Java annotations are some meta-information attached to the code, which are used by some tools to parse and use during compilation and runtime, and serve the function of explanation and configuration.
Annotations will not and cannot affect the actual logic of the code, they only play a supporting role. Contained in the java.lang.annotation package.
1. Meta-annotation
Meta-annotation refers to the annotation of annotation. There are four types including @Retention @Target @Document @Inherited.
1.1. @Retention: Define the retention policy of annotations
Java code
@Retention(RetentionPolicy.SOURCE) //注解仅存在于源码中,在class字节码文件中不包含 @Retention(RetentionPolicy.CLASS) //默认的保留策略,注解会在class字节码文件中存在,但运行时无法获得, @Retention(RetentionPolicy.RUNTIME)//注解会在class字节码文件中存在,在运行时可以通过反射获取到
1.2. @Target: Define the purpose of annotations
Java code
@Target(ElementType.TYPE) //接口、类、枚举、注解 @Target(ElementType.FIELD) //字段、枚举的常量 @Target(ElementType.METHOD) //方法 @Target(ElementType.PARAMETER) //方法参数 @Target(ElementType.CONSTRUCTOR) //构造函数 @Target(ElementType.LOCAL_VARIABLE)//局部变量 @Target(ElementType.ANNOTATION_TYPE)//注解 @Target(ElementType.PACKAGE) ///包
elementType can be Multiple, an annotation can be of a class, a method, a field, etc.
1.3. @Document: Indicates that the annotation will be included in javadoc
1.4. @Inherited: Indicates that subclasses can inherit from parent classes The annotation
The following is an example of a custom annotation
2. Customization of annotations
Java code
@Retention(RetentionPolicy.RUNTIME) @Target(ElementType.METHOD) public @interface HelloWorld { public String name() default ""; }
3. Use of annotations, test class
Java code
public class SayHello { @HelloWorld(name = " 小明 ") public void sayHello(String name) { System.out.println(name + "say hello world!"); }//www.heatpress123.net }
4. Parse annotations
java’s reflection mechanism can help to get annotations. The code is as follows:
Java code
public class AnnTest { public void parseMethod(Class<?> clazz) { Object obj; try { // 通过默认构造方法创建一个新的对象 obj = clazz.getConstructor(new Class[] {}).newInstance( new Object[] {}); for (Method method : clazz.getDeclaredMethods()) { HelloWorld say = method.getAnnotation(HelloWorld.class); String name = ""; if (say != null) { name = say.name(); System.out.println(name); method.invoke(obj, name); } } } catch (Exception e) { e.printStackTrace(); } } public static void main(String[] args) { AnnTest t = new AnnTest(); t.parseMethod(SayHello.class); } }
Please pay attention to more articles related to java custom annotation interface implementation solutions PHP Chinese website!
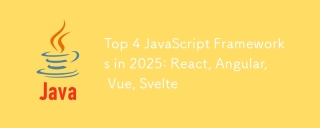
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
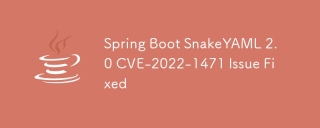
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
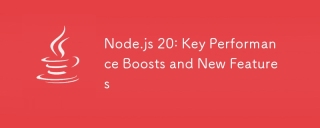
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
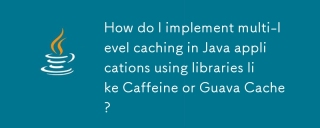
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
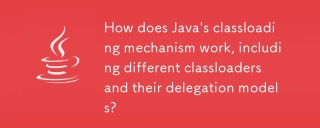
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
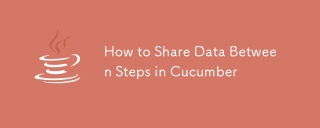
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
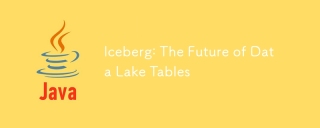
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
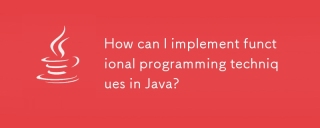
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
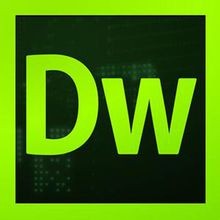
Dreamweaver CS6
Visual web development tools
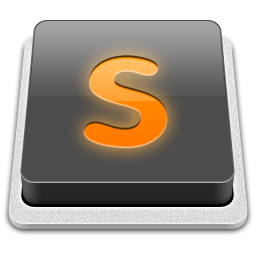
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 English version
Recommended: Win version, supports code prompts!
