheritance
原型链:prototype chain
全局对象:Global Object
标识符:identifier
垃圾回收器:garbage collector
以上就是JavaScript, 闭包,运行机制的内容,更多相关内容请关注PHP中文网(www.php.cn)!
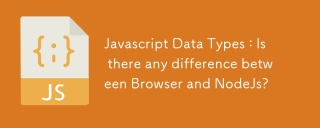
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
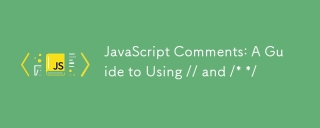
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
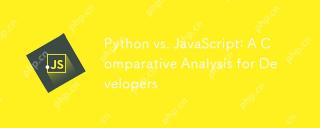
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
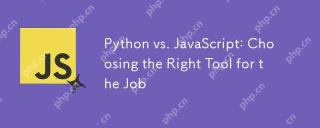
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
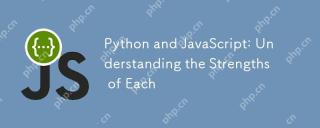
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
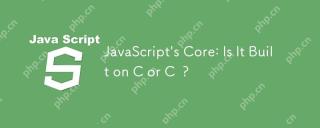
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
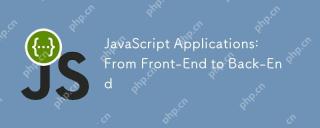
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
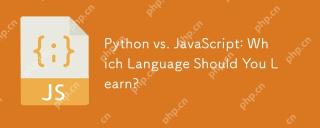
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use
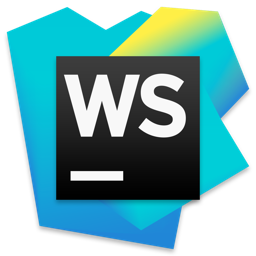
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
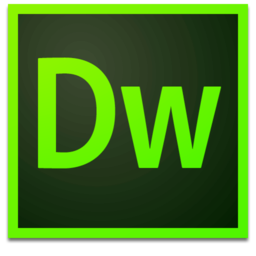
Dreamweaver Mac version
Visual web development tools
