There are many tools that can be used to convert json string to Java object. The following small example is just my practice
import java.util.ArrayList; import java.util.HashMap; import java.util.List; import java.util.Map; import com.jfinal.kit.JsonKit; public class JsonToJavaObject { public static void main(String[] args) { Object o1 = parse("{\"aa\":123,cc:[1,2,3,4,{cd:f,bb:234}]}"); System.out.println(JsonKit.toJson(o1)); } public static Object parse(String json){ if(json == null){ return null; } json = json.trim(); if("string".equals(typeof(json))){ return json; } if("map".equals(typeof(json))){ return parseMap(json); } if("list".equals(typeof(json))){ return parseList(json); } return null; } public static Map parseMap(String json){ if(!"map".equals(typeof(json))){ throw new RuntimeException("json 不是Map类型"); } Map r = new HashMap(); parseToken(r,json,null); return r; } public static List parseList(String json){ if(!"list".equals(typeof(json))){ throw new RuntimeException("json 不是list类型"); } List r = new ArrayList(); parseToken(null, json, r); return r; } public static String typeof(String json){ if(json.length() == 0)return "string"; if('{'==json.charAt(0)){ if('}' == json.charAt(json.length()-1)){ return "map"; } } if('['==json.charAt(0)){ if(']'==json.charAt(json.length()-1)){ return "list"; } } return "string"; } private static void parseToken(Map r, String json,List r2) { boolean syh = true; //双引号 boolean dyh = true;//单引号 boolean dkh = true;//大括号 boolean zkh = true;//中括号 boolean isKey = true; StringBuffer key = new StringBuffer(); StringBuffer value = new StringBuffer(); for(int i=1;i<json.length()-1;i++){ char item = json.charAt(i); if(dyh&&syh&&zkh)if('{' == item || '}' == item){ dkh = !dkh; } if(dyh&&syh&&dkh)if('[' == item || ']' == item){ zkh = !zkh; } if(dyh&&dkh&&zkh)if('"' == item){ syh = !syh; continue; } if(syh&&dkh&&zkh)if(syh)if('\'' == item){ dyh = !dyh; continue; } if(dyh&&syh&&dkh&&zkh)if(r2==null)if(dyh)if(':'==item){ isKey = false; continue; } if(dyh&&syh&&dkh&&zkh)if(','==item){ isKey = true; if(r != null){ r.put(key.toString(), parse(value.toString())); } if(r2 != null){ r2.add(parse(key.toString())); } key = new StringBuffer(); value = new StringBuffer(); continue; } if(isKey){ key.append(item); }else{ value.append(item); } } if(!key.toString().trim().equals("")){ if(r != null){ if(value.toString().trim().equals(""))throw new RuntimeException("json 格式错误"); r.put(key.toString(), parse(value.toString())); } if(r2 != null){ r2.add(parse(key.toString())); } } } }
Console output
{"aa":"123","cc":["1","2","3","4",{"bb":"234","cd":"f"}]}
More json to java object examples related articles Please pay attention to PHP Chinese website!
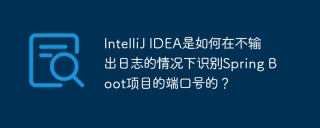
Start Spring using IntelliJIDEAUltimate version...
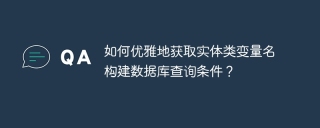
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
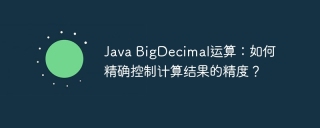
Java...
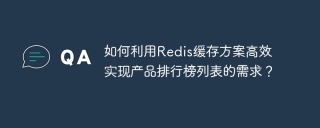
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
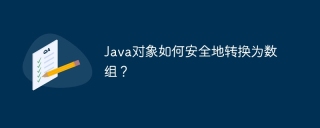
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
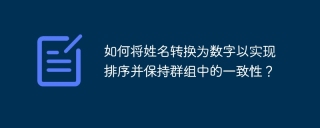
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
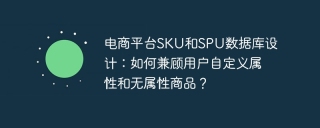
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
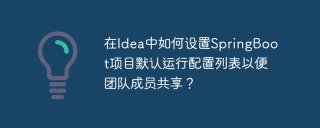
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.