First of all, the following three points need to be considered during development:
1. Entering and pop-up animation effects.
2. Z-index control
3. Overlay masking layer
About animation
vue's processing of animation is relatively simple, just add css transition animation to the component
<template> <div class="modal" transition="modal-scale"> <!--省略其它内容--> </div> </template> <script> // ... </script> <style> .modal-scale-transition{ transition: transform,opacity .3s ease; } .modal-scale-enter, .modal-scale-leave { opacity: 0; } .modal-scale-enter { transform: scale(1.1); } .modal-scale-leave { transform: scale(0.8); } </style>
The external can be controlled by the user, using v-if or v-show to control the display
Z-index control
Regarding the control of z-index, the following points need to be completed
1. Ensure that the z-index of the pop-up box is high enough to make it The outermost layer
2. The z-index of the pop-up box that pops up later is higher than the one that pops up before
To satisfy the above two points, we need the following code to implement
const zIndex = 20141223 // 先预设较高值 const getZIndex = function () { return zIndex++ // 每次获取之后 zindex 自动增加 }
Then bind the z-index to the component
<template> <div class="modal" :style="{'z-index': zIndex}" transition="modal-scale"> <!--省略其它内容--> </div> </template> <script> export default { data () { return { zIndex: getZIndex() } } } </script>
Overlay control of the covering layer
The covering layer is the most difficult part to handle in the pop-up window component. A perfect covering layer control needs to complete the following points:
1. The animation between the covering layer and the pop-up layer needs to be parallel
2. The z-index of the covering layer should be smaller than the pop-up layer
3. When the covering layer pops up, the component page needs to be scrolled
4. Clicking on the covering layer requires feedback on the pop-up layer
5. Ensure that there can be at most one covering layer on the entire page (multiple stacking together will deepen the color of the covering layer)
In order to deal with these problems and ensure that all pop-up box components do not need to be solved individually, we decided to use vue's mixins mechanism to encapsulate the public logic of these pop-up layers into a mixin layer, and each pop-up box component can be directly referenced. .
vue-popup-mixin
After clarifying all the above problems and starting to develop mixin, we first need an overlay (covering layer component);
<template> <div class="overlay" @click="handlerClick" @touchmove="prevent" :style="style" transition="overlay-fade"></div> </template> <script> export default { props: { onClick: { type: Function }, opacity: { type: Number, default: 0.4 }, color: { type: String, default: '#000' } }, computed: { style () { return { 'opacity': this.opacity, 'background-color': this.color } } }, methods: { prevent (event) { event.preventDefault() event.stopPropagation() }, handlerClick () { if (this.onClick) { this.onClick() } } } } </script> <style lang="less"> .overlay { position: fixed; left: 0; right: 0; top: 0; bottom: 0; background-color: #000; opacity: .4; z-index: 1000; } .overlay-fade-transition { transition: all .3s linear; &.overlay-fade-enter, &.overlay-fade-leave { opacity: 0 !important; } } </style>
Then you need a js to manage the display and hiding of overlay.
import Vue from 'vue' import overlayOpt from '../overlay' // 引入 overlay 组件 const Overlay = Vue.extend(overlayOpt) const getDOM = function (dom) { if (dom.nodeType === 3) { dom = dom.nextElementSibling || dom.nextSibling getDOM(dom) } return dom } // z-index 控制 const zIndex = 20141223 const getZIndex = function () { return zIndex++ } // 管理 const PopupManager = { instances: [], // 用来储存所有的弹出层实例 overlay: false, // 弹窗框打开时 调用此方法 open (instance) { if (!instance || this.instances.indexOf(instance) !== -1) return // 当没有遮盖层时,显示遮盖层 if (this.instances.length === 0) { this.showOverlay(instance.overlayColor, instance.overlayOpacity) } this.instances.push(instance) // 储存打开的弹出框组件 this.changeOverlayStyle() // 控制不同弹出层 透明度和颜色 // 给弹出层加上z-index const dom = getDOM(instance.$el) dom.style.zIndex = getZIndex() }, // 弹出框关闭方法 close (instance) { let index = this.instances.indexOf(instance) if (index === -1) return Vue.nextTick(() => { this.instances.splice(index, 1) // 当页面上没有弹出层了就关闭遮盖层 if (this.instances.length === 0) { this.closeOverlay() } this.changeOverlayStyle() }) }, showOverlay (color, opacity) { let overlay = this.overlay = new Overlay({ el: document.createElement('div') }) const dom = getDOM(overlay.$el) dom.style.zIndex = getZIndex() overlay.color = color overlay.opacity = opacity overlay.onClick = this.handlerOverlayClick.bind(this) overlay.$appendTo(document.body) // 禁止页面滚动 this.bodyOverflow = document.body.style.overflow document.body.style.overflow = 'hidden' }, closeOverlay () { if (!this.overlay) return document.body.style.overflow = this.bodyOverflow let overlay = this.overlay this.overlay = null overlay.$remove(() => { overlay.$destroy() }) }, changeOverlayStyle () { if (!this.overlay || this.instances.length === 0) return const instance = this.instances[this.instances.length - 1] this.overlay.color = instance.overlayColor this.overlay.opacity = instance.overlayOpacity }, // 遮盖层点击处理,会自动调用 弹出层的 overlayClick 方法 handlerOverlayClick () { if (this.instances.length === 0) return const instance = this.instances[this.instances.length - 1] if (instance.overlayClick) { instance.overlayClick() } } } window.addEventListener('keydown', function (event) { if (event.keyCode === 27) { // ESC if (PopupManager.instances.length > 0) { const topInstance = PopupManager.instances[PopupManager.instances.length - 1] if (!topInstance) return if (topInstance.escPress) { topInstance.escPress() } } } }) export default PopupManager
Finally encapsulate it into a mixin
import PopupManager from './popup-manager' export default { props: { show: { type: Boolean, default: false }, // 是否显示遮盖层 overlay: { type: Boolean, default: true }, overlayOpacity: { type: Number, default: 0.4 }, overlayColor: { type: String, default: '#000' } }, // 组件被挂载时会判断show的值开控制打开 attached () { if (this.show && this.overlay) { PopupManager.open(this) } }, // 组件被移除时关闭 detached () { PopupManager.close(this) }, watch: { show (val) { // 修改 show 值是调用对于的打开关闭方法 if (val && this.overlay) { PopupManager.open(this) } else { PopupManager.close(this) } } }, beforeDestroy () { PopupManager.close(this) } }
<template> <div class="dialog" v-show="show" transition="dialog-fade"> <div class="dialog-content"> <slot></slot> </div> </div> </template> <style> .dialog { left: 50%; top: 50%; transform: translate(-50%, -50%); position: fixed; width: 90%; } .dialog-content { background: #fff; border-radius: 8px; padding: 20px; text-align: center; } .dialog-fade-transition { transition: opacity .3s linear; } .dialog-fade-enter, .dialog-fade-leave { opacity: 0; } </style> <script> import Popup from '../src' export default { mixins: [Popup], methods: { // 响应 overlay事件 overlayClick () { this.show = false }, // 响应 esc 按键事件 escPress () { this.show = false } } } </script>SummaryThe above is about vue Some knowledge points about the .js pop-up component, I hope it will be helpful to everyone’s study or work. If you have any questions, you can leave a message for communication. Thank you for your support to the PHP Chinese website. For more knowledge points summary about vue.js pop-up component, please pay attention to the PHP Chinese website for related articles!
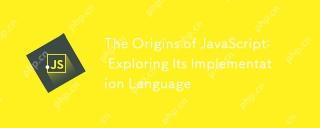
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
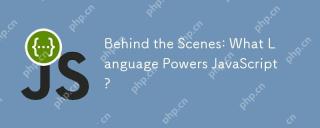
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
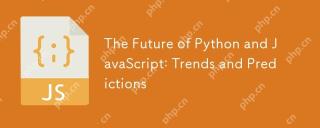
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
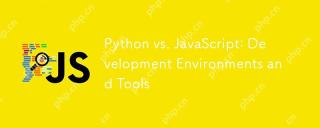
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
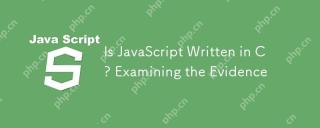
Yes, the engine core of JavaScript is written in C. 1) The C language provides efficient performance and underlying control, which is suitable for the development of JavaScript engine. 2) Taking the V8 engine as an example, its core is written in C, combining the efficiency and object-oriented characteristics of C. 3) The working principle of the JavaScript engine includes parsing, compiling and execution, and the C language plays a key role in these processes.
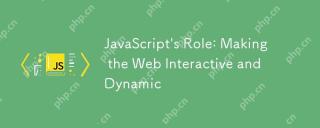
JavaScript is at the heart of modern websites because it enhances the interactivity and dynamicity of web pages. 1) It allows to change content without refreshing the page, 2) manipulate web pages through DOMAPI, 3) support complex interactive effects such as animation and drag-and-drop, 4) optimize performance and best practices to improve user experience.
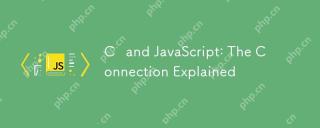
C and JavaScript achieve interoperability through WebAssembly. 1) C code is compiled into WebAssembly module and introduced into JavaScript environment to enhance computing power. 2) In game development, C handles physics engines and graphics rendering, and JavaScript is responsible for game logic and user interface.
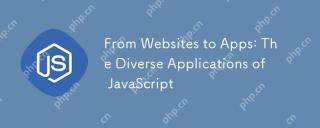
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
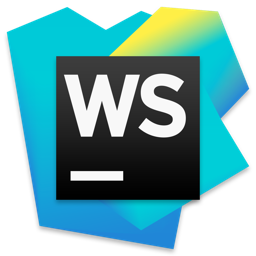
WebStorm Mac version
Useful JavaScript development tools
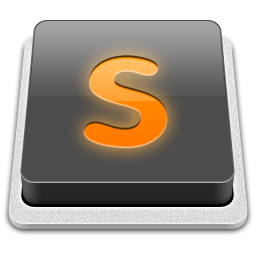
SublimeText3 Mac version
God-level code editing software (SublimeText3)
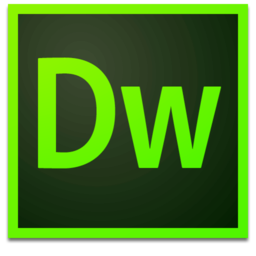
Dreamweaver Mac version
Visual web development tools

Atom editor mac version download
The most popular open source editor
