


A brief introduction to the responsibility chain model in C++ design patterns
ChainOfResponsibility: Allows multiple objects to have the opportunity to process requests, thereby avoiding the coupling relationship between the sender and receiver of the request. Chain this object and pass the request along the chain until an object handles it.
Benefits of chain of responsibility:
When a client submits a request, the request is passed along the chain until a ConcreteHandler object is responsible for processing it.
So that neither the receiver nor the sender has clear information about the other party, and the objects in the chain themselves do not know the structure of the chain. The result is that chains of responsibility simplify linking objects so that they only need to maintain a single reference to its successor, rather than to all of its candidate recipients.
Client:
[code]//Client int main(){ Handler *h1 = new ConcreteHandler; Handler *h2 = new ConcreteHandler2; Handler *h3 = new ConcreteHandler3; //设置职责链上家与下家 h1->setSuccessor(h2); h2->setSuccessor(h3); //请求 int request[8] = {2, 5, 14, 22, 18, 3, 23, 20}; //循环给最小处理者提交请求,不同的数额由不同权限处理者处理 for(auto i = 0; i < 8; ++i){ h1->HandleRequest(request[i]); } // Output: // Handler request // Handler request // Handler2 request // Handler3 request // Handler2 request // Handler request // Handler3 request // Handler3 request return 0; }
Class implementation:
[code]//Handler处理类 class Handler{ protected: Handler *successor; public: //设置继任者 void setSuccessor(Handler *successor){ this->successor = successor; } //处理请求的抽象方法 virtual void HandleRequest(int request){} }; //具体处理类,处理它们负责的请求,可访问的后继者,如果可以处理该请求,就处理之,否则就将该请求转发给它的后继者 class ConcreteHandler: public Handler{ public: virtual void HandleRequest(int request)override{ //0~10在此处理 if(request >= 0 && request < 10){ std::cout << "Handler request\n"; }else if(successor != NULL) //否则转移到下一位 successor->HandleRequest(request); } }; class ConcreteHandler2: public Handler{ public: virtual void HandleRequest(int request)override{ //10~20在此处理 if(request >= 10 && request < 20){ std::cout << "Handler2 request\n"; }else if(successor != NULL) //否则转移到下一位 successor->HandleRequest(request); } }; class ConcreteHandler3: public Handler{ public: virtual void HandleRequest(int request)override{ //20~30在此处理 if(request >= 20 && request < 30){ std::cout << "Handler3 request\n"; }else if(successor != NULL) //否则转移到下一位 successor->HandleRequest(request); } };
The above is the content of a brief understanding of the responsibility chain model of C++ design patterns. For more related content, please pay attention to the PHP Chinese website (www.php.cn)!
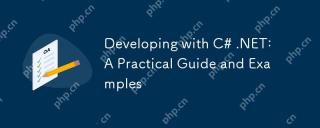
C# and .NET provide powerful features and an efficient development environment. 1) C# is a modern, object-oriented programming language that combines the power of C and the simplicity of Java. 2) The .NET framework is a platform for building and running applications, supporting multiple programming languages. 3) Classes and objects in C# are the core of object-oriented programming. Classes define data and behaviors, and objects are instances of classes. 4) The garbage collection mechanism of .NET automatically manages memory to simplify the work of developers. 5) C# and .NET provide powerful file operation functions, supporting synchronous and asynchronous programming. 6) Common errors can be solved through debugger, logging and exception handling. 7) Performance optimization and best practices include using StringBuild
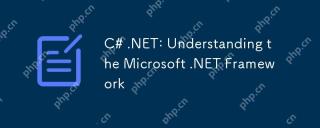
.NETFramework is a cross-language, cross-platform development platform that provides a consistent programming model and a powerful runtime environment. 1) It consists of CLR and FCL, which manages memory and threads, and FCL provides pre-built functions. 2) Examples of usage include reading files and LINQ queries. 3) Common errors involve unhandled exceptions and memory leaks, and need to be resolved using debugging tools. 4) Performance optimization can be achieved through asynchronous programming and caching, and maintaining code readability and maintainability is the key.
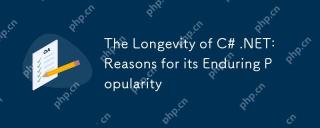
Reasons for C#.NET to remain lasting attractive include its excellent performance, rich ecosystem, strong community support and cross-platform development capabilities. 1) Excellent performance and is suitable for enterprise-level application and game development; 2) The .NET framework provides a wide range of class libraries and tools to support a variety of development fields; 3) It has an active developer community and rich learning resources; 4) .NETCore realizes cross-platform development and expands application scenarios.
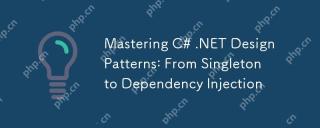
Design patterns in C#.NET include Singleton patterns and dependency injection. 1.Singleton mode ensures that there is only one instance of the class, which is suitable for scenarios where global access points are required, but attention should be paid to thread safety and abuse issues. 2. Dependency injection improves code flexibility and testability by injecting dependencies. It is often used for constructor injection, but it is necessary to avoid excessive use to increase complexity.
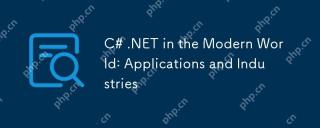
C#.NET is widely used in the modern world in the fields of game development, financial services, the Internet of Things and cloud computing. 1) In game development, use C# to program through the Unity engine. 2) In the field of financial services, C#.NET is used to develop high-performance trading systems and data analysis tools. 3) In terms of IoT and cloud computing, C#.NET provides support through Azure services to develop device control logic and data processing.
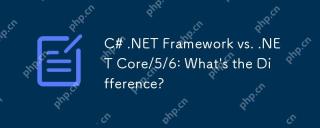
.NETFrameworkisWindows-centric,while.NETCore/5/6supportscross-platformdevelopment.1).NETFramework,since2002,isidealforWindowsapplicationsbutlimitedincross-platformcapabilities.2).NETCore,from2016,anditsevolutions(.NET5/6)offerbetterperformance,cross-
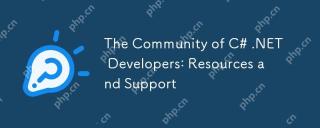
The C#.NET developer community provides rich resources and support, including: 1. Microsoft's official documents, 2. Community forums such as StackOverflow and Reddit, and 3. Open source projects on GitHub. These resources help developers improve their programming skills from basic learning to advanced applications.
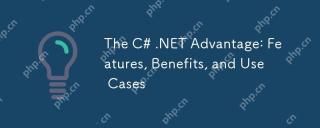
The advantages of C#.NET include: 1) Language features, such as asynchronous programming simplifies development; 2) Performance and reliability, improving efficiency through JIT compilation and garbage collection mechanisms; 3) Cross-platform support, .NETCore expands application scenarios; 4) A wide range of practical applications, with outstanding performance from the Web to desktop and game development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
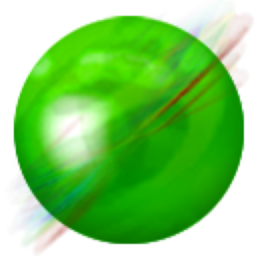
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version
