1: Recursive implementation
Use the formula f[n]=f[n-1]+f[n-2] and calculate recursively in sequence. The recursive end condition is f[1]=1, f[2] =1.
2: Array implementation
The space complexity and time complexity are both 0(n), the efficiency is average, and it is faster than recursion.
Three: Vector
The time complexity is 0(n), and the time complexity is 0(1). I just don’t know whether vector is efficient or not. Of course, vector has its own attributes that will occupy resources. .
Four: Queue
Of course, queues are more suitable for implementing Fibonacci sequences than arrays. The time complexity and space complexity are the same as vector
f(n)=f(n-1)+f(n-2), f(n) is only related to f(n-1) and f(n-2), after f(n) enters the queue, f( n-2) can be dequeued.
5: Iterative implementation
Iterative implementation is the most efficient, with time complexity of 0(n) and space complexity of 0(1).
6: Formula Implementation
When I was searching Baidu, I found that the Fibonacci sequence has a formula, so it can be calculated using the formula.
Since the accuracy of the double type is not enough, the results calculated by the program will have errors. If the formula is expanded and calculated, the result will be correct.
The complete implementation code is as follows:
#include "iostream" #include "queue" #include "cmath" using namespace std; int fib1(int index) //递归实现 { if(index<1) { return -1; } if(index==1 || index==2) return 1; return fib1(index-1)+fib1(index-2); } int fib2(int index) //数组实现 { if(index<1) { return -1; } if(index<3) { return 1; } int *a=new int[index]; a[0]=a[1]=1; for(int i=2;i<index;i++) a[i]=a[i-1]+a[i-2]; int m=a[index-1]; delete a; //释放内存空间 return m; } int fib3(int index) //借用vector<int>实现 { if(index<1) { return -1; } vector<int> a(2,1); //创建一个含有2个元素都为1的向量 a.reserve(3); for(int i=2;i<index;i++) { a.insert(a.begin(),a.at(0)+a.at(1)); a.pop_back(); } return a.at(0); } int fib4(int index) //队列实现 { if(index<1) { return -1; } queue<int>q; q.push(1); q.push(1); for(int i=2;i<index;i++) { q.push(q.front()+q.back()); q.pop(); } return q.back(); } int fib5(int n) //迭代实现 { int i,a=1,b=1,c=1; if(n<1) { return -1; } for(i=2;i<n;i++) { c=a+b; //辗转相加法(类似于求最大公约数的辗转相除法) a=b; b=c; } return c; } int fib6(int n) { double gh5=sqrt((double)5); return (pow((1+gh5),n)-pow((1-gh5),n))/(pow((double)2,n)*gh5); } int main(void) { printf("%d\n",fib3(6)); system("pause"); return 0; }
Seven: Bipartite Matrix Method
The code is posted below:
void multiply(int c[2][2],int a[2][2],int b[2][2],int mod) { int tmp[4]; tmp[0]=a[0][0]*b[0][0]+a[0][1]*b[1][0]; tmp[1]=a[0][0]*b[0][1]+a[0][1]*b[1][1]; tmp[2]=a[1][0]*b[0][0]+a[1][1]*b[1][0]; tmp[3]=a[1][0]*b[0][1]+a[1][1]*b[1][1]; c[0][0]=tmp[0]%mod; c[0][1]=tmp[1]%mod; c[1][0]=tmp[2]%mod; c[1][1]=tmp[3]%mod; }//计算矩阵乘法,c=a*b int fibonacci(int n,int mod)//mod表示数字太大时需要模的数 { if(n==0)return 0; else if(n<=2)return 1;//这里表示第0项为0,第1,2项为1 int a[2][2]={{1,1},{1,0}}; int result[2][2]={{1,0},{0,1}};//初始化为单位矩阵 int s; n-=2; while(n>0) { if(n%2 == 1) multiply(result,result,a,mod); multiply(a,a,a,mod); n /= 2; }//二分法求矩阵幂 s=(result[0][0]+result[0][1])%mod;//结果 return s; }The attached code is posted to calculate the nth power function of a using the bisection method.
int pow(int a,int n) { int ans=1; while(n) { if(n&1) ans*=a; a*=a; n>>=1; } return ans; }For more related articles on seven ways to achieve the general terms of the Fibonacci sequence, please pay attention to the PHP Chinese website!

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
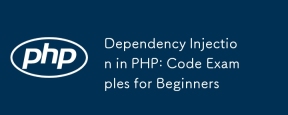
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
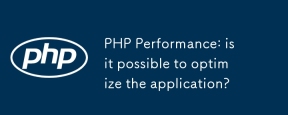
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
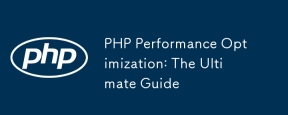
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
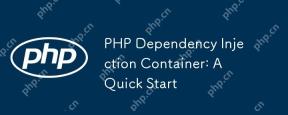
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
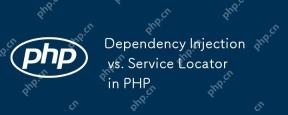
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
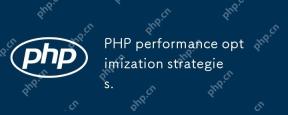
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
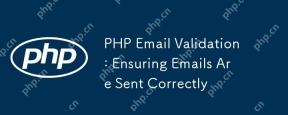
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
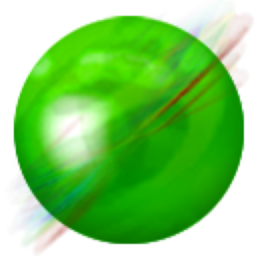
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use
