


Ensure that a class has only one instance and provide a global access point to the instance.
——"Design Pattern"
The concept of singleton pattern is very simple. Below is C# Using language as an example, the advantages and disadvantages of common singleton writing methods are listed.
1. Simple implementation
public sealed class Singleton { static Singleton instance = null; public void Show() { Console.WriteLine( "instance function"); } private Singleton() { } public static Singleton Instance { get { if (instance == null) { instance = new Singleton(); } return instance; } } }
Comments:
Not safe for threads
Requirements have been met in single thread
Advantages:
Since the instance is created inside the Instance property method , so the class can use additional functionality
and not perform instantiation until the object requires an instance; this approach is called "lazy instantiation". Lazy instantiation avoids unnecessary instantiation of singletons when the application starts.
2. Thread safety
public sealed class Singleton { static Singleton instance = null; private static readonly object padlock = new object(); private Singleton() { } public static Singleton Instance { get { lock (padlock) { if (instance == null) { instance = new Singleton(); } } return instance; } } }
Comment:
The only part of the program that is locked at the same time is A thread can enter
The object instance is created by the thread that enters first
Later threads will be false when entering (instance == null) and will not be returned To create object instances
Added additional overhead and lost performance
##3. Double lock
public sealed class Singleton { static Singleton instance = null; private static readonly object padlock = new object(); private Singleton() { } public static Singleton Instance { get { if (instance == null) { lock (padlock) { if (instance == null) { instance = new Singleton(); } } } return instance; } } }Comment:
Multi-thread safety
Threads are not locked every time
Allow instantiation to be delayed until the first time the object is accessed
4. Static initialization
public sealed class Singleton { private static readonly Singleton instance = null; static Singleton() { instance = new Singleton(); } private Singleton() { } public static Singleton Instance { get { return instance; } } }Comment:
Depends on the common language runtime to handle variable initialization
Public static properties provide a Global access point
Less control over the instantiation mechanism (implemented by .NET)
Static initialization is the preferred method to implement Singleton in .NET
Small Note:
# Static constructors have neither access modifiers, C# will automatically mark them as private, why they must be marked For private,
is to prevent code written by developers from calling it. The call to it is always the responsibility of the CLR.
##5. Delayed initialization
public sealed class Singleton { private Singleton() { } public static Singleton Instance { get { return Nested.instance; } } public static void Hello() { } private class Nested { internal static readonly Singleton instance = null; static Nested() { instance = new Singleton(); } } }Comments:
Because the timing of calling static functions is when the class is instantiated or the static member is called, And the .net framework calls the static constructor to initialize the static member variables, So, if you write according to the fourth method, When the Hello method is called again, a Singleton instance will be instantiated. This is not what we want to see, because we may just want to use the Hello method and nothing else.
Notes:
1. The instance constructor in Singleton mode can be set to protected to allow subclasses to be derived.
2. Singleton mode generally does not support the ICloneable interface, because this may lead to multiple object instances, which is contrary to the original intention of Singleton mode.
3. Singleton mode generally does not support serialization, because this may also lead to multiple object instances, which is also contrary to the original intention of Singleton mode.
4. The Singletom mode only takes into account the management of object creation, but does not consider the management of object destruction. In terms of platforms that support garbage collection and the overhead of objects, we generally do not need to perform special management of their destruction.
##Summary:
1. Singleton Patterns limit rather than improve class creation.
2. The core of understanding and extending the Singleton pattern is "how to control the user's arbitrary call to the constructor of a class using new."
3. You can easily modify a Singleton to have a few instances. This is allowed and meaningful.
The above is the content of singleton pattern and common writing method analysis (design pattern 01), more related Please pay attention to the PHP Chinese website (www.php.cn) for content!
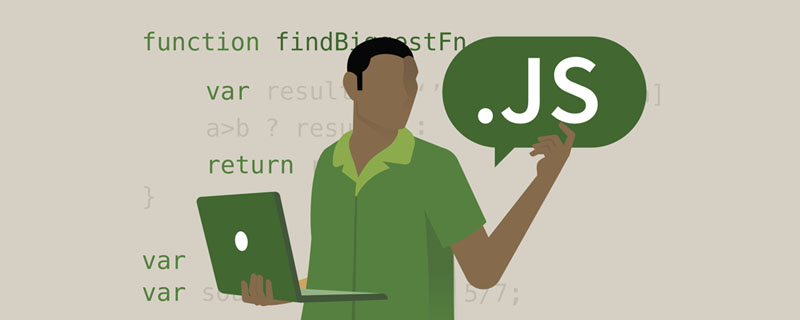
JS 单例模式是一种常用的设计模式,它可以保证一个类只有一个实例。这种模式主要用于管理全局变量,避免命名冲突和重复加载,同时也可以减少内存占用,提高代码的可维护性和可扩展性。
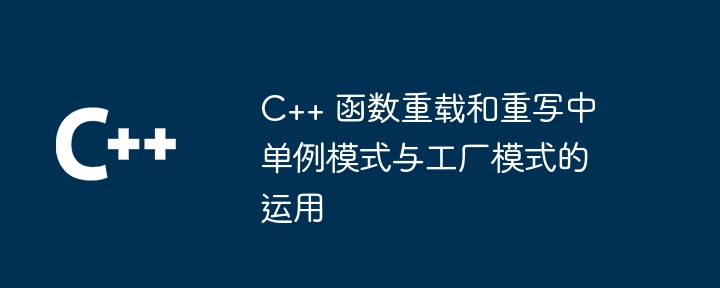
单例模式:通过函数重载提供不同参数的单例实例。工厂模式:通过函数重写创建不同类型的对象,实现创建过程与具体产品类的解耦。
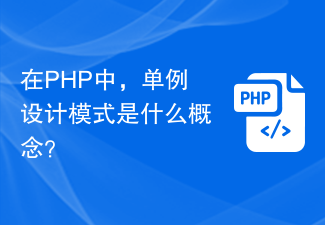
Singleton模式确保一个类只有一个实例,并提供了一个全局的访问点。它确保在应用程序中只有一个对象可用,并处于受控状态。Singleton模式提供了一种访问其唯一对象的方式,可以直接访问,而无需实例化类的对象。示例<?php classdatabase{ publicstatic$connection; privatefunc
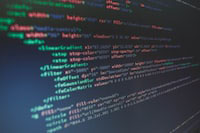
导言PHP设计模式是一组经过验证的解决方案,用于解决软件开发中常见的挑战。通过遵循这些模式,开发者可以创建优雅、健壮和可维护的代码。它们帮助开发者遵循SOLID原则(单一职责、开放-封闭、Liskov替换、接口隔离和依赖反转),从而提高代码的可读性、可维护性和可扩展性。设计模式的类型有许多不同的设计模式,每种模式都有其独特的目的和优点。以下是一些最常用的php设计模式:单例模式:确保一个类只有一个实例,并提供一种全局访问此实例的方法。工厂模式:创建一个对象,而不指定其确切类。它允许开发者根据条件
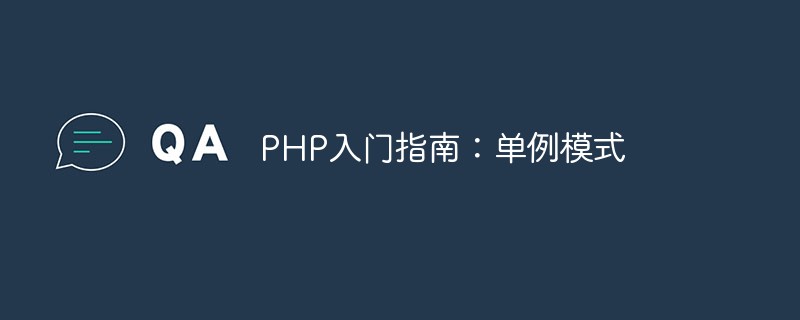
在软件开发中,常常遇到多个对象需要访问同一个资源的情况。为了避免资源冲突以及提高程序的效率,我们可以使用设计模式。其中,单例模式是一种常用的创建对象的方式,即保证一个类只有一个实例,并提供全局访问。本文将为大家介绍如何使用PHP实现单例模式,并提供一些最佳实践的建议。一、什么是单例模式单例模式是一种常用的创建对象的方式,它的特点是保证一个类只有一个实例,并提
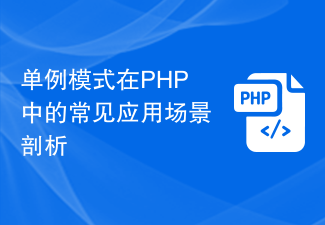
单例模式在PHP中的常见应用场景剖析概述:单例模式(SingletonPattern)是一种创建型设计模式,它确保一个类只有一个实例,并提供一个全局访问点来访问该实例。在PHP中,使用单例模式可以有效地限制类的实例化次数和资源占用,提高代码的性能和可维护性。本文将通过分析常见的应用场景,给出具体的PHP代码示例,来说明单例模式的使用方法和好处。数据库连接管
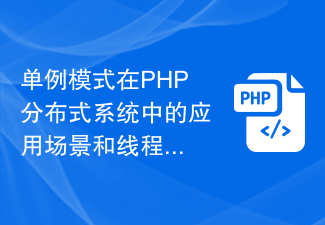
单例模式在PHP分布式系统中的应用场景和线程安全流程引言:随着互联网的迅猛发展,分布式系统已成为现代软件开发的热门话题。而在分布式系统中,线程安全一直是一个重要的问题。在PHP开发中,单例模式是一种常用的设计模式,它可以有效地解决资源共享和线程安全的问题。本文将重点讨论单例模式在PHP分布式系统中的应用场景和线程安全流程,并提供具体的代码示例。一、单例模式的
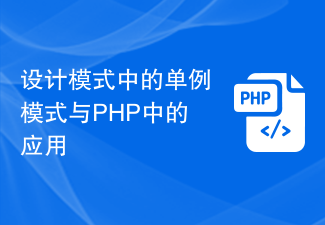
设计模式中的单例模式与PHP中的应用引言:设计模式是在软件设计过程中,经验丰富的软件工程师总结出来的一些解决特定问题的经典模式。其中,单例模式是最常用的设计模式之一。单例模式确保一个类只有一个实例,并提供了一个全局访问点来访问这个实例。在PHP中,单例模式被广泛应用于各种场景。本文将详细介绍单例模式的概念、特点以及在PHP中的具体应用,同时给出相关的代码示例


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
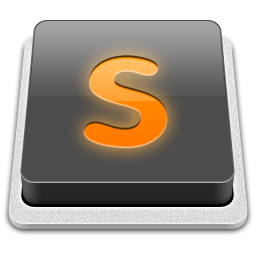
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
