setBackgroundResource(0) can remove the background color of View
Resources.getSystem().getDisplayMetrics().density You can get the screen density without using Context
By overloading ViewGroup dispatchDraw can implement a simple mask effect. For example, when pulling down to refresh, you can add a layer of mask to the contentView. canvas.drawRect(0, mContentView.getTranslationY(), getWidth(), getHeight(), mMaskPaint);
new View can use View.generateViewId() (available for API 17 or above) to generate id, the system Guaranteed to be unique
When using GridView, the effect is better when using android:padding and android:clipToPadding="false" together.
In the layout file, if it is just for placeholder, you can use Space to replace View. The best thing is that Space can skip the Draw process.
TypedValue.applyDimension(int unit, float value, DisplayMetrics metrics) facilitates conversion between dp, px, sp.
Activity.startActivities() The most direct understanding of this method is to use intent to open multiple activities
TextUtils.isEmpty() If the incoming String is NULL or Length is 0, it will return true .
Html.fromHtml() If you are familiar with Html, you can quickly handle some rich text operations through this method. For example, processing of hyperlinks and graphic and text layout.
TextView.setError() Set text box error reminder
Build.VERSION_CODES Sometimes our app needs to perform different operations according to different SDK versions
PhoneNumberUtils.convertKeypadLettersToDigits This method is simple and crude. It will convert the entered letters into numbers according to the mapping on the keyboard.
ArgbEvaluator ArgbEvaluator.evaluate(float fraction, Object startValue, Object endValue); Generate a new color based on a starting color value, an ending color value and an offset, achieving something similar to the bottom of WeChat in minutes Bar sliding color gradient.
ValueAnimator.reverse() Smoothly cancel the animation effect
DateUtils.formatDateTime()) This method can output the corresponding formatted time or date
Pair This class can be used To store a "set" of data. But it is not the relationship between key and value.
SparseArray Currently, there are many places that use SparseArray to replace hashMap from the perspective of performance optimization to save memory and improve performance.
Linkify.addLinks() This class makes it easier to add hyperlinks to text.
android.media.ThumbnailUtils This class is mainly used to handle thumbnail-related work, such as: used to obtain thumbnails of media (pictures, videos);
Bitmap.extractAlpha () ;Return a new Bitmap, capturing the alpha value of the original image. Sometimes we need to dynamically modify the background image of an element and do not want to use multiple images. Through this method, combined with Canvas and Paint, we can dynamically modify the color of a solid-color Bitmap.
When there are messages that need to be transmitted between modules, use LocalBroadcastManager instead of Listener to decouple the modules. In addition to decoupling, sending messages and executing messages are separated by one thread cycle, which can reduce the method call chain. I encountered a StackOverflow problem when the method call chain was too long.
Static variables should not directly or indirectly reference Activity, Service, etc. This will make the Activity and all the objects it refers to unable to be released. Then, as the user operates for a long time, the memory will skyrocket.
One feature of the Handler mechanism is that it will not end with the end of the life cycle of Activity and Service. In other words, if you post a Delay Runnable and then exit the Activity before the Runnable is executed, the Runnable will still be executed after the time is up. If the Runnable contains an operation to update the View, the program crashes.
Many people like to use Context.runOnUiThread when updating Views in child threads. This method has a disadvantage, that is, once the Context life cycle ends, such as when the Activity has been destroyed, it will crash as soon as it is called.
SharedPreferences.Editor.commit This method is synchronous and will not return until the data is synchronized to Flash. The IO operation is uncontrollable, so try to use the apply method instead. apply is only supported in API Level>=9 and needs to be compatible. However, the latest support v4 package has already taken care of it for us, just use SharedPreferencesCompat.EditorCompat.getInstance().apply(editor).
PackageManager.getInstalledPackages This method is often used. You may not know that when the number of results obtained is relatively large, it may take seconds to call it on some models, so try to use it in a child thread. used in. In addition, if there are too many results and exceed the upper limit of the maximum Binder data transmission set by the system, a TransactionException will occur. If you use this method to obtain the list of installed applications on the machine, it is best to take precautions.
If you use Context.startActivity to start an external application, it is best to do some exception prevention, because if the corresponding application cannot be found, an exception will be thrown. If you want to open an Activity within the application, you may want to use an explicit Intent, which can improve the efficiency of the system's search for the target Activity.
The life cycle of Application is the life cycle of the process. The Application will be destroyed only when the process is killed. Even if no Activity or Service is running, the Application will exist. Therefore, in order to reduce memory pressure, try not to reference large objects, Context, etc. in Application.
getWindow().setLayout(ViewGroup.LayoutParams.MATCH_PARENT, ViewGroup.LayoutParams.MATCH_PARENT); The full-screen method must be set after setContentView.
The setCurrentItem of viewpager must be called after the setAdapter method. It will have an effect.
Determine whether the phone is in airplane mode boolean isEnabled = Settings.System.getInt(context.getContentResolver(), Settings.System.AIRPLANE_MODE_ON, 0) == 1;
Traverse The best method of HashMap
public static void printMap(Map mp) { for (Map.Entry m : mp.entrySet()) { System.out.println(m.getKey() + ":" + m.getValue()); } }
Use Java to generate random integer numbers in a range
public static int randInt(int min, int max) { Random rand = new Random(); int randomNum = rand.nextInt((max - min) + 1) + min; return randomNum; }
If the subclass implements the Serializable interface but the parent class does not, the parent class will not be serialized. But at this time, the parent class must have a parameterless constructor, otherwise an InvalidClassException will be thrown.
Transient keyword modified variables can limit serialization.
When using JakeWharton's TabPageIndicator, if you need to do some time-consuming operations first and then display the TabPageIndicator, you need to set mIndirector.setVisibility(View.GONE) first; and then mIndirector after the time-consuming task is completed. setVisibility(View.VISIBLE); Otherwise, an error will be reported
Calling sequence between class inheritance Parent class static members -> Subclass static members -> Parent class ordinary member initialization and initialization block -> Parent class Construction method -> Subclass ordinary member initialization and initialization block -> Subclass construction method
Huawei mobile phone cannot display log solution,.Dial interface input (*#*#2846579#*#*) Service menu will appear.Go to "ProjectMenu" -> "Background Setting" -> "Log Setting"Open "Log switch" and set it to ON.Open "Log level setting" and set the log level you wish.
Background services often appear due to restarts and the like. The parameter passed by Intent in onStartCommand() is null. Change the return value in onStartCommand() to return super.onStartCommand(intent, Service.START_REDELIVER_INTENT, startId); can solve the problem. The following introduces the meaning of several flags
PopupWindow and Dialog cannot be displayed when the Activity is not fully displayed
Do not use SharedPreferences to share data between multiple processes. Although it is possible (MODE_MULTI_PROCESS), it is extremely unstable
For more related articles on some useful methods in Android development that you don’t know, please pay attention to the PHP Chinese website!
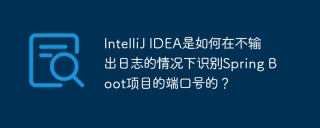
Start Spring using IntelliJIDEAUltimate version...
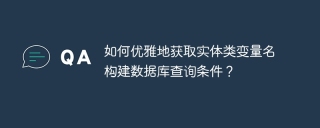
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
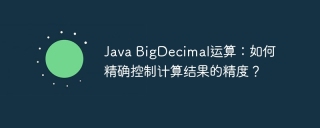
Java...
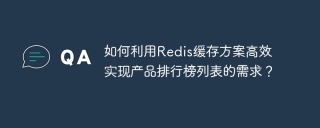
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
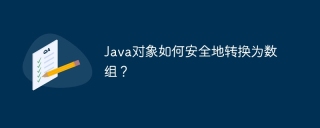
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
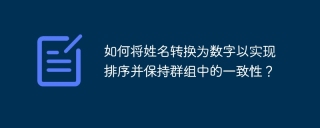
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
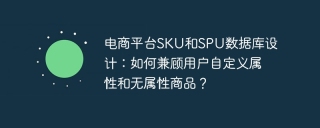
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
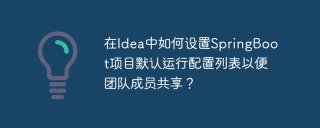
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
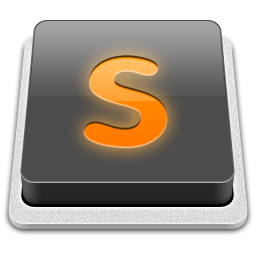
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor