Performance issues with JavaScript loop statements
In most programming languages, loop statements consume most of the time
And loop statements are a very important programming pattern
In our JavaScript, there are four types of loops
for loop
while loop
do-while loop
for-in loop
The first three loops are also very common in other languages
The for-in loop may be new to students who have studied C/C++ in school
It will Search instance and prototype properties, so it incurs more overhead per iteration
The for-in loop ends up being only 1/7 as fast as the other three types
So, unless we explicitly need to iterate over an unknown number of properties object, otherwise we should try to avoid using for-in
Don’t use for-in loops to traverse arrays
We can iterate a clear object like this
var props = ['prop1', 'prop2'], i = 0;while(i < props.length){ fn(obj[props[i++]]); }
This code creates an array of object properties based on the properties in the object, and then uses a while loop to traverse the property list and process the corresponding property values
This eliminates the need to search for each property of the object, reducing the number of loops Overhead
The premise of the above approach is that the properties inside the object are known
If we don’t know the internal implementation of the object
We still have to deal with the properties of the object itself, we can only do this
for(var prop in obj){ if(obj.hasOwnProperty(prop)){ //... } }
The cost is that each iteration has to determine whether the property is the object's own property rather than an inherited property
Except for-in, the performance of other loops is similar, so you should go to Consider the needs and choose the loop type
I believe that friends who have just learned programming are all familiar with writing loops
for(var i = 0; i < arr.length; i++){ fn(arr[i]); }
Every time this loop statement iterates, it must search arr length attribute, this is very time-consuming
So we can optimize,
for(var i = 0, len = arr.length; i < len; i++){ fn(arr[i]); }
Cache the array length value to a local variable, so the problem is solved
The same goes for while, do-while
Depending on the array length, this can save about 25% of running time in many browsers
We can also slightly improve performance by reversing the order of the array
for(var i = items.length; i--;){ process(items[i]); }
var j = items.length;while(j--){ process(items[j]); }
var k = items.length - 1;do { process(items[k]); }while(k--);
Do this every time The iteration control condition is reduced from two judgments (whether the number of iterations is less than the total number, whether it is true) to one judgment (whether it is true), which further improves the loop speed
A few final words
We all may have used some array methods such as arr.forEach() or some framework iteration methods such as jQuery's $().each() to traverse the array.
These methods execute an Functions
Although they are convenient, they are much slower than ordinary loops (calling external methods)
In all cases, loop-based iteration is about 8 times faster than function-based iteration
Therefore, when we can use ordinary loops (for, while, do-while) to solve problems, we try to use these ordinary loops
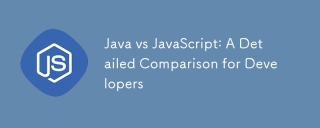
JavaandJavaScriptaredistinctlanguages:Javaisusedforenterpriseandmobileapps,whileJavaScriptisforinteractivewebpages.1)Javaiscompiled,staticallytyped,andrunsonJVM.2)JavaScriptisinterpreted,dynamicallytyped,andrunsinbrowsersorNode.js.3)JavausesOOPwithcl
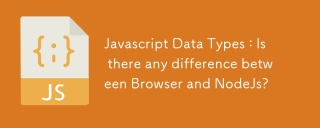
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
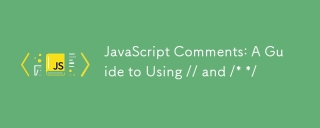
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
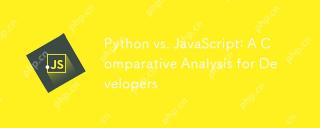
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
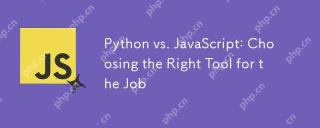
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
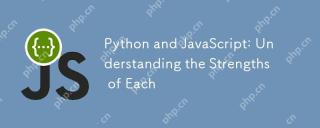
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
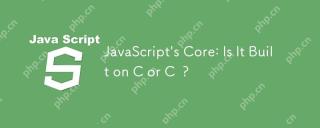
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
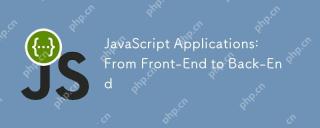
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
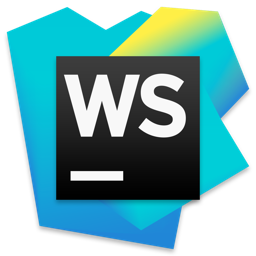
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
