Previous words
Object copy was introduced in the previous blog post. This article will introduce array copy in detail
push
function copyArray(arr){ var result = []; for(var i = 0; i < arr.length; i++){ result.push(arr[i]); } return result; } var obj1=[1,2,3]; var obj2=copyArray(obj1); console.log(obj1); //[1,2,3] console.log(obj2); //[1,2,3] obj2.push(4); console.log(obj1); //[1,2,3] console.log(obj2); //[1,2,3,4]
join
The disadvantage of using this method is that all the items in the array become strings
function copyArray(arr){ var result = []; result = arr.join().split(','); return result; } var obj1=[1,2,3]; var obj2=copyArray(obj1); console.log(obj1); //[1,2,3] console.log(obj2); //['1','2','3'] obj2.push(4); console.log(obj1); //[1,2,3] console.log(obj2); //['1','2','3',4]
concat
function copyArray(arr){ var result = []; result = arr.concat(); return result; } var obj1=[1,2,3]; var obj2=copyArray(obj1); console.log(obj1); //[1,2,3] console.log(obj2); //[1,2,3] obj2.push(4); console.log(obj1); //[1,2,3] console.log(obj2); //[1,2,3,4]
slice
function copyArray(arr){ var result = []; result = arr.slice(); return result; } var obj1=[1,2,3]; var obj2=copyArray(obj1); console.log(obj1); //[1,2,3] console.log(obj2); //[1,2,3] obj2.push(4); console.log(obj1); //[1,2,3] console.log(obj2); //[1,2,3,4]
deep copy
The above method only implements a shallow copy of the array , if you want to implement deep copy of the array, you need to use the recursive method
function copyArray(arr,result){ var result = result || []; for(var i = 0; i < arr.length; i++){ if(arr[i] instanceof Array){ result[i] = []; copyArray(arr[i],result[i]); }else{ result[i] = arr[i]; } } return result; } var obj1=[1,2,[3,4]]; var obj2=copyArray(obj1); console.log(obj1[2]); //[3,4] console.log(obj2[2]); //[3,4] obj2[2].push(5); console.log(obj1[2]); //[3,4] console.log(obj2[2]); //[3,4,5]
For more detailed articles on JavaScript array copy, please pay attention to the PHP Chinese website!
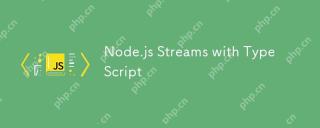
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
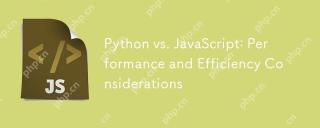
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
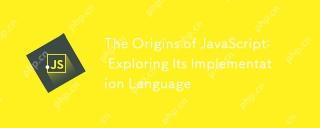
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
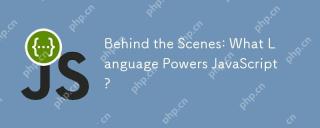
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
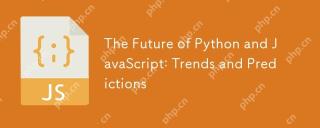
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
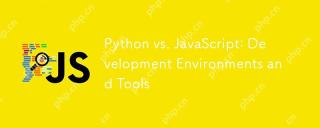
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
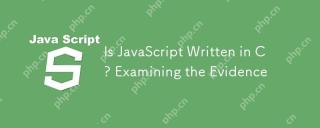
Yes, the engine core of JavaScript is written in C. 1) The C language provides efficient performance and underlying control, which is suitable for the development of JavaScript engine. 2) Taking the V8 engine as an example, its core is written in C, combining the efficiency and object-oriented characteristics of C. 3) The working principle of the JavaScript engine includes parsing, compiling and execution, and the C language plays a key role in these processes.
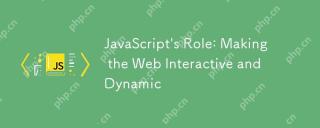
JavaScript is at the heart of modern websites because it enhances the interactivity and dynamicity of web pages. 1) It allows to change content without refreshing the page, 2) manipulate web pages through DOMAPI, 3) support complex interactive effects such as animation and drag-and-drop, 4) optimize performance and best practices to improve user experience.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
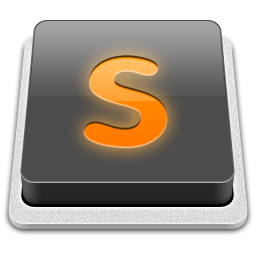
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use
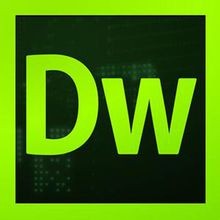
Dreamweaver CS6
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
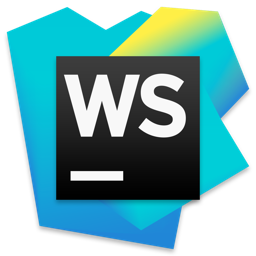
WebStorm Mac version
Useful JavaScript development tools
