As a developer, everyone should know that there are some built-in controls in the browser: Alert, Confirm, etc. However, these controls usually have different shapes according to different browser manufacturers, and the visual effects are often not up to par. UI designer requirements. More importantly, the style of such built-in controls is difficult to unify with the design styles of various Internet products with different styles. Therefore, excellent front-end developers develop their own personalized controls to replace these built-in browser controls. Of course, there are already countless excellent components of this type on the Internet. The purpose of writing this article is not to explain how excellent the component I developed is, nor to show off. I just hope that in this way, I can interact with more Many developers communicate with each other, learn from each other, and make progress together. Okay, without further ado, let’s get down to business.
Function introduction
Replace the Alert and Confirm controls that come with the browser
Customized interface style
The usage method is basically the same as the built-in control
Effect preview
1. Alert control
2. Confirm control
3. Complete code, online preview (see bottom, compressed package download is provided)
Development process
1. Component structure design
First, let’s take a look at the basic usage of built-in components:
alert("内置Alert控件"); if (confirm("关闭内置Confirm控件?")) { alert("True"); } else { alert("False"); }
In order to ensure that our components The usage is consistent with the built-in controls, so we must consider overriding the built-in controls. Taking into account the unified style of component development, ease of use, easy maintenance, and object-oriented features, I plan to use the customized alert and confirm methods as instance methods of a class (Winpop), and finally use the instance methods to override the system's built-in controls. method. In order to achieve the goal, my basic approach is as follows:
var obj = new Winpop(); // 创建一个Winpop的实例对象 // 覆盖alert控件 window.alert = function(str) { obj.alert.call(obj, str); }; // 覆盖confirm控件 window.confirm = function(str, cb) { obj.confirm.call(obj, str, cb); };
It should be noted that since the built-in controls of the browser can prevent other behaviors of the browser, our custom components do not have this ability. To be as unified as possible, as you can see in the preview, we use a full-screen semi-transparent mask layer when popping up the custom component. It is precisely because of the above reasons that some subtle adjustments have been made to the way the confirm component is used, from the built-in method of returning a Boolean value to the method of using a callback function to ensure that "OK" and "Cancel" can be added correctly. logic. Therefore, the use of custom components becomes the following form:
alert("自定义Alert组件"); confirm("关闭自定义Confirm组件?", function(flag){ if (flag) { alert("True"); } else { alert("False"); } });
2. Component code design
Before formally introducing the code of the Winpop component, Let’s first take a look at the basic structure of a Javascript component:
(function(window, undefined) { function JsClassName(cfg) { var config = cfg || {}; this.get = function(n) { return config[n]; } this.set = function(n, v) { config[n] = v; } this.init(); } JsClassName.prototype = { init: function(){}, otherMethod: function(){} }; window.JsClassName = window.JsClassName || JsClassName; })(window);
Use a self-executing anonymous function to wrap our component code to reduce global pollution as much as possible, and finally attach our class to the global For window objects, this is a recommended approach.
The get and set methods in the constructor are not necessary. It is just the author's personal habit. I feel that writing it this way can unify the calling methods of caching and reading the configuration parameters and other components' internal global variables, and it seems to be more convenient. Has an object-oriented style. Readers are welcome to share their thoughts and whether it is good or not to write this way.
Next, let’s take a look at the complete code of the Winpop component:
(function(window, jQuery, undefined) { var HTMLS = { ovl: '<p class="J_WinpopMask winpop-mask" id="J_WinpopMask"></p>' + '<p class="J_WinpopBox winpop-box" id="J_WinpopBox">' + '<p class="J_WinpopMain winpop-main"></p>' + '<p class="J_WinpopBtns winpop-btns"></p>' + '</p>', alert: '<input type="button" class="J_AltBtn pop-btn alert-button" value="确定">', confirm: '<input type="button" class="J_CfmFalse pop-btn confirm-false" value="取消">' + ' <input type="button" class="J_CfmTrue pop-btn confirm-true" value="确定">' } function Winpop() { var config = {}; this.get = function(n) { return config[n]; } this.set = function(n, v) { config[n] = v; } this.init(); } Winpop.prototype = { init: function() { this.createDom(); this.bindEvent(); }, createDom: function() { var body = jQuery("body"), ovl = jQuery("#J_WinpopBox"); if (ovl.length === 0) { body.append(HTMLS.ovl); } this.set("ovl", jQuery("#J_WinpopBox")); this.set("mask", jQuery("#J_WinpopMask")); }, bindEvent: function() { var _this = this, ovl = _this.get("ovl"), mask = _this.get("mask"); ovl.on("click", ".J_AltBtn", function(e) { _this.hide(); }); ovl.on("click", ".J_CfmTrue", function(e) { var cb = _this.get("confirmBack"); _this.hide(); cb && cb(true); }); ovl.on("click", ".J_CfmFalse", function(e) { var cb = _this.get("confirmBack"); _this.hide(); cb && cb(false); }); mask.on("click", function(e) { _this.hide(); }); jQuery(document).on("keyup", function(e) { var kc = e.keyCode, cb = _this.get("confirmBack");; if (kc === 27) { _this.hide(); } else if (kc === 13) { _this.hide(); if (_this.get("type") === "confirm") { cb && cb(true); } } }); }, alert: function(str, btnstr) { var str = typeof str === 'string' ? str : str.toString(), ovl = this.get("ovl"); this.set("type", "alert"); ovl.find(".J_WinpopMain").html(str); if (typeof btnstr == "undefined") { ovl.find(".J_WinpopBtns").html(HTMLS.alert); } else { ovl.find(".J_WinpopBtns").html(btnstr); } this.show(); }, confirm: function(str, callback) { var str = typeof str === 'string' ? str : str.toString(), ovl = this.get("ovl"); this.set("type", "confirm"); ovl.find(".J_WinpopMain").html(str); ovl.find(".J_WinpopBtns").html(HTMLS.confirm); this.set("confirmBack", (callback || function() {})); this.show(); }, show: function() { this.get("ovl").show(); this.get("mask").show(); }, hide: function() { var ovl = this.get("ovl"); ovl.find(".J_WinpopMain").html(""); ovl.find(".J_WinpopBtns").html(""); ovl.hide(); this.get("mask").hide(); }, destory: function() { this.get("ovl").remove(); this.get("mask").remove(); delete window.alert; delete window.confirm; } }; var obj = new Winpop(); window.alert = function(str) { obj.alert.call(obj, str); }; window.confirm = function(str, cb) { obj.confirm.call(obj, str, cb); }; })(window, jQuery);
The code is slightly more, the key points are as follows:
The author stole it I'm lazy and used jQuery. Please make sure you have introduced jQuery before using it
The custom component structure is eventually appended to the body, so before introducing the above js, please make sure The document has been loaded
The component has added the function of pressing ESC and clicking the mask layer to hide the component
-
Note: Although it is not used in this example destroy method, but readers can pay attention to delete window.alert and delete window.confirm in this method. The purpose of writing this is to ensure that after the custom component is destroyed, the Alert and Confirm controls are restored to the browser's built-in effects
If you add window.Winpop = Winpop at the end of the component, you can make the object global for other classes to call
Finally
As a front-end development engineer, I personally think that Javascript component development is a very interesting thing, and the fun can only be realized if you try it yourself. Front-end component development often requires the cooperation of Javascript, CSS and HTML to achieve twice the result with half the effort. The Winpop mentioned above is no exception. Here we provide you with a complete demo compressed package. Interested readers are welcome to spread the word.
The above is the content of developing a complete JavaScript component. For more related content, please pay attention to the PHP Chinese website (www.php.cn)!
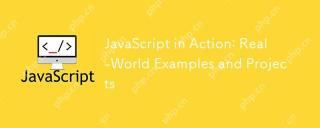
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
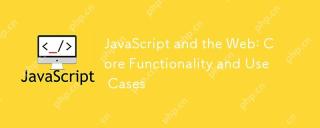
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
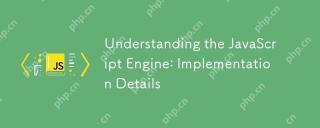
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
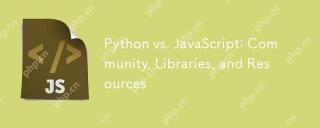
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
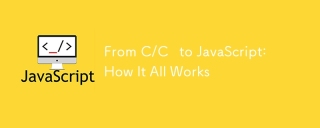
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
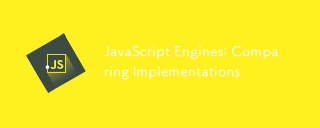
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
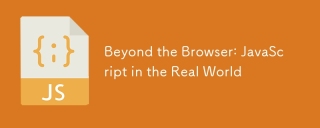
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
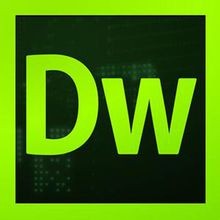
Dreamweaver CS6
Visual web development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

Atom editor mac version download
The most popular open source editor
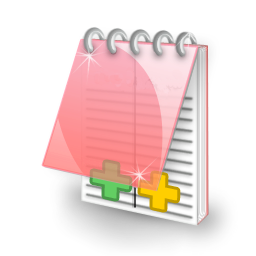
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Chinese version
Chinese version, very easy to use