It seems that Django has given up the use of its own comments since version 1.6. The specific reason has not been investigated, but now the comment function can also be implemented using Django’s internal module, that is, with the help of the forms module. Here is mine A small example.
Environment preparation
•Operating system: windows 7 64-bit ultimate version
•IDE: PyCharm 2016.1
•Python:2.7.11
•Django:1.9.6
Design
The so-called design refers to the underlying model that will be involved in the comment function we are going to implement. My simple design here is as follows. You can set it as you like according to your own ideas. My settings here can be found in the models.py file:
from __future__ import unicode_literals from django.contrib import admin from django.db import models from django import forms # Create your models here. TOPIC_CHOICES = ( ('level1','Bad'), ('level2','SoSo'), ('level3','Good'), ) class RemarkForm(forms.Form): subject = forms.CharField(max_length=100,label='Mark Board') mail = forms.EmailField(label='email') topic = forms.ChoiceField(choices=TOPIC_CHOICES,label='choose one topic') message = forms.CharField(label='content for mark',widget=forms.Textarea) cc_myself = forms.BooleanField(required=False,label='watch this tie') class Remark(models.Model): subject = models.CharField(max_length=100) mail = models.EmailField() topic = models.CharField(max_length=100) message = models.CharField(max_length=300) cc_myself = models.BooleanField() def __unicode__(self): return self.subject class Meta: ordering = ['subject'] admin.site.register([Remark,])
Everyone has seen it, models.py file There is an additional subclass of forms, this time because our operation involves web forms. In this case, it is best to create a Form form class for each model class to facilitate obtaining cleaned_data from the form.
url mapping file urls.py
This file is relatively simple, as follows:
"""FormRelative URL Configuration The `urlpatterns` list routes URLs to views. For more information please see: https://docs.djangoproject.com/en/1.9/topics/http/urls/ Examples: Function views 1. Add an import: from my_app import views 2. Add a URL to urlpatterns: url(r'^$', views.home, name='home') Class-based views 1. Add an import: from other_app.views import Home 2. Add a URL to urlpatterns: url(r'^$', Home.as_view(), name='home') Including another URLconf 1. Import the include() function: from django.conf.urls import url, include 2. Add a URL to urlpatterns: url(r'^blog/', include('blog.urls')) """ from django.conf.urls import url from django.contrib import admin from app.views import * urlpatterns = [ url(r'^admin/', admin.site.urls), url(r'^remark/$',reamark), ] 视图层views.py
This file determines the display view corresponding to the mapping file, so compare importance.
from django.shortcuts import render from app.models import * from django.http import * # Create your views here. # subject = models.CharField(max_length=100) # mail = models.EmailField() # topic = models.CharField(max_length=100) # message = models.CharField(max_length=300) # cc_myself = models.BooleanField() def reamark(request): if request.method =="POST": form = RemarkForm(request.POST) if form.is_valid(): myremark = Remark() myremark.subject=form.cleaned_data['subject'] myremark.mail = form.cleaned_data['mail'] myremark.topic = form.cleaned_data['topic'] myremark.message = form.cleaned_data['message'] myremark.cc_myself = form.cleaned_data['cc_myself'] myremark.save() # return HttpResponse("Publish Success!") else: form = RemarkForm() ctx = { 'form':form, 'ties':Remark.objects.all() } return render(request,'message.html',ctx)
Templates templates/message.html
The use of templates greatly reduces the amount of data and enables more flexible data display The separation of layers reduces the coupling between modules.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> <form action="." method="post"> {% for field in form %} {% csrf_token %} <div> {{ field.label_tag }}:{{ field }} {{ field.errors }} </div> {% endfor %} <div> <input type="submit" value="Remark"> </div> </form> <hr> {% for tie in ties %} <div> <ul> <li>{{ tie.subject }}</li> <li>{{ tie.mail}}</li> <li>{{ tie.topic}}</li> <li>{{ tie.message }}</li> <li>{{ tie.cc_myself }}</li> </ul> <hr> </div> {% endfor%} </body> </html>
Note that the reissue tags and template variables have been declared in the remark method of views.py, so they can be used directly.
Initialize the database
The sqlite database is used here. The configuration in the settings.py file is as follows;
# Database # https://docs.djangoproject.com/en/1.9/ref/settings/#databases DATABASES = { 'default': { 'ENGINE': 'django.db.backends.sqlite3', 'NAME': os.path.join(BASE_DIR, 'db.sqlite3'), } }
Then in the terminal Environment, enter the following commands:
// 创建数据库表结构 python manage.py makemigrations python manage.py migrate // 按照提示进行操作即可,目的是为了创建一个超级管理员 python createsuperuser //在自带的开发服务器上运行我们的项目 python manage.py runserver
Debug Verification
Here we enter in the browser
127.0.0.1:8000/admin
You can see the following
Then enter 127.0.0.1:8000/remark
Database side:
In this way, except for the beautification of the interface, the rest is completed.
Summary
Although this is a very simple example, I also discovered some conceptual problems of my own, such as the unreasonable model design because there is no comment time. This seems very embarrassing.
Then
if request.method =="POST": form = RemarkForm(request.POST) if form.is_valid(): myremark = Remark() myremark.subject=form.cleaned_data['subject'] myremark.mail = form.cleaned_data['mail'] myremark.topic = form.cleaned_data['topic'] myremark.message = form.cleaned_data['message'] myremark.cc_myself = form.cleaned_data['cc_myself'] myremark.save() # return HttpResponse("Publish Success!") else: form = RemarkForm() ctx = { 'form':form, 'ties':Remark.objects.all() } return render(request,'message.html',ctx)
This code, the action in the corresponding form is. This means that the form is submitted to this page, and the comment on the form data is realized. , this is very clever. And there is another advantage of using this feature of Django, that is, asynchronous loading of comments can still be achieved without manually refreshing the page.
Finally, it is the consistency of the Remark model and the RemarkForm form attributes in the model. You should pay special attention to this!
Okay, that’s it for today. Due to my average ability, if there are any mistakes in the code or logic, everyone is welcome to criticize and correct me!
The above is the entire content of this article. I hope it will be helpful to everyone's learning. I also hope that everyone will support the PHP Chinese website.
For more Python Django uses forms to implement the comment function, please pay attention to the PHP Chinese website!
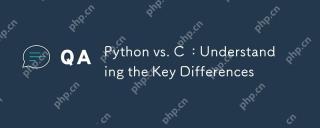
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
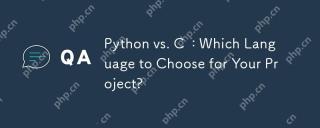
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
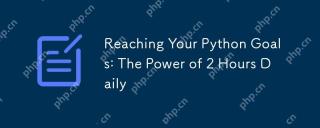
By investing 2 hours of Python learning every day, you can effectively improve your programming skills. 1. Learn new knowledge: read documents or watch tutorials. 2. Practice: Write code and complete exercises. 3. Review: Consolidate the content you have learned. 4. Project practice: Apply what you have learned in actual projects. Such a structured learning plan can help you systematically master Python and achieve career goals.
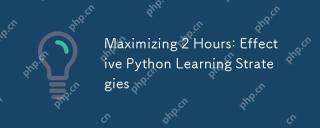
Methods to learn Python efficiently within two hours include: 1. Review the basic knowledge and ensure that you are familiar with Python installation and basic syntax; 2. Understand the core concepts of Python, such as variables, lists, functions, etc.; 3. Master basic and advanced usage by using examples; 4. Learn common errors and debugging techniques; 5. Apply performance optimization and best practices, such as using list comprehensions and following the PEP8 style guide.

Python is suitable for beginners and data science, and C is suitable for system programming and game development. 1. Python is simple and easy to use, suitable for data science and web development. 2.C provides high performance and control, suitable for game development and system programming. The choice should be based on project needs and personal interests.
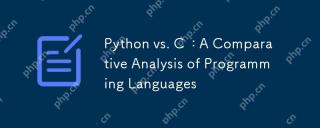
Python is more suitable for data science and rapid development, while C is more suitable for high performance and system programming. 1. Python syntax is concise and easy to learn, suitable for data processing and scientific computing. 2.C has complex syntax but excellent performance and is often used in game development and system programming.
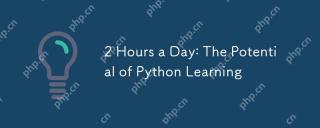
It is feasible to invest two hours a day to learn Python. 1. Learn new knowledge: Learn new concepts in one hour, such as lists and dictionaries. 2. Practice and exercises: Use one hour to perform programming exercises, such as writing small programs. Through reasonable planning and perseverance, you can master the core concepts of Python in a short time.
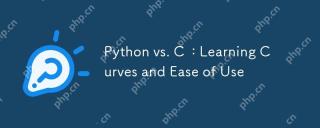
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
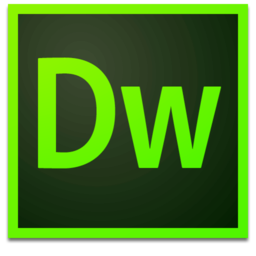
Dreamweaver Mac version
Visual web development tools
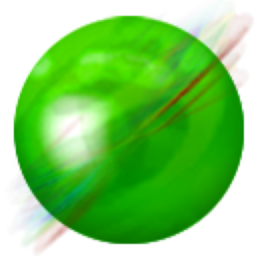
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software