


This article uses a Python script to read image information. There are several instructions as follows:
1. Error handling is not implemented
2. All information is not read, probably only GPS information, Picture resolution, picture pixels, equipment manufacturer, shooting equipment, etc.
3. After simple modification, it should be possible to violently modify the GPS information of the picture
4. But for pictures that do not have GPS information themselves, The implementation is very complex and requires careful calculation of the offset of each descriptor.
After the script is run, the reading results are as follows
View here and Windows properties The content read by the device is exactly the same
The source code is as follows
# -*- coding:utf-8 -*- import binascii class ParseMethod(object): @staticmethod def parse_default(f, count, offset): pass @staticmethod def parse_latitude(f, count, offset): old_pos = f.tell() f.seek(12 + offset) latitude = [0,0,0] for i in xrange(count): byte = f.read(4) numerator = byte.encode('hex') byte = f.read(4) denominator = byte.encode('hex') latitude[i] = float(int(numerator, 16)) / int(denominator, 16) print 'Latitude:\t%.2f %.2f\' %.2f\"' % (latitude[0], latitude[1], latitude[2]) f.seek(old_pos) @staticmethod def parse_longtitude(f, count, offset): old_pos = f.tell() f.seek(12 + offset) longtitude = [0,0,0] for i in xrange(count): byte = f.read(4) numerator = byte.encode('hex') byte = f.read(4) denominator = byte.encode('hex') longtitude[i] = float(int(numerator, 16)) / int(denominator, 16) print 'Longtitude:\t%.2f %.2f\' %.2f\"' % (longtitude[0], longtitude[1], longtitude[2]) f.seek(old_pos) @staticmethod def parse_make(f, count, offset): old_pos = f.tell() f.seek(12 + offset) byte = f.read(count) a = byte.encode('hex') print 'Make:\t\t' + binascii.a2b_hex(a) f.seek(old_pos) @staticmethod def parse_model(f, count, offset): old_pos = f.tell() f.seek(12 + offset) byte = f.read(count) a = byte.encode('hex') print 'Model:\t\t' + binascii.a2b_hex(a) f.seek(old_pos) @staticmethod def parse_datetime(f, count, offset): old_pos = f.tell() f.seek(12 + offset) byte = f.read(count) a = byte.encode('hex') print 'DateTime:\t' + binascii.a2b_hex(a) f.seek(old_pos) # rational data type, 05 @staticmethod def parse_xresolution(f, count, offset): old_pos = f.tell() f.seek(12 + offset) byte = f.read(4) numerator = byte.encode('hex') byte = f.read(4) denominator = byte.encode('hex') xre = int(numerator, 16) / int(denominator, 16) print 'XResolution:\t' + str(xre) + ' dpi' f.seek(old_pos) @staticmethod def parse_yresolution(f, count, offset): old_pos = f.tell() f.seek(12 + offset) byte = f.read(4) numerator = byte.encode('hex') byte = f.read(4) denominator = byte.encode('hex') xre = int(numerator, 16) / int(denominator, 16) print 'YResolution:\t' + str(xre) + ' dpi' f.seek(old_pos) @staticmethod def parse_exif_ifd(f, count, offset): old_pos = f.tell() f.seek(12 + offset) byte = f.read(2) a = byte.encode('hex') exif_ifd_number = int(a, 16) for i in xrange(exif_ifd_number): byte = f.read(2) tag_id = byte.encode('hex') #print tag_id, byte = f.read(2) type_n = byte.encode('hex') #print type_n, byte = f.read(4) count = byte.encode('hex') #print count, byte = f.read(4) value_offset = byte.encode('hex') #print value_offset value_offset = int(value_offset, 16) EXIF_IFD_DICT.get(tag_id, ParseMethod.parse_default)(f, count, value_offset) f.seek(old_pos) @staticmethod def parse_x_pixel(f, count, value): print 'X Pixels:\t' + str(value) @staticmethod def parse_y_pixel(f, count, value): print 'y Pixels:\t' + str(value) @staticmethod def parse_gps_ifd(f, count, offset): old_pos = f.tell() f.seek(12 + offset) byte = f.read(2) a = byte.encode('hex') gps_ifd_number = int(a, 16) for i in xrange(gps_ifd_number): byte = f.read(2) tag_id = byte.encode('hex') #print tag_id, byte = f.read(2) type_n = byte.encode('hex') #print type_n, byte = f.read(4) count = byte.encode('hex') #print count, byte = f.read(4) value_offset = byte.encode('hex') #print value_offset count = int(count, 16) value_offset = int(value_offset, 16) GPS_IFD_DICT.get(tag_id, ParseMethod.parse_default)(f, count, value_offset) f.seek(old_pos) IFD_dict = { '010f' : ParseMethod.parse_make , '0110' : ParseMethod.parse_model , '0132' : ParseMethod.parse_datetime , '011a' : ParseMethod.parse_xresolution , '011b' : ParseMethod.parse_yresolution , '8769' : ParseMethod.parse_exif_ifd , '8825' : ParseMethod.parse_gps_ifd } EXIF_IFD_DICT = { 'a002' : ParseMethod.parse_x_pixel , 'a003' : ParseMethod.parse_y_pixel } GPS_IFD_DICT = { '0002' : ParseMethod.parse_latitude , '0004' : ParseMethod.parse_longtitude } with open('image.jpg', 'rb') as f: byte = f.read(2) a = byte.encode('hex') print 'SOI Marker:\t' + a byte = f.read(2) a = byte.encode('hex') print 'APP1 Marker:\t' + a byte = f.read(2) a = byte.encode('hex') print 'APP1 Length:\t' + str(int(a, 16)) + ' .Dec' byte = f.read(4) a = byte.encode('hex') print 'Identifier:\t' + binascii.a2b_hex(a) byte = f.read(2) a = byte.encode('hex') print 'Pad:\t\t' + a print print 'Begin to print Header.... ' print 'APP1 Body: ' byte = f.read(2) a = byte.encode('hex') print 'Byte Order:\t' + a byte = f.read(2) a = byte.encode('hex') print '42:\t\t' + a byte = f.read(4) a = byte.encode('hex') print '0th IFD Offset:\t' + a print 'Finish print Header' print 'Begin to print 0th IFD....' print #print 'Total: ', byte = f.read(2) a = byte.encode('hex') interoperability_number = int(a, 16) #print interoperability_number for i in xrange(interoperability_number): byte = f.read(2) tag_id = byte.encode('hex') #print tag_id, byte = f.read(2) type_n = byte.encode('hex') #print type_n, byte = f.read(4) count = byte.encode('hex') #print count, byte = f.read(4) value_offset = byte.encode('hex') #print value_offset count = int(count, 16) value_offset = int(value_offset, 16) # simulate switch IFD_dict.get(tag_id, ParseMethod.parse_default)(f, count, value_offset) print print 'Finish print 0th IFD....'
Summary
The implementation method of using Python to read image attribute information is here That’s basically it. Has everyone learned the lesson? I hope this article will bring some help to everyone's study or work.
For more related articles on how to implement Python to read image attribute information, please pay attention to the PHP Chinese website!
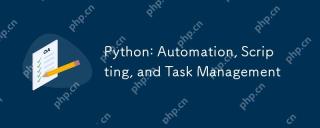
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
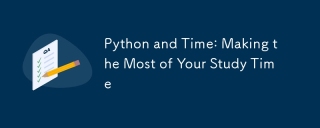
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
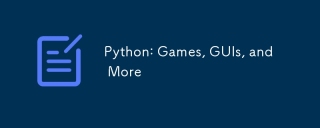
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
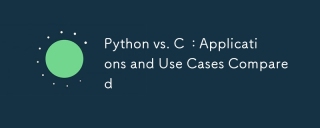
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
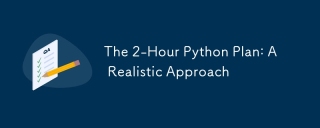
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
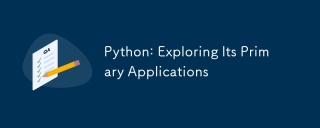
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
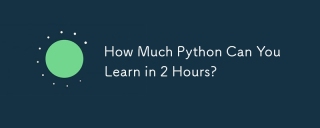
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
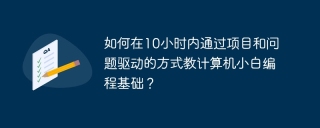
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
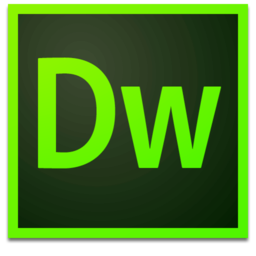
Dreamweaver Mac version
Visual web development tools
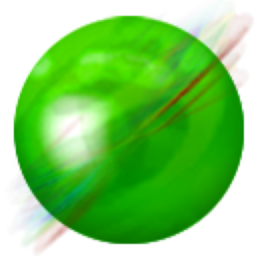
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.