


1. How to implement iterable objects and iterator objects?
Actual case
A certain software requires to capture the smell information of each city from the network and display it next:
北京: 15 ~ 20 天津: 17 ~ 22 长春: 12 ~ 18 ......
If we capture the weather of all cities at once and then display it, there will be a high delay and a waste of storage space when displaying the temperature of the first city. We expect to access it in a time-consuming manner. Strategy, and encapsulates all city temperatures into an object. You can use the for statement to iterate. How to solve it?
Solution
Implement an iterator objectWeatherlterator
,next
The method returns a city temperature each time, implement an Iterable objectWeatherlterable
,————iter__ method returns an iterator object
import requests from collections import Iterable, Iterator # 气温迭代器 class WeatherIterator(Iterator): def __init__(self, cities): self.cities = cities self.index = 0 def getWeather(self, city): r = requests.get('http://wthrcdn.etouch.cn/weather_mini?city=' + city) data = r.json()['data']['forecast'][0] return '%s:%s , %s' % (city, data['low'], data['high']) def __next__(self): if self.index == len(self.cities): raise StopIteration city = self.cities[self.index] self.index += 1 return self.getWeather(city) # 可迭代对象 class WeatherIterable(Iterable): def __init__(self, cities): self.cities = cities def __iter__(self): return WeatherIterator(self.cities) for x in WeatherIterable(['北京', '上海', '广州', '深圳']): print(x)
The execution result is as follows:
C:\Python\Python35\python.exe E:/python-intensive-training/s2.py 北京:低温 21℃ , 高温 30℃ 上海:低温 23℃ , 高温 26℃ 广州:低温 26℃ , 高温 34℃ 深圳:低温 27℃ , 高温 33℃ Process finished with exit code 0
2. How to use generator functions to implement iterable objects?
Actual case
Implement a class of iterable objects that can iterate out all prime numbers in a given range:
python pn = PrimeNumbers(1, 30) for k in pn: print(k) `` 输出结果text 2 3 5 7 11 13 17 19 23 29 “`
Solution
- Implement the generator function in the __iter__
method of this class, each Timesyield
Returns a prime number
class PrimeNumbers: def __init__(self, start, stop): self.start = start self.stop = stop def isPrimeNum(self, k): if k < 2: return False for i in range(2, k): if k % i == 0: return False return True def __iter__(self): for k in range(self.start, self.stop + 1): if self.isPrimeNum(k): yield k for x in PrimeNumbers(1, 20): print(x)
Run result
C:\Python\Python35\python.exe E:/python-intensive-training/s3.py 2 3 5 7 11 13 17 19 Process finished with exit code 0
3. How to perform reverse iteration and how to implement reverse iteration?
Actual case
Implementing a continuous floating point number generatorFloatRange
(and rrange
Similar), generate a series of continuous floating point numbers based on the given range (start
, stop
) and step value (step
), such as iterationFloatRange(3.0,4.0,0.2)
Can generate sequence:
正向:3.0 > 3.2 > 3.4 > 3.6 > 3.8 > 4.0 反向:4.0 > 3.8 > 3.6 > 3.4 > 3.2 > 3.0
Solution
Implementation The __reversed__
method of the reverse iteration protocol, which returns a reverse iterator
class FloatRange: def __init__(self, start, stop, step=0.1): self.start = start self.stop = stop self.step = step def __iter__(self): t = self.start while t <= self.stop: yield t t += self.step def __reversed__(self): t = self.stop while t >= self.start: yield t t -= self.step print("正相迭代-----") for n in FloatRange(1.0, 4.0, 0.5): print(n) print("反迭代-----") for x in reversed(FloatRange(1.0, 4.0, 0.5)): print(x)
output result
C:\Python\Python35\python.exe E:/python-intensive-training/s4.py 正相迭代----- 1.0 1.5 2.0 2.5 3.0 3.5 4.0 反迭代----- 4.0 3.5 3.0 2.5 2.0 1.5 1.0 Process finished with exit code 0
4. How to perform slicing operations on iterators?
Actual case
There is a certain text file, and we want to remove a certain range of content, such as 100~300 lines The content between text files in python is an iterable object. Can we use a method similar to list slicing to get a generator of file content between 100 and 300 lines?
Solution
Use itertools.islice
from the standard library, which returns a generator for slices of iterator objects
from itertools import islice f = open('access.log') # # 前500行 # islice(f, 500) # # 100行以后的 # islice(f, 100, None) for line in islice(f,100,300): print(line)
Every training session of islice will consume the previous iteration object
l = range(20) t = iter(l) for x in islice(t, 5, 10): print(x) print('第二次迭代') for x in t: print(x)
Output result
C:\Python\Python35\python.exe E:/python-intensive-training/s5.py 5 6 7 8 9 第二次迭代 10 11 12 13 14 15 16 17 18 19 Process finished with exit code 0
5. How to iterate multiple iterable objects in a for statement?
Actual case
1. The final exam results of a certain class of students, Chinese, mathematics, and English are stored in 3 lists respectively. , iterate three lists at the same time, and calculate the total score of each student (parallel)
2. There are four classes in a certain age, and the English scores of each class in a certain test are stored in four lists respectively. Iterate through each list in turn and count the number of people whose scores are higher than 90 points in the entire school year (serial)
Solution
Parallel: Use built-in functions zip
, it can merge multiple iterable objects, and each iteration returns a tuple
from random import randint # 申城语文成绩,# 40人,分数再60-100之间 chinese = [randint(60, 100) for _ in range(40)] math = [randint(60, 100) for _ in range(40)] # 数学 english = [randint(60, 100) for _ in range(40)] # 英语 total = [] for c, m, e in zip(chinese, math, english): total.append(c + m + e) print(total)
The execution results are as follows :
C:\Python\Python35\python.exe E:/python-intensive-training/s6.py [232, 234, 259, 248, 241, 236, 245, 253, 275, 238, 240, 239, 283, 256, 232, 224, 201, 255, 206, 239, 254, 216, 287, 268, 235, 223, 289, 221, 266, 222, 231, 240, 226, 235, 255, 232, 235, 250, 241, 225] Process finished with exit code 0
Serial: Use itertools.chain
from the standard library, which can connect multiple iterable objects
from random import randint from itertools import chain # 生成四个班的随机成绩 e1 = [randint(60, 100) for _ in range(40)] e2 = [randint(60, 100) for _ in range(42)] e3 = [randint(60, 100) for _ in range(45)] e4 = [randint(60, 100) for _ in range(50)] # 默认人数=1 count = 0 for s in chain(e1, e2, e3, e4): # 如果当前分数大于90,就让count+1 if s > 90: count += 1 print(count)
Output result
C:\Python\Python35\python.exe E:/python-intensive-training/s6.py 48 Process finished with exit code 0
Summary
The above is the entire content of this article. I hope it will be helpful to everyone’s study or work. If you have any questions, you can leave a message to communicate.
For more related articles on the summary of object iteration and anti-iteration techniques in Python, please pay attention to the PHP Chinese website!
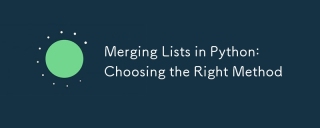
TomergelistsinPython,youcanusethe operator,extendmethod,listcomprehension,oritertools.chain,eachwithspecificadvantages:1)The operatorissimplebutlessefficientforlargelists;2)extendismemory-efficientbutmodifiestheoriginallist;3)listcomprehensionoffersf
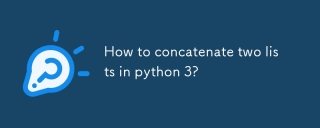
In Python 3, two lists can be connected through a variety of methods: 1) Use operator, which is suitable for small lists, but is inefficient for large lists; 2) Use extend method, which is suitable for large lists, with high memory efficiency, but will modify the original list; 3) Use * operator, which is suitable for merging multiple lists, without modifying the original list; 4) Use itertools.chain, which is suitable for large data sets, with high memory efficiency.
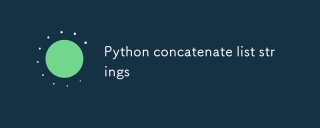
Using the join() method is the most efficient way to connect strings from lists in Python. 1) Use the join() method to be efficient and easy to read. 2) The cycle uses operators inefficiently for large lists. 3) The combination of list comprehension and join() is suitable for scenarios that require conversion. 4) The reduce() method is suitable for other types of reductions, but is inefficient for string concatenation. The complete sentence ends.
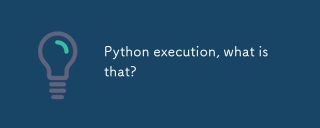
PythonexecutionistheprocessoftransformingPythoncodeintoexecutableinstructions.1)Theinterpreterreadsthecode,convertingitintobytecode,whichthePythonVirtualMachine(PVM)executes.2)TheGlobalInterpreterLock(GIL)managesthreadexecution,potentiallylimitingmul
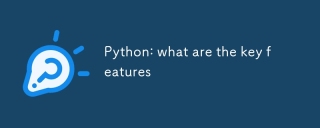
Key features of Python include: 1. The syntax is concise and easy to understand, suitable for beginners; 2. Dynamic type system, improving development speed; 3. Rich standard library, supporting multiple tasks; 4. Strong community and ecosystem, providing extensive support; 5. Interpretation, suitable for scripting and rapid prototyping; 6. Multi-paradigm support, suitable for various programming styles.
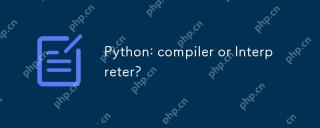
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
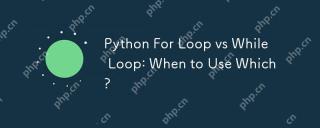
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
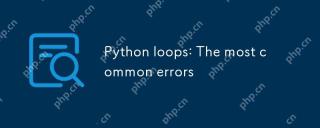
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
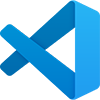
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
