


Abstract Factory Pattern (Abstract Factory): Provides an interface for creating a series of related or interdependent objects without specifying their specific classes.
Pattern implementation:
[code]//create ProductA class ProductA{ public: virtual void Show() = 0; }; class ProductA1: public ProductA{ public: void Show(){ std::cout << "I'm ProductA1\n"; } }; class ProductA2: public ProductA{ public: void Show(){ std::cout << "I'm ProductA2\n"; } }; //create ProductB class ProductB{ public: virtual void Show() = 0; }; class ProductB1: public ProductB{ public: void Show(){ std::cout << "I'm ProductB1\n"; } }; class ProductB2: public ProductB{ public: void Show(){ std::cout << "I'm ProductB2\n"; } }; class Factory{ public: virtual ProductA *CreateProductA() = 0; virtual ProductB *CreateProductB() = 0; }; class Factory1: public Factory{ public: ProductA *CreateProductA(){ return new ProductA1(); } ProductB *CreateProductB(){ return new ProductB1(); } }; class Factory2: public Factory{ public: ProductA *CreateProductA(){ return new ProductA2(); } ProductB *CreateProductB(){ return new ProductB2(); } }; template <typename T> void del(T* obj){ if( obj != NULL){ delete obj; obj = NULL; } }
Client:
[code]int main(){ Factory *factoryObj1 = new Factory1(); ProductA *productObjA1 = factoryObj1->CreateProductA(); ProductB *productObjB1 = factoryObj1->CreateProductB(); productObjA1->Show(); //Output: I'm ProductA1 productObjB1->Show(); //Output: I'm ProductB1 Factory *factoryObj2 = new Factory2(); ProductA *productObjA2 = factoryObj2->CreateProductA(); ProductB *productObjB2 = factoryObj2->CreateProductB(); productObjA2->Show(); //Output:I'm ProductA2 productObjB2->Show(); //Output:I'm ProductB2 del(productObjB2); del(productObjA2); del(factoryObj2); del(productObjB1); del(productObjA1); del(factoryObj1); return 0; }
The above is the content of the abstract factory pattern of C++ design patterns. For more related content, please pay attention to the PHP Chinese website (www .php.cn)!
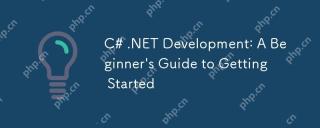
To start C#.NET development, you need to: 1. Understand the basic knowledge of C# and the core concepts of the .NET framework; 2. Master the basic concepts of variables, data types, control structures, functions and classes; 3. Learn advanced features of C#, such as LINQ and asynchronous programming; 4. Be familiar with debugging techniques and performance optimization methods for common errors. With these steps, you can gradually penetrate the world of C#.NET and write efficient applications.
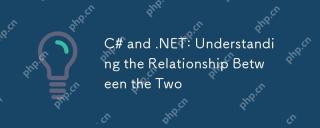
The relationship between C# and .NET is inseparable, but they are not the same thing. C# is a programming language, while .NET is a development platform. C# is used to write code, compile into .NET's intermediate language (IL), and executed by the .NET runtime (CLR).
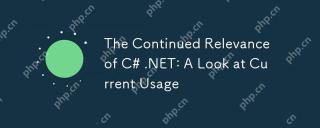
C#.NET is still important because it provides powerful tools and libraries that support multiple application development. 1) C# combines .NET framework to make development efficient and convenient. 2) C#'s type safety and garbage collection mechanism enhance its advantages. 3) .NET provides a cross-platform running environment and rich APIs, improving development flexibility.
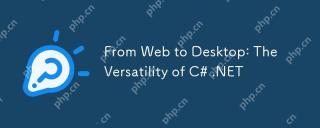
C#.NETisversatileforbothwebanddesktopdevelopment.1)Forweb,useASP.NETfordynamicapplications.2)Fordesktop,employWindowsFormsorWPFforrichinterfaces.3)UseXamarinforcross-platformdevelopment,enablingcodesharingacrossWindows,macOS,Linux,andmobiledevices.
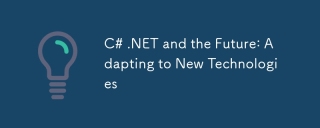
C# and .NET adapt to the needs of emerging technologies through continuous updates and optimizations. 1) C# 9.0 and .NET5 introduce record type and performance optimization. 2) .NETCore enhances cloud native and containerized support. 3) ASP.NETCore integrates with modern web technologies. 4) ML.NET supports machine learning and artificial intelligence. 5) Asynchronous programming and best practices improve performance.
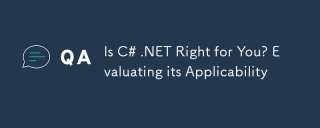
C#.NETissuitableforenterprise-levelapplicationswithintheMicrosoftecosystemduetoitsstrongtyping,richlibraries,androbustperformance.However,itmaynotbeidealforcross-platformdevelopmentorwhenrawspeediscritical,wherelanguageslikeRustorGomightbepreferable.
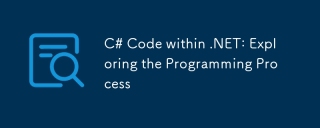
The programming process of C# in .NET includes the following steps: 1) writing C# code, 2) compiling into an intermediate language (IL), and 3) executing by the .NET runtime (CLR). The advantages of C# in .NET are its modern syntax, powerful type system and tight integration with the .NET framework, suitable for various development scenarios from desktop applications to web services.
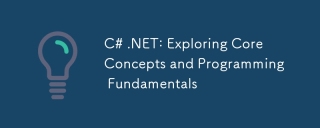
C# is a modern, object-oriented programming language developed by Microsoft and as part of the .NET framework. 1.C# supports object-oriented programming (OOP), including encapsulation, inheritance and polymorphism. 2. Asynchronous programming in C# is implemented through async and await keywords to improve application responsiveness. 3. Use LINQ to process data collections concisely. 4. Common errors include null reference exceptions and index out-of-range exceptions. Debugging skills include using a debugger and exception handling. 5. Performance optimization includes using StringBuilder and avoiding unnecessary packing and unboxing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
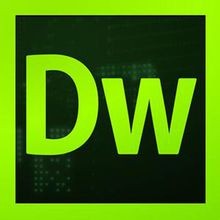
Dreamweaver CS6
Visual web development tools
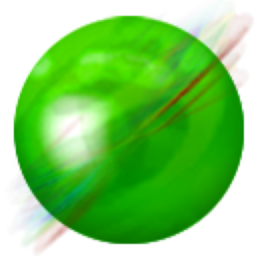
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment